双向链表结构
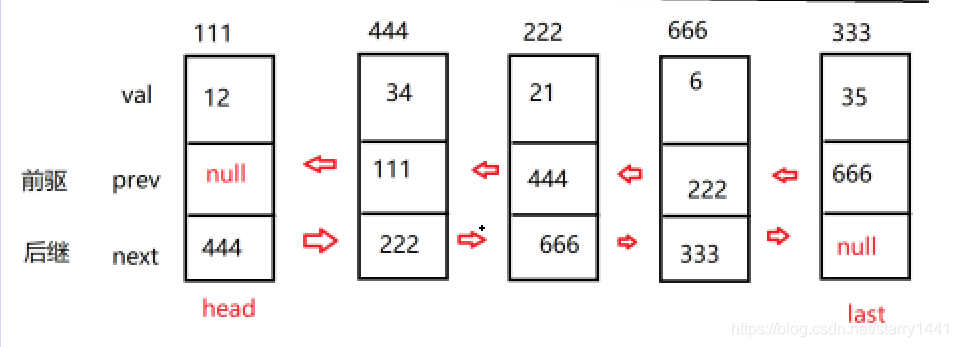
双向链表实现
class ListNode {
public int val;
public ListNode next;
public ListNode prev;
public ListNode(int val) {
this.val = val;
}
}
public class DoubleLinkedList {
public ListNode head;
public ListNode last;
public void addFirst(int data) {
ListNode node = new ListNode(data);
if(head == null) {
head = node;
last = node;
return;
}
node.next = head;
head.prev = node;
head = node;
}
public void addLast(int data){
ListNode node = new ListNode(data);
if(last == null) {
head = node;
last = node;
return;
}
last.next = node;
node.prev = last;
last = node;
}
public void addIndex(int index,int data){
if(index < 0 || index > size()) return;
if(index == 0) {
addFirst(data);
return;
}
if(index == size()) {
addLast(data);
return;
}
ListNode cur = head;
ListNode node = new ListNode(data);
while (index != 0) {
cur = cur.next;
index--;
}
node.next = cur;
node.prev = cur.prev;
cur.prev.next = node;
cur.prev = node;
}
public boolean contains(int key){
ListNode cur = head;
while(cur != null) {
if(cur.val == key) {
return true;
}
cur = cur.next;
}
return false;
}
public ListNode findNode(int key) {
ListNode cur = head;
while (cur != null) {
if(cur.val == key) {
return cur;
}
cur = cur.next;
}
return null;
}
public void remove(int key){
ListNode cur = findNode(key);
if(cur == null) return;
if(cur == head) {
head = head.next;
head.prev = null;
return;
}
if(cur == last) {
last = last.prev;
last.next = null;
return;
}
cur.next.prev = cur.prev;
cur.prev.next = cur.next;
}
public void remove2(int key){
ListNode cur = head;
while(cur != null) {
if(cur.val == key) {
if(cur == head) {
head = head.next;
head.prev = null;
}else {
cur.prev.next = cur.next;
if(cur.next != null) {
cur.next.prev = cur.prev;
}else {
last = last.prev;
}
}
return;
}
cur = cur.next;
}
}
public void removeAllKey(int key){
ListNode cur = head;
while(cur != null) {
if(cur.val == key) {
if(cur == head) {
head = head.next;
head.prev = null;
}else {
cur.prev.next = cur.next;
if(cur.next != null) {
cur.next.prev = cur.prev;
}else {
last = last.prev;
}
}
}
cur = cur.next;
}
}
public int size(){
ListNode cur = head;
int count = 0;
while (cur != null) {
count++;
cur = cur.next;
}
return count;
}
public void display(){
ListNode cur = head;
while(cur != null) {
System.out.print(cur.val + " ");
cur = cur.next;
}
System.out.println();
}
public void clear(){
head = null;
last = null;
}
public void clear2() {
ListNode cur = head;
while(cur != null) {
ListNode curNext = cur.next;
cur.next = null;
cur.prev = null;
cur = curNext;
}
last = null;
head = null;
}
public static void main(String[] args) {
DoubleLinkedList a = new DoubleLinkedList();
a.addFirst(1);
a.addFirst(2);
a.addFirst(3);
a.addFirst(4);
a.display();
System.out.println("=============");
a.addLast(5);
a.addLast(6);
a.display();
System.out.println("=============");
a.addIndex(6,88);
a.addIndex(0,99);
a.addIndex(3,99);
a.display();
System.out.println("=============");
System.out.println(a.contains(5));
System.out.println(a.contains(55));
System.out.println("=============");
a.remove(99);
a.remove(88);
a.remove(99);
a.display();
System.out.println("=============");
a.addFirst(2);
a.addLast(2);
a.display();
a.removeAllKey(2);
a.display();
System.out.println("=============");
a.clear();
}
}