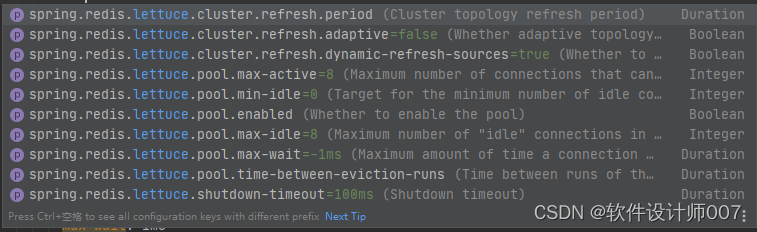
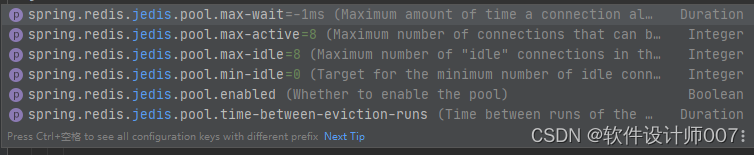
一、pom.xml配置
<!-- redis lettuce客户端(已集成)、RedisTemplate客户端(已集成) -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- jedis客户端(需要额外引入) -->
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.2.0</version>
</dependency>
二、application.properties配置
spring.redis.host=10.1.1.100
spring.redis.password=Abcd@1234
spring.redis.port=6379
# jedis pool setting
spring.redis.jedis.pool.max-active=20
spring.redis.jedis.pool.max-idle=10
spring.redis.jedis.pool.min-idle=5
三、Jedis单机使用
package com.leadpms.qianlistandard.web.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import redis.clients.jedis.Jedis;
/**
* Jedis单机调用
*
* @author Shaoyu Liu
* @date 2021/6/3 15:01
**/
@Configuration
public class JedisConfig {
private static final Jedis jedis = new Jedis("10.1.1.100", 6379);
static {
jedis.auth("Abcd@1234");
jedis.connect();
}
@Bean
public Jedis jedisFactory() {
return jedis;
}
}
package com.leadpms.qianlistandard.web.rest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import redis.clients.jedis.Jedis;
/**
* @author Shaoyu Liu
* @date 2021/6/3 13:43
**/
@RestController
@RequestMapping("/redis")
public class RedisController {
@Autowired
private Jedis jedis;
@GetMapping("/testRedis")
public void testRedis() {
jedis.set("jedis_k3", "v3");
}
}
四、JedisPool使用(Jedis连接池)
package com.leadpms.qianlistandard.web.config;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
/**
* Jedis线程池配置类
*
* @author Shaoyu Liu
* @date 2021/6/3 14:07
**/
@Configuration
public class JedisPoolConfiguration {
@Value("${spring.redis.host:127.0.0.1}")
private String host;
@Value("${spring.redis.port:6379}")
private Integer port;
@Value("${spring.redis.password:}")
private String password;
@Value("${spring.redis.database:0}")
private Integer database;
@Bean
public JedisPool redisPoolFactory() {
JedisPoolConfig jedisPoolConfig = new JedisPoolConfig();
JedisPool jedisPool = new JedisPool(jedisPoolConfig, host, port, 100, password);
return jedisPool;
}
}
package com.leadpms.qianlistandard.web.rest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
/**
* @author Shaoyu Liu
* @date 2021/6/3 13:43
**/
@RestController
@RequestMapping("/redis")
public class RedisController {
@Autowired
private JedisPool jedisPool;
@GetMapping("/testRedis")
public void testRedis() {
Jedis jedis = jedisPool.getResource();
jedis.set("jedisPool_v1", "k1");
}
}
文章参考
jedis和lettuce的对比 - 缘在此季 - 博客园