<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<style>
body {
padding: 0;
margin: 0;
}
.box {
display: none;
width: 400px;
height: 300px;
text-align: center;
border: 1px solid #cccccc;
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
z-index: 2;
background-color: #ffffff;
}
.form {
line-height: 50px;
}
.close {
width: 60px;
height: 60px;
border-radius: 50%;
position: absolute;
right: -30px;
top: -30px;
line-height: 60px;
text-align: center;
background-color: #ffffff;
}
.logbg {
display: none;
width: 100%;
height: 100%;
position: absolute;
left: 0;
top: 0;
background-color: rgba(0, 0, 0, 0.4);
}
.title {
height: 50px;
line-height: 50px;
cursor: move;
}
</style>
</head>
<body>
<button class="open">点击,弹出登录框</button>
<div class="box">
<div style="position: relative">
<div class="title">登录会员</div>
<div class="form">
用户名<input typeof="text">
</div>
<div class="form">
登录密码<input typeof="text">
</div>
<button>登录会员</button>
<a href="javascript:;" class="close">关闭</a>
</div>
</div>
<div class="logbg"></div>
<script>
var box = document.querySelector('.box');
var title = document.querySelector('.title');
var open = document.querySelector('.open');
var logbg = document.querySelector('.logbg');
open.addEventListener('click', function () {
box.style.display = 'block';
logbg.style.display = 'block';
})
title.addEventListener('mousedown', function (e) {
console.log(e)
var left = e.pageX - box.offsetLeft;
var top = e.pageY - box.offsetTop;
function move(e) {
box.style.left = e.pageX - left + 'px';
box.style.top = e.pageY - top + 'px';
}
document.addEventListener('mousemove', move);
document.addEventListener('mouseup', function (e) {
document.removeEventListener('mousemove', move)
})
})
</script>
</body>
</html>
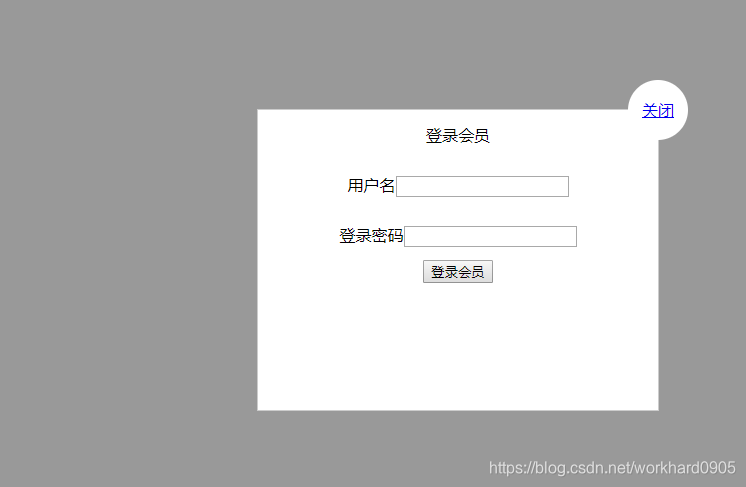