201312-1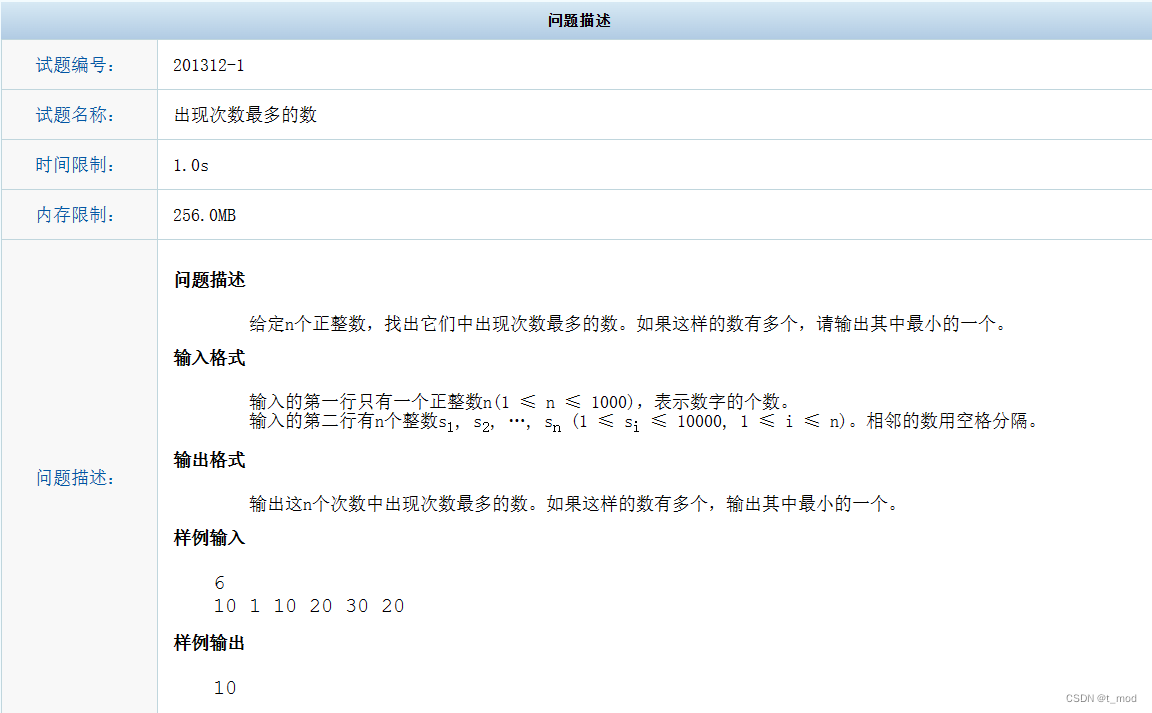
解题思路:使用数组cnt统计数字出现的次数,再倒序找到出现最多的数
参考代码
#include <bits/stdc++.h>
using namespace std;
const int N = 1e4 + 5;
int n, x;
int cnt[N];
int ans, Max;
int main() {
cin >> n;
for (int i = 1; i <= n; i++) {
cin >> x;
cnt[x]++;
}
for (int i = 10000; i >= 1; i--) {
if (cnt[i] >= Max) {
Max = cnt[i];
ans = i;
}
}
cout << ans;
return 0;
}
201312-2
解题思路:直接模拟,注意余数为10的时候得特判‘X’
参考代码
#include <bits/stdc++.h>
using namespace std;
string s;
int num[11];
int ans, cnt;
int main() {
cin >> s;
for (int i = 0; i < s.size(); i++) {
if (s[i] >= '0' && s[i] <= '9') {
num[++cnt] = s[i] - '0';
}
}
for (int i = 1; i <= 9; i++) {
ans += num[i] * i;
ans %= 11;
}
if (ans + '0' == s.back()) {
cout << "Right";
} else {
if (ans != 10) {
s.back() = ans + '0';
cout << s;
} else if (ans == 10 && s.back() == 'X') {
cout << "Right";
} else {
s.back() = 'X';
cout << s;
}
}
return 0;
}
201312-3
解题思路:找规律,发现对于区间 最大的矩形为区间长度乘区间内最低的矩形高度,既
,所以我们需要求区间
的高度最小值,可以使用线段树来实现,枚举每一个区间,不断更新最大值,时间复杂度为
参考代码
#include <bits/stdc++.h>
using namespace std;
#define lc k<<1
#define rc k<<1| 1
#define mid (l + r)/ 2
const int N = 1e3 + 5;
int n;
int h[N], t[N << 2];
inline void pushup(int k) {
t[k] = min(t[lc], t[rc]);
}
void build(int l, int r, int k) {
if (l == r) {
t[k] = h[l];
return;
}
build(l, mid, lc);
build(mid + 1, r, rc);
pushup(k);
}
int ask(int L, int R, int l, int r, int k) {
if (r < L || l > R) return 999999999;
if (L <= l && R >= r) return t[k];
int l_min = ask(L, R, l, mid, lc);
int r_min = ask(L, R, mid + 1, r, rc);
return min(l_min, r_min);
}
int ans;
int main() {
cin >> n;
for (int i = 1; i <= n; i++) cin >> h[i];
build(1, n, 1);
for (int i = 1; i <= n; i++) {
for (int j = 0; j + i <= n; j++) {
ans = max(ans, ask(i, i + j, 1, n, 1) * (j + 1));
}
}
cout << ans;
return 0;
}