1. 二叉树的前序遍历
1)方法1:递归
private void preOrder(TreeNode root){
if(root != null){
System.out.print(root.val + " ");
}
if(root.left != null){
preOrder(root.left);
}
if(root.right != null){
preOrder(root.right);
}
}
2)非递归
private List<Integer> preOrder(TreeNode root){
List<Integer> res = new ArrayList<Integer>();
if(root == null){
return res;
}
Stack<TreeNode> stack = new Stack<>();
while(root != null){
while(root.left != null){
res.push(root.val);
stack.add(root);
root = root.left;
}
root = stack.pop().right;
}
return res;
}
2. 检查两棵树是否相等
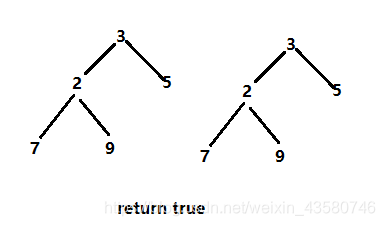
private boolean isSameTree(TreeNode s, TreeNode t){
if(s == null && t == null){
return true;
}
if(s == null || t == null){
return false;
}
if(s.val == t.val){
return isSameTree(s.left, t.left) && isSameTree(s.right, t.right);
} else {
return false;
}
}
3. 检查一个树是否是另一个树的子树
private boolean isSubtree(TreeNode s, TreeNode t){
if(s == null && t != null){
return false;
}
return isSameTree(s,t) || isSubtree(s.left, t) || isSubtree(s.right, t);
}
private boolean isSameTree(TreeNode s, TreeNode t){
if(s == null && t == null){
return true;
}
if(s == null || t == null){
return false;
}
if(s.val == t.val){
return isSameTree(s.left, t.left) && isSameTree(s.right, t.right);
} else {
return false;
}
}
4. 二叉树的最大深度
public int maxDepth(TreeNode root) {
if(root == null){
return 0;
}
if(root != null && root.left == null && root.right == null){
return 1;
}
int leftLength = 0;
if(root.left != null){
leftLength = maxDepth(root.left) + 1;
}
int rightLength = 0;
if(root.right != null){
rightLength = 1 + maxDepth(root.right);
}
return leftLength > rightLength ? leftLength : rightLength;
}
5. 判断一个树是否是二叉平衡树