//官网 https://antv-x6.gitee.io/zh/docs/tutorial/getting-started
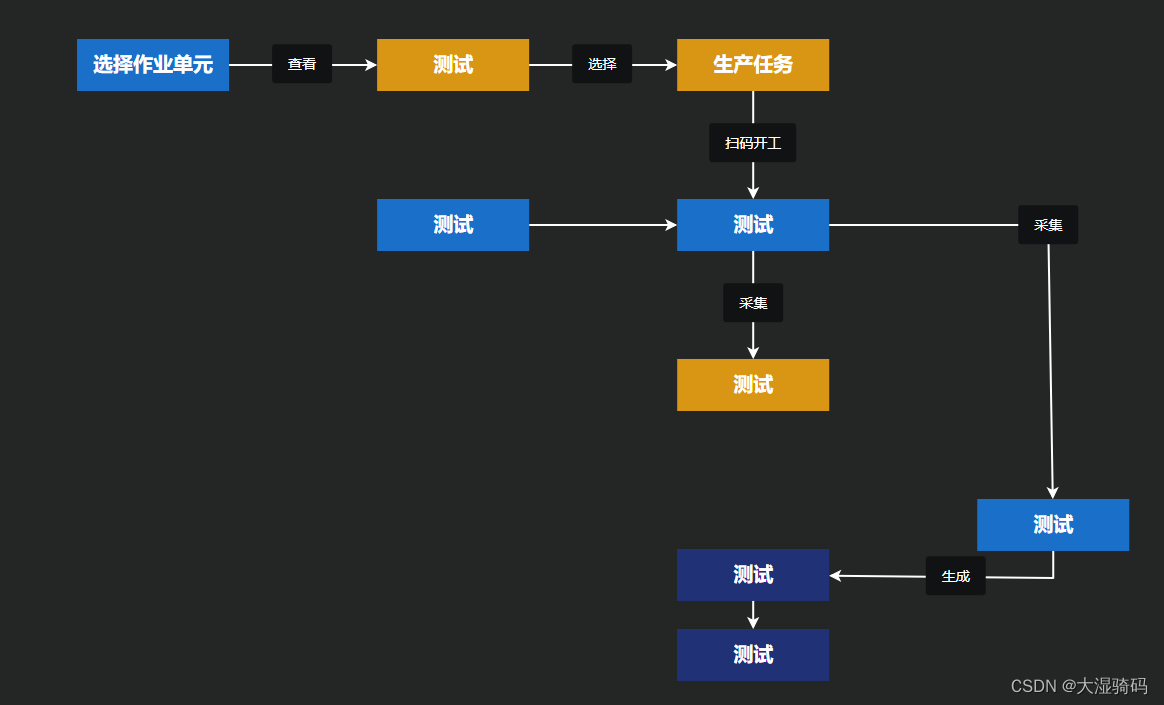
npm install @antv/x6 --save
import { Graph } from '@antv/x6';
//这个不能用v-show进行显示隐藏,目前我也不清楚为什么,因为我用v-show后,连接线会错乱。
<div id="container" style="width:100%;height:100%;"></div>
huihua(){
// 创建边
const graph = new Graph({
container: document.getElementById('container'),
// scroller: {
// enabled: true,
// pannable: true,
// pageVisible: true,
// pageBreak: false,
// },
mousewheel: {
enabled: true,
modifiers: ['ctrl', 'meta'],
},
connecting: {
allowBlank: true,
},
});
var node1_width=window.screen.width
var node1_height=window.screen.height
window.addEventListener("resize", () => {
node1_width=window.screen.width
node1_height=window.screen.height
console.log(node1_width,node1_height)
});
const node1 = graph.addNode({
x: 300, // Number,必选,节点位置的 x 值
y: 40, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'hello', // String,节点标签
attrs: {
body: {
fill: '#1A6FC9',
stroke: '#1A6FC9',
border:0,
},
label: {
text: '测试1',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const node2 = graph.addNode({
x: 600, // Number,必选,节点位置的 x 值
y: 40, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#D89614',
stroke: '#D89614',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试2',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge = graph.addEdge({
source: node1,
target: node2,
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
edge.setLabels({
attrs: {
text: {
text: '查看',
fill: '#ffffff'
},
rect: {
ref: 'label',
fill: '#111213',
rx: 3,
ry: 3,
refWidth: 30,
refHeight: 20,
refX: -15,
refY: -10,
},
},
})
const node3 = graph.addNode({
x: 900, // Number,必选,节点位置的 x 值
y: 40, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#D89614',
stroke: '#D89614',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试3',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge2 = graph.addEdge({
source: node2,
target: node3,
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
edge2.setLabels({
attrs: {
text: {
text: '选择',
fill: '#ffffff'
},
rect: {
ref: 'label',
fill: '#111213',
rx: 3,
ry: 3,
refWidth: 30,
refHeight: 20,
refX: -15,
refY: -10,
},
},
})
const node4 = graph.addNode({
x: 900, // Number,必选,节点位置的 x 值
y: 200, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#1A6FC9',
stroke: '#1A6FC9',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试4',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge3 = graph.addEdge({
source: node3,
target: node4,
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
edge3.setLabels({
attrs: {
text: {
text: '测试4',
fill: '#ffffff'
},
rect: {
ref: 'label',
fill: '#111213',
rx: 3,
ry: 3,
refWidth: 30,
refHeight: 20,
refX: -15,
refY: -10,
},
},
})
const node5 = graph.addNode({
x: 600, // Number,必选,节点位置的 x 值
y: 200, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#1A6FC9',
stroke: '#1A6FC9',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试5',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge4 = graph.addEdge({
source: node5,
target: node4,
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
const node6 = graph.addNode({
x: 900, // Number,必选,节点位置的 x 值
y: 360, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#D89614',
stroke: '#D89614',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试5',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge5 = graph.addEdge({
source: node4,
target: node6,
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
edge5.setLabels({
attrs: {
text: {
text: '采集',
fill: '#ffffff'
},
rect: {
ref: 'label',
fill: '#111213',
rx: 3,
ry: 3,
refWidth: 30,
refHeight: 20,
refX: -15,
refY: -10,
},
},
})
const node7 = graph.addNode({
x: 1200, // Number,必选,节点位置的 x 值
y: 500, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#1A6FC9',
stroke: '#1A6FC9',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试5',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge6 = graph.addEdge({
source: node4,
target: node7,
vertices: [ //相连途中需要途径两个点
{ x: 1270, y: 225}, //点1
// { x: 968, y: 242} //点2
],
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
edge6.setLabels({
attrs: {
text: {
text: '测试6',
fill: '#ffffff'
},
rect: {
ref: 'label',
fill: '#111213',
rx: 3,
ry: 3,
refWidth: 30,
refHeight: 20,
refX: -15,
refY: -10,
},
},
position: {
distance: 220,
},
})
const node8 = graph.addNode({
x: 900, // Number,必选,节点位置的 x 值
y: 550, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#203175',
stroke: '#203175',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试7',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge7 = graph.addEdge({
source: node7,
target: node8,
vertices: [ //相连途中需要途径两个点
{ x: 1275, y: 578}, //点1
// { x: 968, y: 242} //点2
],
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
edge7.setLabels({
attrs: {
text: {
text: '生成',
fill: '#ffffff'
},
rect: {
ref: 'label',
fill: '#111213',
rx: 3,
ry: 3,
refWidth: 30,
refHeight: 20,
refX: -15,
refY: -10,
},
},
})
const node9 = graph.addNode({
x: 900, // Number,必选,节点位置的 x 值
y: 630, // Number,必选,节点位置的 y 值
width: 150, // Number,可选,节点大小的 width 值
height: 50, // Number,可选,节点大小的 height 值
label: 'world', // String,节点标签
attrs: {
body: {
fill: '#203175',
stroke: '#203175',
// strokeDasharray: '10,2',
border:0,
},
label: {
text: '测试8',
fill: '#ffffff',
fontSize: 20,
fontWeight: 'bold',
}
}
})
const edge8 = graph.addEdge({
source: node8,
target: node9,
// vertices: [ //相连途中需要途径两个点
// { x: 1275, y: 578}, //点1
// // { x: 968, y: 242} //点2
// ],
attrs: {
line: {
stroke: '#ffffff',
},
},
},)
//graph为自定义的画布 为画布上的node绑定点击事件
graph.on('node:click', ({ view, e }) => {
e.stopPropagation() //禁止冒泡
console.log(view) //返回当前节点id等元素交流技术加企鹅330586621
console.log(e)
})
},
vue流程图简画
于 2022-10-26 10:15:19 首次发布