参考项目结构以及目录
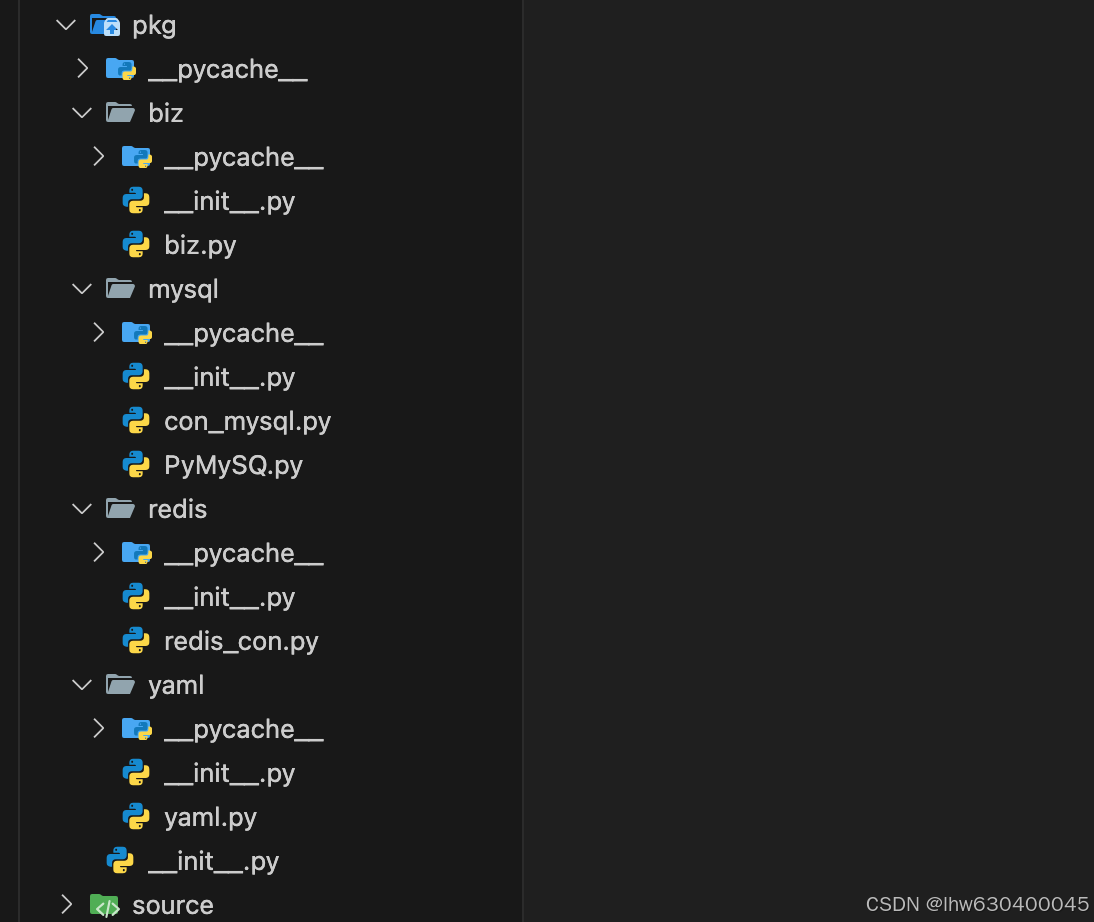
操作MySQL
import mysql.connector
from mysql.connector import pooling
from typing import List, Dict
import json
from datetime import datetime
"""
获取数据库链接
"""
def CreateMysqlPool(conf: Dict[str, str]) -> pooling.MySQLConnectionPool:
pool = None
try:
pool = pooling.MySQLConnectionPool(
pool_name="my-python-pool",
pool_size=5,
**conf
)
except mysql.connector.Error as err:
print(f"Error: {err}")
return pool
def GetMysqlConnect(pool: pooling.MySQLConnectionPool) -> mysql.connector.connection.MySQLConnection:
mydbConnect = pool.get_connection()
print('获取数据库链接信息:', mydbConnect)
return mydbConnect
def getDbInfos(pool: pooling.MySQLConnectionPool) -> List[Dict[str, any]]:
print("=========SHOW DATABASES==========")
connect = GetMysqlConnect(pool)
cursor = connect.cursor(dictionary=True)
result = []
try:
cursor.execute("SHOW DATABASES")
result = cursor.fetchall()
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
cursor.close()
connect.close()
return result
def ExecuteSQL(pool: pooling.MySQLConnectionPool, query: str) -> List[Dict[str, any]]:
connect = GetMysqlConnect(pool)
cursor = connect.cursor(dictionary=True)
result = []
try:
cursor.execute(query)
result = cursor.fetchall()
except mysql.connector.Error as err:
print(f"Error: {err}")
finally:
cursor.close()
connect.close()
return result
class DateTimeEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime):
return obj.isoformat()
return json.JSONEncoder.default(self, obj)
if __name__ == "__main__":
config = {
'host': 'localhost',
'user': 'root',
'password': 'rootroot',
'database': 'test',
'port': 3306,
'charset': 'utf8mb4',
'auth_plugin': 'mysql_native_password'
}
pool = CreateMysqlPool(config)
query = "SELECT * FROM enterprise_info limit 10"
result = ExecuteSQL(pool, query)
if result:
for row in result:
print(json.dumps(row, indent=4, ensure_ascii=False, cls=DateTimeEncoder))
result = getDbInfos(pool)
if result:
for row in result:
print(json.dumps(row, indent=4, ensure_ascii=False, cls=DateTimeEncoder))
import pymysql
from dbutils.pooled_db import PooledDB
import json
from typing import List, Dict
from datetime import datetime
"""
创建连接池
"""
def create_pool(config: Dict[str, str]) -> PooledDB:
pool = PooledDB(
creator=pymysql,
maxconnections=5,
**config
)
print('获取数据库链接池信息:', pool)
return pool
"""
从连接池中获取连接
"""
def GetMysqlConnect(pool: PooledDB):
connection = pool.connection()
print('获取数据库链接信息:', connection)
return connection
"""
获取数据库信息
"""
def getDbInfos(pool: PooledDB) -> List[Dict[str, any]]:
connection = GetMysqlConnect(pool)
cursor = connection.cursor(pymysql.cursors.DictCursor)
result = []
try:
print("=========SHOW DATABASES==========")
cursor.execute("SHOW DATABASES")
result = cursor.fetchall()
except pymysql.MySQLError as err:
print(f"Error: {err}")
finally:
cursor.close()
connection.close()
return result
"""
执行查询
"""
def execute_query(pool: PooledDB, query: str) -> List[Dict[str, any]]:
connection = GetMysqlConnect(pool)
cursor = connection.cursor(pymysql.cursors.DictCursor)
result = []
try:
cursor.execute(query)
result = cursor.fetchall()
except pymysql.MySQLError as err:
print(f"Error: {err}")
finally:
cursor.close()
connection.close()
return result
class DateTimeEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, datetime):
return obj.isoformat()
return json.JSONEncoder.default(self, obj)
if __name__ == "__main__":
config = {
'host': 'localhost',
'user': 'root',
'password': 'rootroot',
'database': 'test',
'port': 3306,
'charset': 'utf8mb4'
}
pool = create_pool(config)
connection = GetMysqlConnect(pool)
query = "SELECT * FROM enterprise_info limit 3"
result = execute_query(pool, query)
if result:
for row in result:
print(json.dumps(row, indent=4, ensure_ascii=False, cls=DateTimeEncoder))
result = getDbInfos(pool)
if result:
for row in result:
print(json.dumps(row, indent=4, ensure_ascii=False, cls=DateTimeEncoder))
操作redis
import redis
from redis.sentinel import Sentinel
from rediscluster import RedisCluster
import time
import json
from typing import Dict, List, Any, Union, Optional, Tuple, Type, TypeVar, Callable,MappingView, ItemsView, KeysView
"""
创建 Redis 连接池
"""
def CreateRedisPool(config: Dict[str, any]) -> redis.ConnectionPool:
try:
pool = redis.ConnectionPool(
host=config['host'],
port=int(config['port']),
db=config['db'],
password=config['password'],
decode_responses=config['decode_responses'],
max_connections=config['max_connections'],
socket_timeout=config['socket_timeout'],
socket_connect_timeout=config['socket_connect_timeout'],
retry_on_timeout=config['retry_on_timeout']
)
return pool
except Exception as e:
print(f"Error creating Redis connection pool: {e}")
raise
"""
创建哨兵连接池
"""
def create_sentinel_pool(config: Dict[str, any]) -> redis.StrictRedis:
try:
sentinel = Sentinel([(config['host'], config['port'])], socket_timeout=config['socket_timeout'])
r = sentinel.master_for(config['service_name'], socket_timeout=config['socket_timeout'], password=config['password'], decode_responses=config['decode_responses'])
return r
except Exception as e:
print(f"Error creating Redis sentinel connection: {e}")
raise
"""
创建集群连接池
"""
def create_cluster_pool(config: Dict[str, any]) -> RedisCluster:
try:
startup_nodes = [{"host": config['host'], "port": config['port']}]
r = RedisCluster(startup_nodes=startup_nodes, decode_responses=config['decode_responses'], password=config['password'])
return r
except Exception as e:
print(f"Error creating Redis cluster connection: {e}")
raise
"""
创建主从连接池
"""
def create_master_slave_pool(config: Dict[str, any]) -> redis.ConnectionPool:
try:
pool = redis.ConnectionPool(
host=config['host'],
port=config['port'],
db=config['db'],
password=config['password'],
decode_responses=config['decode_responses'],
max_connections=config['max_connections'],
socket_timeout=config['socket_timeout'],
socket_connect_timeout=config['socket_connect_timeout'],
retry_on_timeout=config['retry_on_timeout'],
)
return pool
except Exception as e:
print(f"Error creating Redis connection pool: {e}")
raise
"""
获取 Redis 连接
"""
def GetRedisConnection(pool: redis.ConnectionPool) -> redis.Redis:
try:
r = redis.Redis(connection_pool=pool)
print('获取 Redis 连接:', r)
return r
except Exception as e:
print(f"Error getting Redis connection: {e}")
raise
def example_operation(r: redis.Redis):
try:
r.set('foo', 'bar')
value = r.get('foo')
print('获取到的值:', value)
except Exception as e:
print(f"Error during Redis operation: {e}")
finally:
r.close()
"""
设置键值对,设置键值对,并指定 TTL 为 60 秒 setDict(r, key, value, ttl=60)
"""
def SetStrDict(r: redis.Redis, key: str, value: Dict, ttl: int = None):
try:
value_json = json.dumps(value)
if ttl is not None:
r.set(key, value_json, ex=ttl)
print(f"设置键值对: {key} -> {value_json},TTL: {ttl}")
else:
r.set(key, value_json)
print(f"设置键值对: {key} -> {value_json}")
except Exception as e:
print(f"Error setting key-value in Redis: {e}")
"""
设置键值对,设置键值对,并指定 TTL 为 60 秒 setDict(r, key, value, ttl=60)
"""
def SetStr(r: redis.Redis, key: str, value: str, ttl: int = None):
try:
if ttl is not None:
r.set(key, value, ex=ttl)
print(f"设置键值对: {key} -> {value},TTL: {ttl}")
else:
r.set(key, value)
print(f"设置键值对: {key} -> {value}")
except Exception as e:
print(f"Error setting key-value in Redis: {e}")
"""
获取键值对
"""
def GetStrDict(r: redis.Redis, key: str) -> Dict:
try:
value_json = r.get(key)
if value_json:
value = json.loads(value_json)
return value
else:
print(f"键 {key} 不存在")
return {}
except Exception as e:
print(f"Error getting key-value from Redis: {e}")
return {}
"""
获取键值对
"""
def GetStr(r: redis.Redis, key: str) -> str:
try:
value = r.get(key)
if value:
print(f"获取到的值: {key} -> {value}")
return value
else:
print(f"键 {key} 不存在")
return {}
except Exception as e:
print(f"Error getting key-value from Redis: {e}")
return {}
def KeyExists(r: redis.Redis, key: str) -> bool:
try:
exists = r.exists(key)
if exists:
print(f"键 {key} 存在")
else:
print(f"键 {key} 不存在")
return exists
except Exception as e:
print(f"Error checking if key exists in Redis: {e}")
return False
if __name__ == "__main__":
config = {
'host': 'localhost',
'port': 6380,
'db': 10,
'password': 'KYUIAPErZz2Xb0f8XIQOKfuv7dYURu',
'decode_responses': True,
'max_connections': 10,
'socket_timeout': 5,
'socket_connect_timeout': 5,
'retry_on_timeout': True,
'service_name': 'mymaster',
}
try:
pool = CreateRedisPool(config)
r = GetRedisConnection(pool)
example_operation(r)
r = GetRedisConnection(pool)
example_operation(r)
example_operation(r)
example_operation(r)
example_operation(r)
example_operation(r)
key = "my_key"
value = {"name": "Alice", "age": 30, "city": "New York"}
SetStrDict(r, key, value)
SetStrDict(r, key+"_ttl", value, ttl=60)
retrieved_value = GetStrDict(r, key)
print(f"获取到的值: {retrieved_value}")
SetStr(r, "Hello", "Hello, Redis!")
print( GetStr(r, "Hello") )
flag = KeyExists(r, "Hello")
if flag:
print("Key exists")
except Exception as e:
print(f"Error in main execution: {e}")
读取yaml
import yaml
def GetYaml(yaml_path: str) -> dict:
print('----GetYaml----')
with open(yaml_path, 'r') as file:
config = yaml.safe_load(file)
return config
主函数引用并启动服务
import sys
import os
import json
import requests
import json
import time
import random
import pandas as pd
from datetime import datetime,timezone
import yaml
import logging
import redis
sys.path.append(os.path.dirname(os.path.dirname(os.path.abspath(__file__))))
from pkg.mysql.con_mysql import CreateMysqlPool,GetMysqlConnect,ExecuteSQL
from pkg.redis.redis_con import CreateRedisPool,GetRedisConnection,SetStrDict,GetStrDict,KeyExists,SetStr,GetStr
from pkg.yaml.yaml import GetYaml
log_file_path = '/Users/wgg/Desktop/Python/logs/handler_all_data.log'
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(name)s - %(levelname)s - %(message)s',
handlers=[
logging.FileHandler(log_file_path),
logging.StreamHandler(sys.stdout)
]
)
logger = logging.getLogger(__name__)
"""
初始化redis连接池
"""
def initRedisPool(config : dict) -> redis.ConnectionPool:
try:
redis_pool = CreateRedisPool(config)
return redis_pool
except Exception as e:
logger.error(f"Error getting Redis connection: {e}")
raise
"""
业务处理忽略,定缓存成字典,放入redis
"""
def transform(config : dict, redis_pool :redis.ConnectionPool) -> None:
if __name__ == "__main__":
try:
config = GetYaml('/Users/wgg/Desktop/Pythonconfig/configV2.yaml')
logger.info("获取mysql:\n")
logger.info(json.dumps(config['mysql'], indent=4, ensure_ascii=False, sort_keys=False))
logger.info("获取redis:\n")
logger.info(json.dumps(config['redis'], indent=4, ensure_ascii=False, sort_keys=False))
transform(config=config, redis_pool=initRedisPool(config['redis']))
except Exception as e:
logger.error('异常信息:', exc_info=True)
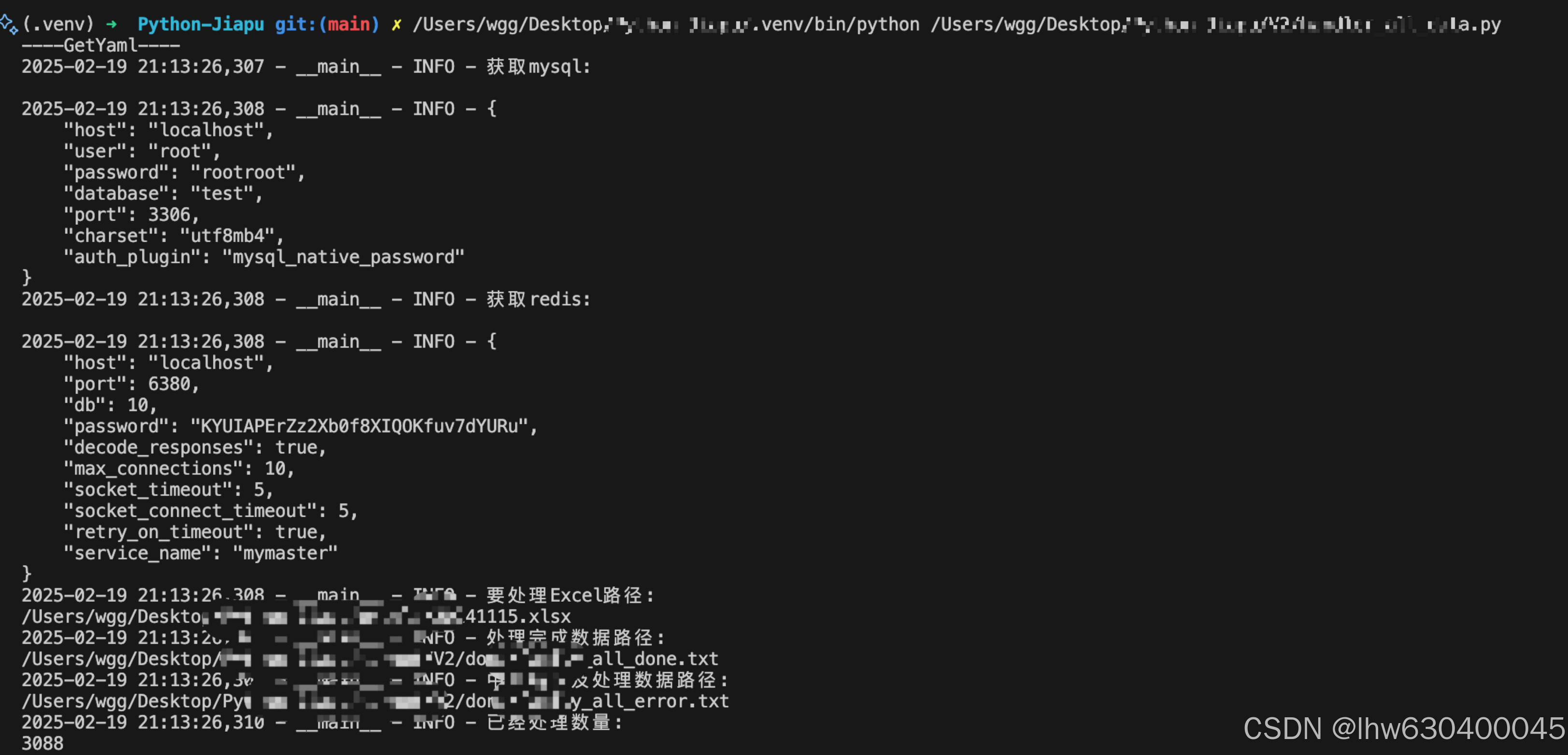
mysql:
host: 'localhost'
user: 'root'
password: 'rootroot'
database: 'test'
port: 3306
charset: 'utf8mb4'
auth_plugin: 'mysql_native_password'
redis:
host: 'localhost'
port: 6380
db: 10
password: 'KYUIAPErZz2Xb0f8XIQOKfuv7dYURu'
decode_responses: True
max_connections: 10
socket_timeout: 5
socket_connect_timeout: 5
retry_on_timeout: True
service_name: 'mymaster'