Java语言跨平台原理
实现跨平台需要依赖Java的虚拟机 JVM (Java Virtual Machine)。java语言是跨平台的,java虚拟机不是跨平台的
JRE和JDK
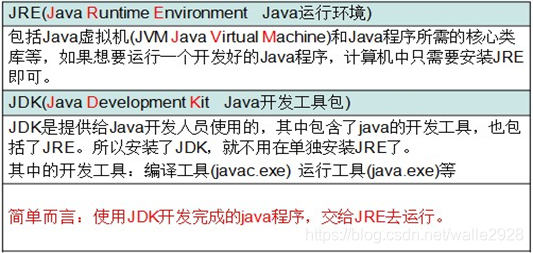
常量
常量类型 | 描述 |
---|
字符串常量 | 用双引号括起来的所有内容(“HelloWorld”) |
字符常量 | 用单引号括起来的一个字符(‘a’,’A’,’0’) |
整数常量 | 所有整数(12,-23) |
小数常量 | 所有小数(12.34) |
布尔常量 | 较为特殊,只有true和false |
空常量 | null |
基本数据类型(4类8种)
四类 | 八种 | 字节数 | 数据表示范围 |
---|
整型(精确) | byte | 1 | -128~127 0101 0111 |
| short | 2 | -32768~32767 |
| int(默认) | 4 | -2147483648~2147483647 |
| long | 8 | -263~263-1 |
浮点型(不精确) | float | 4 | -3.403E38~3.403E38 |
| double(默认) | 8 | -1.798E308~1.798E308 |
字符型 | char | 2 | 表示一个字符,如(‘a’,‘A’,‘0’,‘家’) |
布尔型 | boolean | 1 | 只有两个值true与false |
注意
1.整数默认是int类型,浮点数默认是double。
2.定义long类型数据的时候,要加L或者l,建议加L; 定义float类型数据的时候,要加F或者f,建议加F。
逻辑运算符基本使用
&(与),|(或),^(异或),!(非) ,&&,||
运算符 | 规则 |
---|
与:& | 有false则false |
或:| | 有true则true |
异或:^ | 相同则false,不同则true |
非:! | true则false,false则true |
+的多种用法
- 数字相加
- 字符相加: 用字符在计算机中底层存储对应的数据值来参与运算
- 字符串相加: 这里的+其实不是加法,而是字符串连接符;字符串和其他类型的数据做拼接,结果是字符串类型的。
switch表达式
- 表达式的取值:byte,short,int,char
- JDK5以后可以是枚举
- JDK7以后可以是String
随机数Random类
Random r = new Random();
int number = r.nextInt(10);
方法重载概述
在同一个类中,允许存在一个以上的同名方法,只要它们的参数个数或者参数类型不同即可。
方法重载特点
- 与返回值类型无关,只看方法名和参数列表
- 在调用时,虚拟机jvm通过参数列表的不同来区分同名方法
public static float sum(floata,floatb)
public static int sum(inta,intb,intc)
面试题:以下创建String对象有什么区别:
String str1 = new String("helloworld");
String str = "helloworld";
java.util.Scanner类
方法 | 作用 |
---|
public int nextInt() | 从键盘输入一个整数 |
public double nextDouble() | 从键盘输入一个double类型的数据 |
public String nextLine() | 从键盘获取一行的数据,如何从键盘输入一个字符串 |
java.lang.String类
构造方法 | 作用 |
---|
public String(String original) | 把字符串数据封装成字符串对象 |
public String(char[] value) | 把字符数组的数据封装成字符串对象 |
public String(char[] value,int offset,int count) | 把字符数组中的一部分数据封装成字符 |
判断功能方法 | 作用 |
---|
boolean equals(Object obj) | 比较字符串的内容是否相同 |
boolean equalsIgnoreCase(String str) | 比较字符串的内容是否相同,忽略大小写 |
boolean startsWith(String str) | 判断字符串对象是否以指定的str开头 |
boolean endsWith(String str) | 判断字符串对象是否以指定的str结尾 |
返回值 | 获取功能方法 | 作用 |
---|
int | length() | 获取字符串的长度,其实也就是字符个数 |
char | charAt(int index) | 获取指定索引处的字符 |
int | indexOf(String str) | 获取str在字符串对象中第一次出现的索引,没有返回-1 |
String | substring(int start) | 从start开始截取字符串到字符串结尾 |
String | substring(int start,int end) | 从start开始,到end结束截取字符串;包括start,不包括end |
返回值 | 转换功能方法 | 作用 |
---|
char[] | toCharArray() | 把字符串转换为字符数组 |
String | toLowerCase() | 把字符串转换为小写字符串 |
String | toUpperCase() | 把字符串转换为大写字符串 |
返回值 | 方法 | 作用 |
---|
String | trim() | 去除字符串两端空格 |
String[] | split(String str) | 按照指定符号分割字符串 |
java.lang.StringBuilder类
方法 | 作用 |
---|
public StringBuilder append(E e) | (任意类型):添加数据,并返回自身对象 |
public StringBuilder reverse() | 字符串反转 |
java.util.ArrayList集合 集合是用来存对象的
- 数组和集合的区别:
- 数组长度恒定 可以存储任意类型元素
- 集合长度可变 只能存储引用类型 如果存基本类型 需要存对应的引用类型
- 集合是可变的数组
- 添加元素
public boolean add(E e)
public E get(int index)
public int size()
public boolean remove(Object o)
public E remove(int index)
public E set(int index,E element)
java.io.FileWriter类
public FileWriter(String fileName)
public FileWriter(String fileName, boolean append)
void write(String str)
void write(String str,int index,int len)
void write(int ch)
void write(char[] chs)
void write(char[] chs,int index,int len)
- 路径:
- 相对路径:相对当前项目而言的,在项目的根目录下(a.txt)
- 绝对路径:以盘符开始的路径(d:\a.txt)
java.io.FileReader类
public FileReader(String fileName)
int read()
int read(char[] cbuf)
public class Demo01CopyFile {
public static void main(String[] args) throws IOException {
FileReader fr = new FileReader("Demo01FileWriter.java");
FileWriter fw = new FileWriter("Copy.java");
int ch;
while ((ch = fr.read()) != -1) {
fw.write(ch);
}
fw.close();
fr.close();
}
}
- 复制文本文件: 一次读写一个字符数组复制文本文件 推荐
public class Demo02CopyFile {
public static void main(String[] args) throws IOException {
FileReader fr = new FileReader("Demo01FileWriter.java");
FileWriter fw = new FileWriter("Copy.java");
char[] chs = new char[1024];
int len;
while ((len = fr.read(chs)) != -1) {
fw.write(chs, 0, len);
}
fw.close();
fr.close();
}
}
BufferedWriter和BufferedReader类
public BufferedWriter(FileWriter fw);
void newLine()
String readLine()
public class Demo02CopyFile {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("Demo01FileWriter.java"));
BufferedWriter bw = new BufferedWriter(new FileWriter("Copy.java"));
int ch;
while ((ch = br.read()) != -1) {
bw.write(ch);
}
char[] chs = new char[1024];
int len;
while ((len = br.read(chs)) != -1) {
bw.write(chs, 0, len);
}
bw.close();
br.close();
}
}
public class Demo04CopyFile {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("Demo01FileWriter.java"));
BufferedWriter bw = new BufferedWriter(new FileWriter("Copy.java"));
String line;
while((line=br.readLine())!=null) {
bw.write(line);
bw.newLine();
bw.flush();
}
bw.close();
br.close();
}
}