弹框可以对一些时间长的操作进行一种显著的提示,或者对于操作状态的警示, h5中的window.alert 也是开发中debug错误的一种方式, 所以弹框算是常用的一种组件.
swing实现弹框
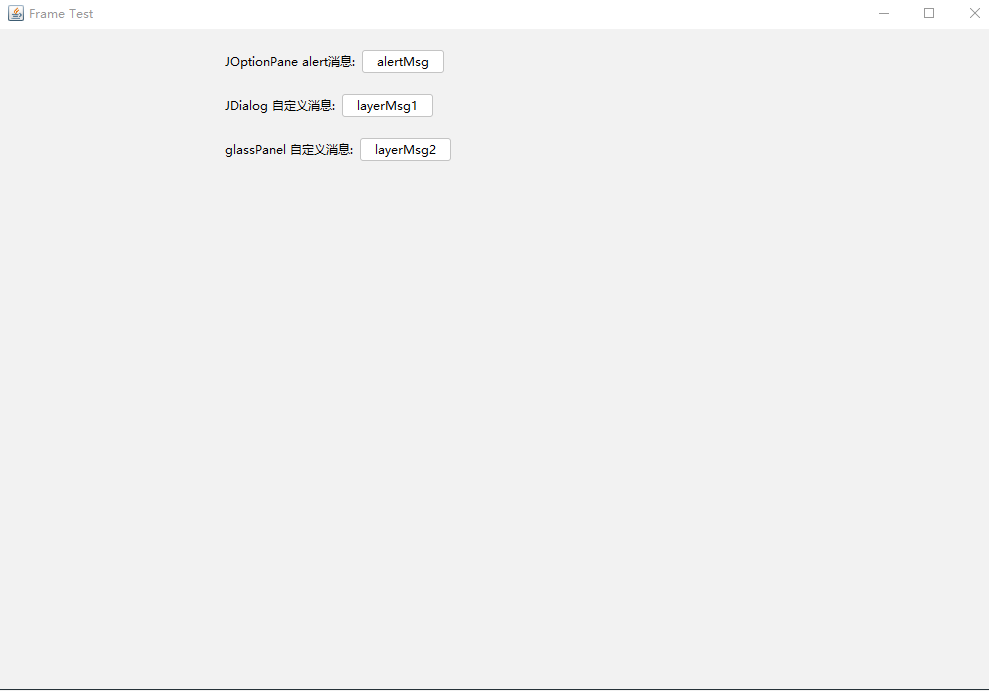
- JOptionPane 是最常用的类似于alert的弹框
- JDialog 可以实现自定义弹框
- GlassPane 也可以实现弹框 (JDialog 弹框会使主屏幕失去焦点,在一些场景上可能欠妥,GlassPane的提示可以很好的替代)
测试代码
package com.mynote.example.demo.tool;
import com.formdev.flatlaf.FlatLightLaf;
import com.mynote.core.comp.DialogLoading;
import com.mynote.core.ui.FontBuilder;
import com.mynote.core.ui.IconBuilder;
import com.mynote.core.util.CompBuilder;
import com.mynote.core.util.FrameUtil;
import com.mynote.example.demo.AbstractDefaultPanel;
import net.miginfocom.swing.MigLayout;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionListener;
public class LayerMsgTest extends AbstractDefaultPanel {
private JButton alertMsg;
private JButton layerMsg1;
private JButton layerMsg2;
@Override
protected void init() {
alertMsg = new JButton("alertMsg");
layerMsg1 = new JButton("layerMsg1");
layerMsg2 = new JButton("layerMsg2");
}
@Override
protected void render() {
view.setLayout(new MigLayout("wrap 1"));
super.createViewRow(new JLabel("JOptionPane alert消息:"), alertMsg);
super.createViewRow(new JLabel("JDialog 自定义消息:"), layerMsg1);
super.createViewRow(new JLabel("glassPanel 自定义消息:"), layerMsg2);
super.add(view);
}
@Override
protected void bindEvents() {
alertMsg.addActionListener((e) -> {
JOptionPane.showMessageDialog(null, "请稍候", "提示", JOptionPane.YES_OPTION);
});
layerMsg1.addActionListener((e) -> {
this.showDialogMsg(getFrame());
});
layerMsg2.addActionListener((e) -> {
this.showGlassPaneMsg(getFrame());
});
}
private JFrame getFrame() {
Window win = SwingUtilities.getWindowAncestor(view);
JFrame frame = (JFrame) win;
if (frame == null) {
throw new RuntimeException("获得不到JFrame窗口");
}
return frame;
}
private void showDialogMsg(JFrame frame) {
SwingUtilities.invokeLater(() -> {
DialogLoading loading = new DialogLoading(frame);
loading.showLoading("加载中...", IconBuilder.ICON_LOADING);
onceRun((event) -> {
loading.hideLoading();
});
});
}
private void showGlassPaneMsg(JFrame frame) {
JPanel bottomPanel = new JPanel(new MigLayout());
bottomPanel.setOpaque(false);
bottomPanel.add(createMessage(), "pos 0.5al 0.5al");
SwingUtilities.invokeLater(() -> {
frame.setGlassPane(bottomPanel);
frame.getGlassPane().setVisible(true);
onceRun((event) -> {
frame.getGlassPane().setVisible(false);
});
});
}
private JPanel createMessage() {
JPanel panel = new JPanel(new MigLayout("w 300,h 240"));
panel.setBackground(Color.WHITE);
JLabel msg = new JLabel("处理完成");
msg.setFont(FontBuilder.getLabelFont(20f));
panel.add(new JLabel(IconBuilder.ICON_COMPLETE), "pos 0.5al 0.5al");
panel.add(msg, "pos 0.5al 0.8al");
return panel;
}
private void onceRun(ActionListener actionListener) {
Timer timer = new Timer(2000, actionListener);
timer.setRepeats(false);
timer.start();
}
public static void main(String[] args) {
FlatLightLaf.install();
FrameUtil.launchTest(new LayerMsgTest());
}
}