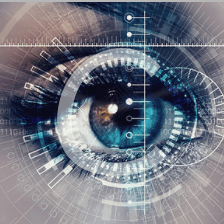
LeetCode
薛离子
假如我年少有为不自卑
展开
-
141. Linked List Cycle
Given a linked list, determine if it has a cycle in it.Follow up: Can you solve it without using extra space?解题思路:最好的方法是时间复杂度 O(n),空间复杂度 O(1) 的。设置两个指针,一个快一个慢,快 的指针每次走两步,慢的指针每次走一步,如果快指针和慢指针相遇,则说明有环。/**原创 2017-01-09 22:40:53 · 257 阅读 · 0 评论 -
LeetCode 12. Integer to Roman
描述 Given an integer, convert it to a roman numeral.Input is guaranteed to be within the range from 1 to 3999.分析 整数转换为罗马数字。 此题因为题目中明确了范围1到3999,所以用每位对应一个数组的方式较为简单。附罗马数字的规则:罗马数字共有7个,即I(1)、V(5)、X(10)、L(原创 2017-02-05 22:20:34 · 327 阅读 · 0 评论 -
LeetCode 67. Add Binary
描述 Given two binary strings, return their sum (also a binary string).For example, a = “11” b = “1” Return “100”.分析 翻转a和b,逐位相加求值,结果存入string中。 注意最高位的情况。代码class Solution {public: string addBina原创 2017-02-05 22:38:03 · 284 阅读 · 0 评论 -
LeetCode 58. Length of Last Word
描述 Given a string s consists of upper/lower-case alphabets and empty space characters ’ ‘, return the length of last word in the string.If the last word does not exist, return 0.Note: A word is define原创 2017-02-05 22:54:47 · 319 阅读 · 0 评论 -
LeetCode 344. Reverse String
描述 Write a function that takes a string as input and returns the string reversed.Example: Given s = “hello”, return “olleh”.分析 翻转字符串。 取字符串长度的一半进行循环,首尾交换。代码class Solution {public: string revers原创 2017-02-05 23:04:01 · 307 阅读 · 0 评论 -
25. Reverse Nodes in k-Group
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.k is a positive integer and is less than or equal to the length of the linked list. If the number of nod原创 2017-01-15 16:38:34 · 275 阅读 · 0 评论 -
92. Reverse Linked List II
Reverse a linked list from position m to n. Do it in-place and in one-pass.For example: Given 1->2->3->4->5->NULL, m = 2 and n = 4,return 1->4->3->2->5->NULL.Note: Given m, n satisfy the following co原创 2017-01-15 17:10:00 · 233 阅读 · 0 评论 -
109. Convert Sorted List to Binary Search Tree
Given a singly linked list where elements are sorted in ascending order, convert it to a height balanced BST./** * Definition for singly-linked list. * struct ListNode { * int val; * ListNo原创 2017-01-15 17:51:53 · 228 阅读 · 0 评论 -
142. Linked List Cycle II
Given a linked list, return the node where the cycle begins. If there is no cycle, return null.Note: Do not modify the linked list.Follow up: Can you solve it without using extra space?解题思路:当 fast 与 s原创 2017-01-15 18:06:25 · 254 阅读 · 0 评论 -
143. Reorder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln, reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→…You must do this in-place without altering the nodes’ values.For example, Given {1,2,3,4}, reorder it to {1,4,2,3}原创 2017-01-15 18:43:43 · 247 阅读 · 0 评论 -
234. Palindrome Linked List
Given a singly linked list, determine if it is a palindrome.Follow up: Could you do it in O(n) time and O(1) space?分析: 反转链表法,将链表后半段原地翻转,再将前半段、后半段依次比较,判断是否相等,时间复杂度O(n),空间复杂度为O(1)满足题目要求。/** * Definit原创 2017-01-15 19:26:19 · 223 阅读 · 0 评论 -
LeetCode 13. Roman to Integer
描述 Given a roman numeral, convert it to an integer.Input is guaranteed to be within the range from 1 to 3999.分析 罗马数字转换为整数。附罗马数字的规则:罗马数字共有7个,即I(1)、V(5)、X(10)、L(50)、C(100)、D(500)和M(1000)。 1、重复数次:一个罗马数原创 2017-02-05 22:07:43 · 309 阅读 · 0 评论 -
LeetCode 125. Valid Palindrome
描述 Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases.For example, “A man, a plan, a canal: Panama” is a palindrome. “race a car” is not a p原创 2017-02-05 19:28:44 · 275 阅读 · 0 评论 -
86. Partition List
Given a linked list and a value x, partition it such that all nodes less than x come before nodes greater than or equal to x.You should preserve the original relative order of the nodes in each of the原创 2017-01-09 22:51:28 · 285 阅读 · 0 评论 -
237. Delete Node in a Linked List
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node.Supposed the linked list is 1 -> 2 -> 3 -> 4 and you are given the third node with value 3, t原创 2017-01-09 22:58:52 · 260 阅读 · 0 评论 -
328. Odd Even Linked List
Given a singly linked list, group all odd nodes together followed by the even nodes. Please note here we are talking about the node number and not the value in the nodes.You should try to do it in plac原创 2017-01-09 23:10:53 · 392 阅读 · 0 评论 -
203. Remove Linked List Elements
Remove all elements from a linked list of integers that have value val.Example Given: 1 –> 2 –> 6 –> 3 –> 4 –> 5 –> 6, val = 6 Return: 1 –> 2 –> 3 –> 4 –> 5Credits: Special thanks to @mithmatt for a原创 2017-01-10 00:03:55 · 260 阅读 · 0 评论 -
82. Remove Duplicates from Sorted List II
Given a sorted linked list, delete all nodes that have duplicate numbers, leaving only distinct numbers from the original list.For example, Given 1->2->3->3->4->4->5, return 1->2->5. Given 1->1->1->2原创 2017-01-14 10:34:38 · 306 阅读 · 0 评论 -
23. Merge k Sorted Lists
Merge k sorted linked lists and return it as one sorted list. Analyze and describe its complexity.解题思路:复用21. Merge Two Sorted Lists的代码,链表两两合并,直到最后只剩一个链表为止。/** * Definition for singly-linked list. * s原创 2017-01-14 11:00:51 · 380 阅读 · 0 评论 -
LeetCode 31. Next Permutation
描述 Implement next permutation, which rearranges numbers into the lexicographically next greater permutation of numbers.If such arrangement is not possible, it must rearrange it as the lowest possible原创 2017-02-05 16:42:36 · 686 阅读 · 0 评论 -
LeetCode 46. Permutations
描述 Given a collection of distinct numbers, return all possible permutations.For example, [1,2,3] have the following permutations:[ [1,2,3], [1,3,2], [2,1,3], [2,3,1], [3,1,2], [3,2,1]]分析原创 2017-02-05 17:29:53 · 321 阅读 · 0 评论 -
LeetCode 60. Permutation Sequence
描述 The set [1,2,3,…,n] contains a total of n! unique permutations.By listing and labeling all of the permutations in order, We get the following sequence (ie, for n = 3):“123” “132” “213” “231” “原创 2017-02-05 17:55:56 · 331 阅读 · 0 评论 -
LeetCode 48. Rotate Image
描述 You are given an n x n 2D matrix representing an image.Rotate the image by 90 degrees (clockwise).Follow up: Could you do this in-place?分析 首先想到,纯模拟,从外到内一圈一圈的转,但这个方法太慢。 如下图,首先沿着副对角线翻转一次,然后沿着水平中原创 2017-02-05 18:24:51 · 297 阅读 · 0 评论 -
66. Plus One
Given a non-negative integer represented as a non-empty array of digits, plus one to the integer.You may assume the integer do not contain any leading zero, except the number 0 itself.The digits are st原创 2017-01-10 23:51:53 · 312 阅读 · 0 评论 -
LeetCode 16. 3Sum Closest
描述 Given an array S of n integers, find three integers in S such that the sum is closest to a given number, target. Return the sum of the three integers. You may assume that each input would have exac原创 2017-02-06 21:05:32 · 304 阅读 · 0 评论 -
160. Intersection of Two Linked Lists
Write a program to find the node at which the intersection of two singly linked lists begins.For example, the following two linked lists:A: a1 → a2 ↘ c1原创 2017-01-15 21:36:15 · 234 阅读 · 0 评论 -
206. Reverse Linked List
Reverse a singly linked list.解题思路:利用头节点,头插法。/** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; *原创 2017-01-09 00:04:16 · 202 阅读 · 0 评论 -
21. Merge Two Sorted Lists
Merge two sorted linked lists and return it as a new list. The new list should be made by splicing together the nodes of the first two lists.解题思路:合并两个有序列表。/** * Definition for singly-linked list. * s原创 2017-01-08 23:13:54 · 275 阅读 · 0 评论 -
LeetCode 7. Reverse Integer
Reverse digits of an integer.Example1: x = 123, return 321 Example2: x = -123, return -321分析:注意溢出情况。class Solution {public: int reverse(int x) { int digit = 0; int sign = 1;原创 2017-01-19 22:33:07 · 352 阅读 · 0 评论 -
LeetCode 8. String to Integer (atoi)
Implement atoi to convert a string to an integer.Hint: Carefully consider all possible input cases. If you want a challenge, please do not see below and ask yourself what are the possible input cases.N原创 2017-01-20 10:57:41 · 448 阅读 · 0 评论 -
LeetCode 9. Palindrome Number
Determine whether an integer is a palindrome. Do this without extra space.分析: 1. 如果将数字转化为字符串,再判断是否为回文字符串,要使用额外空间,不满足要求。 2. 考虑将数字翻转,(LeetCode 7. Reverse Integer )[http://blog.youkuaiyun.com/teffi/article/d原创 2017-01-20 14:19:06 · 246 阅读 · 0 评论 -
LeetCode 485. Max Consecutive Ones
Given a binary array, find the maximum number of consecutive 1s in this array.Example 1:Input: [1,1,0,1,1,1]Output: 3Explanation: The first two digits or the last three digits are consecutive 1s.原创 2017-01-20 22:02:23 · 620 阅读 · 0 评论 -
LeetCode 6. ZigZag Conversion
The string “PAYPALISHIRING” is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility) P A H NA P L S I I G原创 2017-01-20 15:49:16 · 271 阅读 · 0 评论 -
LeetCode 1. Two Sum
题目: Given an array of integers, return indices of the two numbers such that they add up to a specific target.You may assume that each input would have exactly one solution.Example:Given nums = [2, 7,原创 2017-01-21 10:01:50 · 299 阅读 · 0 评论 -
LeetCode 81. Search in Rotated Sorted Array II
描述Follow up for "Search in Rotated Sorted Array":What if duplicates are allowed?Would this affect the run-time complexity? How and why?Suppose an array sorted in ascending order is rotated at some piv原创 2017-02-04 00:00:32 · 285 阅读 · 0 评论 -
LeetCode 33. Search in Rotated Sorted Array
描述 Suppose an array sorted in ascending order is rotated at some pivot unknown to you beforehand.(i.e., 0 1 2 4 5 6 7 might become 4 5 6 7 0 1 2).You are given a target value to search. If found in th原创 2017-02-03 23:48:26 · 261 阅读 · 0 评论 -
LeetCode 136. Single Number
Given an array of integers, every element appears twice except for one. Find that single one.Note: Your algorithm should have a linear runtime complexity. Could you implement it without using extra me原创 2017-01-17 00:50:27 · 237 阅读 · 0 评论 -
LeetCode 138. Copy List with Random Pointer
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.Return a deep copy of the list.分析:见《剑指offer》面试题:26 复杂链表的复制。/** * Defi原创 2017-01-17 00:40:01 · 296 阅读 · 0 评论 -
148. Sort List
Sort a linked list in O(n log n) time using constant space complexity.分析:归并排序,考虑链表和数组的区别,链表每次划分为两段链表,然后有序链表归并。可以复用21. Merge Two Sorted Lists代码。/** * Definition for singly-linked list. * struct ListNo原创 2017-01-15 22:38:20 · 213 阅读 · 0 评论 -
147. Insertion Sort List
Sort a linked list using insertion sort./** * Definition for singly-linked list. * struct ListNode { * int val; * ListNode *next; * ListNode(int x) : val(x), next(NULL) {} * }; */cl原创 2017-01-15 23:28:06 · 239 阅读 · 0 评论