Android初学,转载的一些知识点,共同学习
[1].[图片] 程序截图
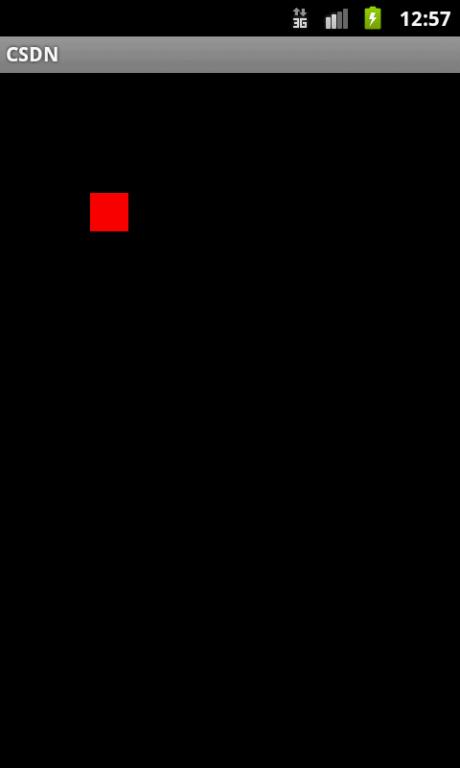
[2].[代码] Activity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
package
com.zhuozhuo;
import
android.app.Activity;
import
android.os.Bundle;
public
class
优快云Activity
extends
Activity {
/** Called when the activity is first created. */
@Override
public
void
onCreate(Bundle savedInstanceState) {
super
.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
|
[3].[代码] CustomView.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
|
package
com.zhuozhuo;
import
android.content.Context;
import
android.graphics.Canvas;
import
android.graphics.Color;
import
android.graphics.Paint;
import
android.graphics.Rect;
import
android.util.AttributeSet;
import
android.view.MotionEvent;
import
android.view.View;
/**
* 自定义的view,需要覆盖onDraw()方法绘制控件,覆盖onTouchEvent()接收触摸消息
*/
public
class
CustomView
extends
View {
private
static
final
int
WIDTH =
40
;
private
Rect rect =
new
Rect(
0
,
0
, WIDTH, WIDTH);
//绘制矩形的区域
private
int
deltaX,deltaY;
//点击位置和图形边界的偏移量
private
static
Paint paint =
new
Paint();
//画笔
public
CustomView(Context context, AttributeSet attrs) {
super
(context, attrs);
paint =
new
Paint();
paint.setColor(Color.RED);
//填充红色
}
@Override
protected
void
onDraw(Canvas canvas) {
canvas.drawRect(rect, paint);
//画矩形
}
@Override
public
boolean
onTouchEvent (MotionEvent event) {
int
x = (
int
) event.getX();
int
y = (
int
) event.getY();
switch
(event.getAction()) {
case
MotionEvent.ACTION_DOWN:
if
(!rect.contains(x, y)) {
return
false
;
//没有在矩形上点击,不处理触摸消息
}
deltaX = x - rect.left;
deltaY = y - rect.top;
break
;
case
MotionEvent.ACTION_MOVE:
case
MotionEvent.ACTION_UP:
Rect old =
new
Rect(rect);
//更新矩形的位置
rect.left = x - deltaX;
rect.top = y - deltaY;
rect.right = rect.left + WIDTH;
rect.bottom = rect.top + WIDTH;
old.union(rect);
//要刷新的区域,求新矩形区域与旧矩形区域的并集
invalidate(old);
//出于效率考虑,设定脏区域,只进行局部刷新,不是刷新整个view
break
;
}
return
true
;
//处理了触摸消息,消息不再传递
}
}
|
[4].[代码] main.xml 布局文件
1
2
3
4
5
6
7
8
9
|
<?
xml
version
=
"1.0"
encoding
=
"utf-8"
?>
<
LinearLayout
xmlns:android
=
"http://schemas.android.com/apk/res/android"
android:orientation
=
"vertical"
android:layout_width
=
"fill_parent"
android:layout_height
=
"fill_parent"
>
<
com.zhuozhuo.CustomView
android:layout_width
=
"fill_parent"
android:layout_height
=
"fill_parent"
/>
</
LinearLayout
>
|