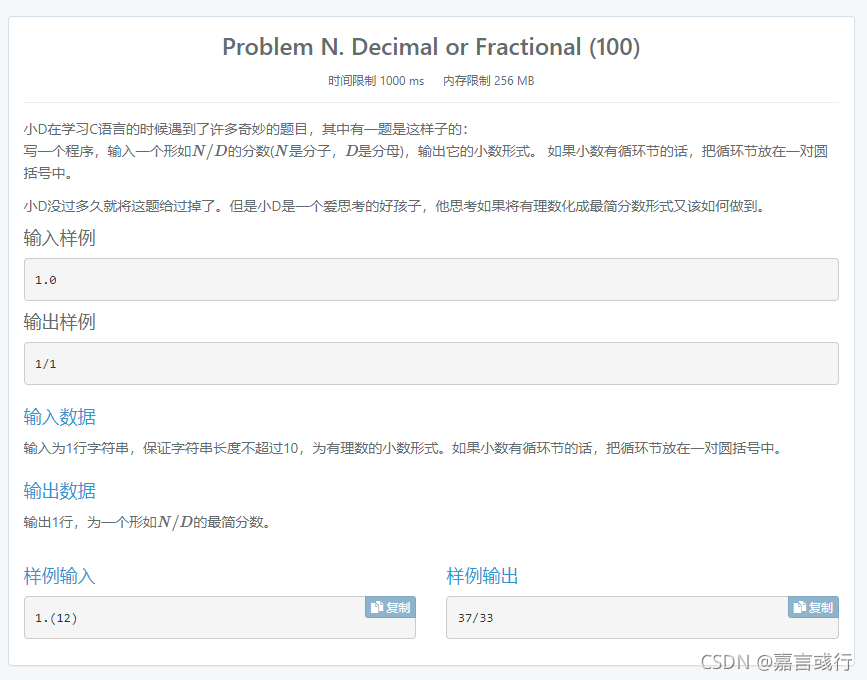
import java.util.Scanner;
public class GCD {
static long gcd(long a,long b) {
if(b==0)
return a;
if(a<0)
return gcd(-a,b);
if(b<0)
return gcd(a,-b);
return gcd(b,a%b);
}
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
String str = sc.next();
String [] arr = str.split("\\.");
String intStr = arr[0];
String decimalStr = arr[1];
int integer = 0;
int noloop = 0;
int loop = 0;
int n =0;
int m =0;
boolean flag = true;
for (int i =0 ;i<intStr.length();i++){
integer =integer*10+ intStr.charAt(i)-'0';
}
for (int i = 0;i < decimalStr.length();i++){
if (flag && decimalStr.charAt(i)!= '('&&decimalStr.charAt(i)!= ')'){
noloop = noloop*10+ decimalStr.charAt(i)-'0';
n++;
}
if(decimalStr.charAt(i)== '('){
flag = false;
continue;
}
if (flag == false&& decimalStr.charAt(i)!= '('&&decimalStr.charAt(i)!= ')'){
loop =loop*10+decimalStr.charAt(i)-'0';
m++;
}
}
if (m ==0){
long top = (long)(noloop+integer*Math.pow(10,n));
long bottom =(long) Math.pow(10,n);
long gcdNum= gcd(top,bottom);
if (gcdNum == 0){
gcdNum =1;
}
top = top/gcdNum;
bottom =bottom/gcdNum;
System.out.println(top+"/"+bottom);
}else {
long top = (long)(noloop*(Math.pow(10,m)-1)+loop+integer*(Math.pow(10,m)-1)*Math.pow(10,n));
long botoom = (long) ((Math.pow(10,m)-1)*Math.pow(10,n));
long gcdNum= gcd(top,botoom);
if (gcdNum == 0){
gcdNum =1;
}
top = top/gcdNum;
botoom =botoom/gcdNum;
System.out.println(top+"/"+botoom);
}
}
}