1 功能介绍
- 默认展示主地图,点击某个省市区根据地区编码展示对应的省市区地图,当匹配不到对应的省市区编码则展示主地图。
- 根据地理位置坐标加标记。
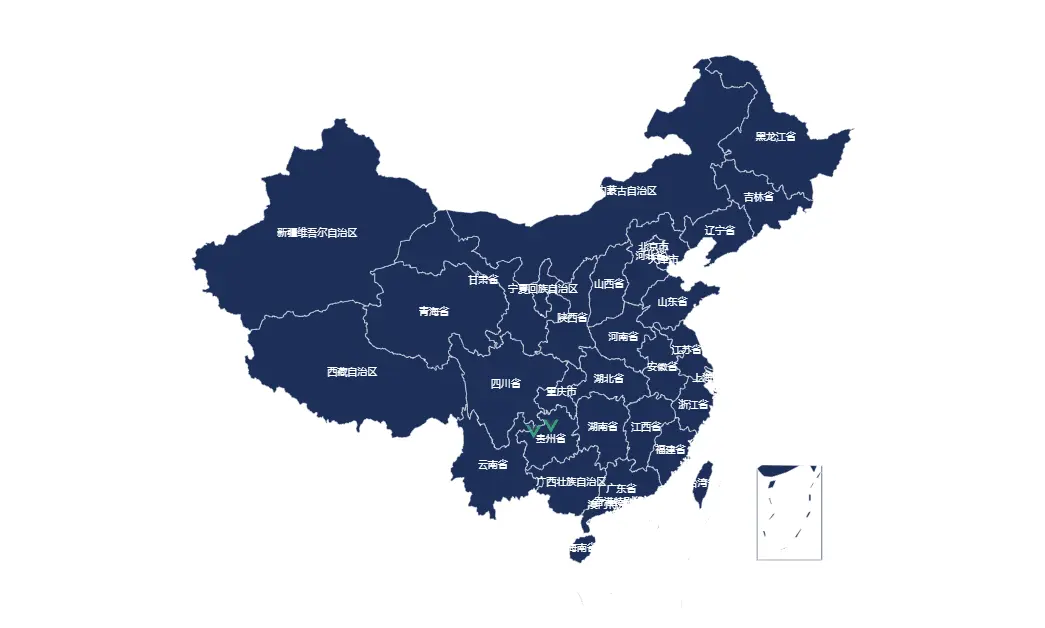
1.1点击省市区展示省市区地图
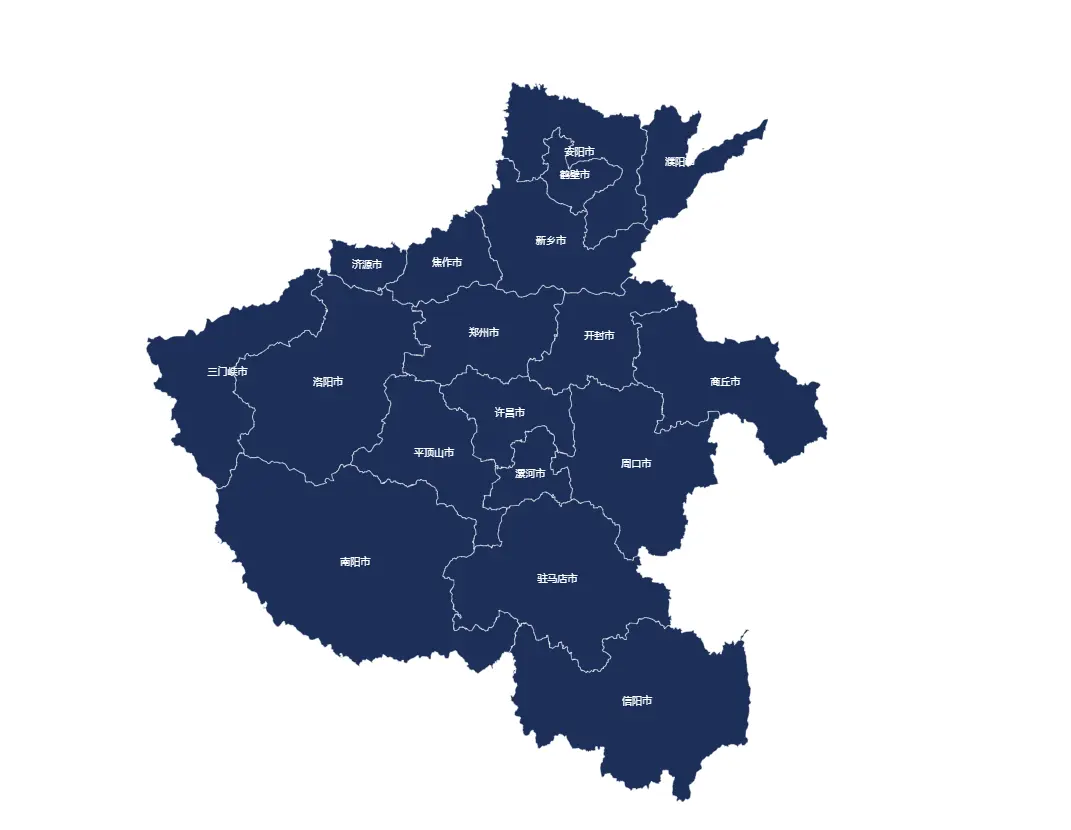
如果觉得地名位置歪/想让省市区位置在区域中心位置,则需下载地图JSON地图文件进行手动修改(一般名字的位置为省会坐标点),具体修改会在 --- 2.3创建echarts地图 --- 区域写到,因为现在写好像并不清楚到底在写什么。
2 功能实现
2.1 下载所需NPM
包
npm i axios
npm i echarts
2.2 引入所需配置文件与初始化
2.2.1 编码JSON数据项(由于数据量过大,有需要麻烦移步下面文章获取)
省市区编码JSON数据
2.2.2 初始化
<template>
<div>
<div id="echatsMap" style="width: 100%; height: 800px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
import axios from "axios";
import { cityCode } from "@/components/cityCode.js";
export default {
name: "echatsMap",
components: {},
data() {
return {
cityCode: cityCode,
myChart: "",
distributionOptions: "",
};
},
mounted() {},
methods: {},
};
</script>
2.3 创建echarts地图
- 此处会用到外部链接来获取地图JSON数据(100000是主视图的地区编码):http://datav.aliyun.com/portal/school/atlas/area_selector#&lat=32.287132632616384&lng=101.1181640625&zoom=4
- 传递不同的编码,会返回不同省市区的JSON数据。
2.3.1 上面有提到省市区名字可能不在中心位置,可以按以下操作:
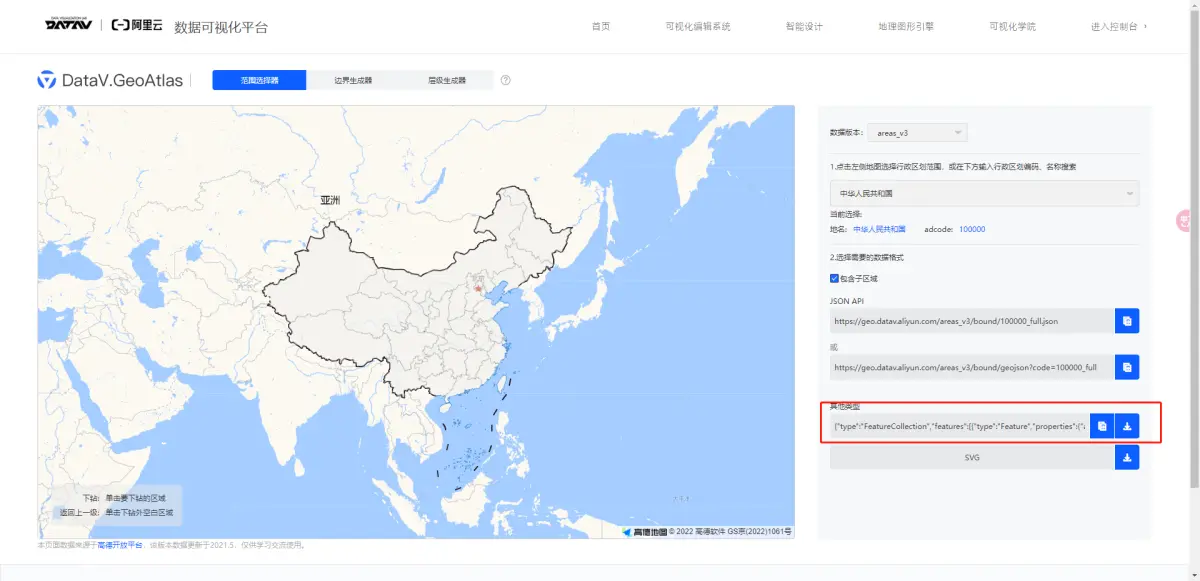
- 点击红框里的下载,就会得到一份本地的JSON文件,
2.3.2的代码块为动态获取方式
,将本地的JSON地图文件进行修改即可,示例:
"properties": {
"adcode": 620000,
"name": "甘肃省",
"center": [
103.823557,
36.058039
],
"childrenNum": 14,
"level": "province",
"parent": {
"adcode": 100000
},
"subFeatureIndex": 27,
"acroutes": [
100000
]
},
"cp": [
103.823557,
36.058039
],
"properties": {
"adcode": 620000,
"name": "甘肃省",
"center": [
103.823557,
36.058039
],
"cp": [
103.823557,
36.058039
],
"childrenNum": 14,
"level": "province",
"parent": {
"adcode": 100000
},
"subFeatureIndex": 27,
"acroutes": [
100000
]
},
修改后就不能用动态获取了,需要存放到本地,让其使用本地的文件示例如下(具体改哪来,):
init() {
let path = require('../../../../../public/mapJson/china.json');
echarts.registerMap("china", path );
this.changeOptions("china");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions)
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
2.3.2 代码实现
<template>
<div>
<div id="echatsMap" style="width: 100%; height: 800px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
import axios from "axios";
import { cityCode } from "@/components/cityCode.js";
export default {
name: "echatsMap",
components: {},
data() {
return {
cityCode: cityCode,
myChart: "",
distributionOptions: "",
};
},
mounted() {
this.$nextTick(() => {
this.init();
});
},
methods: {
init() {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/100000_full.json`;
axios.get(path).then((res) => {
echarts.registerMap("china", res.data);
this.changeOptions("china");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions)
});
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
changeOptions(name) {
const seriesList = [
{
data: [{ value: [106.9, 27.7] }, { value: [105.29, 27.32] }],
},
];
const series = seriesList.map((v) => {
return {
type: "scatter",
coordinateSystem: "geo",
data: v.data,
symbol: function (params, key) {
return "image://" + require("@/assets/logo.png");
},
symbolSize: 16,
};
});
this.distributionOptions = {
tooltip: {
show: true,
trigger: "item",
triggerOn: "mousemove",
formatter: "名称:{b}<br/>坐标:{c}",
},
series,
geo: {
map: name || "china",
layoutCenter: ["50%", "50%"],
layoutSize: "45%",
roam: true,
zoom: 2,
label: {
normal: {
show: true,
textStyle: {
color: "#fff",
fontSize: 10,
fontFamily: "Arial",
},
},
emphasis: {
color: "#fff",
},
},
itemStyle: {
normal: {
borderColor: "#fff",
areaColor: "#1c2f59",
textStyle: {
color: "#fff",
},
},
emphasis: {
areaColor: "#1c2f59",
color: "#fff",
},
},
regions: [
],
},
};
},
},
};
</script>
2.4 点击地图省市区展示省市区地图
- 大概就是点击省市区时根据名称与编码文件去匹配,把匹配到的编码通过外部接口去获取想要的省市区JSON地图数据。再次渲染echarts即可。
注意:如果没匹配到需跳主地图/获取不到此区域的JSON地图数据。
<template>
<div>
<div id="echatsMap" style="width: 100%; height: 800px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
import axios from "axios";
import { cityCode } from "@/components/cityCode.js";
export default {
name: "echatsMap",
components: {},
data() {
return {
cityCode: cityCode,
myChart: "",
distributionOptions: "",
};
},
mounted() {
this.$nextTick(() => {
this.init();
});
},
methods: {
init() {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/100000_full.json`;
axios.get(path).then((res) => {
echarts.registerMap("china", res.data);
this.changeOptions("china");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions);
this.myChart.on("click", (chinaParam) => {
if (!this.cityCode) {
this.cityCode = cityCode;
this.getProvinceMapOpt(100000, "")
return;
}
let code = this.cityCode.find(
(x) => chinaParam.name.indexOf(x ? x.name : "") !== -1
);
if (code) {
this.cityCode = code.city;
this.getProvinceMapOpt(Number(code.adcode), chinaParam.name);
} else {
this.cityCode = cityCode;
this.getProvinceMapOpt(100000, "");
}
});
});
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
getProvinceMapOpt(provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
if (provinceAlphabet === 100000) {
path = "/mapJson/china.json";
}
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
});
},
changeOptions(name) {
const seriesList = [
{
data: [{ value: [106.9, 27.7] }, { value: [105.29, 27.32] }],
},
];
const series = seriesList.map((v) => {
return {
type: "scatter",
coordinateSystem: "geo",
data: v.data,
symbol: function (params, key) {
return "image://" + require("@/assets/logo.png");
},
symbolSize: 16,
};
});
this.distributionOptions = {
tooltip: {
show: true,
trigger: "item",
triggerOn: "mousemove",
formatter: "名称:{b}<br/>坐标:{c}",
},
series,
geo: {
map: name || "china",
layoutCenter: ["50%", "50%"],
layoutSize: "45%",
roam: true,
zoom: 2,
label: {
normal: {
show: true,
textStyle: {
color: "#fff",
fontSize: 10,
fontFamily: "Arial",
},
},
emphasis: {
color: "#fff",
},
},
itemStyle: {
normal: {
borderColor: "#fff",
areaColor: "#1c2f59",
textStyle: {
color: "#fff",
},
},
emphasis: {
areaColor: "#1c2f59",
color: "#fff",
},
},
regions: [
],
},
};
},
},
};
</script>
3 china版地图完整代码
<template>
<div>
<div id="echatsMap" style="width: 100%; height: 800px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
import axios from "axios";
import { cityCode } from "@/components/cityCode.js";
export default {
name: "echatsMap",
components: {},
data() {
return {
cityCode: cityCode,
myChart: "",
distributionOptions: "",
};
},
mounted() {
this.$nextTick(() => {
this.init();
});
},
methods: {
init() {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/100000_full.json`;
axios.get(path).then((res) => {
echarts.registerMap("china", res.data);
this.changeOptions("china");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions);
this.myChart.on("click", (chinaParam) => {
if (!this.cityCode) {
this.cityCode = cityCode;
this.getProvinceMapOpt(100000, "")
return;
}
let code = this.cityCode.find(
(x) => chinaParam.name.indexOf(x ? x.name : "") !== -1
);
if (code) {
this.cityCode = code.city;
this.getProvinceMapOpt(Number(code.adcode), chinaParam.name);
} else {
this.cityCode = cityCode;
this.getProvinceMapOpt(100000, "");
}
});
});
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
getProvinceMapOpt(provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
if (provinceAlphabet === 100000) {
path = "/mapJson/china.json";
}
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
});
},
changeOptions(name) {
const seriesList = [
{
data: [{ value: [106.9, 27.7] }, { value: [105.29, 27.32] }],
},
];
const series = seriesList.map((v) => {
return {
type: "scatter",
coordinateSystem: "geo",
data: v.data,
symbol: function (params, key) {
return "image://" + require("@/assets/logo.png");
},
symbolSize: 16,
};
});
this.distributionOptions = {
tooltip: {
show: true,
trigger: "item",
triggerOn: "mousemove",
formatter: "名称:{b}<br/>坐标:{c}",
},
series,
geo: {
map: name || "china",
layoutCenter: ["50%", "50%"],
layoutSize: "45%",
roam: true,
zoom: 2,
label: {
normal: {
show: true,
textStyle: {
color: "#fff",
fontSize: 10,
fontFamily: "Arial",
},
},
emphasis: {
color: "#fff",
},
},
itemStyle: {
normal: {
borderColor: "#fff",
areaColor: "#1c2f59",
textStyle: {
color: "#fff",
},
},
emphasis: {
areaColor: "#1c2f59",
color: "#fff",
},
},
regions: [
],
},
};
},
},
};
</script>
4 展示某个省的地图
4.1 展示某个省的地图的话,要做一些修改
- 修改默认请求的Json数据
- 修改默认展示的地图名字等
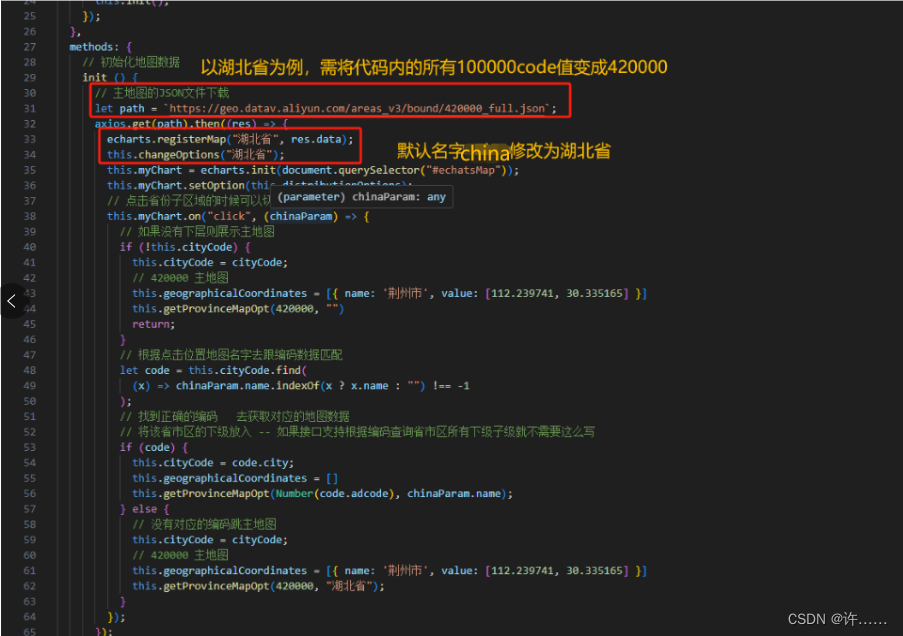
4.2 效果图
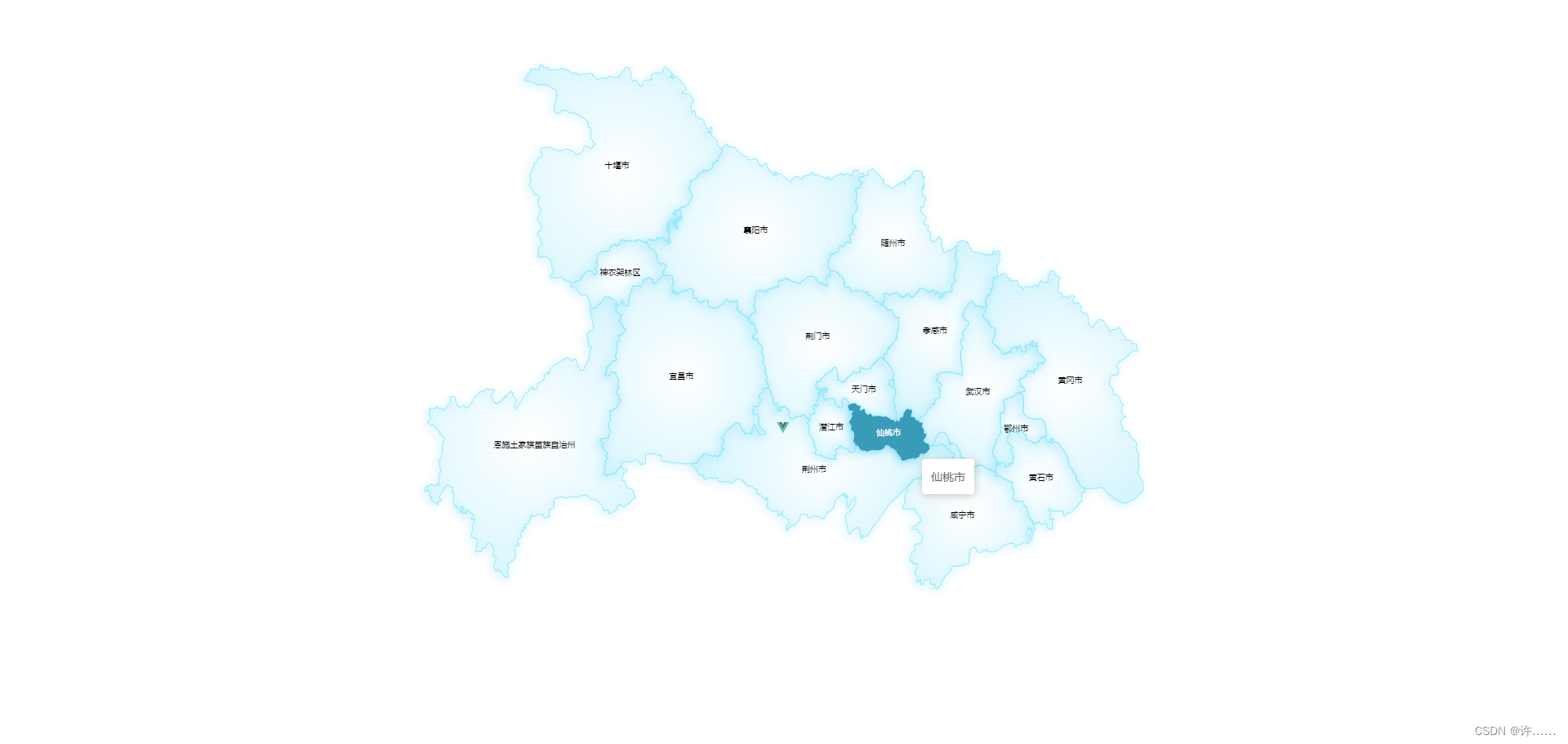
4.3 完整代码
4.3.1 地区编码文件
export const cityCode = [{
"adcode": "420100",
"name": "武汉"
}, {
"adcode": "420200",
"name": "黄石"
}, {
"adcode": "420300",
"name": "十堰"
}, {
"adcode": "420500",
"name": "宜昌"
}, {
"adcode": "420600",
"name": "襄阳"
}, {
"adcode": "420700",
"name": "鄂州"
}, {
"adcode": "420800",
"name": "荆门"
}, {
"adcode": "420900",
"name": "孝感"
}, {
"adcode": "421000",
"name": "荆州"
}, {
"adcode": "421100",
"name": "黄冈"
}, {
"adcode": "421200",
"name": "咸宁"
}, {
"adcode": "421300",
"name": "随州"
}, {
"adcode": "422800",
"name": "恩施土家族苗族自治州"
}, {
"adcode": "429004",
"name": "仙桃"
}, {
"adcode": "429005",
"name": "潜江"
}, {
"adcode": "429006",
"name": "天门"
}, {
"adcode": "429021",
"name": "神农架林区"
}
]
4.3.2 视图文件
<template>
<div>
<div id="echatsMap" style="width: 100%; height: 800px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
import axios from "axios";
import { cityCode } from "@/utils/cityCode.js";
export default {
name: "echatsMap",
components: {},
data () {
return {
cityCode: cityCode,
myChart: "",
distributionOptions: "",
geographicalCoordinates: [{ name: '荆州市', value: [112.239741, 30.335165] }]
};
},
mounted () {
this.$nextTick(() => {
this.init();
});
},
methods: {
init () {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/420000_full.json`;
axios.get(path).then((res) => {
echarts.registerMap("湖北省", res.data);
this.changeOptions("湖北省");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions);
this.myChart.on("click", (chinaParam) => {
if (!this.cityCode) {
this.cityCode = cityCode;
this.geographicalCoordinates = [{ name: '荆州市', value: [112.239741, 30.335165] }]
this.getProvinceMapOpt(420000, "")
return;
}
let code = this.cityCode.find(
(x) => chinaParam.name.indexOf(x ? x.name : "") !== -1
);
if (code) {
this.cityCode = code.city;
this.geographicalCoordinates = []
this.getProvinceMapOpt(Number(code.adcode), chinaParam.name);
} else {
this.cityCode = cityCode;
this.geographicalCoordinates = [{ name: '荆州市', value: [112.239741, 30.335165] }]
this.getProvinceMapOpt(420000, "湖北省");
}
});
});
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
getProvinceMapOpt (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
});
},
changeOptions (name) {
const seriesList = [
{
data: this.geographicalCoordinates,
},
];
const series = seriesList.map((v) => {
return {
type: "scatter",
coordinateSystem: "geo",
data: v.data,
symbol: function (params, key) {
return "image://" + require("@/assets/logo.png");
},
symbolSize: 16,
tooltip: {
trigger: 'item',
formatter: function (params) {
return `名称:${params.data.name}<br/>坐标:<br/>经度:${params.data.value[0]}<br/>纬度:${params.data.value[1]}`;
},
},
};
});
this.distributionOptions = {
tooltip: {},
series,
geo: {
map: name || "湖北省",
layoutCenter: ["50%", "50%"],
layoutSize: "55%",
roam: true,
zoom: 2,
label: {
normal: {
show: true,
textStyle: {
color: "#000",
fontSize: 10,
fontFamily: "Arial",
},
},
emphasis: {
color: "#fff",
},
},
itemStyle: {
normal: {
borderColor: 'rgba(147, 235, 248, 1)',
borderWidth: 1,
areaColor: {
type: 'radial',
x: 0.5,
y: 0.5,
r: 0.8,
colorStops: [{
offset: 0,
color: 'rgba(147, 235, 248, 0)'
}, {
offset: 1,
color: 'rgba(147, 235, 248, .2)'
}],
globalCoord: false
},
shadowColor: 'rgba(128, 217, 248, 1)',
shadowOffsetX: -2,
shadowOffsetY: 2,
shadowBlur: 10
},
emphasis: {
areaColor: '#389BB7',
borderWidth: 0
}
},
regions: [
],
},
};
},
},
};
</script>
4 展示到县级别
- 县级别以上都是用的
https://geo.datav.aliyun.com/areas_v3/bound/${区域编码}_full.json
去获取的JSON地图数据,但到了县这个请求地址就变了,变成了https://geo.datav.aliyun.com/areas_v3/bound/${区域编码}.json
- 以下将以 4 展示某个省的地图完整代码进行修改
4.1 添加县级以上请求地图数据失败捕获
4.1.1 添加错误捕获
getProvinceMapOpt (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
console.error('请求出错:', error);
});
},
4.1.2 添加请求县级的JSON地图数据方法
getProvinceMapOptCounty (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
console.error('请求出错:', error);
});
},
- 此时在县级别以上获取数据失败时去请求,并记录此时请求到了县级别(因为没有县级以下的数据了,再点击县的话就返回第一级)
- 需要再点击县的话就返回第一级代码
data () {
return {
geoCounty: false,
}
}
init () {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/420000_full.json`;
axios.get(path).then((res) => {
echarts.registerMap("湖北省", res.data);
this.changeOptions("湖北省");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions);
this.myChart.on("click", (chinaParam) => {
if (!this.cityCode) {
this.cityCode = cityCode;
this.geoCounty = false
this.getProvinceMapOpt(420000, "")
return;
}
let code = this.cityCode.find(
(x) => chinaParam.name.indexOf(x ? x.name : "") !== -1
);
if (code) {
if (!this.geoCounty) {
this.getProvinceMapOpt(Number(code.adcode), chinaParam.name);
} else {
this.cityCode = cityCode;
this.geoCounty = false
this.getProvinceMapOpt(420000, "湖北省");
}
} else {
this.cityCode = cityCode;
this.geoCounty = false
this.getProvinceMapOpt(420000, "湖北省");
}
});
});
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
getProvinceMapOpt (provinceAlphabet, name) {
console.log(provinceAlphabet);
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
this.geoCounty = true
this.getProvinceMapOptCounty(provinceAlphabet, name)
});
},
getProvinceMapOptCounty (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
console.error('请求出错:', error);
});
},
getProvinceMapOpt (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
this.geoCounty = true
this.getProvinceMapOptCounty(provinceAlphabet, name)
});
},
getProvinceMapOptCounty (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
console.error('请求出错:', error);
});
},
4.2 修改地区编码(cityCode.js)文件
export const cityCode = [{
"adcode": "420100",
"name": "武汉"
}, {
"adcode": "420200",
"name": "黄石市"
}, {
"adcode": "420300",
"name": "十堰"
}, {
"adcode": "420500",
"name": "宜昌市"
}, {
"adcode": "420600",
"name": "襄阳"
}, {
"adcode": "420700",
"name": "鄂州"
}, {
"adcode": "420800",
"name": "荆门"
}, {
"adcode": "420900",
"name": "孝感"
}, {
"adcode": "421000",
"name": "荆州市"
}, {
"adcode": "421100",
"name": "黄冈"
}, {
"adcode": "421200",
"name": "咸宁"
}, {
"adcode": "421300",
"name": "随州"
}, {
"adcode": "422800",
"name": "恩施土家族苗族自治州"
}, {
"adcode": "429004",
"name": "仙桃"
}, {
"adcode": "429005",
"name": "潜江"
}, {
"adcode": "429006",
"name": "天门"
}, {
"adcode": "429021",
"name": "神农架林区"
}, {
"adcode": "420322",
"name": "郧西县"
}, {
"adcode": "420304",
"name": "郧阳区"
}, {
"adcode": "420381",
"name": "丹江口市"
}, {
"adcode": "420303",
"name": "张湾区"
}, {
"adcode": "420302",
"name": "茅箭区"
}, {
"adcode": "420323",
"name": "竹山县"
}, {
"adcode": "420325",
"name": "房县"
}, {
"adcode": "420324",
"name": "竹溪县"
}, {
"adcode": "420682",
"name": "老河口市"
}, {
"adcode": "420607",
"name": "襄州区"
}, {
"adcode": "420683",
"name": "枣阳市"
}, {
"adcode": "420625",
"name": "谷城县"
}, {
"adcode": "420606",
"name": "樊城区"
}, {
"adcode": "420602",
"name": "襄城区"
}, {
"adcode": "420684",
"name": "宜城市"
}, {
"adcode": "420626",
"name": "保康县"
}, {
"adcode": "420624",
"name": "南漳县"
}, {
"adcode": "421321",
"name": "随县"
}, {
"adcode": "421303",
"name": "曾都区"
}, {
"adcode": "421381",
"name": "广水市"
}, {
"adcode": "420922",
"name": "大悟县"
}, {
"adcode": "420982",
"name": "安陆市"
}, {
"adcode": "420921",
"name": "孝昌县"
}, {
"adcode": "420981",
"name": "应城市"
}, {
"adcode": "420923",
"name": "云梦县"
}, {
"adcode": "420902",
"name": "孝南区"
}, {
"adcode": "420984",
"name": "汉川市"
}, {
"adcode": "421122",
"name": "红安县"
}, {
"adcode": "421181",
"name": "麻城市"
}, {
"adcode": "421123",
"name": "罗田县"
}, {
"adcode": "421124",
"name": "英山县"
}, {
"adcode": "421121",
"name": "团风县"
}, {
"adcode": "421125",
"name": "浠水县"
}, {
"adcode": "421126",
"name": "蕲春县"
}, {
"adcode": "421182",
"name": "武穴市"
}, {
"adcode": "421127",
"name": "黄梅县"
}, {
"adcode": "421102",
"name": "黄州区"
}, {
"adcode": "420281",
"name": "大冶市"
}, {
"adcode": "420205",
"name": "铁山区"
}, {
"adcode": "420204",
"name": "下陆区"
}, {
"adcode": "420202",
"name": "黄石港区"
}, {
"adcode": "420203",
"name": "西塞山区"
}, {
"adcode": "420222",
"name": "阳新县"
}, {
"adcode": "420703",
"name": "华容区"
}, {
"adcode": "420704",
"name": "鄂城区"
}, {
"adcode": "420702",
"name": "梁子湖区"
}, {
"adcode": "420116",
"name": "黄陂区"
}, {
"adcode": "420117",
"name": "新洲区"
}, {
"adcode": "420112",
"name": "东西湖区"
}, {
"adcode": "420102",
"name": "江岸区"
}, {
"adcode": "420103",
"name": "江汉区"
}, {
"adcode": "420104",
"name": "硚口区"
}, {
"adcode": "420107",
"name": "青山区"
}, {
"adcode": "420106",
"name": "武昌区"
}, {
"adcode": "420111",
"name": "洪山区"
}, {
"adcode": "420105",
"name": "汉阳区"
}, {
"adcode": "420114",
"name": "蔡甸区"
}, {
"adcode": "420113",
"name": "汉南区"
}, {
"adcode": "420115",
"name": "江夏区"
}, {
"adcode": "420802",
"name": "东宝区"
}, {
"adcode": "420881",
"name": "钟祥市"
}, {
"adcode": "420882",
"name": "京山市"
}, {
"adcode": "420804",
"name": "掇刀区"
}, {
"adcode": "420822",
"name": "沙洋县"
}, {
"adcode": "422802",
"name": "利川市"
}, {
"adcode": "422801",
"name": "恩施市"
}, {
"adcode": "422822",
"name": "建始县"
}, {
"adcode": "422823",
"name": "巴东县"
}, {
"adcode": "422828",
"name": "鹤峰县"
}, {
"adcode": "422825",
"name": "宣恩县"
}, {
"adcode": "422826",
"name": "咸丰县"
}, {
"adcode": "422827",
"name": "来凤县"
}, {
"adcode": "420526",
"name": "兴山县"
}, {
"adcode": "420506",
"name": "夷陵区"
}, {
"adcode": "420525",
"name": "远安县"
}, {
"adcode": "420582",
"name": "当阳市"
}, {
"adcode": "420583",
"name": "枝江市"
}, {
"adcode": "420505",
"name": "猇亭区"
}, {
"adcode": "420503",
"name": "伍家岗区"
}, {
"adcode": "420502",
"name": "西陵区"
}, {
"adcode": "420504",
"name": "点军区"
}, {
"adcode": "420527",
"name": "秭归县"
}, {
"adcode": "420528",
"name": "长阳土家族自治县"
}, {
"adcode": "420529",
"name": "五峰土家族自治县"
}, {
"adcode": "420581",
"name": "宜都市"
}, {
"adcode": "421087",
"name": "松滋市"
}, {
"adcode": "421003",
"name": "荆州区"
}, {
"adcode": "421022",
"name": "公安县"
}, {
"adcode": "421002",
"name": "沙市区"
}, {
"adcode": "421024",
"name": "江陵县"
}, {
"adcode": "421081",
"name": "石首市"
}, {
"adcode": "421023",
"name": "监利市"
}, {
"adcode": "421023",
"name": "监利市"
}, {
"adcode": "421083",
"name": "洪湖市"
},{
"adcode": "421221",
"name": "嘉鱼县"
},{
"adcode": "421202",
"name": "咸安区"
},{
"adcode": "421224",
"name": "通山县"
},{
"adcode": "421281",
"name": "赤壁市"
},{
"adcode": "421223",
"name": "崇阳县"
},{
"adcode": "421222",
"name": "通城县"
},
]
4.3 完整代码(默认带再点击县的话就返回第一级)
<template>
<div>
<div id="echatsMap" style="width: 100%; height: 800px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
import axios from "axios";
import { cityCode } from "@/utils/cityCode.js";
export default {
name: "echatsMap",
components: {},
data () {
return {
cityCode: cityCode,
myChart: "",
distributionOptions: "",
geographicalCoordinates: [
{ name: '荆州市', adcode: '421000', value: [112.239741, 30.335165], chiidren: [{ name: '公安县', adcode: '420325', value: [112.230407, 30.058336] }] },
],
geoCounty: false,
};
},
mounted () {
this.$nextTick(() => {
this.init();
});
},
methods: {
init () {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/420000_full.json`;
axios.get(path).then((res) => {
echarts.registerMap("湖北省", res.data);
this.changeOptions("湖北省");
this.myChart = echarts.init(document.querySelector("#echatsMap"));
this.myChart.setOption(this.distributionOptions);
this.myChart.on("click", (chinaParam) => {
if (!this.cityCode) {
this.cityCode = cityCode;
this.geoCounty = false
this.getProvinceMapOpt(420000, "")
return;
}
let code = this.cityCode.find(
(x) => chinaParam.name.indexOf(x ? x.name : "") !== -1
);
if (code) {
if (!this.geoCounty) {
this.getProvinceMapOpt(Number(code.adcode), chinaParam.name);
} else {
this.cityCode = cityCode;
this.geoCounty = false
this.getProvinceMapOpt(420000, "湖北省");
}
} else {
this.cityCode = cityCode;
this.geoCounty = false
this.getProvinceMapOpt(420000, "湖北省");
}
});
});
window.onresize = function () {
if (this.myChart) this.myChart.resize();
};
},
getProvinceMapOpt (provinceAlphabet, name) {
console.log(provinceAlphabet);
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}_full.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
this.geoCounty = true
this.getProvinceMapOptCounty(provinceAlphabet, name)
});
},
getProvinceMapOptCounty (provinceAlphabet, name) {
let path = `https://geo.datav.aliyun.com/areas_v3/bound/${provinceAlphabet}.json`;
axios.get(path).then((res) => {
echarts.registerMap(name, res.data);
this.changeOptions(name);
this.myChart.setOption(this.distributionOptions, true);
}).catch((error) => {
console.error('请求出错:', error);
});
},
changeOptions (name) {
const geographicalLocation = this.geographicalCoordinates
const seriesList = [
{
data: geographicalLocation,
},
];
const series = seriesList.map((v) => {
return {
type: "scatter",
coordinateSystem: "geo",
data: v.data,
symbol: function (params, key) {
return "image://" + require("@/assets/logo.png");
},
symbolSize: 16,
tooltip: {
trigger: 'item',
formatter: function (params) {
return `名称:${params.data.name}<br/>坐标:<br/>经度:${params.data.value[0]}<br/>纬度:${params.data.value[1]}`;
},
},
clickable: true,
events: {
click: function (params) {
console.log('点击了图标:', params.data.name);
}
}
};
});
this.distributionOptions = {
tooltip: {},
series,
geo: {
map: name || "湖北省",
layoutCenter: ["50%", "50%"],
layoutSize: "55%",
roam: true,
zoom: 2,
label: {
normal: {
show: true,
textStyle: {
color: "#000",
fontSize: 10,
fontFamily: "Arial",
},
},
emphasis: {
color: "#fff",
},
},
itemStyle: {
normal: {
borderColor: 'rgba(147, 235, 248, 1)',
borderWidth: 1,
areaColor: {
type: 'radial',
x: 0.5,
y: 0.5,
r: 0.8,
colorStops: [{
offset: 0,
color: 'rgba(147, 235, 248, 0)'
}, {
offset: 1,
color: 'rgba(147, 235, 248, .2)'
}],
globalCoord: false
},
shadowColor: 'rgba(128, 217, 248, 1)',
shadowOffsetX: -2,
shadowOffsetY: 2,
shadowBlur: 10
},
emphasis: {
areaColor: '#389BB7',
borderWidth: 0
}
},
regions: [
],
},
};
},
},
};
</script>