#include <iostream>
#include <vector>
using namespace std;
#include <afx.h>
#include <windows.h>
bool IsStringEndWithPostfix(wstring DirName, wstring PostFix)
{
int str_len1 = DirName.size();
int str_len2 = PostFix.size();
int pos = DirName.rfind(PostFix);
bool Valid = false;
if ((pos >= 0) && (pos == str_len1 - str_len2))
{
Valid = true;
}
return Valid;
}
void SearchFilesInDir(wstring DirName, wstring PostFix, vector<wstring> & FileNameList)
{
std::wstring pattern(DirName);
pattern.append(L"\\*");
WIN32_FIND_DATA data;
HANDLE hFind;
vector<wstring> FolderNameList;
if ((hFind = FindFirstFile(pattern.c_str(), &data)) != INVALID_HANDLE_VALUE) {
do {
bool valid0 = !IsStringEndWithPostfix(data.cFileName, L".");
if (valid0)
{
if (data.dwFileAttributes == FILE_ATTRIBUTE_DIRECTORY)
{
// is folder
FolderNameList.push_back(DirName + L"\\" + data.cFileName);
}
else
{
// is file
bool valid1 = IsStringEndWithPostfix(data.cFileName, PostFix);
bool valid2 = IsStringEndWithPostfix(data.cFileName, L".tif");
if (valid1 && valid2)
{
FileNameList.push_back(DirName + L"\\" + data.cFileName);
}
// wprintf(L"search file:%s\n", data.cFileName);
}
}
} while (FindNextFile(hFind, &data) != 0);
FindClose(hFind);
}
// search sub-folders
for (int i = 0; i < FolderNameList.size(); i++)
{
SearchFilesInDir(FolderNameList[i], PostFix, FileNameList);
}
}
int main()
{
wchar_t* str = L"G:\\test images";
wstring DirName = str;
wstring PostFix = L".tif";
vector<wstring> FileNameList;
SearchFilesInDir(DirName, PostFix, FileNameList);
int FileNumber = FileNameList.size();
printf("file number:%d\n", FileNumber);
CString CurStr;
for (int cnt = 0; cnt < FileNumber; cnt++)
{
CurStr = FileNameList[cnt].c_str();
wprintf(L"filename: %s\n", CurStr);
}
return 0;
}
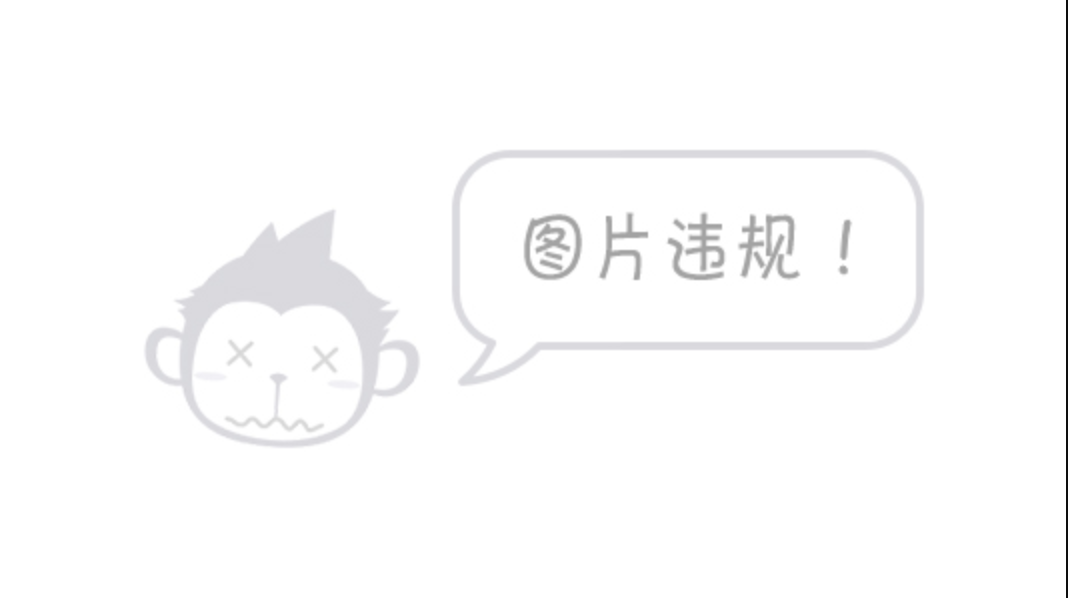
参考了部分网上的代码
linux系统下文件搜索可以用popen等方式通过调用shell命令来实现。