与队列有关的卡牌插入游戏
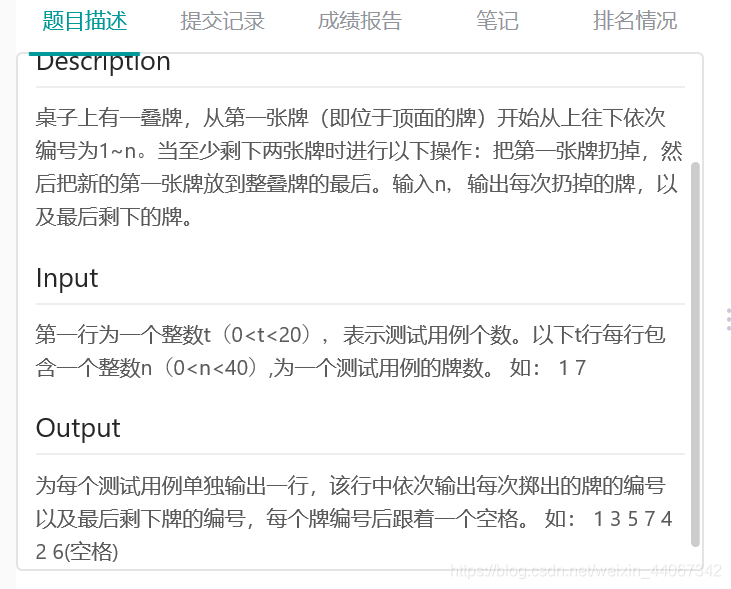
#include"stdio.h"
#include"stdlib.h"
typedef struct QNode {
int data;
struct QNode* next;
}QNode, * QueuePtr;
typedef struct {
QueuePtr front;
QueuePtr rear;
}LinkQueue;
int InitStack(LinkQueue& S) {
S.front = (QueuePtr)malloc(sizeof(QNode));
S.rear = S.front;
if (!S.front)
return 0;
S.front->next = NULL;
return 1;
}
int EnQueue(LinkQueue& S, int e) {
QueuePtr p = NULL;
p = (QueuePtr)malloc(sizeof(QNode));
if (!p)exit(1);
p->data = e;
p->next = 0;
S.rear->next = p;
S.rear = p;
return 1;
}
void ClearQueue(LinkQueue& S) {
QueuePtr p = NULL;
p = S.front;
while (S.front != S.rear) {
p = S.front->next;
free(S.front);
S.front = p;
}
}
void QueueEmpty(LinkQueue& S) {
if (S.front == S.rear)
printf("判空:是\n");
else
printf("判空:否\n");
}
int QueueLen(LinkQueue& S) {
QueuePtr p = NULL;
int len = 0;
p = S.front;
if (S.front == S.rear)
return len;
else {
while (p != S.rear) {
len++;
p = p->next;
}
return len;
}
}
int pop(LinkQueue& S, LinkQueue& T) {
int tem = 0;
QueuePtr p = NULL;
if (S.front->next == NULL)
return 0;
else {
tem = S.front->next->data;
EnQueue(T, tem);
p = S.front->next;
free(S.front);
S.front = p;
return tem;
}
}
int pop1(LinkQueue& S) {
int tem = 0;
QueuePtr p = NULL;
if (S.front->next == NULL)
return 0;
else {
tem = S.front->next->data;
EnQueue(S, tem);
p = S.front->next;
free(S.front);
S.front = p;
return tem;
}
}
void QueueTraverse(LinkQueue& S) {
QueuePtr p = S.front;
while (p != S.rear) {
printf("%d ", p->next->data);
p = p->next;
}
}
int main() {
LinkQueue S;
InitStack(S);
LinkQueue T;
InitStack(T);
LinkQueue R;
InitStack(R);
int a, b;
scanf_s("%d",&a);
for (int i = 0; i < a; i++) {
scanf_s("%d", &b);
EnQueue(S, b);
}
while (QueueLen(S) > 2) {
pop(S, T);
pop1(S);
}
QueueTraverse(T);
QueueTraverse(S);
return 0;
}