题目地址:天梯赛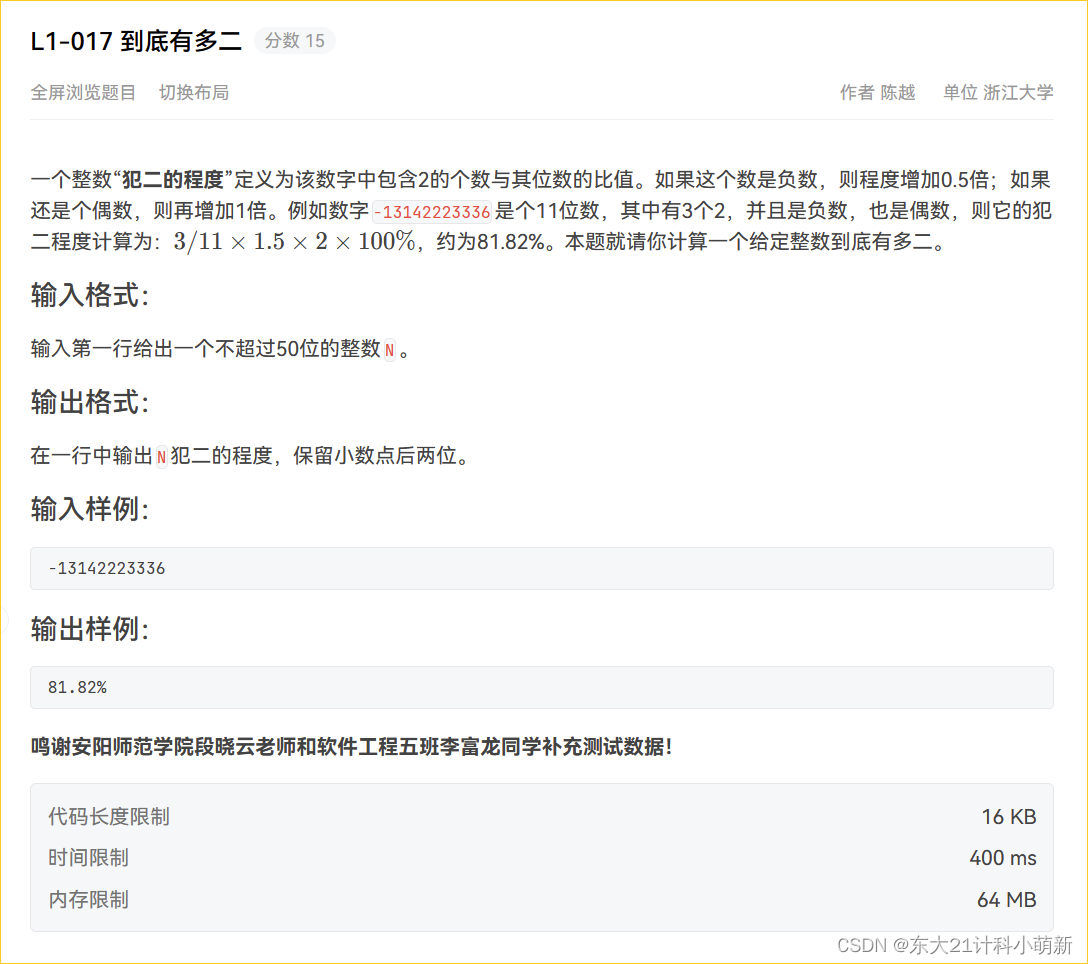
#include<bits/stdc++.h>
using namespace std;
int two;
string s;
double ans;
int main() {
cin >> s;
for(auto& x : s) {
if(x == '2') ++two;
}
if(s[0] == '-') ans = (double)two / (s.length() - 1) * 1.5;
else ans = double(two) / s.length();
if(!((s.back() - '0') % 2)) ans *= 2;
printf("%.2f%%", ans * 100);
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int hh, mm;
int main() {
scanf("%d:%d", &hh, &mm);
if(hh <= 11 || hh == 12 && mm == 0) {
printf("Only %02d:%02d. Too early to Dang.", hh, mm);
} else {
hh -= 12;
if(mm) ++hh;
for(int i = 0; i < hh; ++i) {
cout << "Dang";
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int jia, yi, n, jia_hua, yi_hua, jia_han, yi_han, jia_jiu, yi_jiu;
int main() {
cin >> jia_jiu >> yi_jiu >> n;
for(int i = 0; i < n; ++i) {
cin >> jia_han >> jia_hua >> yi_han >> yi_hua;
if(jia_han + yi_han == jia_hua && jia_hua != yi_hua) ++jia;
if(jia_han + yi_han == yi_hua && jia_hua != yi_hua) ++yi;
if(jia > jia_jiu) {
cout << "A" << endl << yi;
return 0;
}
if(yi > yi_jiu) {
cout << "B" << endl << jia;
return 0;
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n, k;
unordered_set<string> st;
string s;
int main() {
cin >> n;
for(int i = 0; i < n; ++i) {
cin >> k;
for(int j = 0; j < k; ++j) {
cin >> s;
if(k > 1) st.insert(s);
}
}
cin >> n;
k = 0;
for(int i = 0; i < n; ++i) {
cin >> s;
if(!st.count(s)) {
cout << (k++ ? " " : "") << s;
st.insert(s);
}
}
if(!k) cout << "No one is handsome";
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int main() {
for(int i = 0; i < 3; ++i) {
puts("I'm gonna WIN!");
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n, x, ans;
int main() {
cin >> n;
for(int i = 0; i < n; ++i) {
cin >> x;
if(x & 1) ++ans;
}
cout << ans << " " << n - ans;
return 0;
}
#include<bits/stdc++.h>
using namespace std;
string s;
unordered_map<char, int> mp;
int cnt, i;
char f[] = "GPLT";
int main() {
cin >> s;
for(auto& x : s) {
x = toupper(x), ++mp[x];
if(x == 'G' || x == 'P' || x == 'L' || x == 'T') ++cnt;
}
while(cnt > 0) {
if(mp[f[i]] > 0) {
cout << f[i];
--mp[f[i]], --cnt;
}
++i;
if(i >= 5) i = 0;
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n;
int main() {
cin >> n;
cout << ((n += 2) > 7 ? n -= 7 : n);
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n, k, a, b;
const int N = 1e4 + 5;
struct node{
int id, cnt, sizes;
}p[N];
int main() {
cin >> n;
for(int i = 1; i <= n; ++i) {
p[i].id = i, p[i].cnt = 0, p[i].sizes = 0;
}
for(int i = 1; i <= n; ++i) {
cin >> k;
int sum = 0;
for(int j = 0; j < k; ++j) {
cin >> a >> b;
p[a].cnt += b, ++p[a].sizes, sum += b;
}
p[i].cnt -= sum;
}
sort(p + 1, p + n + 1, [](node A, node B) {
if(A.cnt == B.cnt) {
if(A.sizes == B.sizes) return A.id < B.id;
return A.sizes > B.sizes;
} else {
return A.cnt > B.cnt;
}
});
for(int i = 1; i <= n; ++i) {
printf("%d %.2f\n", p[i].id, (double)p[i].cnt / 100);
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
int n, m, k, p1, p2, relationship;
int f[105], a[105][105];
int find(int x) {
return f[x] == x ? x : f[x] = find(f[x]);
}
int main() {
cin >> n >> m >> k;
for(int i = 1; i <= n; ++i) {
f[i] = i;
}
while(m--) {
cin >> p1 >> p2 >> relationship;
a[p1][p2] = a[p2][p1] = relationship;
if(relationship == 1) {
p1 = find(p1), p2 = find(p2);
if(p1 != p2) {
f[p1] = p2;
}
}
}
while(k--) {
cin >> p1 >> p2;
if(a[p1][p2] == -1) {
cout << (find(p1) == find(p2) ? "OK but..." : "No way") << endl;
} else {
cout << (find(p1) == find(p2) ? "No problem" : "OK") << endl;
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
vector<int> pre_order, in_order;
int n, x;
unordered_map<int, int> mp;
unordered_map<int, pair<int, int>>Tree;
queue<int> Q;
int build(int l_p, int r_p, int l_i, int r_i) {
if(l_p > r_p || l_i > r_i) return -1;
int pre_root = l_p, in_root = mp[pre_order[l_p]], cnt = in_root - l_i;
Tree[pre_order[l_p]] = {build(l_p + 1, l_p + cnt, l_i, in_root - 1), build(l_p + cnt + 1, r_p, in_root + 1, r_i)};
return pre_order[l_p];
}
void bfs(int x) {
cout << x;
if(Tree[x].second != -1) Q.push(Tree[x].second);
if(Tree[x].first != -1) Q.push(Tree[x].first);
while(!Q.empty()) {
x = Q.front();
Q.pop();
cout << " " << x;
if(Tree[x].second != -1) Q.push(Tree[x].second);
if(Tree[x].first != -1) Q.push(Tree[x].first);
}
}
int main() {
cin >> n;
for(int i = 0; i < n; ++i) {
cin >> x;
in_order.emplace_back(x), mp.insert({x, i});
}
for(int i = 0; i < n; ++i) {
cin >> x;
pre_order.emplace_back(x);
}
int root = build(0, n-1, 0, n-1);
bfs(root);
return 0;
}
`
#include<bits/stdc++.h>
using namespace std;
int n, m, x, y;
string s;
vector heap(0, 0);
map<int, int> mp;
int main() {
cin >> n >> m;
for(int i = 0; i < n; ++i) {
cin >> x;
heap.push_back(x), make_heap(heap.begin(), heap.end(), greater<int>());
}
for(int i = 0; i < n; ++i) {
mp.insert({heap[i], i + 1});
}
while(m--) {
cin >> x >> s;
if(s[0] == 'a') {
cin >> y >> s >> s;
cout << (mp[x] / 2 == mp[y] / 2 ? "T" : "F") << endl;
} else {
cin >> s >> s;
if(s[0] == 'r') {
cout << (mp[x] == 1 ? "T" : "F") << endl;
} else if (s[0] == 'p') {
cin >> s >> y;
cout << (mp[x] == mp[y] / 2 ? "T" : "F") << endl;
} else{
cin >> s >> y;
cout << (mp[x] / 2 == mp[y] ? "T" : "F") << endl;
}
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
const int N = 505;
int n, m, u, v, one_way, len, t;
struct node {
int pos, len, time, cnt, from;
};
vector<node> a[N];
vector<int> road[2];
int res[N], ans[2], from[N];
class cmp_dis {
public:
bool operator () (const node& n1, const node& n2) {
return n1.len > n2.len || n1.len == n2.len && n1.cnt > n2.cnt;
}
};
class cmp_time {
public:
bool operator () (const node& n1, const node& n2) {
return n1.time > n2.time || n1.time == n2.time && n1.len > n2.len;
}
};
void dij(int op) {
priority_queue<node, vector<node>, function<bool(const node&, const node&)>> Q;
if (op == 1) Q = priority_queue<node, vector<node>, function<bool(const node&, const node&)>>(cmp_dis());
else Q = priority_queue<node, vector<node>, function<bool(const node&, const node&)>>(cmp_time());
for (int i = 0; i <= n; ++i) {
from[i] = -1, res[i] = 0x7f7f7f;
}
Q.push({u, 0, 0, 0, -1});
while (!Q.empty()) {
auto x = Q.top();
Q.pop();
int mid = (op == 1 ? x.len : x.time);
if (mid < res[x.pos]) {
res[x.pos] = mid, from[x.pos] = x.from;
for (auto& xx : a[x.pos]) {
Q.push({xx.pos, xx.len + x.len, xx.time + x.time, x.cnt + 1, x.pos});
}
}
}
ans[op] = res[v];
function<void(int)> print = [&](int x) {
road[op].push_back(x);
if (from[x] != -1) print(from[x]);
};
print(v);
}
int main() {
cin >> n >> m;
while (m--) {
cin >> u >> v >> one_way >> len >> t;
a[u].push_back({v, len, t, 0, u});
if (!one_way) a[v].push_back({u, len, t, 0, v});
}
cin >> u >> v;
for (int i = 0; i <= 1; ++i) {
dij(i);
}
if (road[0] != road[1]) {
cout << "Time = " << ans[0] << ": ";
for (int i = road[0].size() - 1; i >= 0; --i) {
cout << road[0][i] << (i ? " => " : "\n");
}
cout << "Distance = " << ans[1] << ": ";
for (int i = road[1].size() - 1; i >= 0; --i) {
cout << road[1][i] << (i ? " => " : "\n");
}
} else {
cout << "Time = " << ans[0] << "; Distance = " << ans[1] << ": ";
for (int i = road[0].size() - 1; i >= 0; --i) {
cout << road[0][i] << (i ? " => " : "\n");
}
}
return 0;
}
#include<bits/stdc++.h>
using namespace std;
const int N = 10005;
int n, m, k, a, b, res;
vector<int> road[N];
bool vis[N];
int main() {
cin >> n >> m >> k;
while(m--) {
cin >> a >> b;
road[a].push_back(b), road[b].push_back(a);
}
while(k--) {
cin >> a;
if(road[a].empty()) {
cout << 0 << endl;
} else {
memset(vis, false, sizeof(vis));
queue<int> Q;
Q.push(a);
vis[a] = true;
while(!Q.empty()) {
int k = Q.size();
res = Q.front();
for(int i = 0; i < k; ++i) {
int x = Q.front();
Q.pop();
res = min(res, x);
for(int j = 0; j < (int)road[x].size(); ++j) {
if(!vis[road[x][j]]) {
Q.push(road[x][j]);
vis[road[x][j]] = true;
}
}
}
}
cout << res << endl;
}
}
return 0;
}