POI简单实例
1. 创建空的Excel
package com.hengxin.platform.demo;
import java.io.FileOutputStream;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
public class Test {
public static void main(String[] args) {
try {
HSSFWorkbook workbook = new HSSFWorkbook();
FileOutputStream out = new FileOutputStream("D:/课程表.xls");
workbook.write(out);
out.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
2. 单元格赋值
package com.hengxin.platform.demo
import java.io.FileOutputStream
import java.util.Random
import org.apache.poi.hpsf.DocumentSummaryInformation
import org.apache.poi.hpsf.SummaryInformation
import org.apache.poi.hssf.usermodel.HSSFCell
import org.apache.poi.hssf.usermodel.HSSFCellStyle
import org.apache.poi.hssf.usermodel.HSSFFont
import org.apache.poi.hssf.usermodel.HSSFRow
import org.apache.poi.hssf.usermodel.HSSFSheet
import org.apache.poi.hssf.usermodel.HSSFWorkbook
import org.apache.poi.hssf.util.HSSFColor
import org.apache.poi.ss.util.Region
public class TestExcel {
public static HSSFCellStyle getStyleHeader(HSSFWorkbook workbook) {
HSSFFont font = workbook.createFont()
font.setFontHeightInPoints((short) 12)
font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD)
font.setFontName("宋体")
HSSFCellStyle style = workbook.createCellStyle()
style.setAlignment(HSSFCellStyle.ALIGN_CENTER)
style.setFillForegroundColor(HSSFColor.SKY_BLUE.index)
style.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND)
//边框填充
style.setBorderTop(HSSFCellStyle.BORDER_THIN)
style.setTopBorderColor(HSSFColor.BLACK.index)
style.setBorderRight(HSSFCellStyle.BORDER_THIN)
style.setRightBorderColor(HSSFColor.BLACK.index)
style.setBorderBottom(HSSFCellStyle.BORDER_THIN)
style.setBottomBorderColor(HSSFColor.BLACK.index)
style.setBorderLeft(HSSFCellStyle.BORDER_THIN)
style.setLeftBorderColor(HSSFColor.BLACK.index)
style.setFont(font)
style.setWrapText(true)
style.setAlignment(HSSFCellStyle.ALIGN_LEFT)
style.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER)
return style
}
public static HSSFCellStyle getStyleBody(HSSFWorkbook workbook) {
HSSFFont font = workbook.createFont()
font.setFontHeightInPoints((short) 12)
font.setFontName("宋体")
HSSFCellStyle style = workbook.createCellStyle()
style.setBorderBottom(HSSFCellStyle.BORDER_THIN)
style.setBottomBorderColor(HSSFColor.BLACK.index)
style.setBorderLeft(HSSFCellStyle.BORDER_THIN)
style.setLeftBorderColor(HSSFColor.BLACK.index)
style.setBorderRight(HSSFCellStyle.BORDER_THIN)
style.setRightBorderColor(HSSFColor.BLACK.index)
style.setBorderTop(HSSFCellStyle.BORDER_THIN)
style.setTopBorderColor(HSSFColor.BLACK.index)
style.setFont(font)
style.setWrapText(true)
style.setAlignment(HSSFCellStyle.ALIGN_LEFT)
style.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER)
return style
}
public static void main(String[] args) {
try {
HSSFWorkbook workbook = new HSSFWorkbook()
HSSFSheet sheet = workbook.createSheet("课程表")
HSSFCellStyle headerStyle = getStyleHeader(workbook)
HSSFCellStyle bodyStyle = getStyleBody(workbook)
HSSFRow row = sheet.createRow(0)
HSSFCell cell00 = row.createCell(0)
cell00.setCellType(HSSFCell.CELL_TYPE_STRING)
cell00.setCellValue("星期一")
cell00.setCellStyle(headerStyle)
HSSFCell cell01 = row.createCell(1)
cell01.setCellType(HSSFCell.CELL_TYPE_STRING)
cell01.setCellValue("星期二")
cell01.setCellStyle(headerStyle)
HSSFCell cell02 = row.createCell(2)
cell02.setCellType(HSSFCell.CELL_TYPE_STRING)
cell02.setCellValue("星期三")
cell02.setCellStyle(headerStyle)
HSSFCell cell03 = row.createCell(3)
cell03.setCellType(HSSFCell.CELL_TYPE_STRING)
cell03.setCellValue("星期四")
cell03.setCellStyle(headerStyle)
HSSFCell cell04 = row.createCell(4)
cell04.setCellType(HSSFCell.CELL_TYPE_STRING)
cell04.setCellValue("星期五")
cell04.setCellStyle(headerStyle)
Random random = new Random()
String[] course = {"语文","数学","英语","物理","化学","政治","历史","音乐","美术","体育"}
for (int rowNo = 1
HSSFRow rowHSSF = sheet.createRow(rowNo)
for (int cellNo = 0
int i = random.nextInt(10)
HSSFCell cell = rowHSSF.createCell(cellNo)
cell.setCellValue(course[i])
cell.setCellType(HSSFCell.CELL_TYPE_STRING)
cell.setCellStyle(bodyStyle)
}
}
FileOutputStream out = new FileOutputStream("D:/课程表.xls")
workbook.write(out)
out.close()
} catch (Exception e) {
e.printStackTrace()
}
}
}
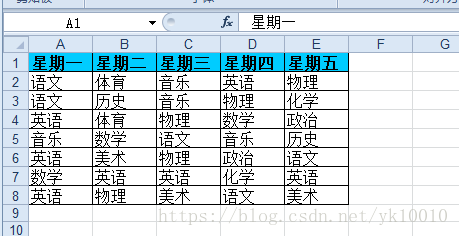
3. 单元格合并
public static void main(String[] args) {
try {
HSSFWorkbook workbook = new HSSFWorkbook()
HSSFSheet sheet = workbook.createSheet("课程表")
HSSFCellStyle headerStyle = getStyleHeader(workbook)
HSSFRow row00 = sheet.createRow(0)
HSSFCell row0_cell00 = row00.createCell(0)
row0_cell00.setCellType(HSSFCell.CELL_TYPE_STRING)
row0_cell00.setCellValue("课程表")
row0_cell00.setCellStyle(headerStyle)
HSSFCell row10_cell01 = row00.createCell(1)
row10_cell01.setCellType(HSSFCell.CELL_TYPE_STRING)
row10_cell01.setCellStyle(headerStyle)
Region region1 = new Region(0, (short) 0, 0, (short) 1)
sheet.addMergedRegion(region1)
FileOutputStream out=new FileOutputStream("D:/课程表.xls")
workbook.write(out)
out.close()
} catch (Exception e) {
e.printStackTrace()
}
}
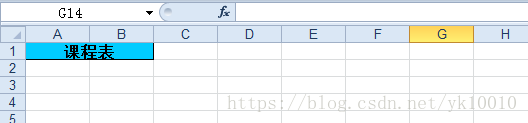
4. 创建文档摘要信息
public static void main(String[] args) {
try{
FileInputStream is = new FileInputStream("D:/课程表.xls");
HSSFWorkbook workbook = new HSSFWorkbook(is);
workbook.createInformationProperties();
DocumentSummaryInformation dsi= workbook.getDocumentSummaryInformation();
dsi.setCategory("类别:Excel文件");
dsi.setManager("管理者:Liki");
dsi.setCompany("公司:无");
SummaryInformation si = workbook.getSummaryInformation();
si.setSubject("主题:课程表");
si.setTitle("标题:初中课程表");
si.setAuthor("作者:zyn");
si.setComments("备注:课程表展示");
FileOutputStream out=new FileOutputStream("D:/课程表.xls");
workbook.write(out);
out.close();
is.close();
}catch(Exception e){
e.printStackTrace();
}
}
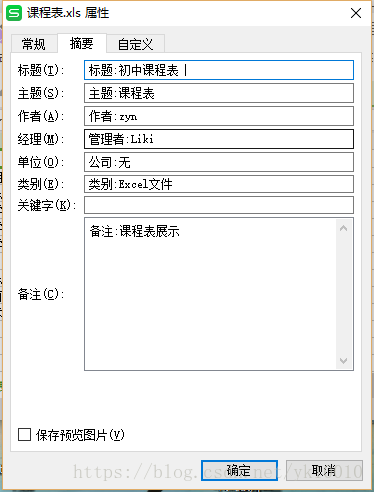
5. 设置批注
public static void main(String[] args) {
try{
FileInputStream is = new FileInputStream("D:/课程表.xls");
HSSFWorkbook workbook = new HSSFWorkbook(is);
HSSFSheet sheet=workbook.getSheet("课程表");
HSSFPatriarch patr = sheet.createDrawingPatriarch();
HSSFClientAnchor anchor = patr.createAnchor(0,0,0,0, 9,0, 11,6);
HSSFComment comment = patr.createCellComment(anchor);
comment.setString(new HSSFRichTextString("这是一个批注段落!"));
comment.setAuthor("HM");
comment.setVisible(true);
HSSFCell cell = sheet.getRow(0).getCell(0);
cell.setCellComment(comment);
FileOutputStream out=new FileOutputStream("D:/课程表.xls");
workbook.write(out);
out.close();
is.close();
}catch(Exception e){
e.printStackTrace();
}
}
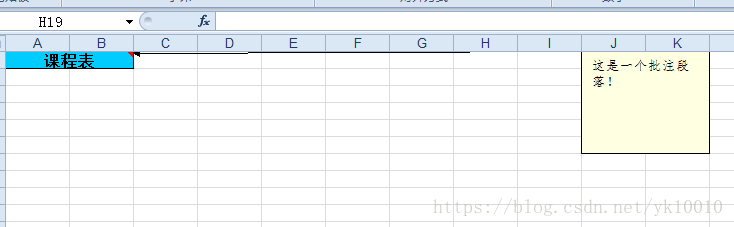
6. 单元格格式操作
public static void main(String[] args) {
try{
HSSFWorkbook workbook = new HSSFWorkbook()
HSSFSheet sheet=workbook.createSheet("格式转换")
HSSFRow row0=sheet.createRow(0)
Date date=new Date()
HSSFCell row1_cell1=row0.createCell(0)
HSSFCell row1_cell2=row0.createCell(1)
HSSFCell row1_cell3=row0.createCell(2)
row1_cell1.setCellValue(date)
row1_cell2.setCellValue(date)
row1_cell3.setCellValue(date)
HSSFCellStyle style1=workbook.createCellStyle()
style1.setDataFormat(HSSFDataFormat.getBuiltinFormat("m/d/yy h:mm"))
HSSFCellStyle style2=workbook.createCellStyle()
style2.setDataFormat(workbook.createDataFormat().getFormat("yyyy-mm-dd hh:m:ss"))
row1_cell2.setCellStyle(style1)
row1_cell3.setCellStyle(style2)
HSSFRow row1=sheet.createRow(1)
double db=3.1415926
HSSFCell row2_cell1=row1.createCell(0)
HSSFCell row2_cell2=row1.createCell(1)
HSSFCell row2_cell3=row1.createCell(2)
row2_cell1.setCellValue(db)
row2_cell2.setCellValue(db)
row2_cell3.setCellValue(db)
HSSFCellStyle style3=workbook.createCellStyle()
style3.setDataFormat(HSSFDataFormat.getBuiltinFormat("0.00"))
HSSFCellStyle style4=workbook.createCellStyle()
style4.setDataFormat(workbook.createDataFormat().getFormat("0.00"))
row2_cell2.setCellStyle(style3)
row2_cell3.setCellStyle(style4)
HSSFRow row2=sheet.createRow(2)
double money=12345.6789
HSSFCell row3_cell1=row2.createCell(0)
HSSFCell row3_cell2=row2.createCell(1)
HSSFCell row3_cell3=row2.createCell(2)
row3_cell1.setCellValue(money)
row3_cell2.setCellValue(money)
row3_cell3.setCellValue(money)
HSSFCellStyle style5=workbook.createCellStyle()
style5.setDataFormat(HSSFDataFormat.getBuiltinFormat("¥#,##0.00"))
HSSFCellStyle style6=workbook.createCellStyle()
style6.setDataFormat(workbook.createDataFormat().getFormat("¥#,##0.00"))
row3_cell2.setCellStyle(style3)
row3_cell3.setCellStyle(style4)
FileOutputStream out=new FileOutputStream("D:/格式转换.xls")
workbook.write(out)
out.close()
}catch(Exception e){
e.printStackTrace()
}
}
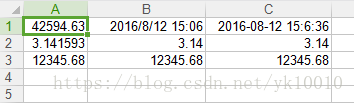
7. 基本计算
public static void main(String[] args) {
try{
HSSFWorkbook workbook = new HSSFWorkbook()
HSSFSheet sheet=workbook.createSheet("基本计算")
HSSFRow row=sheet.createRow(0)
HSSFCell cell0=row.createCell(0)
cell0.setCellFormula("5*5+2")
cell0=row.createCell(1)
cell0.setCellValue(20)
cell0=row.createCell(2)
cell0.setCellFormula("A1+B1")
cell0=row.createCell(3)
cell0.setCellFormula("A1-B1")
cell0=row.createCell(4)
cell0.setCellFormula("A1*B1")
cell0=row.createCell(5)
cell0.setCellFormula("A1/B1")
FileOutputStream out=new FileOutputStream("D:/基本计算.xls")
workbook.write(out)
out.close()
}catch(Exception e){
e.printStackTrace()
}
}

8. SUM函数
public static void main(String[] args) {
try{
HSSFWorkbook workbook = new HSSFWorkbook()
HSSFSheet sheet=workbook.createSheet("基本计算")
HSSFRow row=sheet.createRow(0)
row.createCell(0).setCellValue(1)
row.createCell(1).setCellValue(2)
row.createCell(2).setCellValue(3)
row.createCell(3).setCellValue(4)
row.createCell(4).setCellValue(5)
row.createCell(5).setCellValue(6)
row.createCell(6).setCellValue(7)
//第七/八列进行计算,两种都等价A1+B1+C1+D1+E1+F1+G1
row.createCell(7).setCellFormula("sum(A1,B1,C1,D1,E1,F1,G1)")
row.createCell(8).setCellFormula("sum(A1:G1)")
FileOutputStream out=new FileOutputStream("D:/基本计算.xls")
workbook.write(out)
out.close()
}catch(Exception e){
e.printStackTrace()
}
}

9. ABS绝对值、INT取整函数、ROUND四舍五入
public static void main(String[] args) {
try{
HSSFWorkbook workbook = new HSSFWorkbook();
HSSFSheet sheet=workbook.createSheet("基本计算");
HSSFRow row0=sheet.createRow(0);
row0.createCell(0).setCellValue(-1234);
row0.createCell(1).setCellValue(5678);
HSSFRow row1=sheet.createRow(1);
row1.createCell(0).setCellValue(23.456);
row1.createCell(1).setCellValue(-54.562);
HSSFRow row2=sheet.createRow(2);
row2.createCell(0).setCellValue(8.49314);
row2.createCell(1).setCellValue(12.927);
/**
* 取绝对值
*/
row0.createCell(2).setCellFormula("ABS(A1)");
row0.createCell(3).setCellFormula("ABS(B1)");
/**
* 取整
*/
row1.createCell(2).setCellFormula("INT(A2)");
row1.createCell(3).setCellFormula("INT(B2)");
/**
* 四舍五入
*/
row2.createCell(2).setCellFormula("ROUND(A3,1)");
row2.createCell(3).setCellFormula("ROUND(B3,1)");
FileOutputStream out=new FileOutputStream("D:/基本计算.xls");
workbook.write(out);
out.close();
}catch(Exception e){
e.printStackTrace();
}
}
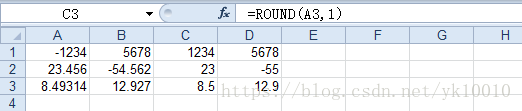