C++语言,用顺序栈实现十进制向任意进制的转换
using namespace std;
typedef int ElemType;
struct Stack{
ElemType *stack;
int top;
int MaxSize;
};
void InitStack(Stack& S){
S.MaxSize=10;
S.stack=new ElemType[S.MaxSize];
if(!S.stack){
cerr<<"动态存储空间分配失败!"<<endl;
exit(1);
}
S.top=-1;
}
void Push(Stack& S,ElemType item){
if(S.top==S.MaxSize-1){
int k=sizeof(ElemType);
S.stack=(ElemType*)realloc(S.stack,2*S.MaxSize*k);
S.MaxSize=2*S.MaxSize;
}
S.top++;
S.stack[S.top]=item;
}
ElemType Pop(Stack& S){\
if(S.top==-1){
cerr<<"Stack is empty!"<<endl;
exit(1);
}
S.top--;
return S.stack[S.top+1];
}
ElemType Peek(Stack& S){
if(S.top==-1){
cerr<<"Stack is empty!"<<endl;
exit(1);
}
return S.stack[S.top];
}
bool EmptyStack(Stack& S){
return S.top==-1;
}
void ClearStack(Stack& S){
if(S.stack){
delete []S.stack;
S.stack=0;
}
S.top=-1;
S.MaxSize=0;
}
main(){
Stack s;
InitStack(s);
int n,b;
cout<<" 请输入待转换的十进制数:"<<endl;
cin>>n;
cout<<" 请输入转换的进制:"<<endl;
cin>>b;
while(n){
int i=n%b;
Push(s,i);
n=n/b;
}
cout<<"转换结果为:";
while(!EmptyStack(s)){
cout<<Pop(s);
}
cout<<endl;
cout<<endl;
cout<<endl;
while(1)
main();
}
运行结果
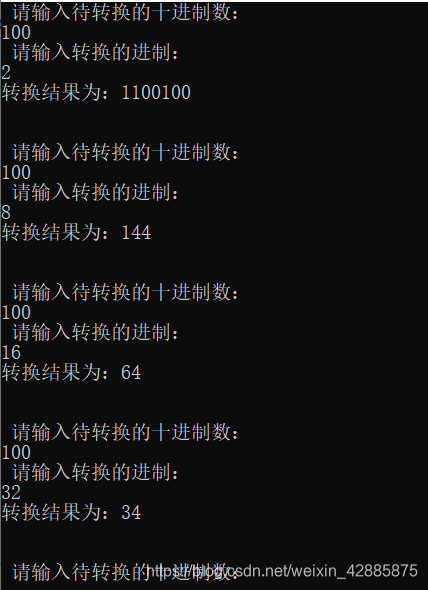