夫传子
father.vue
<template>
<div>
<h3>这是父组件</h3>
<input type="text" placeholder="请输入老婆的名字" v-model="wife">
<p>老婆的名字是:{{ wife }}</p>
<son :mother="wife"></son>
</div>
</template>
<script>
// 子组件需要父组件传递过来的数据,就写到props里
// 写到props代表这个数据是父组件传递过来
// 父组件怎么传?在用这个子组件标签的地方写,:props里的属性
// 例如::mother="给子组件传递的值"
import son from './son.vue'
export default {
components:{
son
},
data() {
return {
wife:""
}
},
}
</script>
<style>
</style>
son.vue
<template>
<div class="son">
<h3>这是儿子</h3>
<p>妈妈的名字是:{{ mother }}</p>
</div>
</template>
<script>
export default {
props:[ 'mother' ]
}
</script>
<style>
.son{
width: 200px;
height: 200px;
background-color: #f00;
}
</style>
- 夫组件中 在input中通过v-model 绑定 wife
- 导入子组件
- 在
\<son 这里> \</son>
中设置 子组件需要接收的值 - 因为子组件需要通过mother 接收 所以 设置为
:mother="wife"
//wife 父组件中绑定的值 - 子组件中 通过
props:[ 'mother' ]
进行接收 - 在要使用的地方设置
{ mother }
转化
// 子组件需要父组件传递过来的数据,就写到props
里
// 写到props
代表这个数据是父组件传递过来
// 父组件怎么传?在用这个子组件标签的地方写,:props
里的属性
// 例如::mother="给子组件传递的值"
图解
在这里插入图片描述
子传夫
father.vue
<template>
<div>
<h3>这是父组件</h3>
<son @xx="erxi = $event"></son>
<p>儿媳妇的名字是:{{ erxi }}</p>
</div>
</template>
<script>
/*
1.找传递的时机
2.写$emit传递数据,参数1:事件名 参数2:传递的值
3.父组件监听这个事件,拿到$event,赋值给自己想要的地方
*/
import son from './son.vue'
export default {
components:{
son
},
data() {
return {
erxi:"暂时为空"
}
},
}
</script>
<style>
</style>
son.vue
<template>
<div class="son">
<h3>这是儿子</h3>
<input type="text" @input="$emit('xx',wife)" v-model="wife" placeholder="请输入老婆名字">
<p>老婆名字是:{{ wife }}</p>
</div>
</template>
<script>
export default {
data() {
return {
wife:""
}
},
}
</script>
<style>
.son{
width: 200px;
height: 200px;
background-color: #f00;
}
</style>
- 在子组件中 通过
@input = " $emit( '事件名' , 传递的值 ) "
把值暴露出来 - 在父组件中通过
<son @事件名 = " 本页面需要修改的值 = $event ">
图解
组件间传值的 v-model 方法
father.vue
<template>
<div>
<h3>这是父组件</h3>
<input type="text" v-model="wife">
<!-- <son :mother="wife" @xx="wife = $event"></son> -->
<!--
想找个东西帮我们生成
:mother="wife"
xx = wife=$event
那就是v-model
-->
<son v-model="wife"></son>
</div>
</template>
<script>
import son from './son.vue'
export default {
components:{
son
},
data() {
return {
wife:""
}
},
}
</script>
<style>
</style>
son.vue
<template>
<div class="son">
<h3>这是儿子</h3>
<!--
给文本框既可以赋值,也可以取值
取值:就是把文本框输入的内容给了mother
mother是写到props里,代表只能是父组件传过来的
vue不建议我们直接修改这种父组件传递过来的值
修改就会报错,要遵循单向数据流规则
直接传值:只能是父传子
我现在只是想把父组件传递过来的值仍在
input里显示,而不需要input修改它
input有个属性叫value专门用来设置文本框默认值的
v-bind:value="mother"
但是我现在就是想把文本框内容传递给父组件
又没法直接改mother,怎么办?
就用之前的子传父,虽然不直接,但有效
$event在原生标签里是事件对象
事件对象有个属性叫target找到事件源
此案例里事件源是input
input有个属性叫value就可以获取到它输入的内容
-->
<input @input="$emit('xx',$event.target.value)" type="text" :value="mother">
</div>
</template>
<script>
export default {
props:[ 'mother' ],
model:{
prop:"mother",
event:"xx"
}
}
</script>
<style>
.son{
width: 200px;
height: 200px;
background-color: #f00;
}
</style>
图解 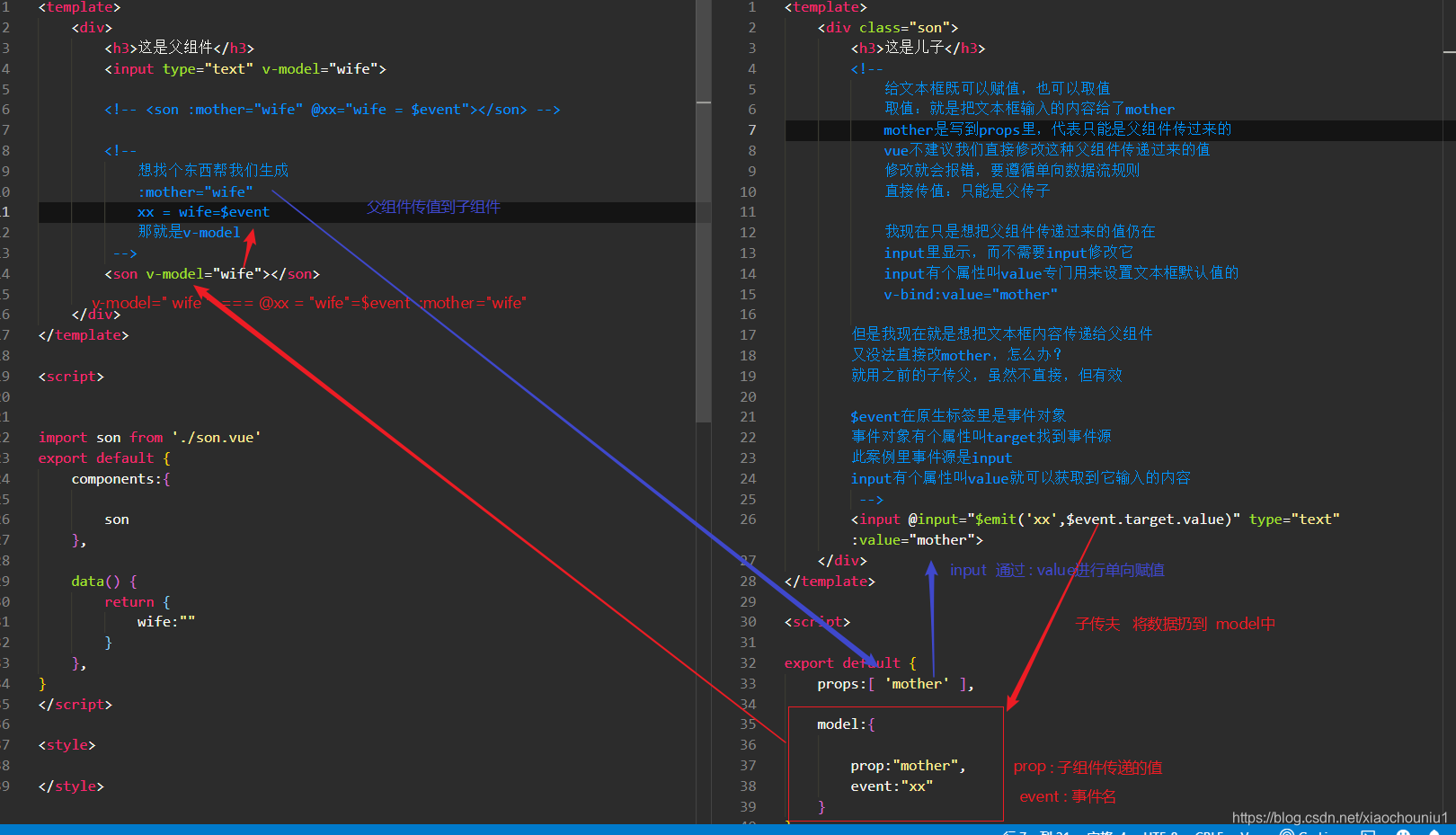
语法 & 注意点
1 . 夫传子
1.1.1 夫: 先要导入子组件 并且给接口 方法: components:{son},
1.1.2 在接口位置 设置夫组件需要传递的值名称 要加冒号 <son :mother="wife"></son>
1.2.1 子 props : [ 子组件接收数据名 ] : 夫传子 子接收的地方
1.2.2 在需要用到值的位置 设置 子组件接收到的数据名
2. 子传夫
2.1 子 通过 @input="$emit('事件名',子页面中的值)" 传递值
2.2 夫 通过在接口位置设置 @事件名="夫页面需要修改的值 = $event"
$event 在组件中为 传递的值
不在组件中就是事件对象
3. v-model的使用
3.1 父组件中 v-model:本页面需要传的值 === :子组件页面需要接收的值="夫组件页面需要传递的值" @事件名 = " ' 事件名' =$event"
3.2 子组件中 需要设置model : { prop : 父组件对应要传的值
event : 对应的事件名
}
还需要设置 prop是:[ ' 需要从父组件传来的值 名称 ' ]
互传
父组件
<template>
<channelpopup v-model=" 本页面的数据" />
</template>
<scipt>
import channnelpopup from '子组件路劲'
comonnents:{ channelpopup }
data (){return{ show:'' }}
`
子组件
<tempulate>
<van-popup
:value = 'value'
@input="$emit('input', false)"
/>
</tempulate>
<script>
props:[ ' value ' ]
</script>
当父组件使用的是v-model 那么子组件可以直接使用 props[ ’ value ’ ] 传值或者赋值