基于Spring + CXF框架的Web Service
1、在之前的基础上修改的,如果想使用JQuery、Ajax来调用WebService服务,必须需要先获取到请求的参数值,我的参数值是下面的这部分,如何获取到这参数值呢,只需要在客户端配置出拦截器即可,后台打印的参数,然后配置到自己需要的地方即可。
1 <soap:Envelope xmlns:soap='http://schemas.xmlsoap.org/soap/envelope/'><soap:Header><zhangsansan><name>张姗姗</name><password>123456</password></zhangsansan></soap:Header><soap:Body><ns2:getOrderById xmlns:ns2='http://ws.webservice.bie.com/'><arg0>1</arg0></ns2:getOrderById></soap:Body></soap:Envelope>
下面配置服务器端的beans.xml配置,这里还是使用账号密码验证服务,新增了出拦截器配置,其他还是使用上篇的类和配置。
1 <?xml version="1.0" encoding="UTF-8"?>
2 <beans xmlns="http://www.springframework.org/schema/beans"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xmlns:jaxws="http://cxf.apache.org/jaxws"
5 xsi:schemaLocation="http://www.springframework.org/schema/beans
6 http://www.springframework.org/schema/beans/spring-beans.xsd
7 http://cxf.apache.org/jaxws http://cxf.apache.org/jaxws">
8
9 <!-- 引cxf-2.5.9.jar此包下面的配置,这些配置不在此项目中,cxf的一些核心配置 -->
10 <import resource="classpath:META-INF/cxf/cxf.xml" />
11 <import resource="classpath:META-INF/cxf/cxf-extension-soap.xml" />
12 <import resource="classpath:META-INF/cxf/cxf-servlet.xml" />
13
14 <!--
15 jaxws:endpoint标签配置终端。
16 implementor是实现类。
17 address是虚拟地址。
18 -->
19 <jaxws:endpoint id="orderWS"
20 implementor="com.bie.webservice.ws.impl.OrderWSImpl"
21 address="/orderws">
22 <!-- 服务器端配置入拦截器 -->
23 <jaxws:inInterceptors>
24 <!-- 配置自定义的拦截器,注入到ioc容器中 -->
25 <bean class="com.bie.webservice.interceptor.CheckUserInterceptor"></bean>
26 </jaxws:inInterceptors>
27 <!-- 服务器端日志出拦截器 -->
28 <jaxws:outInterceptors>
29 <bean class="org.apache.cxf.interceptor.LoggingOutInterceptor" />
30 </jaxws:outInterceptors>
31 </jaxws:endpoint>
32
33 </beans>
下面配置客户端的client-beans.xml配置,这里还是使用账号密码验证服务,新增了入拦截器配置,其他还是使用上篇的类和配置。
1 <?xml version="1.0" encoding="UTF-8"?>
2 <beans xmlns="http://www.springframework.org/schema/beans"
3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
4 xmlns:jaxws="http://cxf.apache.org/jaxws"
5 xsi:schemaLocation="http://www.springframework.org/schema/beans
6 http://www.springframework.org/schema/beans/spring-beans.xsd
7 http://cxf.apache.org/jaxws http://cxf.apache.org/jaxws">
8
9 <!--
10 客户端配置:
11 serviceClass根据此类动态产生接口的代理对象。
12 address是指的是webservice请求的地址。
13 -->
14 <jaxws:client id="orderClient"
15 serviceClass="com.bie.webservice.ws.OrderWS"
16 address="http://localhost:8080/webServiceCxf_Spring/orderws">
17
18 <!-- 客户端配置出拦截器类 -->
19 <jaxws:outInterceptors>
20 <!-- 客户端出拦截器 -->
21 <bean class="org.apache.cxf.interceptor.LoggingOutInterceptor" />
22 <bean
23 class="com.bie.webservice.ws.interceptor.ClientValidateUserInterceptor">
24 <!-- 通过构造参数传递给客户端拦截器类 -->
25 <constructor-arg name="name" value="张姗姗" />
26 <constructor-arg name="password" value="123456" />
27 </bean>
28 </jaxws:outInterceptors>
29 <!-- 客户端入拦截器 -->
30 <jaxws:inInterceptors>
31 <bean class="org.apache.cxf.interceptor.LoggingInInterceptor" />
32 </jaxws:inInterceptors>
33 </jaxws:client>
34
35 </beans>
2、然后搞一个index.jsp类,引用jquery,bootstrap样式这些东西,如下所示:
1 <%@ page language="java" contentType="text/html; charset=utf-8"
2 pageEncoding="utf-8"%>
3 <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
4 <html>
5 <head>
6 <meta http-equiv="Content-Type" content="text/html; charset=utf-8">
7 <title>搞什么???</title>
8 <link rel="stylesheet" href="bootstrap.min.css">
9
10 <script src="jquery-1.7.2.js"></script>
11 <script src="bootstrap.min.js"></script>
12
13 <script type="text/javascript">
14
15 $(function(){
16
17 // HttpURLConnection请求webservice
18 $("#btn2").click(function(){
19 var name = document.getElementById("name").value;
20 var password = document.getElementById("password").value;
21 $.post(
22 "HttpURLConnectionServlet",
23 {
24 "name":name,
25 "password":password
26 },
27 function(msg) {
28 // console.log(msg);
29 var $Result = $(msg);
30 var value = $Result.find("return").text();
31 console.log(value);
32 },
33 "xml"
34 );
35 });
36
37 // Jquery请求webservice
38 $("#btn").click(function(){ //回调函数
39 var name = document.getElementById("name").value;
40 var password = document.getElementById("password").value;
41 // var data = '<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><soap:Body><ns2:sayHello xmlns:ns2="http://sei.webservice.bie.com/"><arg0>'+name+'</arg0></ns2:sayHello></soap:Body></soap:Envelope>';
42 var data = '<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><soap:Header><zhangsansan><name>'+name+'</name><password>'+password+'</password></zhangsansan></soap:Header><soap:Body><ns2:getOrderById xmlns:ns2="http://ws.webservice.bie.com/"><arg0>1</arg0></ns2:getOrderById></soap:Body></soap:Envelope>';
43 console.log(data);
44 $.ajax({
45 type : "post",
46 url : "http://localhost:8080/webServiceCxf_Spring/orderws",
47 data : data,
48 success : function(msg){
49 console.log("------");
50 var $Result = $(msg);
51 var value = $Result.find("return").text();
52 console.log(value);
53 },
54 error : function(msg) {
55 console.log("-----" + msg);
56 },
57 dataType : "xml"
58 });
59 });
60 });
61
62
63 // AJax请求webservice
64 function reqWebService() {
65 // 获取到name属性的值
66 var name = document.getElementById("name").value;
67 var password = document.getElementById("password").value;
68 // 并将传递的name属性值拼接到该data字符串中
69 // var data = '<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><soap:Body><ns2:sayHello xmlns:ns2="http://sei.webservice.bie.com/"><arg0>'+name+'</arg0></ns2:sayHello></soap:Body></soap:Envelope>';
70 var data = '<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"><soap:Header><zhangsansan><name>'+name+'</name><password>'+password+'</password></zhangsansan></soap:Header><soap:Body><ns2:getOrderById xmlns:ns2="http://ws.webservice.bie.com/"><arg0>1</arg0></ns2:getOrderById></soap:Body></soap:Envelope>';
71 // 创建XMLHttpRequest对象
72 var request = getRequest();
73 // 响应返回的数据,设置回调函数
74 request.onreadystatechange = function(){
75 if(request.readyState==4 && request.status==200) {
76 var result = request.responseXML;
77 console.log(result.all[0]);
78 result = result.all[0]
79 var returnEle = result.getElementsByTagName("return")[0];
80 console.log(returnEle);
81
82 var firstNodeName = returnEle.firstChild.nodeName;
83 console.log(firstNodeName);
84 var firstNodeNameVlaue = returnEle.firstChild.innerHTML;
85 console.log(firstNodeNameVlaue);
86
87 var secondNodeName = returnEle.firstChild.nextElementSibling.nodeName;
88 console.log(secondNodeName);
89 var secondNodeVlaue = returnEle.firstChild.nextElementSibling.innerHTML;
90 console.log(secondNodeVlaue);
91
92 var thirdNodeName = returnEle.firstChild.nextElementSibling.nextElementSibling.nodeName;
93 console.log(thirdNodeName);
94 var thirdNodeVlaue = returnEle.firstChild.nextElementSibling.nextElementSibling.innerHTML;
95 console.log(thirdNodeVlaue);
96 }
97 };
98
99 // 请求地址,打开连接
100 request.open("POST", "http://localhost:8080/webServiceCxf_Spring/orderws");
101 // 如果是POST请求,要包含一个请求头,设置字符集编码,不然后台获取到的是乱码的,设置一个请求头
102 request.setRequestHeader("Content-type", "application/x-www-form-urlencoded;charset=utf-8");
103 // 发送请求体,发送请求,将data作为请求体发送过去
104 request.send(data);
105 }
106
107 // XMLHttpRequest对象,使用各个浏览器之间的操作
108 function getRequest() {
109 var xmlHttp = null;
110 try {
111 // Firefox, Opera 8.0+, Safari chrome
112 xmlHttp = new XMLHttpRequest();
113 } catch (e) {
114 // Internet Explorer
115 try {
116 xmlHttp = new ActiveXObject("Msxml2.XMLHTTP");
117 } catch (e) {
118 xmlHttp = new ActiveXObject("Microsoft.XMLHTTP");
119 }
120 }
121 return xmlHttp;
122 }
123 </script>
124 </head>
125 <body>
126
127
128
129 <center>
130
131 <h1></h1>
132 <div class="row">
133 <div class="col-md-3"></div>
134 <div class="col-md-3">
135 账号:<input type="text" class="form-control" id="name" name="name" value="" /> <br/>
136 </div>
137 <div class="col-md-3">
138 密码:<input type="text" class="form-control" id="password" name="password" value="" />
139 </div>
140 <div class="col-md-3"></div>
141 </div>
142
143 <br><br/>
144 <button onclick="reqWebService()" class="btn btn-primary">AJax请求webservice</button>
145 <button id="btn" class="btn btn-success">Jquery请求webservice</button>
146 <button id="btn2" class="btn btn-danger">HttpURLConnection请求webservice</button>
147 </center>
148 </body>
149 </html>
这里使用了三种方式,分别是AJax请求webservice、Jquery请求webservice、HttpURLConnection请求webservice,所以搞一个HttpURLConnection的servlet来接收请求,如下所示:
1 package com.bie.webservice.ws.servlet;
2
3 import java.io.IOException;
4 import java.io.InputStream;
5 import java.io.OutputStream;
6 import java.net.HttpURLConnection;
7 import java.net.URL;
8
9 import javax.servlet.ServletException;
10 import javax.servlet.ServletOutputStream;
11 import javax.servlet.http.HttpServlet;
12 import javax.servlet.http.HttpServletRequest;
13 import javax.servlet.http.HttpServletResponse;
14
15 /**
16 *
17 * @author
18 *
19 */
20 public class HttpURLConnectionServlet extends HttpServlet {
21
22 private static final long serialVersionUID = 1L;
23
24 protected void doPost(HttpServletRequest request, HttpServletResponse response)
25 throws ServletException, IOException {
26 // 获取到账号密码的值
27 String name = request.getParameter("name");
28 String password = request.getParameter("password");
29 System.out.println("doPost " + name + ", " + password);
30
31 // String data = "<soap:Envelope xmlns:soap='http://schemas.xmlsoap.org/soap/envelope/'>"
32 // + "<soap:Body><ns2:sayHello xmlns:ns2='http://sei.webservice.bie.com/'><arg0>" + name
33 // + "</arg0></ns2:sayHello></soap:Body></soap:Envelope>";
34
35 // 组织数据,将数据拼接一下
36 String data = "<soap:Envelope xmlns:soap='http://schemas.xmlsoap.org/soap/envelope/'>"
37 + "<soap:Header><zhangsansan><name>" + name + "</name><password>" + password + "</password>"
38 + "</zhangsansan></soap:Header><soap:Body>"
39 + "<ns2:getOrderById xmlns:ns2='http://ws.webservice.bie.com/'>"
40 + "<arg0>1</arg0></ns2:getOrderById></soap:Body></soap:Envelope>";
41
42 // 第一步:创建服务地址,不是WSDL地址
43 URL url = new URL("http://localhost:8080/webServiceCxf_Spring/orderws");
44
45 // 第二步:打开一个通向服务地址的连接
46 HttpURLConnection connection = (HttpURLConnection) url.openConnection();
47
48 // 第三步:设置参数
49 // 发送方式设置:POST必须大写
50 connection.setRequestMethod("POST");
51 // 设置输入输出,因为默认新创建的connection没有读写权限
52 connection.setDoOutput(true);
53 connection.setDoInput(true);
54 // 设置数据格式:content-type
55 connection.setRequestProperty("Content-Type", "text/xml;charset=utf-8");
56
57 // 第四步:组织SOAP数据,发送请求
58 OutputStream os = connection.getOutputStream();
59 os.write(data.getBytes("utf-8"));
60
61 // 第五步:接收服务端响应,打印
62 int responseCode = connection.getResponseCode();
63 // 表示服务端响应成功
64 if (responseCode == 200) {
65 // String xml
66 InputStream is = connection.getInputStream();
67 System.out.println("return " + is.available());
68 // 将字节流转换为字符流
69 response.setContentType("text/xml;charset=utf-8");
70 ServletOutputStream outputStream = response.getOutputStream();
71
72 byte[] buffer = new byte[1024];
73 int len = 0;
74 while ((len = is.read(buffer)) > 0) {
75 outputStream.write(buffer, 0, len);
76 }
77 outputStream.flush();
78 }
79 }
80
81 }
需要注意的是,客户端需要在web.xml配置一下请求地址和转发的类,或者搞一个Servlet注解也可以,如下所示:
1 <?xml version="1.0" encoding="UTF-8"?>
2 <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
3 xmlns="http://java.sun.com/xml/ns/javaee"
4 xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
5 http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
6 id="WebApp_ID" version="2.5">
7 <display-name>webServiceCxf_Spring_Client</display-name>
8 <welcome-file-list>
9 <welcome-file>index.html</welcome-file>
10 <welcome-file>index.jsp</welcome-file>
11 </welcome-file-list>
12
13 <servlet>
14 <description></description>
15 <display-name>HttpURLConnectionServlet</display-name>
16 <servlet-name>HttpURLConnectionServlet</servlet-name>
17 <servlet-class>com.bie.webservice.ws.servlet.HttpURLConnectionServlet</servlet-class>
18 </servlet>
19 <servlet-mapping>
20 <servlet-name>HttpURLConnectionServlet</servlet-name>
21 <url-pattern>/HttpURLConnectionServlet</url-pattern>
22 </servlet-mapping>
23
24
25 </web-app>
演示效果,如下所示:
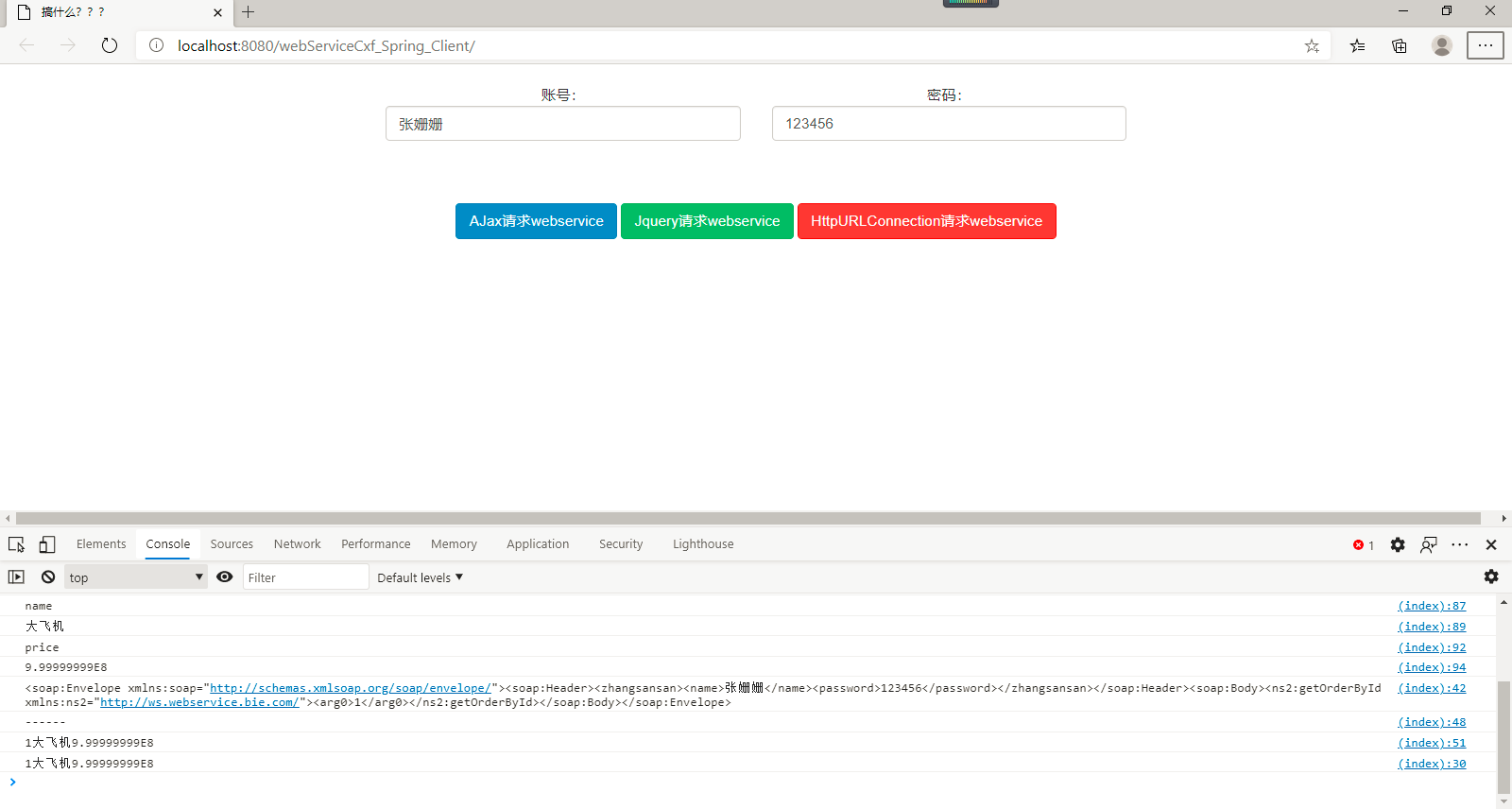