文件编程概述:
应用中比如:账单,游戏进度,配置文件等。
用代码操作文件:实现文件创建,打开,编辑等自动化执行。
那么在Linux中如何编写一个文件呢?
步骤:打开(open)/创建(creat)->读写(read/write)->关闭(close)。
1.打开/创建文件:open()函数
#include <sys/types.h>
#include <sys/stat.h>
#include <fcutl.h>
int open(const char *pathname,int flags);
const char *pathname 要打开文件名(包含路径)
int flags 标志,指打开文件的方式
flags:
O_RDONLY 只读打开
O_WRONLY 只写打开
O_RDWR 可读可写)
以上这三个常数中应当只指定一个。下列常数是可选择的:
O_CREAT 若文件不存在则创建它。使用此选项时,需要同时说明第三个参数mode,
用其说明该新文件的存取许可权限。
O_EXCL 如果同时指定了OCREAT,而文件已经存在,则出错,返回-1。
O_APPEND 每次写时都加到文件的尾端。
O_TRUNC 属性去打开文件时,如果这个文件中本来是有内容的,而且为只读或只写成功打开,
则将其长度截短为0。
int open(const char *patname,int flags,mode_t mode);
前两个参数和上面一样
mode_t mode :一定是在flags中使用了O_CREAT标志,mode记录待创建的文件的访问权限
例:open("./file1",O_RDWR|O_CREAT,0600);//以可读可写的方式打开文件,如果没有就创
//建一个可读可写的文件。权限0600,6=4+2;2可写 4可读。
open函数返回的是一个文件描述符,是一个非负整数。
什么是文件描述符:对于内核而言,所有打开文件都由文件描述符引用。当打开一个现存文件或者创建一个新文件时,内核向进程返回一个文件描述符。
open函数打开文件,打开成功返回一个文件描述符,打开失败则返回-1。
下面用代码举例
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include<stdio.h>
int main ()
{
int fd;//定义一个文件标识符
fd=open("./file1",O_RDWR);//以可读可写的方式打开
printf("%d\n",fd);//输出文件标识符的值
return 0;
}

在文件三种打开模式之外,还有几种模式
O_CREAT:若文件不存在则创建它
O_EXCL:如果同时制定了O_CREAT,而文件存在,则返回-1。
O_APPEND:每次写时都加到文件的尾端。
O_TRUNC:去打开问件事,如果原文件有内容,则把原文覆盖。
举例:
O_CREAT:若文件不存在则创建它
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include<stdio.h>
int main ()
{
int fd;
fd=open("./file2",O_RDWR|O_CREAT,0600);//以可读可写的方式打开file2,没有则创建它。
printf("%d\n",fd);
return 0;
}
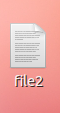
创建成功。
O_EXCL:如果同时制定了O_CREAT,而文件存在,则返回-1。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include<stdio.h>
int main ()
{
int fd;
fd=open("./file2",O_EXCL|O_CREAT,0600);
printf("fd=%d\n",fd);
return 0;
}

O_APPEND:每次写时都加到文件的尾端。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include<stdio.h>
#include<string.h>
int main ()
{
int fd;
char *buf="this is new add";
fd=open("./file2",O_RDWR|O_APPEND);//每次打开在file2原有的内容后加上缓冲区buf的内容。
write(fd,buf,strlen(buf));
close(fd);
return 0;
}
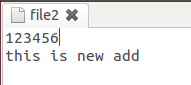
O_TRUNC:去打开问件事,如果原文件有内容,则把原文覆盖。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include<stdio.h>
#include<string.h>
int main ()
{
int fd;
char *buf="NEW TXT";
fd=open("./file2",O_RDWR|O_TRUNC);//O_TRUNC每次打开文件都会把原来内容覆盖
write(fd,buf,strlen(buf));
close(fd);
printf("fd=%d\n",fd);
return 0;
}

2.创建文件creat()函数
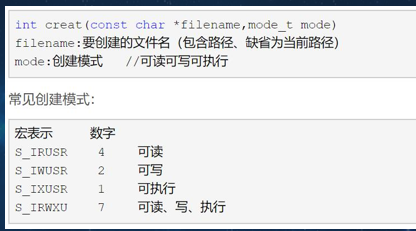
int creat(const char *filename,mode_t mode);
filebane:要创建的文件名(包含路径、缺省为当前路径)
mode:创建模式 //可读可写可执行
常见创建模式:
宏表示 | 数字 | |
S_IRUSR | 4 | 可读 |
S_IWUSR | 2 | 可写 |
S_IXUSR | 1 | 可执行 |
S_IRWXU | 7 | 可读可写可执行 |
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include<stdio.h>
#include<string.h>
int main ()
{
int fd;
fd=creat("./file3",S_IRWXU);
return 0;
}
3.文件写入操作:write()函数
#include <unistd.h>
ssize_t write(int fd, const void *buf, size_t count);
int fd 文件描述符
const void *buf 文件缓冲区,把这个文件写入到fd中
size_t count 要写入的大小
可简单理解为:将缓冲区的数据,写count个字节,写到fd中,写入成功的话则返回字节大小,没有内容则返回0,错误写入则返回-1。
代码示例如下:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
int main ()
{
int fd;
char *buf="CLC henshuai";
fd=open("./file1",O_RDWR|O_CREAT,0600);
write(fd,buf,strlen(buf));//strlen函数计算字符数量
close(fd);
return 0;
}
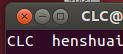
file1文件内容写入成功。
4.文件读取操作:read()函数
#include <unistd.h>
ssize_t read(int fd, void *buf, size_t count);
int fd 文件描述符
const void *buf 读上来的数据保存在缓冲区buf中,同时文件的当前读写位置向后移
size_t count 请求读取的字节数
返回值:正确读取则返回读取到的内容字节大小,没有则返回0,错误读取则返回-1。
代码示例:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main ()
{
int fd;
char *buf="CLC henshuai";
fd=open("./file1",O_RDWR|O_CREAT,0600);
int n_write=write(fd,buf,strlen(buf));
lseek(fd,0,SEEK_SET);
char* readBuf=(char *)malloc(sizeof(char)*n_write);
i int n_read=read(fd,readBuf,n_write);
printf("n_read:%d context:%s\n",n_read,readBuf);
close(fd);
return 0;
}
//lseek(fd,0,SEEK_SET); 光标重新回到初始位置
//(char *)malloc(sizeof(char)*n_write); 动态创建一个char大小的空间 乘以n_write个
//(char *) 强制转换分配的内存为char类型的指针

输出读取字节数量和输出内容。
5.关闭文件操作:close()函数
#include <unistd.h>
int close(int filedes);
int filedes 指定文件,一般为定义的文件标识符
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
int main ()
{
int fd;
char *buf="CLC henshuai";
fd=open("./file1",O_RDWR|O_CREAT,0600);
write(fd,buf,strlen(buf));//strlen函数计算字符数量
close(fd);//关闭文件
return 0;
}
关闭指定文件。
6.文件光标位置lseek()函数
#include <sys/types.h>
#include <unistd.h>
off_t lseek(int fd, off_t offset, int whence);
int fd 文件标识符
off_t offset 偏移值
int whence 固定位置
固定位置:
1.SEEK_SET lseek(fd,0,SEEK_SET);//指向文件的头位置
2.SEEK_CUR lseek(fd,0,SEEK_CUR);//指向当前光标位置
3.SEEK_END lseek(fd,0,SEEK_END);//指向文件的尾位置
返回值:返回针对文件开头的偏移值,出错返回-1。
理解:将文件读写指针相对whence移动offset个字节。
计算文件字节大小
代码如下:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main ()
{
int fd;
char *buf="CLC henshuai";
fd=open("./file1",O_RDWR|O_CREAT,0600);
if(fd==-1)
{
printf("open fail\n");
if(fd>0)
{
printf("open successfully\n");
}
}
int n_write=write(fd,buf,strlen(buf));
// close(fd);
// open("./file1",O_RDWR);
// off_t lseek(int fd, off_t offset, int whence);
lseek(fd,0,SEEK_SET);
char* readBuf=(char *)malloc(sizeof(char)*n_write);
int n_read=read(fd,readBuf,n_write);
printf("read:%d context:%s\n",n_read,readBuf);
//file size
int filesize=lseek(fd,0,SEEK_CUR);
printf("filesize is %d\n",filesize);
close(fd);
return 0;
}
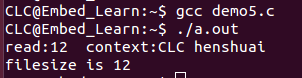