SQlite数据库 | 编写类继承SQLiteOpenHelper publicMySqlOpenHelper(Contextcontext){ //上下文数据库名结果集(游标)数据库版本 super(context,"sqlite.db",null,1); } MySqlOpenHelper helper=new MySqlOpenHelper(getApplicationContext()); //打开或者创建一个数据库。第一次就是创建 SQLiteDatabasedatabase1=helper.getWritableDatabase(); //如果磁盘满了,返回一个只读的 SQLiteDatabasedatabase2=helper.getReadableDatabase(); @Override public void onCreate(SQLiteDatabase db) { //数据库底层全是以string存,来提升性能; db.execSQL("create table info(_id integer primary key autoincrement,name varchar(20))"); } 版本跟换之后会执行适合做更新表的结构
增 SQLiteDatabase db=helper.getWritableDatabase(); ContentValues values=new ContentValues(); values.put("name", "dsy"); values.put("phone", "256521"); long l=db.insert("info", null, values); db.close(); | 删 SQLiteDatabase db=helper.getReadableDatabase(); //返回值代表影响的行数 int delete=db.delete("info", "name=?", new String[]{"dsy"}); db.close(); | 查 SQLiteDatabase db=helper.getWritableDatabase(); ContentValues values=new ContentValues(); values.put("phone", "123"); int update=db.update("info", values, "name=?", new String[]{"dsy"}); db.close(); | 改 SQLiteDatabase db=helper.getWritableDatabase(); //第二个参数null表示查询所有 Cursor cursor=db.query("info", new String[]{"phone"}, "name=?", new String[]{"dsy"}, null, null, null); if(cursor!=null&&cursor.getCount()>0){ while(cursor.moveToNext()){ System.out.println(cursor.getString(0)); } } db.close(); | SQLiteDatabase db=helper.getWritableDatabase(); //第二个参数null表示查询所有 Cursor cursor=db.query("info", new String[]{"phone"}, "name=?", new String[]{"dsy"}, null, null, null); if(cursor!=null&&cursor.getCount()>0){ while(cursor.moveToNext()){ System.out.println(cursor.getString(0)); } } db.close(); |
public void zz(View v){ db=helper.getWritableDatabase(); db.beginTransaction(); try { db.execSQL("update count set money=money+100 where name=?",new Object[]{"dsy"}); db.execSQL("update count set money=money-100 where name=?",new Object[]{"scy"}); db.setTransactionSuccessful(); } catch (Exception e) { Toast.makeText(getApplicationContext(), "服务器忙请稍后!", 1).show(); }finally{ db.endTransaction(); } } |
- 数据库事物操作
- 创建的数据库位于项目包名下的databases/
- 在命令行进入目录,使用sqlite3 数据库名字来查询
- 增删查改操作可以使用传统的语句操作,也可以使用一下API操作
- 创建数据库onUpgrade
- 创建数据库onCreate方法合适做表结构的初始化
- 创建需要写带Context的参数的构造方法
- 使用Sqlite
|
LitePal2.0数据库 | Android studio 需要在build.grade中修改一下配置,使用Eclipse需要导入一下jar包 <https://github.com/LitePalFramework/LitePal/blob/master/downloads/litepal-2.0.0.jar>
dependencies { implementation 'org.litepal.android:core:2.0.0' } |
- 在app/src/main 下新建assets文件夹,在文件夹中新建litepal.xml
<litepal> <dbname value=""></dbname> <version value="1"></version> <list> <mapping class="org.litepal.litepalsample.model.Book" /> </list> </litepal> |
- 编写实体类Book继承LitePalSupport作为映射
- 在maniFest的application中加入
android:name="org.litepal.LitePalApplication"
- 在Activity中直接调用Connector.getDatabase();
- 增删查改实体类需要继承LitePalSupport
增 对象.setXx() 对象.save() | 删 LitePalSupport.deleteAll(指定表名和约束); | 查 LitePalSupport.findAll(指定表名和约束); findFirst findLast LitePalSupport.select("name","price").find(指定表名); //查询指定列 LitePalSupport.where("page>?","200").find(指定表名); LitePalSupport.order("price desc").find(指定表名); LitePalSupport.limit(3).find(指定表名); LitePalSupport.limit(3).offset(1).find(指定表名); LitePalSupport.findBySQL("sql语句"); | 改 常规对象.set
- 对象.save()
- 对象.updateAll("name=? And author=?","a","b")
- 对象.setToDefault(“列名”) 对象.updateAll() 用来把字段值更新成默认值
|
|
ListView的基本用法 | 优化1:应为ListView 快速拖动会导致内存溢出 原因是应为消耗的内存大于释放的内存,所以需要使用缓存机制
@Override public View getView(finalint position, View convertView, ViewGroup parent) { ViewHolder holder; if (convertView == null) { convertView = mInflater.inflate(R.layout.item,null); holder = new ViewHolder(); /*得到各个控件的对象*/ holder.title = (TextView) convertView.findViewById(R.id.ItemTitle); holder.text = (TextView) convertView.findViewById(R.id.ItemText); holder.bt = (Button) convertView.findViewById(R.id.ItemButton); convertView.setTag(holder);//绑定ViewHolder对象 }else{ holder = (ViewHolder)convertView.getTag();//取出ViewHolder对象 } /*设置TextView显示的内容,即我们存放在动态数组中的数据*/ holder.title.setText(getDate().get(position).get("ItemTitle").toString()); holder.text.setText(getDate().get(position).get("ItemText").toString()); holder.bt.setOnClickListener(new OnClickListener() { @Override publicvoid onClick(View v) { }); return convertView; } |
优化2:ListView 的Layout_height需要使用 match-parent 使用wrap-content需要校验多次,运行更多次的getView()方法,效率就差了
- 设置监听 setOnItemClickListener
- 新建类继承BaseAdapter,实现构造函数 getCount getItem getItemId getView
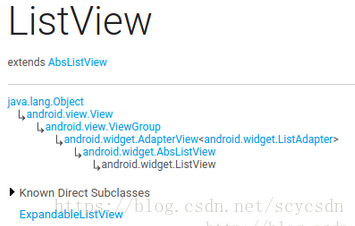 - 如何使用
- 在布局加入ListView控件
- 在Activity中初始化
- 设置数据
- lv.setAdapter(new ArrayAdapter<String>(this,android.R.layout.simple_list_item_1, strs));
- SimpleAdapter mSimpleAdapter = new SimpleAdapter(this,listItem,new String[] {"ItemImage","ItemTitle", "ItemText"},
|
inflater对象的获取三种方式 | View view=LayoutInflater.from(getApplicationContext()).inflate(R.layout.item,null); View view=View.inflate(getApplicationContext(), R.layout.item, null); View View=getSystemService(LAYOUT_INFLATER_SERVICE).inflate(R.layout.item,null); |