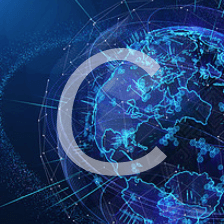
CareerCup
文章平均质量分 65
代码与单车
这个作者很懒,什么都没留下…
展开
-
CareerCup-BASE CASE AND BUILD
这个题刷起来感觉比编程之美什么的easy多了Description: Solve the algorithm first for a base case (e g , just one element) Then, try to solve it for elements one and two, assuming that you have the answer for element原创 2013-04-23 18:55:58 · 505 阅读 · 0 评论 -
CareerCup-2.2
Implement an algorithm to find the nth to last element of a singly linked list #include using namespace std;// Creating a Linked List:struct Node{ Node(int d):data(d){next = NULL;}; i原创 2013-05-08 18:49:23 · 427 阅读 · 0 评论 -
CareerCup-3.1
Describe how you could use a single array to implement three stacksApproach 1:#include using namespace std;#define SIZE 60#define PERSIZE SIZE/3typedef int Data;class Stack{public: S原创 2013-05-10 13:54:13 · 559 阅读 · 0 评论 -
CareerCup-3.2
How would you design a stack which, in addition to push and pop, also has a function min which returns the minimum element? Push, pop and min should all operate in O(1) time微软实习生一面就遇到了这个问题,当时想到的原创 2013-05-10 21:17:05 · 538 阅读 · 0 评论 -
CareerCup-3.3
Imagine a (literal) stack of plates If the stack gets too high, it might topple Therefore, in real life, we would likely start a new stack when the previous stack exceeds some threshold Implement a da原创 2013-05-11 14:00:32 · 676 阅读 · 0 评论 -
CareerCup-3.4
In the classic problem of the Towers of Hanoi, you have 3 rods and N disks of different sizes which can slide onto any tower The puzzle starts with disks sorted in ascending order of size from top原创 2013-05-11 15:27:43 · 591 阅读 · 0 评论 -
CareerCup-4.2
Given a directed graph, design an algorithm to find out whether there is a route between two nodes 开始想用Dijkstra算法,后来发现根本不需要最短只求连通性。回头还得回忆下Dijkstra,写起来有点生疏了。#include #include using namespace std;原创 2013-05-12 10:53:48 · 606 阅读 · 0 评论 -
CareerCup-4.3
Given a sorted (increasing order) array, write an algorithm to create a binary tree withminimal height#include using namespace std;typedef int Data;struct Node{ Node(Data d):data(原创 2013-05-12 11:42:32 · 563 阅读 · 0 评论 -
CareerCup-4.4
Given a binary search tree, design an algorithm which creates a linked list of all the nodes at each depth (i e , if you have a tree with depth D, you’ll have D linked lists) #include using n原创 2013-05-12 13:47:37 · 515 阅读 · 0 评论 -
CareerCup-4.5
Write an algorithm to find the ‘next’ node (i e , in-order successor) of a given node in a binary search tree where each node has a link to its parent #include using namespace std; typedef原创 2013-05-12 14:20:30 · 459 阅读 · 0 评论 -
CareerCup-3.5
Implement a MyQueue class which implements a queue using two stacks开始想到的方法是入队的时候数据进入栈0,出队的时候数据移入栈1再pop。看了答案方法更好。只需要单向挪动。问题在于思维定势,想到队列,总觉得这些数据必须按照顺序在一个栈里。#include using namespace std;typedef int原创 2013-05-11 15:57:03 · 496 阅读 · 0 评论 -
CareerCup-3.6
Write a program to sort a stack in ascending order You should not make any assumptions about how the stack is implemented The following are the only functions that should be used to write this progr原创 2013-05-11 16:54:04 · 537 阅读 · 0 评论 -
CareerCup-4.1
Implement a function to check if a tree is balanced For the purposes of this question, a balanced tree is defined to be a tree such that no two leaf nodes differ in distance from the root by more原创 2013-05-12 09:36:57 · 573 阅读 · 0 评论 -
CareerCup-4.6
Design an algorithm and write code to find the first common ancestor of two nodes in a binary tree Avoid storing additional nodes in a data structure NOTE: This is not necessarily a binary search原创 2013-05-12 14:42:56 · 622 阅读 · 0 评论 -
CareerCup-4.7
You have two very large binary trees: T1, with millions of nodes, and T2, with hundreds of nodes Create an algorithm to decide if T2 is a subtree of T1 #include using namespace std; typedef int原创 2013-05-13 10:49:16 · 601 阅读 · 0 评论 -
CareerCup-5.1
You are given two 32-bit numbers, N and M, and two bit positions, i and j Write a method to set all bits between i and j in N equal to M (e g , M becomes a substring of N located at i and starting原创 2013-05-15 16:28:10 · 625 阅读 · 0 评论 -
CareerCup-2.4
You have two numbers represented by a linked list, where each node contains a single digit The digits are stored in reverse order, such that the 1’s digit is at the head of the list Write a function t原创 2013-05-08 18:52:46 · 490 阅读 · 0 评论 -
CareerCup-2.3
Implement an algorithm to delete a node in the middle of a single linked list, given only access to that node EXAMPLEInput: the node ‘c’ from the linked list a->b->c->d->eResult: nothing is retu原创 2013-05-08 18:50:30 · 432 阅读 · 0 评论 -
CareerCup-PATTERN MATCHING
CareerCup是一本相当好Code面试的书,开始研读Description: Consider what problems the algorithm is similar to, and figure out if you can modify the solution to develop an algorithm for this problem Example: A s原创 2013-04-23 17:35:35 · 624 阅读 · 0 评论 -
CareerCup-1.1
Question:Implement an algorithm to determine if a string has all unique characters What if you can not use additional data structures?#include using namespace std;bool isUnique(char* s){ b原创 2013-04-24 10:33:28 · 545 阅读 · 0 评论 -
CareerCup-1.3
Design an algorithm and write code to remove the duplicate characters in a string without using any additional buffer NOTE: One or two additional variables are fine An extra copy of the array is not.原创 2013-04-25 17:38:18 · 559 阅读 · 0 评论 -
CareerCup-1.2
Write code to reverse a C-Style String (C-String means that “abcd” is represented as five characters, including the null character )#include using namespace std;void swap2(char* a, char* b){原创 2013-04-25 16:38:33 · 507 阅读 · 0 评论 -
CareerCup-1.4
Write a method to decide if two strings are anagrams or not Solution1#先排序再比较字符串是否相同Solution2#统计字符数比较数量是否相等#include using namespace std;bool anagram(char* s, char* t) { if(strlen(s)原创 2013-04-25 20:15:13 · 527 阅读 · 0 评论 -
CareerCup-1.5
Write a method to replace all spaces in a string with ‘%20’简单题仍然无法做到无误。一定要摆脱对IDE和编译器的依赖!#include using namespace std;char* replaceSpace(char* str) { int count=0; char *p; for(p=str;原创 2013-04-25 21:28:51 · 585 阅读 · 0 评论 -
CareerCup-1.7
Write an algorithm such that if an element in an MxN matrix is 0, its entire row and column is set to 0纸上代码终于准确无误,虽然是个水题#include using namespace std;#define M 3#define N 5void setZero(int da原创 2013-04-26 09:57:23 · 615 阅读 · 0 评论 -
CareerCup-1.6
Given an image represented by an NxN matrix, where each pixel in the image is 4 bytes, write a method to rotate the image by 90 degrees Can you do this in place?唉!还是会有错误,这次控制到只有一处错误。#include usin原创 2013-04-25 22:39:04 · 611 阅读 · 0 评论 -
CareerCup-1.8
Assume you have a method isSubstring which checks if one word is a substring of another Given two strings, s1 and s2, write code to check if s2 is a rotation of s1 using only one call to isSubstring (原创 2013-05-02 20:21:57 · 502 阅读 · 0 评论 -
CareerCup-5.3
Given an integer, print the next smallest and next largest number that have the same number of 1 bits in their binary representation 理解题意费了些时间。明白了之后就没什么难度了。答案没考虑负数的情况和无法找到这样的数字的情况,同时有一定的冗余。#原创 2013-05-16 21:12:07 · 586 阅读 · 0 评论 -
CareerCup-5.5
Write a function to determine the number of bits required to convert integer A to integer B Input: 31, 14Output: 2#include using namespace std;int convertCount(int a, int b){ int t = a原创 2013-05-16 21:40:19 · 522 阅读 · 0 评论 -
CareerCup-5.6
Write a program to swap odd and even bits in an integer with as few instructions as possible (e g , bit 0 and bit 1 are swapped, bit 2 and bit 3 are swapped, etc) 嘿嘿,这个题小Case#include using name原创 2013-05-16 22:46:12 · 517 阅读 · 0 评论 -
CareerCup-6.1
Add arithmetic operators (plus, minus, times, divide) to make the following expression true: 3 1 3 6 = 8 You can use any parentheses you’d like 书上说谷歌微软已经ban掉了智力题,没事做做还是有好处的。但是第一道题就感觉智商捉鸡了。答案是((原创 2013-05-17 21:21:34 · 664 阅读 · 0 评论 -
CareerCup-5.7
An array A[1 n] contains all the integers from 0 to n except for one number which is missing In this problem, we cannot access an entire integer in A with a single operation The elements of A are re原创 2013-05-17 14:49:19 · 775 阅读 · 0 评论 -
CareerCup-2.5
Given a circular linked list, implement an algorithm which returns node at the beginning of the loop DEFINITIONCircular linked list: A (corrupt) linked list in which a node’s next pointer points t原创 2013-05-08 18:57:29 · 594 阅读 · 0 评论 -
CareerCup-2.1
Write code to remove duplicates from an unsorted linked list FOLLOW UP How would you solve this problem if a temporary buffer is not allowed?#include using namespace std;// Creating a Linked Li原创 2013-05-08 18:48:27 · 514 阅读 · 0 评论 -
CareerCup-5.2
Given a (decimal - e g 3.72) number that is passed in as a string, print the binary representation. If the number can not be represented accurately in binary, print “ERROR”答案没有考虑负数的情况。但是有没有负数从题目无法推断原创 2013-05-15 19:19:59 · 636 阅读 · 0 评论