题目地址:天梯赛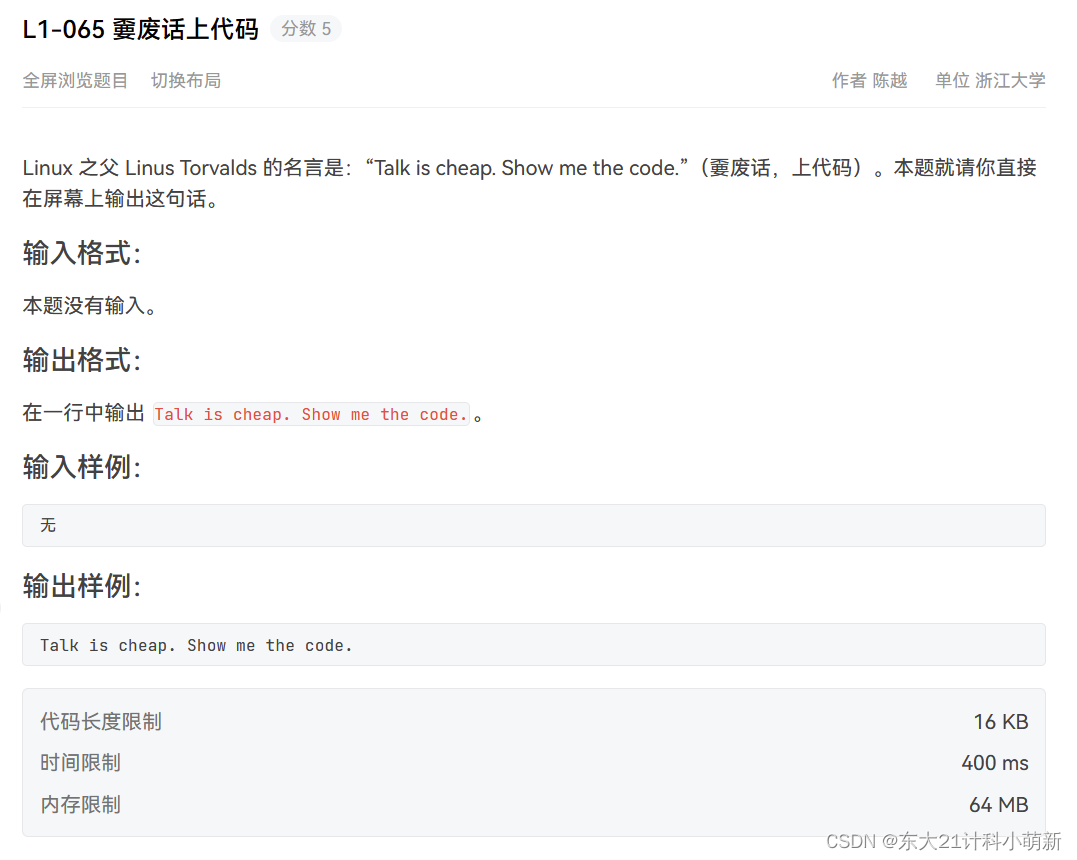
#include<bits/stdc++.h>
using namespace std;
int main() {
puts("Talk is cheap. Show me the code.");
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int a, b, c;
int main() {
cin >> a >> b >> c;
cout << a * b * c;
return 0;
}
#include<bits/stdc++.h>
using namespace std;
double a, b, c;
int main() {
cin >> a >> b >> c;
b = b ? 1.26 : 2.455;
printf("%.2f ", a * b);
cout << (a * b > c ? "T_T" : "^_^");
return 0;
}
#include<bits/stdc++.h>
using namespace std;
double n, cnt, x;
int main() {
cin >> n;
for(int i = 0; i < n; ++i) {
cin >> x, cnt += 1 / x;
}
printf("%.2f", 1 / (cnt / n));
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int a[6], cnt, maxx, check;
int main() {
for(int i = 0; i < 6; ++i) {
cin >> a[i];
if(i < 4) maxx = max(a[i], maxx);
}
for(int i = 0; i < 4; ++i) {
if(maxx - a[i] > a[5] || a[i] < a[4]) ++cnt, check = i;
}
if(!cnt) puts("Normal");
else if(cnt < 2) printf("Warning: please check #%d!", check + 1);
else puts("Warning: please check all the tires!");
return 0;
}
#include<bits/stdc++.h>
using namespace std;
string s, check = "chi1 huo3 guo1";
int n, flag, cnt;
int main() {
while(getline(cin, s)) {
if(s == ".") {
cout << n << endl;
if(!flag) cout << "-_-#";
else cout << flag << " " << cnt;
return 0;
}
++n;
if(s.find(check) != s.npos) {
if(!flag) flag = n;
++cnt;
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n, m;
string s;
int main() {
cin >> n >> m;
while(m--) {
cin >> s;
int cnt = pow(2, n), index = 1;
for(int i = 0; i < n; ++i) {
cnt /= 2;
if(s[i] == 'n') index += cnt;
}
cout << index << endl;
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int a[3][3], x, y, cnt = 1 ^ 2 ^ 3 ^ 4 ^ 5 ^ 6 ^ 7 ^ 8 ^ 9, ans;
int t[25] = {0, 0, 0, 0, 0, 0, 10000, 36, 720, 360, 80, 252, 108, 72, 54, 180, 72, 180, 119, 36, 306, 1080, 144, 1800, 3600};
int main() {
for(int i = 0; i < 3; ++i) {
for(int j = 0; j < 3; ++j) {
cin >> a[i][j], cnt ^= a[i][j];
}
}
for(int i = 0; i < 3; ++i) {
cin >> x >> y;
cout << a[--x][--y] << endl;
}
cin >> x;
if(x <= 3) {
for(int i = 0; i < 3; ++i) {
ans += a[x - 1][i];
if(!a[x - 1][i]) ans += cnt;
}
} else if(x <= 6) {
for(int i = 0; i < 3; ++i) {
ans += a[i][x - 4];
if(!a[i][x - 4]) ans += cnt;
}
} else if(x == 7) {
for(int i = 0; i < 3; ++i) {
ans += a[i][i];
if(!a[i][i]) ans += cnt;
}
} else {
for(int i = 0; i < 3; ++i) {
ans += a[2 - i][i];
if(!a[2 - i][i]) ans += cnt;
}
}
cout << t[ans];
return 0;
}
#include <bits/stdc++.h>
using namespace std;
int n, x;
stack<int> num;
stack<char> op;
char c;
void calculate() {
int num_2 = num.top(); num.pop();
int num_1 = num.top(); num.pop();
c = op.top(); op.pop();
switch(c) {
case '+':{
num.push(num_1 + num_2);
break;
}
case '-':{
num.push(num_1 - num_2);
break;
}
case '*':{
num.push(num_1 * num_2);
break;
}
case '/':{
if(!num_2){
printf("ERROR: %d/0", num_1);
exit(0);
}
num.push(num_1 / num_2);
break;
}
}
}
int main() {
cin >> n;
for(int i = 0; i < n; ++i) {
cin >> x, num.push(x);
}
for(int i = 0; i < n - 1; ++i) {
getchar(), c = getchar(), op.push(c);
}
while(!op.empty()) {
calculate();
}
cout << num.top();
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int d, p, t, s;
struct node {
string name, id;
int x, hh, mm, i, d;
}person;
unordered_map<string, int> mp;
unordered_set<string> st;
vector<pair<string, string>> ans;
bool check(string& s) {
if(s.length() != 18) return false;
for(auto& x : s) {
if(!isdigit(x)) return false;
}
return true;
}
int main(){
cin >> d >> p;
for(int k = 0; k < d; ++k) {
cin >> t >> s;
vector<node> mid;
for(int i = 0; i < t; ++i) {
cin >> person.name >> person.id >> person.x;
scanf("%d:%d", &person.hh, &person.mm);
person.i = i, person.d = k;
if(check(person.id)) mid.emplace_back(person);
else continue;
if(person.x && !st.count(person.id)) {
ans.emplace_back(person.name, person.id), st.emplace(person.id);
}
}
sort(mid.begin(), mid.end(), [](node A, node B){
return A.hh < B.hh || A.hh == B.hh && A.mm < B.mm || A.hh == B.hh && A.mm == B.mm && A.i < B.i;
});
int cnt = 0;
for(int i = 0; i < mid.size() && cnt < s; ++i) {
if(!mp.count(mid[i].id) || mp[mid[i].id] + p < k) {
mp[mid[i].id] = k;
cout << mid[i].name << ' ' << mid[i].id << '\n';
++cnt;
}
}
}
for(auto& [key, value] : ans) {
cout << key << ' ' << value << '\n';
}
return 0;
}
#include <bits/stdc++.h>
using namespace std;
int n, j = 1;
int a[35];
void build(int x) {
if(x > n) return;
build(x << 1), build((x << 1) + 1);
cin >> a[x];
}
int main() {
cin >> n;
build(1);
for(int i = 1; i <= n; ++i) {
cout << (i == 1 ? "" : " ") << a[i];
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n, m, a, b, cnt[505];
vector<int> road[505];
bool check = true;
int main() {
cin >> n >> m;
while(m--) {
cin >> a >> b;
road[a].push_back(b);
}
cin >> a >> b;
cnt[b] = 1;
function<void(int)> dfs = [&](int x) {
if(cnt[x]) return cnt[x];
if(road[x].empty()) check = false;
int res = 0;
for(auto& y : road[x]) {
if(!cnt[y]) dfs(y);
res += cnt[y];
}
return cnt[x] = res;
};
dfs(a);
cout << cnt[a] << " " << (check ? "Yes" : "No");
return 0;
}