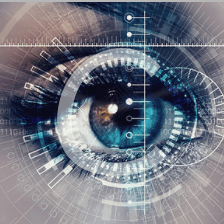
leetcode
GoRustNeverStop
这个作者很懒,什么都没留下…
展开
-
leetcode: Maximum Depth of Binary Tree
Given a binary tree, find its maximum depth.The maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.BFS:/** * Definition for bina原创 2014-11-29 14:09:09 · 586 阅读 · 0 评论 -
leetcode: Convert Sorted Array to Binary Search Tree
Given an array where elements are sorted in ascending order, convert it to a height balanced BST./** * Definition for binary tree * struct TreeNode { * int val; * TreeNode *left; *原创 2014-12-10 23:20:33 · 586 阅读 · 0 评论 -
leetcode: Merge Two Sorted Lists
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists.analysis: find a main link and a sub link./** *原创 2014-12-11 22:22:52 · 585 阅读 · 0 评论 -
[leetcode]爬楼梯的递归和非递归方法
【Description】You are climbing a stair case. It takes n steps to reach to the top.Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?【分析】利用枚原创 2015-05-18 23:31:00 · 4374 阅读 · 0 评论 -
[leetcode]101.Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie, symmetric around its center).For example, this binary tree is symmetric: 1 / \ 2 2 / \ / \3 4 4 3But the f原创 2016-01-03 23:09:36 · 486 阅读 · 0 评论 -
【leetcode】107. Binary Tree Level Order Traversal II
Given a binary tree, return the bottom-up level order traversal of its nodes' values. (ie, from left to right, level by level from leaf to root).For example:Given binary tree {3,9,20,#,#,15,7},原创 2016-01-05 22:42:29 · 641 阅读 · 0 评论 -
[leetcode] 369. Plus One Linked List 解题报告
题目链接: https://leetcode.com/problems/plus-one-linked-list/Given a non-negative number represented as a singly linked list of digits, plus one to the number.The digits are stored such that t原创 2018-01-16 09:15:50 · 552 阅读 · 0 评论 -
[leetcode]647. Palindromic Substrings
Given a string, your task is to count how many palindromic substrings in this string.The substrings with different start indexes or end indexes are counted as different substrings even they consis原创 2018-01-31 15:28:01 · 431 阅读 · 0 评论 -
Longest Palindromic Substring Part II
Longest Palindromic Substring Part IINovember 20, 2011 by 1337c0d3r 158 RepliesGiven a string S, find the longest palindromic substring in S.Note:This is Part II of the article转载 2018-02-06 15:34:09 · 296 阅读 · 0 评论 -
A summary: how to use bit manipulation to solve problems easily and efficiently
WikiBit manipulation is the act of algorithmically manipulating bits or other pieces of data shorter than a word. Computer programming tasks that require bit manipulation include low-level device cont...转载 2018-03-31 11:17:18 · 295 阅读 · 0 评论 -
leetcode: Majority Element
Given an array of size n, find the majority element. The majority element is the element that appears more than ⌊ n/2 ⌋ times.You may assume that the array is non-empty and the majority element原创 2014-12-22 23:06:40 · 724 阅读 · 0 评论 -
leetcode: Binary Tree Inorder Traversal
Given a binary tree, return the inorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,3,2].Note: Recursive solutio原创 2014-12-01 20:56:47 · 612 阅读 · 0 评论 -
leetcode:Binary Tree Preorder Traversal
Given a binary tree, return the preorder traversal of its nodes' values.For example:Given binary tree {1,#,2,3}, 1 \ 2 / 3return [1,2,3].Note: Recursive soluti原创 2014-11-30 15:32:34 · 510 阅读 · 0 评论 -
LeetCode:Single Number
Given an array of integers, every element appears twice except for one. Find that single one.Note:Your algorithm should have a linear runtime complexity. Could you implement it without using ext原创 2014-11-29 13:26:50 · 621 阅读 · 0 评论 -
leetcode:Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321Example2: x = -123, return -321Have you thought about this?Here are some good questions to ask before coding. Bonus points for you原创 2014-11-29 19:00:08 · 515 阅读 · 0 评论 -
leetcode:Linked List Cycle
Given a linked list, determine if it has a cycle in it.Follow up:Can you solve it without using extra space?/** * Definition for singly-linked list. * struct ListNode { * int val; *原创 2014-11-30 11:30:31 · 579 阅读 · 0 评论 -
leetcode:Same Tree
Given two binary trees, write a function to check if they are equal or not.Two binary trees are considered equal if they are structurally identical and the nodes have the same value./**原创 2014-11-30 15:34:56 · 562 阅读 · 0 评论 -
leetcode: Intersection of Two Linked Lists
Write a program to find the node at which the intersection of two singly linked lists begins.For example, the following two linked lists:A: a1 → a2 ↘原创 2014-11-29 13:35:59 · 591 阅读 · 0 评论 -
leetcode:Search Insert Position
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order.You may assume no duplicates in the array.原创 2014-11-30 10:23:16 · 582 阅读 · 0 评论 -
leetcode:Remove Duplicates from Sorted List
Given a sorted linked list, delete all duplicates such that each element appear only once.For example,Given 1->1->2, return 1->2.Given 1->1->2->3->3, return 1->2->3./** * Definition原创 2014-12-01 21:04:46 · 589 阅读 · 0 评论 -
leetcode: Remove Element
Given an array and a value, remove all instances of that value in place and return the new length.The order of elements can be changed. It doesn't matter what you leave beyond the new length.解原创 2014-12-01 22:58:46 · 595 阅读 · 0 评论 -
[Leetcode]17. Letter Combinations of a Phone Number
[Description]Given a digit string, return all possible letter combinations that the number could represent.A mapping of digit to letters (just like on the telephone buttons) is given below.Input:Digit...原创 2018-03-31 15:54:25 · 179 阅读 · 0 评论