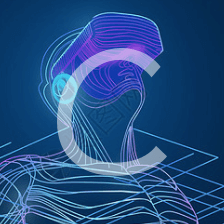
leetcode
Spider--Man
灵魂画家
展开
-
344. Reverse String
Write a function that takes a string as input and returns the string reversed. 字符串反转/** * @param {string} s * @return {string} */var reverseString = function(s) { return s.split('').reverse().原创 2017-03-28 22:03:48 · 207 阅读 · 0 评论 -
136. Single Number
Given an array of integers, every element appears twice except for one. Find that single one. 整数数组,除了一个元素出现两次,其它均出现一次,求出现一次的值 Note: Your algorithm should have a linear runtime complexity. Could you原创 2017-03-26 20:04:20 · 197 阅读 · 0 评论 -
350. Intersection of Two Arrays II
Given two arrays, write a function to compute their intersection. 求两个数组的交集Example: Given nums1 = [1, 2, 2, 1], nums2 = [2, 2], return [2, 2].Note: Each element in the result should appear as many ti原创 2017-03-26 20:52:47 · 200 阅读 · 0 评论 -
Missing letters
Find the missing letter in the passed letter range and return it.If all letters are present in the range, return undefined.判断字符串相邻字符是否连续,若连续返回undefined,否则返回缺失字符function fearNotLetter(str) { var arr原创 2017-04-01 11:23:15 · 685 阅读 · 1 评论 -
DNA Pairing
The DNA strand is missing the pairing element. Take each character, get its pair, and return the results as a 2d array.Base pairs are a pair of AT and CG. Match the missing element to the provided char原创 2017-04-01 11:07:41 · 393 阅读 · 0 评论 -
Pig Latin
Translate the provided string to pig latin.Pig Latin takes the first consonant (or consonant cluster) of an English word, moves it to the end of the word and suffixes an “ay”.If a word begins with a vo原创 2017-04-01 11:01:42 · 452 阅读 · 0 评论 -
Search and Replace
Perform a search and replace on the sentence using the arguments provided and return the new sentence.First argument is the sentence to perform the search and replace on.Second argument is the word tha原创 2017-04-01 10:38:06 · 429 阅读 · 0 评论 -
Wherefore art thou
返回collection内对象包含source对象内所有属性,并且值相等function whatIsInAName(collection, source) { // What's in a name? var arr = []; // Only change code below this line collection.forEach((cur) => { var has原创 2017-04-01 10:22:27 · 439 阅读 · 0 评论 -
Roman Numeral Converter
Roman Numeral Converter 把数字转换成罗马数字转换规则function convertToRoman(num) { var a=[["","I","II","III","IV","V","VI","VII","VIII","IX"], ["","X","XX","XXX","XL","L","LX","LXX","LXXX","XC"],原创 2017-04-01 01:01:31 · 378 阅读 · 0 评论 -
Diff Two Arrays
Diff Two Arrays 求两个数组不同的值function diffArray(arr1, arr2) { var newArr = []; // Same, same; but different. var arr1Diff = arr1.filter((cur) => { return arr2.indexOf(cur) === -1; }); var a原创 2017-04-01 00:37:51 · 731 阅读 · 0 评论 -
Sum All Numbers in a Range
Sum All Numbers in a Range 求两个数字间所有数字之和function sumAll(arr) { var min = Math.min(arr[0], arr[1]); var max = Math.max(arr[0], arr[1]); var arrOpen = []; for (var i = min; i<=max; i++){ arrO原创 2017-04-01 00:33:39 · 529 阅读 · 0 评论 -
206. Reverse Linked List
Reverse a singly linked list. 反转单向链表解题思路:迭代或递归迭代/** * Definition for singly-linked list. * function ListNode(val) { * this.val = val; * this.next = null; * } *//** * @param {ListNode}原创 2017-03-29 22:56:05 · 225 阅读 · 0 评论 -
504. Base 7
Given an integer, return its base 7 string representation.把一个整数转换成7进制的字符串解题思路:直接toString(7)或除7取余/** * @param {number} num * @return {string} */var convertToBase7 = function(num) { return num.to原创 2017-03-29 22:06:32 · 210 阅读 · 0 评论 -
104. Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth. 求二叉树的最大深度 The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node. 最大深度是自根节点到最远叶子节点的距离解题思路:函数递归原创 2017-03-26 22:17:45 · 182 阅读 · 0 评论