1.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<!--配置spring创建IOC容器时要扫描的包-->
<context:component-scan base-package="test10month.test1016"/>
<!--配置数据源-->
<bean id="druidRuntimeException" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/rod"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</bean>
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="druidRuntimeException"/>
</bean>
<!--配置aop-->
<aop:aspectj-autoproxy />
</beans>
2.操作数据库类-接口
public interface Affair {
void update(int money,int idadd,int reduce);
}
@Repository
public class AffairImp implements Affair {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public void update(int money, int idadd, int idreduce) {
String sqladd = "update customer set money=money+? where id=?";
Object[] objadd = {money, idadd};
jdbcTemplate.update(sqladd, objadd);
String sqlreduce = "update customer set money=money-? where id=?";
Object[] objreduce = {money, idreduce};
jdbcTemplate.update(sqlreduce, objreduce);
}
}
3.Service----服务类:
@Service
public class AffirService {
@Autowired
private AffairImp affairImp;
public void transaction(int money, int idadd, int idreduce) {
affairImp.update(money, idadd, idreduce);
}
}
4.测试类
package test10month.test1016.affair;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class AffairTest {
public static void main(String[] args) {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("test10month/test1016/jdbc.xml");
AffirService affirService = context.getBean("affirService", AffirService.class);
affirService.transaction(100,1,2);
}
}
5.结果
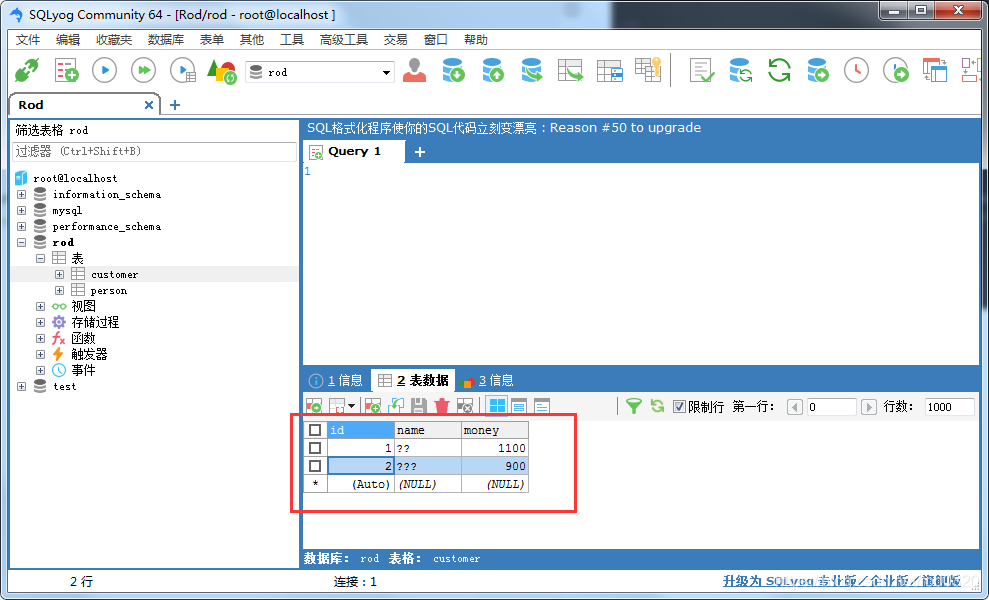