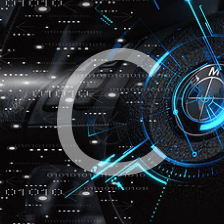
Leetcode系列
SummerHmh
这个作者很懒,什么都没留下…
展开
-
557. Reverse Words in a String III
题目链接:https://leetcode.com/problems/reverse-words-in-a-string-iii/代码class Solution: def reverseWords(self, s: str) -> str: n=len(s) start=0 res='' i=0 w...原创 2019-06-17 10:48:38 · 156 阅读 · 0 评论 -
206. Reverse Linked List
题目链接:https://leetcode.com/problems/reverse-linked-list/代码# Definition for singly-linked list.# class ListNode(object):# def __init__(self, x):# self.val = x# self.next = None...原创 2019-06-15 22:31:40 · 127 阅读 · 0 评论 -
169. Majority Element
题目链接:https://leetcode.com/problems/majority-element/代码class Solution(object): def majorityElement(self, nums): """ :type nums: List[int] :rtype: int """ f...原创 2019-06-15 22:21:42 · 139 阅读 · 0 评论 -
160. Intersection of Two Linked Lists
题目链接:https://leetcode.com/problems/intersection-of-two-linked-lists/submissions/代码# Definition for singly-linked list.# class ListNode(object):# def __init__(self, x):# self.val = x#...原创 2019-06-15 22:12:45 · 142 阅读 · 0 评论 -
155. Min Stack
题目链接:https://leetcode.com/problems/min-stack/submissions/代码class MinStack: def __init__(self): """ initialize your data structure here. """ self.stack_list=[] ...原创 2019-06-15 21:48:16 · 119 阅读 · 0 评论 -
122. Best Time to Buy and Sell Stock II
题目链接:https://leetcode.com/problems/best-time-to-buy-and-sell-stock-ii/代码class Solution: def maxProfit(self, prices) -> int: if len(prices)<1: return 0 profit=0 min_...原创 2019-06-10 00:12:54 · 112 阅读 · 0 评论 -
121. Best Time to Buy and Sell Stock
题目链接:https://leetcode.com/problems/best-time-to-buy-and-sell-stock/代码class Solution: def maxProfit(self, prices: List[int]) -> int: n,res = len(prices),0 dp=[0]*n for ...原创 2019-06-09 23:44:02 · 121 阅读 · 0 评论 -
104. Maximum Depth of Binary Tree
题目链接:https://leetcode.com/problems/maximum-depth-of-binary-tree/代码# Definition for a binary tree node.# class TreeNode:# def __init__(self, x):# self.val = x# self.left = Non...原创 2019-06-09 23:37:52 · 129 阅读 · 0 评论 -
89. Gray Code
题目链接:https://leetcode.com/problems/gray-code/submissions/代码class Solution: def grayCode(self, n: int) -> List[int]: return [ i^i>>1 for i in range(1<<n)]思路详解格雷编码:在一组数的编...原创 2019-06-09 23:29:15 · 132 阅读 · 0 评论 -
70. Climbing Stairs
题目链接:https://leetcode.com/problems/climbing-stairs/submissions/动态规划法代码class Solution: def climbStairs(self, n: int) -> int: #入参判断 if not n or n ==1 : return 1 #初始赋值 ...原创 2019-06-03 09:49:34 · 162 阅读 · 0 评论 -
148. Sort List
题目链接:https://leetcode.com/problems/sort-list/归并排序法代码# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Noneclass Solu...原创 2019-06-14 10:54:05 · 242 阅读 · 0 评论 -
215. Kth Largest Element in an Array
题目链接:https://leetcode.com/problems/kth-largest-element-in-an-array/submissions/代码class Solution: def findKthLargest(self, nums: List[int], k: int) -> int: """ :type nums: List...原创 2019-06-16 00:47:23 · 183 阅读 · 0 评论 -
217. Contains Duplicate
题目链接:https://leetcode.com/problems/contains-duplicate/submissions/代码class Solution: def containsDuplicate(self, nums: List[int]) -> bool: if not nums:return False frequen=set(...原创 2019-06-16 00:54:13 · 197 阅读 · 0 评论 -
344. Reverse String
题目链接:https://leetcode.com/problems/reverse-string/代码class Solution: def reverseString(self, s: List[str]) -> None: """ Do not return anything, modify s in-place instead. ...原创 2019-06-17 10:45:52 · 244 阅读 · 0 评论 -
292. Nim Game
题目链接:https://leetcode.com/problems/nim-game/代码class Solution: def canWinNim(self, n: int) -> bool: return n%4!=0原创 2019-06-17 10:19:11 · 157 阅读 · 0 评论 -
238. Product of Array Except Self
题目链接:https://leetcode.com/problems/product-of-array-except-self/代码class Solution: def productExceptSelf(self, nums: List[int]) -> List[int]: n=len(nums) dp1,dp2=[1]*n,[1]*n ...原创 2019-06-17 10:02:24 · 181 阅读 · 0 评论 -
237. Delete Node in a Linked List
题目链接:https://leetcode.com/problems/delete-node-in-a-linked-list/代码# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Non...原创 2019-06-17 09:30:51 · 128 阅读 · 0 评论 -
236. Lowest Common Ancestor of a Binary Tree
题目链接:https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-tree/代码# Definition for a binary tree node.# class TreeNode:# def __init__(self, x):# self.val = x# self...原创 2019-06-17 09:23:50 · 155 阅读 · 0 评论 -
235. Lowest Common Ancestor of a Binary Search Tree
题目链接:https://leetcode.com/problems/lowest-common-ancestor-of-a-binary-search-tree/代码# Definition for a binary tree node.# class TreeNode:# def __init__(self, x):# self.val = x# ...原创 2019-06-17 09:21:15 · 131 阅读 · 0 评论 -
231. Power of Two
题目链接:https://leetcode.com/problems/power-of-two/代码class Solution: def isPowerOfTwo(self, n: int) -> bool: if n==0:return False return not n&(n-1)解题思路2n2^n2n在二进制上只有一位是1,...原创 2019-06-16 23:14:28 · 154 阅读 · 0 评论 -
230. Kth Smallest Element in a BST
题目链接:https://leetcode.com/problems/kth-smallest-element-in-a-bst/代码# Definition for a binary tree node.# class TreeNode:# def __init__(self, x):# self.val = x# self.left = No...原创 2019-06-16 22:57:34 · 122 阅读 · 0 评论 -
136. Single Number
题目链接:https://leetcode.com/problems/single-number/代码class Solution: def singleNumber(self, nums: List[int]) -> int: for i in range(1,len(nums)): nums[0]^=nums[i] re...原创 2019-06-11 11:26:25 · 114 阅读 · 0 评论 -
124. Binary Tree Maximum Path Sum
题目链接:https://leetcode.com/problems/binary-tree-maximum-path-sum/代码# Definition for a binary tree node.# class TreeNode:# def __init__(self, x):# self.val = x# self.left = Non...原创 2019-06-11 11:15:14 · 144 阅读 · 0 评论 -
146. LRU Cache
题目链接:https://leetcode.com/problems/lru-cache/代码class LRUCache(object): def __init__(self, capacity): """ :type capacity: int """ self.capacity=capacity s...原创 2019-06-14 09:59:30 · 134 阅读 · 0 评论 -
62. Unique Paths
题目链接:https://leetcode.com/problems/unique-paths/自己的思路代码class Solution: def uniquePaths(self, m, n) -> int: while not m or not n : return 0 dp = [[0 for col in range(n)] for r...原创 2019-06-03 00:30:37 · 117 阅读 · 0 评论 -
54. Spiral Matrix
题目链接:https://leetcode.com/problems/spiral-matrix/代码class Solution: def spiralOrder(self, matrix: List[List[int]]) -> List[int]: return matrix and [*matrix.pop(0)] + self.spiralOrder([...原创 2019-05-29 23:25:15 · 209 阅读 · 0 评论 -
23. Merge k Sorted Lists
题目链接:https://leetcode.com/problems/merge-k-sorted-lists/代码# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Noneclass...原创 2019-05-21 22:09:56 · 91 阅读 · 0 评论 -
21. Merge Two Sorted Lists
题目链接:https://leetcode.com/problems/merge-two-sorted-lists/代码# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Nonecla...原创 2019-05-21 22:02:30 · 114 阅读 · 0 评论 -
20. Valid Parentheses
题目链接:https://leetcode.com/problems/valid-parentheses/方法一 栈的方式代码class Solution: def isValid(self, s: str) -> bool: if not s or s==[] : return True if len(s)&1 :return Fals...原创 2019-05-21 21:35:08 · 126 阅读 · 0 评论 -
5. Longest Palindromic Substring
题目链接:https://leetcode.com/problems/longest-palindromic-substring/代码:class Solution: def longestPalindrome(self, s: str) -> str: def helper(i, j): while i >=0 and j...原创 2019-05-12 11:59:55 · 125 阅读 · 0 评论 -
26. Remove Duplicates from Sorted Array
题目链接:https://leetcode.com/problems/remove-duplicates-from-sorted-array/代码class Solution: def removeDuplicates(self, nums: List[int]) -> int: if len(nums) == 0: return 0 ...原创 2019-05-24 23:40:17 · 113 阅读 · 0 评论 -
4. Median of Two Sorted Arrays
题目链接:https://leetcode.com/problems/median-of-two-sorted-arrays/代码class Solution: def findMedianSortedArrays(self, nums1: List[int], nums2: List[int]) -> float: #思路:有序扔除一些数,直到扔出一半 ...原创 2019-05-11 12:01:01 · 125 阅读 · 0 评论 -
2. Add Two Numbers
题目链接:https://leetcode.com/problems/add-two-numbers/代码# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Noneclass Solu...原创 2019-05-10 16:52:24 · 176 阅读 · 0 评论 -
9. Palindrome Number
题目链接:https://leetcode.com/problems/palindrome-number/代码全部反转后对比class Solution: def isPalindrome(self, x: int) -> bool: if x<0: return False else: ...原创 2019-05-15 12:00:32 · 155 阅读 · 0 评论 -
16. 3Sum Closest
题目链接:https://leetcode.com/problems/3sum-closest/代码class Solution: def threeSumClosest(self, nums, target) -> int: l=len(nums) nums.sort() res=float('inf') for ...原创 2019-05-20 11:52:08 · 113 阅读 · 0 评论 -
15. 3Sum
题目链接:https://leetcode.com/problems/3sum/代码class Solution: def threeSum(self, nums: List[int]) -> List[List[int]]: count={} #统计出现的次数,放入dict里面,其中key为值,value为次数 for num i...原创 2019-05-20 10:37:21 · 170 阅读 · 0 评论 -
11. Container With Most Water
题目链接:https://leetcode.com/problems/container-with-most-water/代码class Solution: def maxArea(self, height: List[int]) -> int: max_area,l=0,len(height) left,right=0,l-1 w...原创 2019-05-16 23:45:16 · 151 阅读 · 0 评论 -
7. Reverse Integer
题目链接:https://leetcode.com/problems/reverse-integer/思路一:作为值处理代码class Solution: def reverse(self, x: int) -> int: res,x_abs=0,abs(x) while x_abs: res,x_abs=res*10+x...原创 2019-05-13 10:13:02 · 128 阅读 · 0 评论 -
53. Maximum Subarray
题目链接:https://leetcode.com/problems/maximum-subarray/代码class Solution: def maxSubArray(self, nums: List[int]) -> int: # 为空的时候,不懂得为啥这么设置 if not nums: return -2147483648 ...原创 2019-05-29 22:57:12 · 122 阅读 · 0 评论 -
61. Rotate List
题目链接:https://leetcode.com/problems/rotate-list/自己的思路代码# Definition for singly-linked list.# class ListNode:# def __init__(self, x):# self.val = x# self.next = Noneclass So...原创 2019-06-02 11:23:22 · 117 阅读 · 0 评论