Vue.js
Download node.js from https://nodejs.org/en/:
Node.js integrates NPM and you can view the version using the following command:
$ node -v
v10.13.0
$ npm -v
6.4.1
Install vue-cli:
$ npm install -g vue-cli
Open the execution command in the project directory to create the project:
$ vue init webpack <projectName>
Test run:
$ cd lanye_project_name
$ npm run dev
If something goes wrong in the process, you can’t download node_modules
, you can install again:
$ npm install
This command will find
lanye_project_name/package.json
- dependencies and install these tonode_modules
package.
+ iView
Install iView on your project:
$ cnpm install --save iview
If it works, lanye_project_name/package.json
- dependencies will add iview
:
"dependencies": {
"iview": "^3.2.1",
"vue": "^2.5.2",
"vue-router": "^3.0.1"
}
And lanye_project_name/node_modules
will have iview
package:
Import iView globally: lanye_project_name/src/main.js
:
import iView from 'iview'
import 'iview/dist/styles/iview.css'
Vue.use(iView)
Complete code:
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import router from './router'
import iView from 'iview'
import 'iview/dist/styles/iview.css'
Vue.config.productionTip = false
Vue.use(iView)
/* eslint-disable no-new */
new Vue({
el: '#app',
router,
components: { App },
template: '<App/>'
})
And then, you can use iView on the whole project. IView docs: https://www.iviewui.com/docs/guide/install-en
+ i18n
Install iView on your project:
$ cnpm install --save i18n
If it works, lanye_project_name/package.json
- dependencies will add vue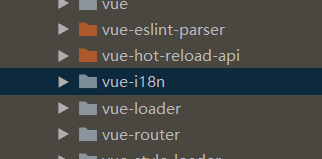i18n
:
"dependencies": {
"iview": "^3.2.1",
"vue-i18n": "^8.6.0",
"vue": "^2.5.2",
"vue-router": "^3.0.1"
}
And lanye_project_name/node_modules
will have vue-i18n
package:
Import iView globally:
lanye_project_name/src/i18n
:
Create en_US.json
:
{
"message": {
"password": "password"
},
"placeholder": {
"tips": "tips"
}
}
Create zh_CN.json
:
{
"message": {
"password": "密码"
},
"placeholder": {
"tips": "提示"
}
}
Create index.js
:
import VueI18n from 'vue-i18n'
import Vue from 'vue'
Vue.use(VueI18n)
var Messages = {
zh: require('./zh_CN.json'),
en: require('./en_US.json')
}
var i18n = new VueI18n({
locale: 'zh',
messages: Messages
})
export default i18n
lanye_project_name/src/main.js
import i18n from './i18n'
/* add i18n */
new Vue({
el: '#app',
router,
i18n,
components: { App },
template: '<App/>'
})
Complete code:
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import router from './router'
import iView from 'iview'
import 'iview/dist/styles/iview.css'
import i18n from './i18n'
Vue.config.productionTip = false
Vue.use(iView)
Vue.prototype.$axios = axios
/* eslint-disable no-new */
new Vue({
el: '#app',
router,
i18n,
components: { App },
template: '<App/>'
})
- Simple example:
<div>
<p>{{ $t("placeholder.tips") }}</p>
</div>
Input:
<Input type="text" v-model="formInline.userName" :placeholder="$t('form.userName')">
+ Axios
Install iView on your project:
$ cnpm install --save axios
If it works, lanye_project_name/package.json
- dependencies will add axios
:
"dependencies": {
"axios": "^0.18.0",
"iview": "^3.2.1",
"vue": "^2.5.2",
"vue-i18n": "^8.6.0",
"vue-router": "^3.0.1"
}
And lanye_project_name/node_modules
will have axios
package:
Import iView globally: lanye_project_name/src/main.js
:
import axios from 'axios'
Vue.prototype.$axios = axios
Complete code:
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import router from './router'
import iView from 'iview'
import 'iview/dist/styles/iview.css'
import axios from 'axios'
import i18n from './i18n'
Vue.config.productionTip = false
Vue.use(iView)
Vue.prototype.$axios = axios
/* eslint-disable no-new */
new Vue({
el: '#app',
router,
i18n,
components: { App },
template: '<App/>'
})
Simple usage:
//Send a `GET` request (default)
axios('/user/12345');
//Send a `POST`request
axios({
method:"POST",
url:'/user/12345',
data:{
firstName:"Fred",
lastName:"Flintstone"
}
});
Recommend an address that uses Axios in detail: https://www.jianshu.com/p/df464b26ae58.