vue2
<template>
<div>
<div>
<div class="dropzone" @dragover.prevent @drop="dropFile">
<span>拖拽文件到此处或<em style="color:blue;" @click="showFileInput">点击上传</em></span>
<input ref="fileInput" class="file_upload" type="file" @change="onFileChange" multiple style="display:none;">
</div>
</div>
<el-table :data="fileList" max-height="400">
<el-table-column prop="name" label="文件名"></el-table-column>
<el-table-column prop="size" label="文件大小"></el-table-column>
<el-table-column prop="type" label="文件类型"></el-table-column>
<el-table-column label="上传进度">
<template slot-scope="scope">
<el-progress :percentage="scope.row.progress" />
<div v-if="scope.row.status === 'failed'">
<span style="color: red;">上传失败</span>
</div>
<div v-if="scope.row.status === 'success'">
<span style="color: #49af2f;">上传成功</span>
</div>
</template>
</el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button v-if="scope.row.status === 'failed'" type="primary" size="mini"
@click="retryUpload(scope.$index)">重新上传</el-button>
<el-button size="mini" @click="deleteUpload(scope.$index)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-button type="primary" @click="upload_async">上传(async方式)</el-button>
<el-button type="primary" @click="upload_promises">上传(promises方式)</el-button>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
allowedTypes: [ "gif",
"jpeg",
"jpg",
"png",
"psd",
"rar",
"zip",
"pdf",
"doc",
"docx",
"ppt",
"pptx",
"txt",
"xls",
"xlsx",
],
fileList: [],
api: 'http://leju.bufan.cloud/lejuAdmin/material/uploadFileOss',
token: 'eyJhbGciOiJIUzUxMiIsInppcCI6IkdaSVAifQ.H4sIAAAAAAAAAKtWKi5NUrJSSkzJzcxT0lFKrShQsjI0szAwsDQ1M7CsBQAhz0vSIAAAAA.BoZCaxWqEkJEOIPjWPbd5EU_Fi84upgT_obDgQ3V1--oRLCDMkxVzbD2hkYjNHZOf0Hhvp9KcV4V4kTAkiZo9w'
}
},
methods: {
addFiles_Push(files) {
let invalidFilesCount = 0;
let invalidFileNames = [];
const filesArray = Array.from(files);
for (let i = 0; i < filesArray.length; i++) {
const file = filesArray[i]
const fileType = file.name.split(".").pop().toLowerCase();
if (!this.allowedTypes.includes(fileType)) {
invalidFilesCount++;
invalidFileNames.push(file.name);
continue;
}
//验证文件大小 10MB
const maxFileSize = 10 * 1024 * 1024;
if (file.size > maxFileSize) {
invalidFilesCount++;
invalidFileNames.push(file.name);
continue;
}
this.fileList.push({
name: file.name,
size: file.size,
type: file.type,
progress: 0,
CalibrationSupplier: "",
CertificateNumber: "",
status: 'pending',
file: file,
})
}
if (invalidFilesCount > 0) {
let errorMessage = `有 ${invalidFilesCount} 个文件类型或大小不允许上传:`;
if (invalidFileNames.length > 0) {
errorMessage += `${invalidFileNames.join("、")}`;
}
this.$notify.error({ title: '错误', message: errorMessage });
}
console.log('这个是需要上传的文件', this.files)
},
dropFile(e) {
e.preventDefault();
// e.dataTransfer.files 是获取拖拽文件的
const files = e.dataTransfer.files
this.addFiles_Push(files)
},
showFileInput() {
this.$refs.fileInput.click()
},
onFileChange(e) {
// e.target.files 是获取选择文件的
const files = e.target.files
this.addFiles_Push(files)
},
async upload_async() {
for (let i = 0; i < this.fileList.length; i++) {
const fileItem = this.fileList[i]
if (fileItem.status === 'pending') {
fileItem.status = 'uploading'
try {
const formData = new FormData()
formData.append('file', fileItem.file)
console.log('这个是上传时的', formData)
const response = await axios.post('http://leju.bufan.cloud/lejuAdmin/material/uploadFileOss', formData, {
headers: {
token: ` ${this.token}` // 在请求头中添加 token 字段 但是依旧添加错了
},
onUploadProgress: (progressEvent) => {
const progress = Math.round((progressEvent.loaded * 100) / progressEvent.total)
fileItem.progress = progress
},
})
fileItem.status = 'success'
} catch (error) {
fileItem.status = 'failed'
}
}
}
},
async upload_promises() {
const promises = [];
for (let i = 0; i < this.fileList.length; i++) {
const fileItem = this.fileList[i];
if (fileItem.status === "pending") {
fileItem.status = "uploading";
const formData = new FormData();
formData.append("file", fileItem.file);
const promise = axios
.post(this.api, formData, {
headers: {
token: this.token,
},
onUploadProgress: (progressEvent) => {
const progress = Math.round(
(progressEvent.loaded * 100) / progressEvent.total
);
fileItem.progress = progress;
},
})
.then(() => {
fileItem.status = "success";
})
.catch(() => {
fileItem.status = "failed";
});
promises.push(promise);
}
}
await Promise.all(promises);
},
retryUpload(index) {
const fileItem = this.fileList[index]
fileItem.status = 'pending'
fileItem.progress = 0
this.upload()
},
deleteUpload(index) {
this.fileList.splice(index, 1)
}
},
}
</script>
<style scoped>
.dropzone {
background-color: #eee;
border: 1px dashed #ccc;
margin-bottom: 10px;
padding: 20px;
}
p {
margin: 0;
}
</style>
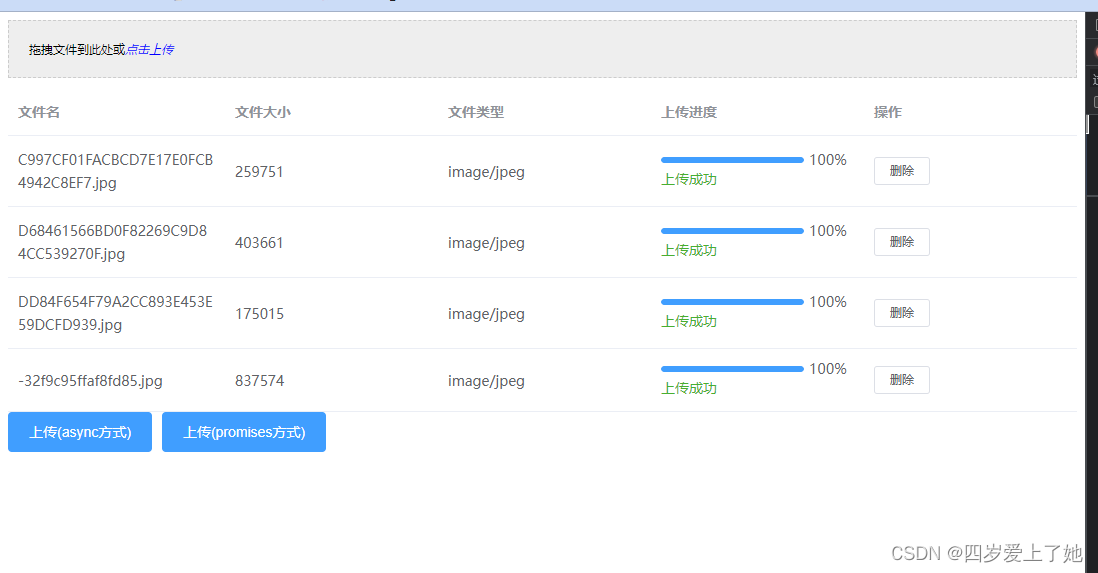
async uploadFiles() {
const CHUNK_SIZE = 1024 * 1024;
const promises = [];
for (let i = 0; i < this.files.length; i++) {
const fileItem = this.files[i];
if (fileItem.status === "pending") {
fileItem.status = "uploading";
const formData = new FormData();
formData.append("name", fileItem.name);
formData.append("size", fileItem.size);
formData.append("type", fileItem.type);
formData.append("__RequestVerificationToken", $.lrToken);
let startByte = 0;
let endByte = Math.min(CHUNK_SIZE, fileItem.size);
let chunkIndex = 0;
while (startByte < fileItem.size) {
const chunkFormData = new FormData();
const chunkBlob = fileItem.file.slice(startByte, endByte);
chunkFormData.append('file', chunkBlob, fileItem.name + '.' + chunkIndex);
chunkFormData.append('chunkIndex', chunkIndex);
console.log('这个是上传的', chunkFormData);
const promise = new Promise((resolve, reject) => {
$.ajax({
url: top.$.rootUrl + "/LR_SystemModule/Annexes/UploadAnnexesFileChunk",
type: "POST",
data: chunkFormData,
processData: false,
contentType: false,
xhr: () => {
const xhr = new XMLHttpRequest();
xhr.upload.onprogress = (e) => {
const progress = Math.round(((startByte + e.loaded) * 100) / fileItem.size);
fileItem.progress = progress;
};
return xhr;
},
success: () => {
chunkIndex++;
startByte = endByte;
endByte = Math.min(endByte + CHUNK_SIZE, fileItem.size);
if (startByte >= fileItem.size) {
$.ajax({
url: top.$.rootUrl + "/LR_SystemModule/Annexes/MergeAnnexesFileChunks",
type: "POST",
data: formData,
processData: false,
contentType: false,
success: () => {
fileItem.status = "success";
resolve();
},
error: () => {
fileItem.status = "failed";
reject();
}
});
}
},
error: () => {
fileItem.status = "failed";
reject();
}
});
});
promises.push(promise);
}
}
}
await Promise.all(promises);
},
vue2第二种方式
<template>
<div style="width: 100%">
<el-descriptions class="margin-top" :column="3" size="small" border>
<el-descriptions-item
label="上传附件:"
span="3"
width="130px"
:labelStyle="rowRight"
>
<div style="display: flex; justify-content: space-between">
<div style="width: 50%; height: 100%">
<el-upload
drag
action=""
ref="upload"
:auto-upload="false"
:show-file-list="false"
multiple
:file-list="fileList"
:on-change="handleChange"
>
<div class="el-upload__text">
请拖入文件,或<em>点击此处选择文件</em>
</div>
</el-upload>
</div>
<div style="flex: 1">
<h3 style="color: red">上传说明:</h3>
<h4>
1、上传时前几秒可能会卡顿,耐心等待一小会,然后表格里的进度条就会执行的,别着急啊,亲。文件越多时间越长...
</h4>
<h4>2、已经上传成功的文件不会重复上传。</h4>
<h4>
3、上传前请选择好文件类型,因为上传成功后就不允许修改,要修改的话就只能重选文件进行上传。
</h4>
<h4>
4、<span style="color: red"
>.xls文件和.doc文件不允许上传,如果需要上传excel表格和word文档,就请选择.xlsx文件和.docx文件</span
>。
</h4>
</div>
</div>
<div style="display: flex; justify-content: space-around">
<el-button
style="color: #36d; background-color: #d1a4a4"
size="mini"
@click="upload_dataFileTwoList()"
>确认上传表格文件</el-button
>
<el-button
style="color: #000; background-color: #258ccb"
size="mini"
@click="qingkong()"
>一键清空表格文件</el-button
>
</div>
</el-descriptions-item>
</el-descriptions>
<div style="margin-top: 10px">选择的附件如下:</div>
<el-table
ref="FileData"
:data="fileList"
size="mini"
style="width: 100%"
height="300"
border
>
<el-table-column type="index" width="50" align="center" />
<el-table-column
prop="name"
label="文件名称"
show-overflow-tooltip
align="center"
>
<template slot-scope="scope">
<div>
<el-input size="mini" v-model="scope.row.name"></el-input>
</div>
</template>
</el-table-column>
<el-table-column
prop="ACTION_TYPE"
label="文件类型"
show-overflow-tooltip
align="center"
>
<template slot-scope="scope">
<div>
<el-select
style="width: 86%"
:disabled="scope.row.status === 'success' ? true : false"
@change="(event) => onChange(event, scope.$index)"
v-model="scope.row['ACTION_TYPE']"
size="mini"
filterable
placeholder="请选择"
>
<el-option
v-for="item in FuJian_Type_List.list"
:key="item.value"
:label="item.label"
:value="item.value"
>
</el-option>
</el-select>
</div>
</template>
</el-table-column>
<el-table-column
prop="SizeName"
label="文件大小"
show-overflow-tooltip
align="center"
>
</el-table-column>
<el-table-column label="上传进度">
<template slot-scope="scope">
<!-- <el-progress :percentage="scope.row.progress" /> -->
<div v-if="scope.row.status === 'failed'">
<span style="color: red" :id="'item-' + scope.$index"
>上传失败</span
>
</div>
<div v-if="scope.row.status === 'success'">
<span style="color: #49af2f" :id="'item-' + scope.$index"
>上传成功</span
>
</div>
<div v-if="scope.row.status === '上传中'">
<span style="color: #36d" :id="'item-' + scope.$index"
>正在上传中:{{ scope.row.progress }}%</span
>
</div>
</template>
</el-table-column>
<el-table-column
prop="AxiosUrl"
label="下载"
show-overflow-tooltip
align="center"
>
<template slot-scope="scope">
<div
style="cursor: pointer"
v-if="scope.row.status === 'success'"
@click="downloadEvent(scope.row)"
>
文件下载
</div>
</template>
</el-table-column>
<el-table-column fixed="right" align="center" label="操作" width="180">
<template slot-scope="scope">
<el-button
link
type="success"
size="mini"
v-if="scope.row.status === 'failed'"
@click.prevent="congxinshangchuan(scope.$index, scope.row)"
>
重新上传
</el-button>
<el-button
link
type="primary"
size="mini"
@click.prevent="deleteRow_OK(scope.$index, scope.row)"
>
删除
</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
import axios from "axios";
import { DeleteBaoXianFuJian } from "../api/ExpenseAccount";
import { DeleteFuJian } from "../api/index";
export default {
props: {
bxcode: {
type: String,
default: null,
},
tbxcode: {
type: String,
default: null,
},
},
data() {
return {
FuJian_Type_List: {
list: [
{
value: "附件",
label: "附件",
},
{
value: "发票",
label: "发票",
},
{
value: "合同",
label: "合同",
},
{
value: "其他",
label: "其他",
},
],
type: "附件",
},
rowRight: {
"text-align": "right",
},
Cookie_USER: null,
fileList: [],
fileList_OK: [],
NewCode: {
bxcode: "",
tbxcode: "",
},
};
},
mounted() {
this.$store.dispatch("COOLIE_VALUE_USER");
this.Cookie_USER = this.$store.state.cookieUser;
this.NewCode.bxcode = this.bxcode;
this.NewCode.tbxcode = this.tbxcode;
},
methods: {
onChange(e, index) {
this.$set(this.fileList[index], "ACTION_TYPE", e);
let list = [];
for (let i = 0; i < this.fileList.length; i++) {
list.push({
...this.fileList[i],
raw: this.cloneFile(this.fileList[i].raw),
});
}
this.fileList = list;
},
cloneFile(file) {
return new File([file], file.name, { type: file.type });
},
upload_dataFileTwoList() {
if (this.fileList.length > 0) {
for (let i = 0; i < this.fileList.length; i++) {
let list = this.fileList[i];
if (list.status == "failed" || list.status == "pending") {
this.upload_FN(i, list);
}
}
} else {
this.$message({
message: "老铁,需要先选择文件在点击上传",
type: "warning",
});
}
},
handleChange(file, fileList) {
console.log("上传的文件类型", file);
if (this.isFileTypeAllowed(file.raw)) {
file.progress = 0;
file.status = "pending";
file.NewName = file.name;
file.SizeName = this.bytesToMegabytes(file.size);
file.AxiosUrl = "";
file.ACTION_TYPE = this.FuJian_Type_List.type;
file.Del_id = "";
this.fileList.push(file);
} else {
console.log("格式不支持");
this.$notify.error({
title: "提示",
message:
".xls和.doc这两种文件不支持,如果需要上传表格和word文档就请选择 .xlsx文件 和 .docx文件",
});
}
},
bytesToMegabytes(bytes) {
const megabytes = bytes / (1024 * 1024);
return megabytes % 1 === 0
? `${megabytes.toFixed(0)}M`
: `${megabytes.toFixed(2)}M`;
},
isFileTypeAllowed(file) {
const forbiddenTypes = [
"application/vnd.ms-excel",
"application/msword",
];
const fileType = file.type;
if (forbiddenTypes.includes(fileType)) {
return false;
}
return true;
},
async upload_FN(itemINdex, FileItem, qiepian = 0.3) {
console.log(this.YM, "aaaaa", FileItem);
let baseURl_QiePian = "https://jcnh.jiuchang.work:17776/api/JcShare/UploadSlice";
try {
let { ACTION_TYPE, NewName, name, size, raw: uploadfile } = FileItem;
let uploadedChunks = 0;
let hash = this.generateRandomStringWithTimestamp(6);
const chunkSize = qiepian * 1024 * 1024;
const totalChunks = Math.ceil(size / chunkSize);
const concurrency = 3;
const promises = [];
for (let index = 0; index < totalChunks; index++) {
const start = index * chunkSize;
const end = Math.min(start + chunkSize, size);
const chunk = uploadfile.slice(start, end);
const chunkFile = new File([chunk], NewName);
const formData = new FormData();
formData.append("file", chunkFile);
formData.append("sliceIndex", index);
formData.append("totalSlices", totalChunks);
formData.append("fileName", hash + NewName);
formData.append("savefile", "fileList");
formData.append("newname", name ? name : NewName);
formData.append("tbxcode", this.NewCode.tbxcode);
formData.append(
"bxcode",
this.NewCode.bxcode ? this.NewCode.bxcode : this.NewCode.tbxcode
);
formData.append("type", ACTION_TYPE);
formData.append("fillname", this.Cookie_USER.realname1);
const promise = axios
.post(baseURl_QiePian, formData, {
headers: { "Content-type": "multipart/form-data" },
})
.then((response) => {
uploadedChunks++;
let progress = Math.round((uploadedChunks / totalChunks) * 100);
let uploadCube = document.querySelector(`#item-${itemINdex}`);
if (response.data.code == 200 && response.data.msg == "成功") {
FileItem.progress = 100;
if (uploadCube) {
uploadCube.innerText = `上传成功:${FileItem.progress}%`;
}
FileItem.status = "success";
FileItem.AxiosUrl = response.data.data;
if (this.ActionType == "编辑") {
this.$refs.editLOOK.gengxin();
}
} else if (
response.data.code == 200 &&
response.data.msg == "等待上传中"
) {
FileItem.progress = progress;
FileItem.status = "上传中";
FileItem.AxiosUrl = "";
if (uploadCube) {
uploadCube.innerText = `正在上传中:${FileItem.progress}%`;
}
this.$nextTick(() => {
console.log("这个是不是一直在执行", progress);
this.$refs.FileData.doLayout();
});
} else {
FileItem.progress = progress;
if (uploadCube) {
uploadCube.innerText = `上传失败:${FileItem.progress}%`;
}
FileItem.status = "failed";
FileItem.AxiosUrl = "";
}
});
promises.push(promise);
if (promises.length >= concurrency || index === totalChunks - 1) {
await Promise.all(promises);
promises.length = 0;
}
}
} catch (error) {
console.error("上传失败", error);
}
},
generateRandomStringWithTimestamp(length) {
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
let result = "";
const charactersLength = characters.length;
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * charactersLength);
result += characters.charAt(randomIndex);
}
const timestamp = Date.now().toString();
result += timestamp;
return result;
},
congxinshangchuan(index, val) {
val.progress = 0;
val.status = "pending";
val.AxiosUrl = "";
this.upload_FN(index, val);
},
deleteRow_OK(index, val) {
if (val.status == "success") {
const loading = this.$loading({
lock: true,
text: "文件删除中。。。",
spinner: "el-icon-loading",
background: "rgba(0, 0, 0, 0.7)",
});
let obj = {
tbxcode: this.NewCode.tbxcode,
id: val.AxiosUrl,
};
let that = this;
setTimeout(() => {
let api = null;
if (this.YM == "改变") {
api = DeleteFuJian(obj);
} else {
api = DeleteBaoXianFuJian(obj);
}
api
.then((res) => {
if (res.code == 200) {
that.fileList.splice(index, 1);
if (this.ActionType == "编辑") {
this.$refs.editLOOK.gengxin();
}
} else {
that.$notify.error({
title: "提示",
message: res.msg,
});
}
})
.finally(() => {
loading.close();
});
}, 1500);
} else {
this.fileList.splice(index, 1);
}
},
qingkong() {
this.fileList = [];
},
downloadEvent(val) {
const loading = this.$loading({
lock: true,
text: "文件准备中。。。",
spinner: "el-icon-loading",
background: "rgba(0, 0, 0, 0.7)",
});
let name = "";
if (val.name.includes(".")) {
const parts = val.name.split(".");
name = parts[0];
} else {
name = val.name;
}
fetch(val.AxiosUrl)
.then((response) => response.blob())
.then((blob) => {
loading.close();
const blobUrl = URL.createObjectURL(blob);
const a = document.createElement("a");
a.href = blobUrl;
a.download = name;
a.click();
URL.revokeObjectURL(blobUrl);
})
.catch((error) => {
loading.close();
this.$notify.error({
title: "提示",
message: "文件暂时不能下载",
});
console.error("文件下载失败:", error);
});
},
},
};
</script>
<style scoped>
.el-upload {
width: 100%;
height: 100%;
}
.el-upload-dragger {
width: 100%;
height: 100%;
}
</style>
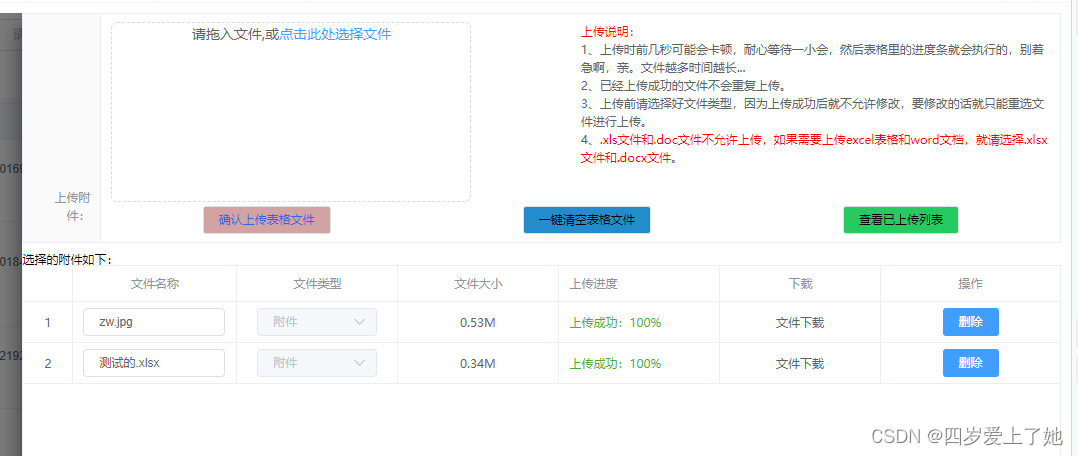
vue3
<template>
<el-upload
class="upload-demo"
drag
ref="upload"
:auto-upload="false"
:show-file-list="false"
multiple
:file-list="fileList"
@change="handleChange"
>
<el-icon class="el-icon--upload"><upload-filled /></el-icon>
<div class="el-upload__text">请拖入文件,或<em>点击此处选择文件</em></div>
</el-upload>
<div
style="width: 100%; margin: 10px 0; display: flex; justify-content: center"
>
<el-button color="#626aef" size="small" @click="upload_promises"
>确认上传(杰)</el-button
>
<el-button color="#626aef" size="small" @click="upload_promisesTwo"
>确认上传(阳)</el-button
>
<el-button color="#626aef" size="small" @click="onClick_UP_List"
>确认上传{{ fileList.length }}个选择的附件</el-button
>
</div>
<el-table :data="fileList" style="width: 100%" max-height="250" border>
<el-table-column type="index" width="50" align="center" />
<el-table-column
prop="name"
label="选项"
show-overflow-tooltip
align="center"
/>
<el-table-column label="上传进度">
<template #default="scope">
<el-progress :percentage="scope.row.progress" />
<div v-if="scope.row.status === 'failed'">
<span style="color: red">上传失败</span>
</div>
<div v-if="scope.row.status === 'success'">
<span style="color: #49af2f">上传成功</span>
</div>
</template>
</el-table-column>
<el-table-column fixed="right" label="操作" width="120">
<template #default="scope">
<el-button
link
type="primary"
size="small"
@click.prevent="deleteRow(scope.$index)"
>
删除
</el-button>
</template>
</el-table-column>
</el-table>
</template>
<script setup>
import { ref, onMounted, nextTick } from "vue";
import { JcUploadFile, TXUploadFile } from "../api/JCNB/index";
import {CSUpload} from '../api/index'
import { ElMessage, ElLoading } from "element-plus";
import axios from "axios";
const emit = defineEmits(["submitOK"]);
const baseURL_JIE = "xxxxxx.com";
const baseURL_YANG='xxx.com'
const props = defineProps({
limit: {
type: Number,
default: 1,
},
upType: {
type: String,
default: "普通",
},
});
let queryParams = ref({
type: "普通",
});
const upload_promisesTwo =async () => {
let list = fileList.value;
if (list.length > 0) {
const promises = [];
for (let i = 0; i < list.length; i++) {
const fileItem = list[i];
console.log("执行了", fileItem);
if (fileItem.status === "pending") {
fileItem.status = "uploading";
const formData = new FormData();
formData.append("file", fileItem.raw);
formData.append("testing", "storage");
formData.append("action", "up/qingjia");
console.log("执行了??");
const promise = axios
.post(baseURL_YANG, formData, {
onUploadProgress: (progressEvent) => {
const progress = Math.round(
(progressEvent.loaded * 100) / progressEvent.total
);
console.log("进度", progress);
fileItem.progress = progress;
},
})
.then(() => {
fileItem.status = "success";
})
.catch(() => {
fileItem.status = "failed";
});
promises.push(promise);
}
}
await Promise.all(promises);
} else {
ElMessage({
message: `至少选择一个文件才能点击此按钮`,
type: "warning",
});
}
};
const upload_promises = async () => {
let list = fileList.value;
if (list.length > 0) {
const promises = [];
for (let i = 0; i < list.length; i++) {
const fileItem = list[i];
console.log("执行了", fileItem);
if (fileItem.status === "pending") {
fileItem.status = "uploading";
const formData = new FormData();
formData.append("file", fileItem.raw);
formData.append("testing", "storage");
formData.append("action", "up/qingjia");
console.log("执行了??");
const promise = axios
.post(baseURL_JIE, formData, {
onUploadProgress: (progressEvent) => {
const progress = Math.round(
(progressEvent.loaded * 100) / progressEvent.total
);
console.log("进度", progress);
fileItem.progress = progress;
},
})
.then(() => {
fileItem.status = "success";
})
.catch(() => {
fileItem.status = "failed";
});
promises.push(promise);
}
}
await Promise.all(promises);
} else {
ElMessage({
message: `至少选择一个文件才能点击此按钮`,
type: "warning",
});
}
};
onMounted(() => {
queryParams.value.type = props.upType;
console.log(queryParams.value.type);
});
const deleteRow = (val) => {
fileList.value.splice(val, 1);
};
const upload = ref(null);
const fileList = ref([]);
const handleChange = (file, e) => {
let list = e.map((item) => {
item.progress = 0;
item.status = "pending";
return item;
});
console.log("文件e", list);
fileList.value = list;
};
const handleRemove = (e, list) => {
fileList.value = list;
};
const handleExceed = (uploadFile, uploadFiles) => {
ElMessage({
message: `文件最大上传个数是:${uploadFiles.length}个文件`,
type: "warning",
});
};
const onClick_UP_List = () => {
let list = fileList.value;
if (list.length > 0) {
const loading = ElLoading.service({
lock: true,
text: "附件上传操作中。。。",
background: "rgba(0, 0, 0, 0.7)",
});
let promises = list.map((item) => submitUpload(item.raw));
Promise.all(promises)
.then((res) => {
emit("submitOK", res);
})
.finally(() => {
loading.close();
});
} else {
ElMessage({
message: `至少选择一个文件才能点击此按钮`,
type: "warning",
});
}
};
const submitUpload = (file) => {
return new Promise((resolve, reject) => {
if (queryParams.value.type == "普通") {
const formData = new FormData();
formData.append("file", file);
formData.append("action", "up/qingjia");
JcUploadFile(formData)
.then((res) => {
let obj = {
name: file.name,
data: res.data,
};
resolve(obj);
})
.catch((error) => {
reject();
});
} else {
const formData = new FormData();
formData.append("file", file);
formData.append("action", "up/permit/file");
TXUploadFile(formData)
.then((res) => {
let obj = {
name: file.name,
msg: res.msg,
code: res.code,
data: res.data,
};
resolve(obj);
})
.catch((error) => {
reject();
});
}
});
};
</script>
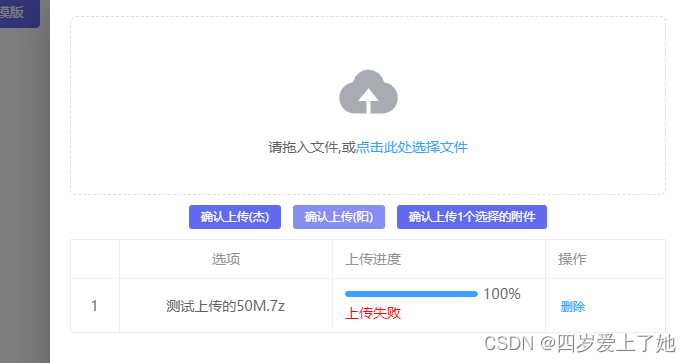
这个是一个批量切片上传文件的demo,切片大小自己选择,有100kb,500kb,1M三种切片规格
<template>
<el-upload
drag
ref="upload"
:auto-upload="false"
:show-file-list="false"
multiple
:file-list="fileList"
@change="handleChange"
>
<!-- <el-icon class="el-icon--upload"><upload-filled /></el-icon> -->
<div class="el-upload__text">请拖入文件,或<em>点击此处选择文件</em></div>
</el-upload>
<div
style="width: 100%; margin: 10px 0; display: flex; justify-content: center"
>
<el-button color="#36d" size="small" @click="upload_dataFileTwoList('0.1')"
>确认上传(0.1)</el-button
>
<el-button color="#36d" size="small" @click="upload_dataFileTwoList('0.5')"
>确认上传(0.5)</el-button
>
<el-button color="#36d" size="small" @click="upload_dataFileTwoList('1')"
>确认上传(1)</el-button
>
</div>
<el-table :data="fileList" style="width: 100%" max-height="250" border>
<el-table-column type="index" width="50" align="center" />
<el-table-column
prop="name"
label="选项"
show-overflow-tooltip
align="center"
/>
<el-table-column label="上传进度">
<template #default="scope">
<el-progress :percentage="scope.row.progress" />
<div v-if="scope.row.status === 'failed'">
<span style="color: red">上传失败</span>
</div>
<div v-if="scope.row.status === 'success'">
<span style="color: #49af2f">上传成功</span>
</div>
</template>
</el-table-column>
<el-table-column fixed="right" label="操作" width="120">
<template #default="scope">
<el-button
link
type="primary"
size="small"
@click.prevent="deleteRow(scope.$index)"
>
删除
</el-button>
</template>
</el-table-column>
</el-table>
</template>
<script setup>
import { ref, onMounted, nextTick } from "vue";
import { ElMessage, ElLoading } from "element-plus";
import axios from "axios";
const emit = defineEmits(["submitOK"]);
const baseURl_QiePian='xxxxxx.com'
const props = defineProps({
limit: {
type: Number,
default: 1,
},
upType: {
type: String,
default: "普通",
},
});
let queryParams = ref({
type: "普通",
});
const upload_dataFile = async () => {
let fileData = fileList.value[0];
let { name, size, raw: uploadfile } = fileData;
let index = 0;
let chunkSize = 0.1 * 1024 * 1024;
let startSize = 0;
startSize = chunkSize * index;
const promises = [];
while (startSize < size) {
let box = null;
if (startSize + chunkSize > size) {
box = uploadfile.slice(startSize, size);
} else {
box = uploadfile.slice(startSize, startSize + chunkSize);
}
startSize += chunkSize;
console.log(box);
let boxFile = new File([box], name);
let formData = new FormData();
formData.append("index", index);
formData.append("file", boxFile);
const promise = axios.post(baseURL_YANG, formData, {
headers: { "Content-type": "multipart/form-data" },
});
promises.push(promise);
index += 1;
}
try {
const responses = await Promise.all(promises);
console.log(responses);
} catch (error) {
console.error(error);
}
};
const upload_dataFileTwoList = async (val) => {
for (let i = 0; i < fileList.value.length; i++) {
let list = fileList.value[i];
let qiepian=0
if(val=='0.1'){
qiepian=0.1
}else if(val=='0.5'){
qiepian=0.5
}else{
qiepian=1
}
upload_FN(list,qiepian);
}
};
const upload_FN = async (FileItem,qiepian) => {
try {
let { name, size, raw: uploadfile} = FileItem;
let uploadedChunks = 0;
const chunkSize = qiepian * 1024 * 1024;
const totalChunks = Math.ceil(size / chunkSize);
const concurrency = 3;
const promises = [];
for (let index = 0; index < totalChunks; index++) {
const start = index * chunkSize;
const end = Math.min(start + chunkSize, size);
const chunk = uploadfile.slice(start, end);
const chunkFile = new File([chunk], name);
const formData = new FormData();
formData.append("sliceIndex", index);
formData.append("file", chunkFile);
formData.append("totalSlices", totalChunks);
formData.append("fileName", name);
formData.append("address", '测试');
const promise = axios.post(baseURl_QiePian, formData, {
headers: { "Content-type": "multipart/form-data" },
}).then((response) => {
uploadedChunks++;
FileItem.progress = Math.round((uploadedChunks / totalChunks) * 100);
if(FileItem.progress==100){
console.log('文件上传后在这进行文件路径赋值', FileItem.progress,FileItem.name)
}
});
promises.push(promise);
if (promises.length >= concurrency || index === totalChunks - 1) {
await Promise.all(promises)
promises.length = 0;
}
}
FileItem.status = "success";
} catch (error) {
console.error('上传失败', error);
FileItem.status = "failed";
}
};
onMounted(() => {
queryParams.value.type = props.upType;
console.log(queryParams.value.type);
});
const deleteRow = (val) => {
fileList.value.splice(val, 1);
};
const upload = ref(null);
const fileList = ref([]);
const handleChange = (file, e) => {
let list = e.map((item) => {
item.progress = 0;
item.status = "pending";
return item;
});
fileList.value = list;
};
</script>
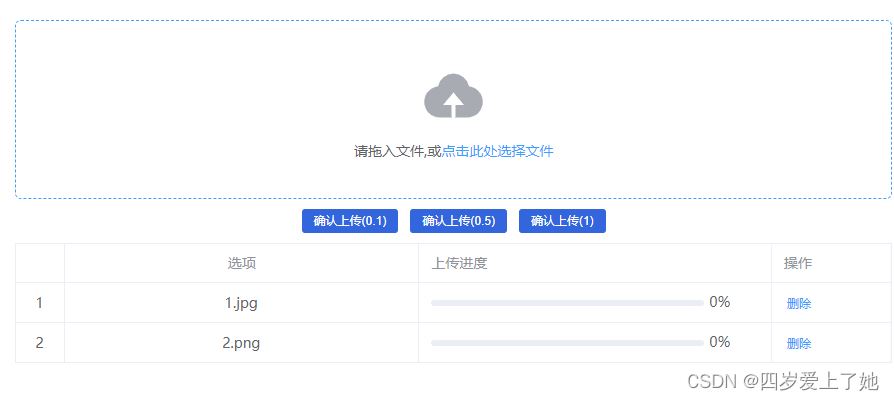