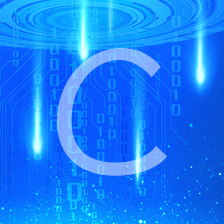
Python
DamonHao
明光村某附属大学
展开
-
Three special statements: pass, del, and exec
1)Nothing Happened by pass Sometimes you need to do nothing. This may not be very often, but when it happens, it's good to know that you have the pass statement:>>> pass>>>another example:原创 2013-05-26 08:14:03 · 1201 阅读 · 0 评论 -
About Exception
1) rise exceptionTo raise an exception, you use the raise statement with an argument that is either a class (which should subclass Exception for the "catch clause") or an instance.>>> raise Ex原创 2013-05-31 22:54:12 · 826 阅读 · 0 评论 -
About Constructor
Consrtuctor is one of the so called "magic methods" in python. What separates constructors from ordinary methods, is that the constructors are called automatically right after an object has been cre原创 2013-06-02 21:55:22 · 983 阅读 · 0 评论 -
Three Standard Streams: stdin, stdout, stderr
First, you shoud keep in mind that standard input/output is one of the best way to check bug. Because the data they offer is raw.These are actually files (or file-like objects), and you can apply原创 2013-06-14 22:47:06 · 799 阅读 · 0 评论 -
About Generator
Generators are relatively new to Python, and are (along with iterators) perhaps one of the most powerful features to come along for years.The following example is a function that flattens nested l原创 2013-06-14 21:45:48 · 919 阅读 · 0 评论 -
The Property Function
First, consider the following Rectangle class:class Rectangle: def __init__(self): self.width = 0 self.height = 0 def setSize(self, size): self.width, self.原创 2013-06-14 21:58:49 · 790 阅读 · 0 评论 -
The With Statement
The with statement is actually a quite general construct, allowing you to use so-called context managers. A context manager is an object that supports two methods: __enter__ and __exit__. Like:c原创 2013-06-14 22:53:56 · 774 阅读 · 0 评论 -
Multiple inheritance
class Calculator: def calculate(self, expression): self.value = eval(expression)class Talker: def talk(self): print 'Hi, my value is', self.valueclass TalkingCalculat原创 2013-05-31 22:47:47 · 855 阅读 · 0 评论 -
Some function to handle class and its interface info
1) get some class infothe following example show basic inheritanceclass Filter: def init(self): self.blocked = [] def filter(self, sequence): return [x for x in s原创 2013-05-31 22:41:13 · 823 阅读 · 0 评论 -
The Meaning of Class Definitions
we have to keep in mind that the meaning of class definitions are simply code sections that are executed !>>>class C: print 'Class C being defined...'Class C being defined...>>>class Me原创 2013-05-31 22:17:10 · 883 阅读 · 0 评论 -
About Class
1) method and functionOne big difference between methods and other functions is that methods always receive the object they are part of as their first argument, usually called self.>>> class Class原创 2013-05-31 21:55:48 · 854 阅读 · 0 评论 -
Parameters
1)positional,keyword and default parameterFor positional parameters,values are assigned to different parameters according to their positions; def hello_1(greeting, name): print '%s, %s!' %原创 2013-05-26 09:23:05 · 1022 阅读 · 0 评论 -
Scope
The problem of shadowingReading the value of global variables is not a problem in general, but one thing may make it problematic. If a local variable or parameter exists with the same name as the gl原创 2013-05-26 08:32:10 · 749 阅读 · 0 评论 -
Some iteration utilities
Python has several functions that can be useful when iterating over a sequence (or other iterable object). Some of these are available in the itertools module , but there are some built-in functions原创 2013-05-25 22:38:33 · 862 阅读 · 0 评论 -
About Modules
First you must keep in mind that modules are used to define things and reuse code.1) the .pyc fileWhen you import a module, you may notice that a new file appears, like c:\python\hello.p原创 2013-06-14 22:21:00 · 822 阅读 · 0 评论