## Spring 简介
1、spring是什么?
Spring是分层的Java SE/EE应用full-stack轻量级开源框架,以loC(Inverse Of Control:反转控制)和AOP(Aspect Oriented Programming:面向切面编程)为内核,提供展现层SpringMVC和持久层Spring JDBCTemplete 以及业务层事务管理等众多的企业及应用技术,还能整合开源世界众多著名的第三方框架和类库
2、Spring发展历程
3、Spring的优势
1、方便解耦,简化开发
通过Spring提供的IoC容器,可以将对象间的依赖关系交有Spring进行控制,避免硬编码造成的过度耦合。
用户也不必在为单例模式类、属性文件解析等这些很底层的需求编写代码,可以更专注于上层的应用
2、AOP编程的支持
通过spring的AOP功能,方便进行面向切面编程,许多不容易用传统OOP实现的功能可以通过AOP轻松实现
3、声明式事务的支持
可以将我们从单调烦闷的事务管理代码中解脱出来,通过声明式方式灵活的进行事务管理,提高开发效率的质量
4、方便程序的测试
可以用非容器依赖的编程方式进行几乎所有的测试工作,测试不在是昂贵的操作,而是随手可做的事情
5、方便集成各种优秀框架
Spring对各种优秀框架(Struts、Hibemate、Hessian、Quartz等)的支持
6、降低Java EE API的使用难度
Spring对Java EE API(如JDBC、JavaMail、远程调用等)进行了薄薄的封装测,使这些API的使用难度大为降低
7、Java源码是经典学习范例
Spring的源代码设计精妙、结构清晰、匠心独运,处处体现着大是对Java设计模式灵活运用以及对Java技术的高深造诣。他的源代码
无疑是Java技术的最佳实践的范例
4、Spring体系结构
##Spring 快速入门
1、Spring程序开发步骤
1、导入Spring开发的基本包坐标
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.5.RELEASE</version>
</dependency>
</dependencies>
2、编写Dao接口和实现类
public interface UserDao {
void save();
}
public class UserDaoImpl implements UserDao {
public void save() {
System.out.println("hello world");
}
}
3、创建Spring核心配置文件
4、在Spring配置文件中配置UserDaoImpl
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="UserDao" class="UserDao.UserDaoImpl.UserDaoImpl"></bean>
</beans>
5、使用Spring的API获得Bean实例
public class UserDaoDemo {
public static void main(String[] args) {
ApplicationContext app = new ClassPathXmlApplicationContext("ApplicationContext.xml");
UserDao userDao =(UserDao) app.getBean("UserDao");
userDao.save();
}
}
## Spring配置文件
1、Bean标签基本配置
用于配置对象交由Spring来创建
默认情况下他调用的是类中的无参构造函数,如果没有无参构造函数则不能创建成功
基本属性:
id:Bean实例在Spring容器中的唯一标识
class:Bean的全限定名称
2、Bean标签范围配置
scope:值对象的作用范围,取值如下:
1、当scope的取值为singleton是
Bean的实例化个数:1个
Bean的实例化时机:当Spring核心文件被加载时,实例配置的Bean实例
Bean的生命周期:
对象创建:当应用加载,创建容器时,对象就被创建了
对象运行:只要容器在,对象一直活着
对象销毁:当应用卸载,销毁容器时,对象就销毁了
2、当scope的取值为prototype时
Bean的实例化个数:多个
Bean的实例化时机:当调用getBean()方法时实例化Bean
对象创建:当时用对象时,创建新的对象实例
对象运行:只要对象在使用中,就一直活着
对象销毁:当对象长时间不用是,被Java的垃圾回收期回收了
3、Bean生命周期配置
init-method:指定类中的初始化方法名称
destory-method:指定类中销毁方法名称
public UserDaoImpl(){
System.out.println("创建了");
}
public void init(){
System.out.println("初始化方法....");
}
public void destroy(){
System.out.println("销毁方法....");
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="UserDao" class="UserDao.UserDaoImpl.UserDaoImpl" init-method="init" destroy-method="destroy"></bean>
</beans>
public class SpringTest {
@Test
public void Test1(){
ClassPathXmlApplicationContext app = new ClassPathXmlApplicationContext("ApplicationContext.xml");
UserDao userDao1 =(UserDao) app.getBean("UserDao");
System.out.println(userDao1);
app.close();
}
}
4、Bean实例化三种方式
1、无参构造方法实例化
2、工厂静态方法实例化
<bean id="userDao" class="Factory.StaticFactory" factory-method="userDao"></bean>
public class StaticFactory {
public static UserDao userDao(){
return new UserDaoImpl();
}
}
@Test
public void Test1(){
ApplicationContext app = new ClassPathXmlApplicationContext("ApplicationContext.xml");
UserDao userDao1 =(UserDao) app.getBean("userDao");
System.out.println(userDao1);
}
3、工厂实例方法实例化
<bean id="factory" class="Factory.DynamicFactory"></bean>
<bean id="userDao" factory-bean="factory" factory-method="userDao"></bean>
public class DynamicFactory {
public UserDao userDao(){
return new UserDaoImpl();
}
}
@Test
public void Test1(){
ApplicationContext app = new ClassPathXmlApplicationContext("ApplicationContext.xml");
UserDao userDao1 =(UserDao) app.getBean("userDao");
System.out.println(userDao1);
}
5、Bean的依赖注入分析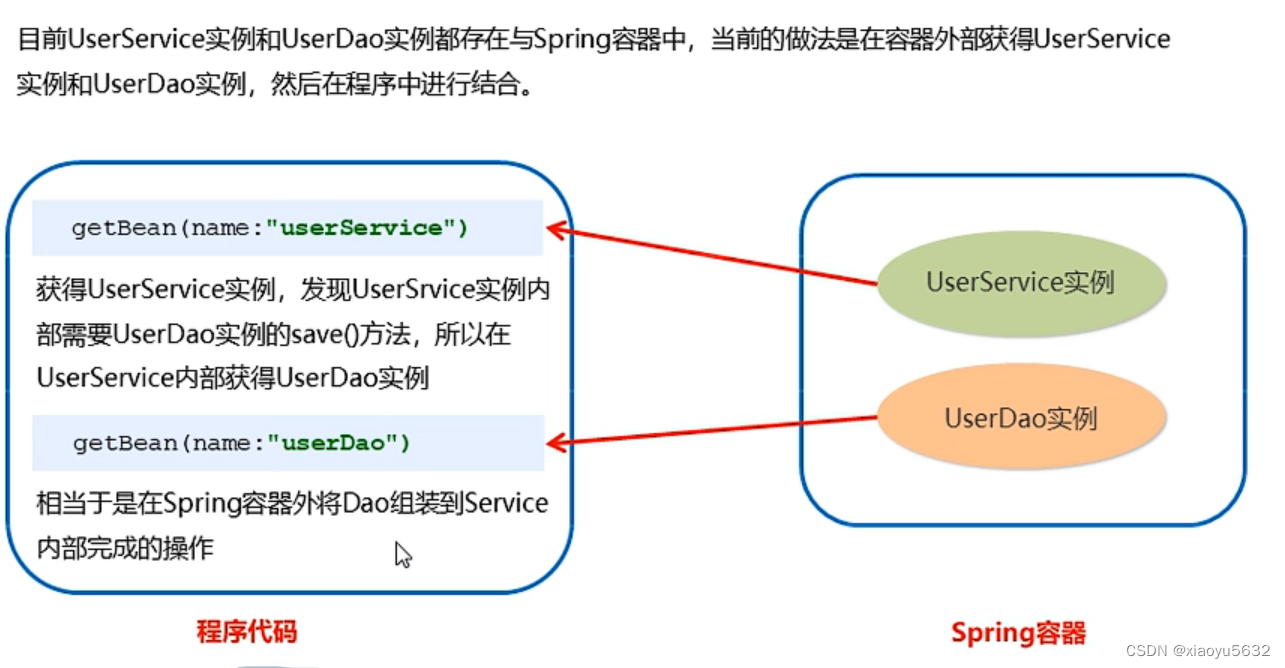
6、Bean的依赖注入概念
依赖注入(Dependency Injection ):他是Spring框架核心IOC的具体实现
在编写程序时,通过控制反转,把对象的创建交给了Spring,但是代码中不可能出现没有依赖的情况
IOC解耦只是降低他们的依赖关系,但不会消除,例如:业务层仍会调用持久层的方法
那种业务层和持久层的依赖关系,在使用Spring之后,就让Spring来维护了
简单的说,就是坐等框架把持久层对象传入业务层,而不用我们自己去获取
7、Bean的依赖注入方式
将UserDao注入到UserService内部中
1、构造方法
<bean id="userService" class="UserService.UserServiceImpl.UserServiceImpl">
<constructor-arg ref="userDao" name="userDao"></constructor-arg>
</bean>
private UserDao userDao;
public UserServiceImpl(UserDao userDao) {
this.userDao = userDao;
}
public UserServiceImpl() {
}
public void save() {
userDao.save();
}
2、set方法
<bean id="userService" class="UserService.UserServiceImpl.UserServiceImpl">
<property name="userDao" ref="userDao"></property>
</bean>
public static void main(String[] args) {
ApplicationContext app = new ClassPathXmlApplicationContext("ApplicationContext.xml");
UserService userService = (UserService) app.getBean("userService");
userService.save();
}
private UserDao userDao;
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
public void save() {
userDao.save();
}
8、Bean的依赖注入的数据类型
注入数据的三种数据类型
1、普通数据类型
<bean id="userDao" class="UserDao.UserDaoImpl.UserDaoImpl" >
<property name="age" value="18"></property>
<property name="username" value="刘泽宇"></property>
</bean>
private String username;
private int age;
public void setUsername(String username) {
this.username = username;
}
public void setAge(int age) {
this.age = age;
}
public void save() {
System.out.println(username+"====="+age);
System.out.println("hello world");
}
2、引用数据类型
3、集合数据类型
<bean id="userDao" class="UserDao.UserDaoImpl.UserDaoImpl">
<property name="list">
<list>
<value>aaa</value>
<value>nnn</value>
<value>cccc</value>
</list>
</property>
<property name="map">
<map>
<entry key="user1" value-ref="u1"></entry>
<entry key="user2" value-ref="u2"></entry>
</map>
</property>
<property name="properties">
<props>
<prop key="小红">111</prop>
<prop key="效率">222</prop>
<prop key="小兰">333</prop>
</props>
</property>
</bean>
<bean id="u1" class="Domain.User">
<property name="name" value="小明"></property>
<property name="addr" value="新乐"></property>
</bean>
<bean id="u2" class="Domain.User">
<property name="name" value="小网"></property>
<property name="addr" value="石家庄"></property>
</bean>
private List<String> list;
private Map<String, User> map;
private Properties properties;
public void setProperties(Properties properties) {
this.properties = properties;
}
public void setMap(Map<String, User> map) {
this.map = map;
}
public void setList(List<String> list) {
this.list = list;
}
@Override
public String toString() {
return "UserDaoImpl{" +
"map=" + map +
'}';
}
public void save() {
// System.out.println(username+"====="+age);
System.out.println("hello world");
System.out.println(list);
System.out.println(map);
System.out.println(properties);
}
9、引入其他配置文件(分模块开发)
实际开发中,Spring的配置内容非常多,这就导致Spring配置很繁杂且体积很大,所以,可以将部分配置拆解到其他配置文件汇总,儿子 啊 Spring主配置文件通过import标签进行加载
##Spring 相关 API
1、ApplicationContext的继承体系
applicationContext:接口类型,代表应用上下文,可以通过其实例获得Spring容器中的Bean对象
2、ApplicationContext的实现类
1、ClassPathXmlApplicationContext
他是从类的根路径下加载配置文件推荐使用这种
2、FileSystemXmlApplicationContext
他是从磁盘路径上加载配置文件,配置文件可以再磁盘的任意位置
3、AnnotationConfigApplicationContext
当使用注解配置容器对象时,需要使用此类来创建Spring容器,他用来读取注解
## Spring配置数据源
1、数据源(连接池)的作用
1. 数据源(连接池)是提高程序性能的
2. 事先实例化数据源,初始化部分链接资源
3.使用链接资源时从数据源中获取
4.使用完毕后将链接资源归还给数据源
常见的数据源*(连接池):DBCP、C3P0、BoneCP、Druid等
2、数据源的开发步骤
1、导入数据源的坐标和数据库驱动坐标
2、创建数据源对象
3、设置数据源的基本连接数据
4、使用数据源获取链接资源和归还链接资源
手动创建c3p0数据源
@Test
//测试手动创建c3p0数据源
public void test1() throws PropertyVetoException, SQLException {
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClass("com.mysql.jdbc.Driver");
dataSource.setJdbcUrl("jdbc:mysql://localhost:3306/test");
dataSource.setUser("root");
dataSource.setPassword("root");
Connection connection = dataSource.getConnection();
System.out.println(connection);
connection.close();
}
手动创建Druid数据源
@Test
public void test2() throws SQLException {
DruidDataSource druidDataSource = new DruidDataSource();
druidDataSource.setDriverClassName("com.mysql.jdbc.Driver");
druidDataSource.setUrl("jdbc:mysql://localhost:3306/test");
druidDataSource.setUsername("root");
druidDataSource.setPassword("root");
DruidPooledConnection connection = druidDataSource.getConnection();
System.out.println(connection);
connection.close();
}
抽取properties文件配置数据源
@Test
//创建c3p0数据源(配置properties文件)
public void test3() throws PropertyVetoException, SQLException {
ResourceBundle resourceBundle = ResourceBundle.getBundle("jdbc");
String driver = resourceBundle.getString("jdbc.Driver");
String url = resourceBundle.getString("jdbc.Url");
String username = resourceBundle.getString("jdbc.Username");
String password = resourceBundle.getString("jdbc.Password");
//创建数据源对象,设置链接参数
ComboPooledDataSource comboPooledDataSource = new ComboPooledDataSource();
comboPooledDataSource.setDriverClass(driver);
comboPooledDataSource.setJdbcUrl(url);
comboPooledDataSource.setUser(username);
comboPooledDataSource.setPassword(password);
Connection connection = comboPooledDataSource.getConnection();
System.out.println(connection);
connection.close();
}
3、Spring 配置数据源
可以将DataSource的创建权交由Spring容器去完成
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.jdbc.Driver"></property>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/test"></property>
<property name="user" value="root"></property>
<property name="password" value="root"></property>
</bean>
@Test
//Spring创建c3p0数据源
public void test4() throws Exception {
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("ApplicationContext1.xml");
ComboPooledDataSource dataSource = (ComboPooledDataSource) applicationContext.getBean("dataSource");
Connection connection = dataSource.getConnection();
System.out.println(connection);
connection.close();
}
4、抽取jdbc配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<context:property-placeholder location="jdbc.properties"></context:property-placeholder>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.Driver}"></property>
<property name="jdbcUrl" value="${jdbc.Url}"></property>
<property name="user" value="${jdbc.Username}"></property>
<property name="password" value="${jdbc.Password}"></property>
</bean>
</beans>
## Spring注解开发
1、Spring原始注解
Spring是轻代码而重配置的框架,配置比较繁重,影响开发效率,所以注解开发是一种趋势,注解代替xml配置文件,
可以简化配置,提高开发效率
2、Spring新注解
public class DataSourceConfig {
@Value("${jdbc.Driver}")
private String driver;
@Value("${jdbc.Url}")
private String url;
@Value("${jdbc.Username}")
private String username;
@Value("${jdbc.Password}")
private String password;
@Bean("dataSource")
public DataSource getDataSource() throws PropertyVetoException {
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClass(driver);
dataSource.setJdbcUrl(url);
dataSource.setUser(username);
dataSource.setPassword(password);
return dataSource;
}
}
//标志该类是Spring核心配置类
@Configuration
/*<!-- 注解的组件扫描-->
<context:component-scan base-package="UserDao"></context:component-scan>
<context:component-scan base-package="UserService"></context:component-scan>*/
@ComponentScan("UserDao")
@PropertySource("classpath:jdbc.properties")
@Import(DataSourceConfig.class)
public class SpringConfig {
}
## Spring继承Junit
1、原始Junit测试Spring的问题
在测试类中,每个测试方法都有以下两行代码:
这两行代码的作用 是获取容器,如果不写的话会,直接提示空指针异常,所以不能轻易删除。
2、解决思路
让SpringJunit负责创建Spring容器,但是需要将配置文件的名称告诉它
将需要进行测试Bean直接在测试类中进行注入
3、Spring继承Junit步骤
1、导入Spring继承Junit的坐标
2、使用@Runwith注解替换原来的运行器
3、使用@ContextConfiguration指定配置文件或配置类
4、使用@Autowired注入需要测试的对象
5、创建测试方法进行测试
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = {SpringConfig.class})
public class SpringJunitTest {
@Autowired
private UserService userService;
@Autowired
private DataSource dataSource;
@Test
public void test1() throws SQLException {
userService.save();
System.out.println(dataSource.getConnection());
}
}
## Spring集成web环境
知识要点
Spring集成web环境步骤
1、配置ContextLoaderListener监听器
2、使用WebApplicationContextUtils获得应用上下文
public class UserServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws javax.servlet.ServletException, IOException {
ServletContext servletContext = request.getServletContext();
WebApplicationContext app = WebApplicationContextUtils.getWebApplicationContext(servletContext);
UserDao userDao = app.getBean(UserDao.class);
userDao.save();
}
<!-- 全局初始化参数-->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:ApplicationContext.xml</param-value>
</context-param>
<!--配置监听器-->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
##SpringMVC简介
1、SpringMVC快速入门
需求:客户端发起请求,服务器端接受请求,执行逻辑并进行试图跳转
开发步骤:
1、导入SpringMVC相关坐标
2、配置SpringMVC核心控制器DispathcerServlet
<!-- 配置SpringMVC前段控制器-->
<servlet>
<servlet-name>DispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:spring-mvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>DispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
3、创建Controller类和视图页面
4、使用注解配置Controller类业务方法的映射地址
@Controller
public class UserController {
@RequestMapping
public String save(){
return "success.jsp"; //返回要跳转的页面
}
}
5、配置SpringMVC核心文件spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 组件扫描-->
<context:component-scan base-package="Controller"></context:component-scan>
</beans>
6、客户端发起请求测试
##SpringMVC组件解析
2、SpringMVC注解解析
@RequestMapping
作用:用于建立请求URL和处理请求方法之间的对应关系
位置:
类上,请求URL的第一级访问目录。此处不写的话,相当于应用的根目录
方法上,请求URL的第二级访问目录,与类上使用@RequestMapping标注的一级目录一起组成访问虚拟路径属性:
# value:用于指定请求的URL。他和path属性的作用是一样的
# method:用于指定请求的方式
# params:用于指定限制请求参数的条件,他支持简单的表达式。要求请求参数的key和value必须和配置的一模一样
例如:
params = {"accountName"},表示请求参数必须有accountName
params = {"money!100"} ,表示请求参数中Money不能是100
@RequestMapping("/user")
public class UserController {
//请求地址为localhost:8080/user/quick
@RequestMapping(value = "/quick",method = RequestMethod.POST,params = {"username"})
public String save(){
return "/success.jsp"; //返回要跳转的页面
}
}
知识要点
SpringMVC的相关组件
前段控制器:DispatcherServlet
处理器映射器:HandlerMapping
处理器适配器:HandlerAdapter
处理器:Handler
试图解析器:View Resolver
视图:View
SpringMVC的注解和配置
请求映射注解:@RequestMapping
视图解析器配置:
<!-- 配置内部资源视图解析器-->
<bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<!-- 加路径-->
<property name="prefix" value="/jsp/"></property>
<!-- 加后缀名-->
<property name="suffix" value=".jsp"></property>
</bean>
## SpringMVC的数据响应
1、SpringMVC的数据响应方式
1、页面跳转
直接返回字符串
通过ModelAndView对象返回
2、回写数据
直接返回字符串
返回对象或集合
2、页面跳转
1.返回字符串形式
直接返回字符串:此种方式会将返回的字符串与视图解析器的前后缀拼接后跳转
返回带有前缀的字符串:
转发:forward:/WEB-INF/view/index.jsp
重定向:redirect: /index.jsp
2、返回ModelAndView对象
@RequestMapping("/quick3")
public ModelAndView save2(ModelAndView modelAndView){
modelAndView.setViewName("success");
modelAndView.addObject("username","雄安王");
return modelAndView;
}
@RequestMapping("/quick4")
public Model save3(Model model){
model.addAttribute("username","基督教大家都");
return model;
}
@RequestMapping(value = "/quick1")
public ModelAndView save1(){
/*
* Model:模型 作用封装数据
* View:视图 作用于展示数据
* */
ModelAndView modelAndView = new ModelAndView();
//设置模型数据
modelAndView.addObject("username","xiaowan");
//设置视图名称
modelAndView.setViewName("index");
return modelAndView;
}
@RequestMapping("/quick5")
public String save5(HttpServletRequest httpServletRequest){
httpServletRequest.setAttribute("username","回到家四号和");
return "success";
}
3、回写数据
1、直接返回字符串
Web基础截断,客户端访问服务器端,如果想直接回写字符串作为响应体返回的话,只需要使用 response.getWriter().print("helloworld") 即可,那么在Controller中直接回写字符串该怎样呢?
1.通过SpringMVC框架注入的response对象,使用response.getWriter().print("helloworld")回写数据,此时不需要视图跳转,
业务方法返回值为void
2.将需要回写的字符串直接返回,但此时需要通过@ResponseBody注解告知SpringMVC框架,方法返回的字符串不是跳转是直接
在http响应体中返回
@RequestMapping("/quick6")
@ResponseBody //告知SpringMVC框架,不进行试图跳转,直接数据响应
public String save6(){
return "小 ,加完告白";
}
2、直接回写json格式字符串
@RequestMapping("/quick7")
@ResponseBody //告知SpringMVC框架,不进行试图跳转,直接数据响应
public String save7() throws JsonProcessingException {
User user = new User();
user.setAge(10);
user.setUsername("刘泽宇");
//使用json的转换工具将对象转换为json格式字符串在返回
ObjectMapper objectMapper = new ObjectMapper();
String s = objectMapper.writeValueAsString(user);
return s;
}
3、返回对象或集合
@RequestMapping("/quick8")
@ResponseBody //告知SpringMVC框架,不进行试图跳转,直接数据响应
public User save8() throws JsonProcessingException {
User user = new User();
user.setAge(10);
user.setUsername("刘泽宇");
//使用SpringMVC框架配置之后直接得出
return user;
}
<!-- 配置处理器映射器-->
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter">
<property name="messageConverters">
<list>
<bean class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter"></bean>
</list>
</property>
</bean>

<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!-- mvc的注解驱动-->
<mvc:annotation-driven></mvc:annotation-driven>
</beans>
4、获得请求参数
客户端请求参数的格式:name=value & name=value....
服务器端要获得的请求的参数,有时还需要进行数据的封装,SpringMVC可以接受如下类型的参数:
基本类型参数
POJO类型参数
数组类型参数
集合类型参数
1、获得基本参数类型
@RequestMapping("/quick9")
@ResponseBody
public void save9(String username,int age) throws JsonProcessingException {
System.out.println(username);
System.out.println(age);
}
2、获得POJO类型参数
Controller中的业务方法的POJO参数的属性名与请求参数的name一致,参数值会自动映射匹配
@RequestMapping("/quick10")
@ResponseBody
public void save10(User user) throws JsonProcessingException {
System.out.println(user);
}
3、获得数组类型参数
Controller中的业务方法数组名称与请求参数的name一致,参数值会自动映射匹配
@RequestMapping("/quick11")
@ResponseBody
public void save11(String[] strings) throws JsonProcessingException {
System.out.println(Arrays.asList(strings));
}
4、获得集合类型参数
获得集合参数时,要将参数包装到一个POJO中才可以 (不重要)
@RequestMapping("/quick12")
@ResponseBody
public void save12(Vo vo) throws JsonProcessingException {
System.out.println(vo);
}
public class Vo {
private List<User> list;
public List<User> getList() {
return list;
}
@Override
public String toString() {
return "Vo{" +
"list=" + list +
'}';
}
public void setList(List<User> list) {
this.list = list;
}
}
<form action="${pageContext.request.contextPath}/user/quick12" method="post">
<input type="text" name="userList[0].username"><br>
<input type="text" name="userList[0].age"><br>
<input type="text" name="userList[1].username"><br>
<input type="text" name="userList[1].age"><br>
</form>
当时用Ajax提交是,可以指定contentType为json形式,那么在方法参数位置使用@RequestBody可以直接接受集合数据而无需使用 POJO进行包装
静态资源的开启
<!-- 开发资源访问-->
<!--mapping是资源访问路径,location是所访问资源的所在的目录-->
<mbc:resources mapping="/js/**" location="/js/"></mbc:resources>
配置全局过滤的Filter
<!-- 配置全局过滤的filter-->
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
5、参数绑定注解@requestParam
当请求的参数名称与Controller的业务方法参数名称不一致时,就需要通过@RequestParam注解显示的绑定
注解@RequestParam还有如下参数可以使用:
value :与请求参数名称
required:再次指定的请求参数是否必须包括,默认是true,提交是如果没有参数会报错
defaultValue:当没有指定请求参数时,则使用指定的默认值赋值
@RequestMapping(value = "/quick12/${name}",method = RequestMethod.GET)
@ResponseBody
public void save13(@PathVariable(value = "name") String name) throws JsonProcessingException {
System.out.println(name);
}
9、自定义类型转换器
SpringMVC默认已经提供了一些常用的类型转换器,例如客户端提交的字符串转换成int类型进行参数设置
但是不是所有的数据类型都提供了转换器,没有提供的就需要自定义转换器,例如:日期类型的数据就需要自定义转换器
自定义类型转换器的开发步骤:
1.定义转换器类实现Converter接口
public class DateConverter implements Converter<String,Date> {
public Date convert(String dateStr) {
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
Date date = null;
try {
date=format.parse(dateStr);
} catch (ParseException e) {
e.printStackTrace();
}
return date;
}
@Override
public JavaType getInputType(TypeFactory typeFactory) {
return null;
}
@Override
public JavaType getOutputType(TypeFactory typeFactory) {
return null;
}
}
2.在配置文件中声明转换器
<!-- 声明转换器-->
<bean id="ConversionService" class="org.springframework.context.support.ConversionServiceFactoryBean">
<property name="converters">
<list>
<bean class="Converter.DateConverter"></bean>
</list>
</property>
</bean>
3.在<annotation-dribven>中引用转换器
<!-- mvc的注解驱动-->
<mvc:annotation-driven conversion-service="ConversionService"></mvc:annotation-driven>
5、文件上传
1.文件上传客户端三要素
表单项type = "file"
表单的提交方式是post
表单的enctype属性是多部分表单形式,及enctype = "multipart/form-data"
单文件上传步骤
1.导入fileupload和io坐标
2.配置文件上传解析器
<!-- 配置文件上传解析器-->
<bean id="commonsMultipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<!-- 上传文件的编码类型-->
<property name="defaultEncoding" value="UTF-8"/>
<!-- 上传文件总大小-->
<property name="maxUploadSize" value="500000"/>
<!-- 上传单个文件大小-->
<property name="maxUploadSizePerFile" value="40000000"/>
</bean>
3.编写文件上传代码
@RequestMapping("/quick15")
@ResponseBody
public void save15(String name, MultipartFile multipartFile) throws IOException {
System.out.println(name);
//获得上窜文件的名称
String filename = multipartFile.getOriginalFilename();
multipartFile.transferTo(new File("D:\\qqq"+filename));
}
@RequestMapping("/quick15")
@ResponseBody
public void save15(String name, MultipartFile[] multipartFile) throws IOException {
System.out.println(name);
//获得上窜文件的名称
for (MultipartFile file : multipartFile) {
String originalFilename = file.getOriginalFilename();
file.transferTo(new File("D:\\ww" + originalFilename));
}
}
## Spring JdbcTemplate基本使用
1、JdbcTemplate概述
他是Spring框架中提供的一个对象,是对原始繁琐的Jdbc API对象的简单封装。spring框架为我们提供了很多的操作模板类。
例如:操作关系型数据的Jdbc Template和HibernateTemplate ,操作nosql数据库的Redis Template,操作消息队列的JmsTemplate等
2、JdbcTemplate开发步骤
1.导入Spring-jdbc 和 pring-tx坐标
2.创建数据库表和实体
3.创建JdbcTemplate对象
4.执行数据库操作
@Test
public void test1() throws PropertyVetoException, SQLException {
//创建数据源对象
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClass("com.mysql.jdbc.Driver");
dataSource.setJdbcUrl("jdbc:mysql://localhost:3306/test");
dataSource.setUser("root");
dataSource.setPassword("root");
JdbcTemplate jdbcTemplate = new JdbcTemplate();
//设置数据源对象,知道数据源在哪
jdbcTemplate.setDataSource(dataSource);
//执行操作
int tom = jdbcTemplate.update("insert into account (name,money) value (?,?)", "tom", 50000);
System.out.println(tom);
}
3、Spring产生JdbcTemplate对象
我们可以将JdbcTemplate的创建权交给Spring,将数据源DataSource的创建权交给Spring,在Spring容器内部将数据源DataSource注
入到JdbcTemplate模板对象中,配置如下:
<!-- 配置数据源对象-->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.jdbc.Driver"></property>
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/test"></property>
<property name="user" value="root"></property>
<property name="password" value="root"></property>
</bean>
<!-- 配置JdbcTemplate模板对象-->
<bean id="JdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"></property>
</bean>
@Test
public void test2(){
ApplicationContext applicationContext = new ClassPathXmlApplicationContext();
JdbcTemplate jdbcTemplate = applicationContext.getBean(JdbcTemplate.class);
//执行操作
int tom = jdbcTemplate.update("insert into account (name,money) value (?,?)", "tom", 50000);
System.out.println(tom);
}
<context:property-placeholder location="jdbc.properties"></context:property-placeholder>
<!-- 配置数据源对象-->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driver}"></property>
<property name="jdbcUrl" value="${jdbc.url}"></property>
<property name="user" value="${jdbc.user}"></property>
<property name="password" value="${jdbc.password}"></property>
</bean>
4、JdbcTemplate的常用操作
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:ApplicationContext.xml")
public class JdbcTemplateCURDTest {
@Autowired
private JdbcTemplate jdbcTemplate;
@Test
public void test4(){
int tom = jdbcTemplate.update("update account set money=? where name=?", 22222, "tom");
System.out.println(tom);
}
@Test
public void test5(){
jdbcTemplate.update("delete from account where name=?","tom");
}
@Test
public void test6(){
List<Account> a = jdbcTemplate.query("select * from account", new BeanPropertyRowMapper<Account>(Account.class));
System.out.println(a);
}
@Test
public void test7(){
List<Account> accounts = jdbcTemplate.query("select * from account where name=?", new BeanPropertyRowMapper<Account>(Account.class), "小尾");
System.out.println(accounts);
}
@Test
public void test8(){
Long aLong = jdbcTemplate.queryForObject("select count(*) from account", Long.class);
System.out.println(aLong);
}
}
## SpringMVC拦截器
1、拦截器(interceptor)的作用
Spring MVC的拦截器类似于Servlet开发中的过滤器Filter,用于对处理器进行预处理和后处理
将拦截器按一定的顺序结成一条链,这条链称为拦截器链。在访问被拦截的方法或字段是,拦截器链中的拦截器就会按其他之前定义的
顺序被调用。拦截器也是AOP思想的具体实现
2、拦截器和过滤器的区别
3、快速入门
1、创建拦截器类实现HandlerInterceptor接口
public class MyInterceptor1 implements HandlerInterceptor {
//在目标方法执行前执行
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
System.out.println("pre........");
return true;
}
//在目标方法执行之后,视图方法执行之前 执行
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception {
System.out.println("post........");
}
//所有执行方法执行之后 ,执行
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception {
System.out.println("after........");
}
}
2、配置拦截器
<!--配置拦截器-->
<mvc:interceptors>
<mvc:interceptor>
<!--对那些资源进行拦截-->
<mvc:mapping path="/**"/>
<bean class="interceptor.MyInterceptor1"/>
</mvc:interceptor>
</mvc:interceptors>
3、测试拦截器的拦截效果
4、拦截方法说明
## Spring 异常处理
1、异常处理的思路
2、异常处理两种方式
1.使用SpringMVC提供的简单异常处理器SimpleMappingExpectionRosolver
2.使用Spring的异常处理接口HandlerExpectionResolver自定义自己的异常处理器
3、简单异常处理器SimpleMappingExpectionResovler
4、自定义异常处理步骤
1、创建异常处理器类实现HandlerExpectionResolver
2、配置异常处理器
3、编写异常页面
4、测试异常跳转
## Spring 的AOP 简介
1、什么是AOP?
2、AOP的作用及优势
作用:在程序运行期间,在不修改源码的情况下对方法进行功能增强
优势:减少重复代码,提高开发效率,并且便于维护
3、AOP的底层实现
4、AOP的动态代理技术
常用的动态代理技术
JDk代理:基于接口的动态代理技术
public class test {
public static void main(String[] args) {
final Target target = new Target();
final Advice advice = new Advice();
TaskInterce proxyInstance = (TaskInterce) Proxy.newProxyInstance(
target.getClass().getClassLoader(), //目标类对象代理器
target.getClass().getInterfaces(),//目标对象相同的接口字节码对象数组
new InvocationHandler() {
@Override
//调用代理对象的任何方法,实质是执行的都是invoke的方法
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
//前置增强
advice.before();
Object invoke = method.invoke(target, args);
//后置增强
advice.after();
return invoke;
}
}
);
proxyInstance.save();
}
}
cglib代理:基于父类的动态代理技术
public class test {
public static void main(String[] args) {
final Target target = new Target();
final Advice advice = new Advice();
//返回值就是生成的代理对象 基于cglib
//1.创建增强器
Enhancer enhancer = new Enhancer();
//2.设置父类目标
enhancer.setSuperClass(Target.class);
//3.设置回调
enhancer.setCallback(new MethodInterceptor()){
public Object intercept(Object proxy,Method method,Object[] args,MethodProxy me){
advice.before();
Object invoke = method.invoke(target,args); //执行目标
advice.after();
return invoke;
}
}
//4.创建代理对象
Target pro = enhancer.create();
pro.save();
}
}
5、AOP相关概念
常用术语:
* Target(目标对象):代理的目标对象
* Proxy(代理):一个类被AOP织入增强后,就产生了一个结果代理类
* Joinpoint(连接点):所谓连接点是指那些被拦截到的点。在spring中,这些点指的是方法,因为spring只支持方法类型的链接点
* Pointcut(切入点):所谓切入点是指我们要对那些Joinpoint进行拦截的定义
* Advice(通知/增强):所谓通知是指拦截到Joinpoint之后所要做的事情就是通知
* Aspect(切面):是切入点和通知(引介)的结合
* Weaving(织入):是指把增强应用到目标对象来创建新的代理对象的过程。spring采用动态代理织入,而Aspect采用编译期织入和类 装载期织入
6、AOP开发明确的事项
1、需要编写的内容
* 编写核心业务代码(目标类的目标方法)
*编写切面类,切面类汇总有通知(增强功能方法)
*在配置文件中,配置织入关系,即将那些通知与哪些连接点进行结合
2、 AOP技术实现的内容
Spring框架监控切入点方法的执行。一旦监控到切入点方法被运行,使用代理机制,动态创建目标对象的代理对象
根据通知类别,在代理对象的对应位置,将通知对应的功能织入,完成完整的代码逻辑运行
3、AOP底层使用哪种代理方式
在spring,中框架会根据目标类是否实现类接口来决定采用哪种动态代理的方式
## 基于XML的AOP开发
1、快速入门
1.导入AOP相关坐标
2.创建目标接口和目标类(内部有切点)
3.创建切面类(内部有增强方法)
4.将目标类和切面类的对象创建权交给spring
5.在applicationContext.xml中配置织入关系
6.测试代码
<!--目标对象-->
<bean id="target" class="AOP.Target"></bean>
<!-- 切面对象-->
<bean id="myAsect" class="AOP.MyAsect">
</bean>
<!-- 配置织入 :告诉spring框架 哪些方法(切点)需要进行哪些增强(前置、后置...)-->
<aop:config>
<!-- 声明切面-->
<aop:aspect ref="myAsect">
<!-- 切面:切点+通知-->
<!--method:前置增强的方法名称,pointcut:需要增强的方法路径-->
<aop:before method="before" pointcut="execution(public void save())"></aop:before>
</aop:aspect>
</aop:config>
2、切点表达式写法
表达式语法:
execution ([修饰符] 返回值类型 包名.类名.方法名(参数))
# 访问修饰符可以省略
# 返回值类型、包名、类名、方法名可以使用星号*代表任意
# 包名与类名之间一个点,代表当前包下的类,两个点.. 表示当前包及其子包下的类
# 参数列表可以使用两个点.. 表示任意个数,任意类型的参数列表
例如:
3、通知的类型
通知的配置语法
<aop : 通知类型 method="切面类中方法名" pontcut="切点表达式"></aop:通知类型>
4、切点表达式的抽取
## 基于注解的AOP开发
1、快速入门
基于注解的AOP开发步骤:
1.创建目标接口类和目标类(内部有切点)
2.创建切面类(内部有增强方法)
3.将目标类和切面类的对象创建全交给spring
4.在切面类中使用注解配置织入关系
5.在配置文件中开启组件扫描和AOP的自动代理
6.测试
2、注解通知的类型
通知的配置语法: @通知注解("切点表达式")
3、切点表达式的抽取
## 编程式事务控制相关对象
1、PlatformTransactionManager(事务平台管理器)
PlatformTransactionManager接口是spring的事务管理器,他里面提供了我们常用的操作事务的方法
2、TransactionDefinition
TransactionDefinition是事务的定义信息对象,里面有如下方法:
1.事务隔离级别
设置隔离级别,可以解决事务并发产生的问题,如脏读、不可重复和虚读
2.事务传播行为
3、TransactionStatus
TransactionStatus接口提供的是事务具体的运行状态,方法介绍如下:
## 基于XML的声明式事务控制
1、什么是声明式事务控制
Spring的声明式事务顾名思义就是采用声明的方式来处理事务。这里所说的声明,就是指在配置文件中声明
用在Spring配置文件中声明式的处理事务来代替代码式的处理事务
声明式事务处理的作用:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd
">
<!--目标对象 内部的方法就是切点-->
<bean id="accountService" class="com.itheima.service.impl.AccountServiceImpl">
<property name="accountDao" ref="accountDao"/>
</bean>
<!--配置平台事务管理器-->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<!--通知 事务的增强-->
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<!--设置事务的属性信息的-->
<tx:attributes>
<tx:method name="transfer" isolation="REPEATABLE_READ" propagation="REQUIRED" read-only="false"/>
<tx:method name="save" isolation="REPEATABLE_READ" propagation="REQUIRED" read-only="false"/>
<tx:method name="findAll" isolation="REPEATABLE_READ" propagation="REQUIRED" read-only="true"/>
<tx:method name="update*" isolation="REPEATABLE_READ" propagation="REQUIRED" read-only="true"/>
<tx:method name="*"/>
</tx:attributes>
</tx:advice>
<!--配置事务的aop织入-->
<aop:config>
<aop:pointcut id="txPointcut" expression="execution(* com.itheima.service.impl.*.*(..))"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="txPointcut"/>
</aop:config>
</beans>
## Mybatis简介
1、原始jdbc操作分析
2、什么是Mybatis
## Mybatis的快速入门
1、mybaits开发步骤
1.添加Mybaits的坐标
2.创建user数据表
3.编写User实体类
4.编写映射文件UserMapper.xml (就是写sql语句的)
<?xml version="1.0" encoding="utf-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="userMapper">
<select id="findAll" resultType="domain.User">
select * from user;
</select>
</mapper>
5.编写核心文件SqlMapConfig.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--数据源环境-->
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"></transactionManager>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/test"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
</environments>
<!--加载映射文件-->
<mappers>
<mapper resource="UserMapper.xml"></mapper>
</mappers>
</configuration>
6.编写测试类
@Test
public void test1() throws IOException {
//获得核心配置文件
InputStream resourceAsStream = Resources.getResourceAsStream("sqlMapConfig.xml");
//获得session工厂对象
SqlSessionFactory build = new SqlSessionFactoryBuilder().build(resourceAsStream);
//获得session会话对象
SqlSession sqlSession = build.openSession();
//执行操作 参数:namespace+id
List<User> user = sqlSession.selectList("userMapper.findAll");
System.out.println(user);
//释放资源
sqlSession.close();
}
## MyBatis的映射文件概述
## MyBatis的增删改查数据操作
1、插入操作注意问题
*插入语句使用insert标签
*在映射文件中使用parameterType属性指定要插入的数据类型
*Sql语句中使用#{实体属性名}方式引用实体中的属性值
*插入操作使用的API是sqlSession.insert(“命名空间+id”,实体对象)
*插入操作设计数据库数据变化,所以要使用sqlSession对象显示提交事务 .commit()
2、删除操作注意问题
*删除语句使用delete标签
*Sql语句中使用#{任意字符串}方式引用传递的单个参数
*删除操作使用的是API是sqlSession.delete(“命名空间.id”,Object) ;
3、修改操作注意问题
* 修改语句使用update标签
* 修改操作使用的是API时sqlSession.update(“命名空间.id”,实体对象);
## MyBatis核心配置文件概述
1、MyBatis核心配置文件层级关系
2、environments标签
3、mapper标签
4、properties标签
5、typeAliases标签
## MyBatis相关API
1、SqlSession工厂构建器SqlSessionFactopryBuilder
2、SqlSession工厂对象SqlSessionFactory
3、SqlSession会话对象
## MyBatis的DAO层实现
1、代理开发方式介绍
<mapper namespace="dao.UserMapper">
<!--接口代理实现查找-->
<select id="findById" parameterType="int" resultType="User">
select * from user where id=#{id}
</select>
</mapper>
public interface UserMapper {
public User findById(int id);
}
public static void main(String[] args) throws IOException {
InputStream resourceAsStream = Resources.getResourceAsStream("sqlMapConfig.xml");
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(resourceAsStream);
SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获得代理对象
UserMapper userMapper = sqlSession.getMapper(UserMapper.class);
User byId = userMapper.findById(1);
System.out.println(byId);
}
## MyBatis映射文件深入
1、动态sql语句
动态sql之 <if>
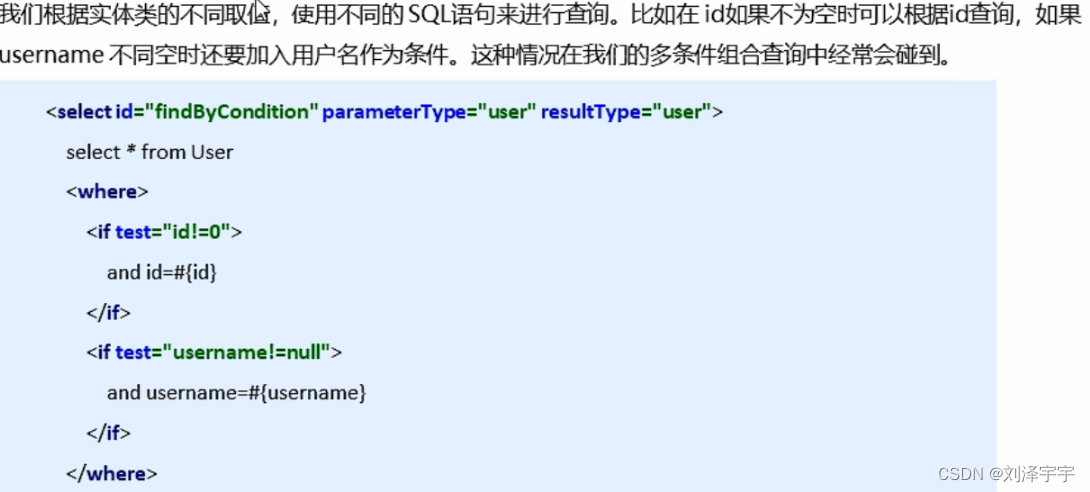
<!--动态sql实现-->
<select id="findByCon" parameterType="User" resultType="domain.User">
select * from user;
<where>
<if test="id != 0">
id=#{id}
</if>
<if test="username != null">
username=#{username}
</if>
<if test="password != null">
password=#{password}
</if>
</where>
</select>
动态sql之<foreach>
sql片段抽取
## MyBatis核心配置文件深入
1、typeHandlers标签
public class DateTypeHandler extends BaseTypeHandler<Date> {
//将java类型转换成数据库需要的类型
@Override
public void setNonNullParameter(PreparedStatement preparedStatement, int i, Date date, JdbcType jdbcType) throws SQLException {
long time = date.getTime();
preparedStatement.setLong(i,time);
}
//将数据库中的类型转换成java类型
@Override
public Date getNullableResult(ResultSet resultSet, String s) throws SQLException {
long along = resultSet.getLong(s);
Date date = new Date(along);
return date;
}
//将数据库中的类型转换成java类型
@Override
public Date getNullableResult(ResultSet resultSet, int i) throws SQLException {
long aLong = resultSet.getLong(i);
Date date = new Date(aLong);
return date;
}
//将数据库中的类型转换成java类型
@Override
public Date getNullableResult(CallableStatement callableStatement, int i) throws SQLException {
long aLong = callableStatement.getLong(i);
Date date = new Date(aLong);
return date;
}
}
<!--注册类型处理器-->
<typeHandlers>
<typeHandler handler="handler.DateTypeHandler"></typeHandler>
</typeHandlers>
2、plugins标签
<!--配置分页助手插件-->
<plugins>
<plugin interceptor="com.github.pagehelper.PageHelper">
<!--注册方言-->
<property name="dialect" value="mysql"/>
</plugin>
</plugins>
@Test
public void test7() throws IOException {
InputStream resourceAsStream = Resources.getResourceAsStream("sqlMapConfig.xml");
SqlSessionFactory build = new SqlSessionFactoryBuilder().build(resourceAsStream);
SqlSession sqlSession = build.openSession(true);
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
//设置分页相关参数 当前页+每页显示的条数
PageHelper.startPage(1,3);
List<User> list = mapper.findAll();
for (User user : list) {
System.out.println(user);
}
//获得分页相关的参数
PageInfo<User> pageInfo = new PageInfo<>(list);
System.out.println("当前页:"+pageInfo.getPageNum());
System.out.println("每页显示条数:"+pageInfo.getPageSize());
System.out.println("总条数:"+pageInfo.getTotal());
System.out.println("总页数:"+pageInfo.getPages());
System.out.println("上一页:"+pageInfo.getPrePage());
System.out.println("下一页:"+pageInfo.getNextPage());
System.out.println("是否为第一页:"+pageInfo.isIsFirstPage());
System.out.println("是否为最后一页:"+pageInfo.isIsLastPage());
sqlSession.close();
}
#MyBatis多表查询
1、一对一查询
1.一对一查询的模型
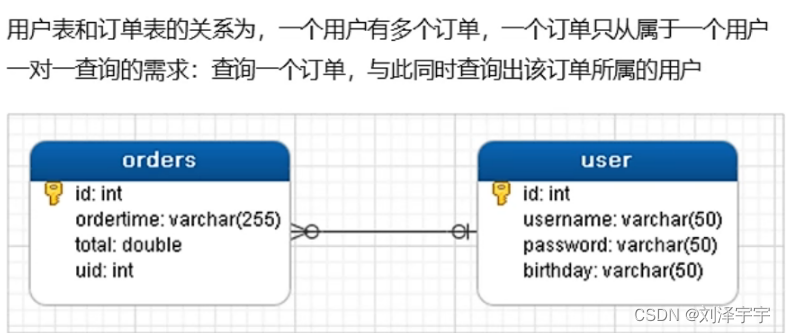
<!--一对一表查询-->
<resultMap id="orderMap" type="order">
<!--手动指定字段与实体属性的映射关系
column:数据表的字段名称 相当于别名
property:实体的属性名称 真正的名
-->
<id column="oid" property="id"></id>
<result column="ordertime" property="ordertime"></result>
<result column="total" property="total"></result>
<!--<result column="uid" property="user.id"></result>
<result column="password" property="user.password"></result>
<result column="birthday" property="user.birthday"></result>-->
<!--第二种方式-->
<!--
property:当前实体(order)中的属性名称(private User user)
javaType:当前实体(order)中的属性的类型(User)
-->
<association property="user" javaType="domain.User">
<id column="uid" property="id"></id>
<result column="username" property="username"></result>
<result column="password" property="password"></result>
<result column="birthday" property="birthday"></result>
</association>
</resultMap>
<select id="findAll" resultMap="orderMap">
select *,o.id oid from order o, user u where o.oid=u.id
</select>
2、一对多查询的模型
<!--一对多表实现-->
<resultMap id="userMap" type="domain.User">
<id column="uid" property="id"></id>
<result column="username" property="username"></result>
<result column="password" property="password"></result>
<result column="birthday" property="birthday"></result>
<!--配置集合信息
property:集合名称
ofType:当前集合中的数据类型
-->
<collection property="orderList" ofType="domain.Order">
<!--封装的order数据-->
<id column="oid" property="id"></id>
<result column="ordertime" property="ordertime"></result>
<result column="total" property="total"></result>
</collection>
</resultMap>
<select id="findAlll" resultMap="userMap">
select *,o.id oid from user u.order o where u.id=o.uid;
</select>
3、多对多查询的模型
## MyBatis注解开发
1、MyBatis的常用注解
@Insert:实现新增
@Update:实现更新
@Delete:实现删除
@Select:实现查询
@Result:实现结果集封装
@Results:可以与@Result一起使用,封装多个结果集
@One:实现一对一结果集封装
@Many:实现一对多结果集封装
//一对一查询注解实现
@Select("select * from orders")
@Results(
{
@Result(column = "id",property = "id"),
@Result(column = "ordertime",property = "ordertime"),
@Result(column = "total",property = "total")
@Result(
property = "user", //要封装的属性名称
column = "uid", //根据那个字段去查询user表的数据
javaType = User.class, //要封装的实体类型
//select属性 代表查询那个接口的方法获得数据
one = @One(select = "dao.UserMapper.findById")
)
}
)
//一对多查询注解实现
@Select("select * from user")
@Results(
{
@Result(id = true,column = "id",property = "id"),
@Result(column = "ordertime",property = "ordertime"),
@Result(column = "total",property = "total"),
@Result(
property = "orderList",
column = "id",
javaType = List.class,
many = @Many(select = "domain.OrderMapper.findById")
)
}
)
SSM整合自己学去吧 ,反正我已经会了 hhhhhhhhhhhhhhhhhhhhhhhhhhhh




