public class BinaryTreeSearch {
public static void main(String[] args) {
BinarySearch binarySearch = new BinarySearch();
Data root = new Data(0);
Data one = new Data(1);
Data two = new Data(2);
Data three = new Data(3);
Data four = new Data(4);
Data five = new Data(5);
Data six = new Data(6);
Data seven = new Data(7);
Data eight = new Data(8);
binarySearch.setRoot(root);
root.left = one;
root.right = two;
one.left = three;
one.right = four;
two.left = five;
two.right = six;
three.right = seven;
five.left = eight;
System.out.println("-----先序查找-----");
System.out.println("查到数据:" + binarySearch.preSearch(5));
System.out.println("查找次数:" + binarySearch.count());
System.out.println("-----中序查找-----");
System.out.println("查到数据:" + binarySearch.infixSearch(0));
System.out.println("查找次数:" + binarySearch.count());
System.out.println("-----后序查找-----");
System.out.println("查到数据:" + binarySearch.postSearch(0));
System.out.println("查找次数:" + binarySearch.count());
}
}
class BinarySearch {
public Data root;
public void setRoot(Data root) {
this.root = root;
}
public int count() {
int n = Data.count;
Data.count = 0;
return n;
}
public Data preSearch(int n) {
if (root != null) {
return root.preSearch(n);
} else {
System.out.println("二叉树为空,无法遍历~");
return null;
}
}
public Data infixSearch(int n) {
if (root != null) {
return root.infixSearch(n);
} else {
System.out.println("二叉树为空,无法遍历~");
return null;
}
}
public Data postSearch(int n) {
if (root != null) {
return root.postSearch(n);
} else {
System.out.println("二叉树为空,无法遍历~");
return null;
}
}
}
class Data {
public int num;
public Data left;
public Data right;
public static int count;
public Data(int num) {
this.num = num;
}
@Override
public String toString() {
return num + "";
}
public Data preSearch(int n) {
count++;
if (this.num == n) {
return this;
}
Data temp = null;
if (this.left != null) {
temp = this.left.preSearch(n);
}
if (temp != null) {
return temp;
}
if (this.right != null) {
temp = this.right.preSearch(n);
}
return temp;
}
public Data infixSearch(int n) {
Data temp = null;
if (this.left != null) {
temp = this.left.infixSearch(n);
}
if (temp != null) {
return temp;
}
count++;
if (this.num == n) {
return this;
}
if (this.right != null) {
temp = this.right.infixSearch(n);
}
return temp;
}
public Data postSearch(int n) {
Data temp = null;
if (this.left != null) {
temp = this.left.postSearch(n);
}
if (temp != null) {
return temp;
}
if (this.right != null) {
temp = this.right.postSearch(n);
}
if (temp != null) {
return temp;
}
count++;
if (this.num == n) {
return this;
}
return temp;
}
}
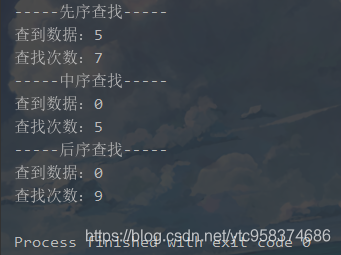