You are to write a program that has to decide whether a given line segment intersects a given rectangle.
An example:
line: start point: (4,9)
end point: (11,2)
rectangle: left-top: (1,5)
right-bottom: (7,1)
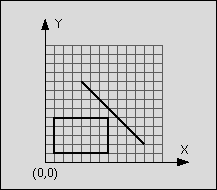
Figure 1: Line segment does not intersect rectangle
The line is said to intersect the rectangle if the line and the rectangle have at least one point in common. The rectangle consists of four straight lines and the area in between. Although all input values are integer numbers, valid intersection points do not have to lay on the integer grid.
Input
The input consists of n test cases. The first line of the input file contains the number n. Each following line contains one test case of the format:
xstart ystart xend yend xleft ytop xright ybottom
where (xstart, ystart) is the start and (xend, yend) the end point of the line and (xleft, ytop) the top left and (xright, ybottom) the bottom right corner of the rectangle. The eight numbers are separated by a blank. The terms top left and bottom right do not imply any ordering of coordinates.
Output
For each test case in the input file, the output file should contain a line consisting either of the letter "T" if the line segment intersects the rectangle or the letter "F" if the line segment does not intersect the rectangle.
Sample Input
1
4 9 11 2 1 5 7 1
Sample Output
F
这道题的大概意思让你输出一个数n,代表有多少个测试样例,然后接下来的n行分别有8个数,前四个代表一个线段的两个端点坐标,后四个代表一个矩形的左上顶点和右下顶点坐标(不是按顺序给出的),如果线段与矩形相交,输出“T”,否则输出“F”。
思路:题目的意思很简单,本来以为是道水题,判断线段是否相交即可,结果坑点多多。需注意的地方:矩形的左上顶点和右下顶点不是按顺序给的,需要自己判断;如果线段在矩形内部,也算相交。所以我们需要先判断矩形的顶点坐标,然后先判断是否有线段的端点在其内部或边上,若有,输出“T”,没有则判断是否线段与矩形的边是否相交即可,在判断是否相交的时候,要先特判是否矩形的某条边是线段的一部分,因为重合也算相交啊(因为漏判wa了两天,刚想通,都是泪啊),其次判断是否相交,用叉乘判即可。
如何判断线段是否相交,例如线段AB和线段CD,线段AB与线段CD相交的充要条件是A点和B点分别在直线CD两边或在其上,且C点和D点分别在直线AB两端或在其上。
代码如下:
#include <algorithm>
#include <iostream>
#include <stdio.h>
#include <string.h>
#include <math.h>
using namespace std;
struct note
{
double x1;
double y1;
double x2;
double y2;
};
struct note a[5];
//求最大值
double big(double a,double b)
{
return a>b?a:b;
}
//求最小值
double small(double a,double b)
{
return a<b?a:b;
}
//判断线段是否有端点在矩形内部或边上(用坐标)
int neibu(note p,note q)
{
if(q.x1<=p.x1&&p.x1<=q.x2&&q.y2<=p.y1&&p.y1<=q.y1)
return 1;
if(q.x1<=p.x2&&p.x2<=q.x2&&q.y2<=p.y2&&p.y2<=q.y1)
return 1;
return 0;
}
//叉乘判断一个点在直线的哪一边或在直线上
int chacheng(double x1,double y1,double x2,double y2)
{
if(x1*y2-x2*y1<0)
return 1;
else if(x1*y2-x2*y1==0)
return 0;
else
return -1;
}
//判断矩形是否和线段相交
int xiangjiao(note p,note q)
{
//特判矩形的某条边是否为线段的一部分
if(p.x1==p.x2&&(p.x1==q.x1||p.x1==q.x2)&&small(p.y1,p.y2)<q.y2&&big(p.y1,p.y2)>q.y1)
return 1;
if(p.y1==p.y2&&(p.y1==q.y1||p.y1==q.y2)&&small(p.x1,p.x2)<q.x1&&big(p.x1,p.x2)>q.x2)
return 1;
//判断线段是否和矩形的边相交
if((chacheng(p.x2-p.x1,p.y2-p.y1,q.x1-p.x1,q.y1-p.y1)*chacheng(p.x2-p.x1,p.y2-p.y1,q.x1-p.x1,q.y2-p.y1)<0)&&(chacheng(0,q.y2-q.y1,p.x1-q.x1,p.y1-q.y1)*chacheng(0,q.y2-q.y1,p.x2-q.x1,p.y2-q.y1)<0))
return 1;
if((chacheng(p.x2-p.x1,p.y2-p.y1,q.x1-p.x1,q.y1-p.y1)*chacheng(p.x2-p.x1,p.y2-p.y1,q.x2-p.x1,q.y1-p.y1)<0)&&(chacheng(q.x2-q.x1,0,p.x1-q.x1,p.y1-q.y1)*chacheng(q.x2-q.x1,0,p.x2-q.x1,p.y2-q.y1)<0))
return 1;
if((chacheng(p.x2-p.x1,p.y2-p.y1,q.x2-p.x1,q.y2-p.y1)*chacheng(p.x2-p.x1,p.y2-p.y1,q.x2-p.x1,q.y1-p.y1)<0)&&(chacheng(0,q.y1-q.y2,p.x1-q.x2,p.y1-q.y2)*chacheng(0,q.y1-q.y2,p.x2-q.x2,p.y2-q.y2)<0))
return 1;
if((chacheng(p.x2-p.x1,p.y2-p.y1,q.x2-p.x1,q.y2-p.y1)*chacheng(p.x2-p.x1,p.y2-p.y1,q.x1-p.x1,q.y2-p.y1)<0)&&(chacheng(q.x1-q.x2,0,p.x1-q.x2,p.y1-q.y2)*chacheng(q.x1-q.x2,0,p.x2-q.x2,p.y2-q.y2)<0))
return 1;
return 0;
}
int main()
{
int n;
scanf("%d",&n);
while(n--)
{
scanf("%lf %lf %lf %lf",&a[0].x1,&a[0].y1,&a[0].x2,&a[0].y2);
scanf("%lf %lf %lf %lf",&a[1].x1,&a[1].y1,&a[1].x2,&a[1].y2);
//判断矩形的左上顶点和右下顶点坐标
if(a[1].x1>a[1].x2)
{
double tx;
tx=a[1].x1;
a[1].x1=a[1].x2;
a[1].x2=tx;
}
if(a[1].y1<a[1].y2)
{
double ty;
ty=a[1].y1;
a[1].y1=a[1].y2;
a[1].y2=ty;
}
//判断线段是否有端点在矩形内部或边上
if(neibu(a[0],a[1]))
{
printf("T\n");
continue;
}
//判断线段是否和矩形相交
if(xiangjiao(a[0],a[1]))
printf("T\n");
else
printf("F\n");
}
return 0;
}