1.先从gitee上获取最新的代码库,在data文件夹内获得ip2region.db文件
代码下载
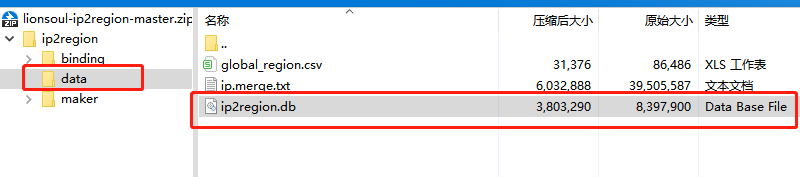
2.放进项目内的resources文件夹内
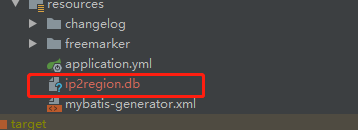
3.项目中引入ip2region
<!-- ip定位-->
<dependency>
<groupId>org.lionsoul</groupId>
<artifactId>ip2region</artifactId>
<version>1.7.2</version>
</dependency>
4.通过工具类实现IP获取地址信息的功能,此处放一份我自己稍微改了下往上代码的工具类
package com.sunny.system.util;
import java.io.File;
import java.io.FileNotFoundException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import io.swagger.annotations.ApiModelProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.lionsoul.ip2region.*;
public class IpUtil {
public static void main(String[] args) throws FileNotFoundException, NoSuchMethodException, DbMakerConfigException, IllegalAccessException, InvocationTargetException {
System.out.println(getCityInfo("112.80.18.246"));
}
private static DataBlock getBlockData(String ip) throws FileNotFoundException, DbMakerConfigException, NoSuchMethodException, InvocationTargetException, IllegalAccessException {
if (!Util.isIpAddress(ip)) {
throw new IllegalArgumentException("parse failed,invalid ip address");
}
String dbPath = IpUtil.class.getResource("/ip2region.db").getPath();
File file = new File(dbPath);
if (!file.exists()) {
throw new FileNotFoundException("ip db file not exist!");
}
int algorithm = DbSearcher.BTREE_ALGORITHM;
DbSearcher searcher = new DbSearcher(new DbConfig(), dbPath);
Method method = searcher.getClass().getMethod("btreeSearch", String.class);
return (DataBlock) method.invoke(searcher, ip);
}
public static String getCityString(String ip) throws InvocationTargetException, FileNotFoundException, IllegalAccessException, DbMakerConfigException, NoSuchMethodException {
DataBlock dataBlock = getBlockData(ip);
return dataBlock.getRegion();
}
public static IpAddressInfo getCityInfo(String ip) throws InvocationTargetException, FileNotFoundException, IllegalAccessException, DbMakerConfigException, NoSuchMethodException {
DataBlock dataBlock = getBlockData(ip);
String[] regionInfo = dataBlock.getRegion().split("\\|");
return new IpAddressInfo(dataBlock.getCityId(), regionInfo[0], regionInfo[1], regionInfo[2], regionInfo[3], regionInfo[4]);
}
@Data
@NoArgsConstructor
@AllArgsConstructor
@Builder
public static class IpAddressInfo {
@ApiModelProperty(value = "城市id")
private Integer cityId;
@ApiModelProperty(value = "国家")
private String country;
@ApiModelProperty(value = "区域")
private String area;
@ApiModelProperty(value = "省份")
private String province;
@ApiModelProperty(value = "城市")
private String city;
@ApiModelProperty(value = "互联网服务提供商(Internet Service Provider)")
private String isp;
}
}