系统需求
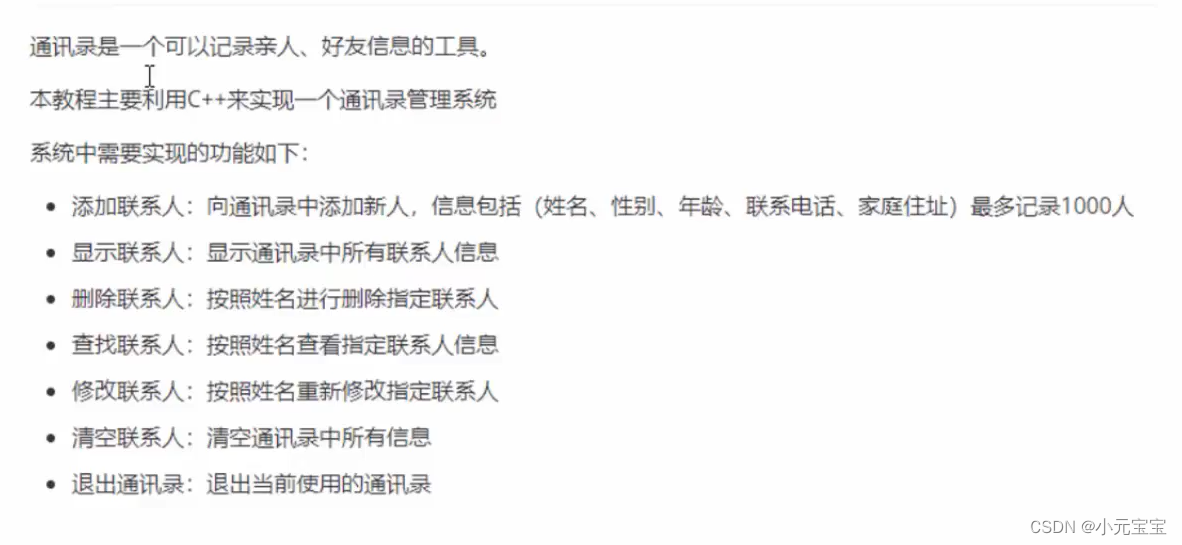
代码
#include<iostream>
#include<string>
#define MAX 1000
using namespace std;
struct Person
{
string m_name;
int m_sex;
int m_age;
string m_phone;
string m_address;;
};
struct AddressBook {
Person personArray[MAX];
int currentNum;
};
void ShowMenu() {
cout << "************************" << endl;
cout << "******1.添加联系人******" << endl;
cout << "******2.显示联系人******" << endl;
cout << "******3.删除联系人******" << endl;
cout << "******4.查找联系人******" << endl;
cout << "******5.修改联系人******" << endl;
cout << "******6.清空联系人******" << endl;
cout << "******0.退出通讯录******" << endl;
cout << "************************" << endl;
}
void addPerson(AddressBook* p_book) {
if (p_book->currentNum == MAX) {
cout << "通讯录已存满,添加失败" << endl;
return;
}
else {
cout << "请输入联系人的姓名" << endl;
cin >> p_book->personArray[p_book->currentNum].m_name;
int sex = 0;
while (sex!=1 && sex!=2)
{
cout << "请输入联系人的性别(1:男/2:女)" << endl;
cin >> sex;
}
p_book->personArray[p_book->currentNum].m_sex = sex;
int age = 0;
do
{
cout << "请输入联系人的年龄" << endl;
cin >> age;
} while (age<=0 || age>150);
p_book->personArray[p_book->currentNum].m_age = age;
cout << "请输入联系人的电话" << endl;
cin >> p_book->personArray[p_book->currentNum].m_phone;
cout << "请输入联系人的地址" << endl;
cin >> p_book->personArray[p_book->currentNum].m_address;
cout << "添加成功" << endl;
p_book->currentNum++;
system("pause");
system("cls");
}
}
void showPerson(AddressBook* p_book) {
if (p_book->currentNum == 0) {
cout << "通讯录为空" << endl;
system("pause");
system("cls");
return;
}
else {
for (int i = 0; i < p_book->currentNum; i++) {
cout << "姓名:" << p_book->personArray[i].m_name
<< "\t性别:" << (p_book->personArray[i].m_sex==1?"男":"女")
<< "\t年龄:" << p_book->personArray[i].m_age
<< "\t电话:" << p_book->personArray[i].m_phone
<< "\t住址:" << p_book->personArray[i].m_address << endl;
}
system("pause");
system("cls");
}
}
int isExist(AddressBook* p_book, string name) {
for (int i = 0; i < p_book->currentNum; i++) {
if (p_book->personArray[i].m_name == name) {
return i;
}
}
return -1;
}
void deletePerson(AddressBook* p_book) {
string name;
cout << "请输入要删除的联系人" << endl;
cin >> name;
int index = isExist(p_book, name);
if (index == -1) {
cout << "查无此人" << endl;
system("pause");
system("cls");
return;
}
else {
for (int i = index; i < p_book->currentNum; i++) {
p_book->personArray[i] = p_book->personArray[i + 1];
}
p_book->currentNum--;
cout << "删除成功" << endl;
system("pause");
system("cls");
}
}
void searchPerson(AddressBook* p_book) {
string name;
cout << "请输入待查找的联系人的姓名" << endl;
cin >> name;
int index = isExist(p_book, name);
if (index != -1) {
cout << "查找成功" << endl;
cout << "姓名:" << p_book->personArray[index].m_name
<< "\t性别:" << (p_book->personArray[index].m_sex == 1 ? "男" : "女")
<< "\t年龄:" << p_book->personArray[index].m_age
<< "\t电话:" << p_book->personArray[index].m_phone
<< "\t住址:" << p_book->personArray[index].m_address << endl;
}
else {
cout << "查无此人" << endl;
}
system("pause");
system("cls");
}
void modifyPerson(AddressBook* p_book) {
string name;
cout << "请输入待修改的联系人的姓名" << endl;
cin >> name;
int index = isExist(p_book, name);
if (index != -1) {
cout << "请输入修改后联系人的姓名" << endl;
cin >> p_book->personArray[index].m_name;
cout << "请输入修改后联系人的性别(1男2女)" << endl;
int sex = 0;
while (true) {
cin >> sex;
if (sex == 1 || sex == 2) {
p_book->personArray[index].m_sex = sex;
break;
}
cout << "请输入正确的性别" << endl;
}
cout << "请输入修改后联系人的年龄" << endl;
cin >> p_book->personArray[index].m_age;
cout << "请输入修改后联系人的电话" << endl;
cin >> p_book->personArray[index].m_phone;
cout << "请输入修改后联系人地址" << endl;
cin >> p_book->personArray[index].m_address;
cout << "修改成功" << endl;
}
else {
cout << "查无此人" << endl;
}
system("pause");
system("cls");
}
void cleanPerson(AddressBook* p_book) {
cout << "是否确认清空(y/n)" << endl;
char select;
cin >> select;
if (select == 'y') {
p_book->currentNum = 0;
cout << "清空成功" << endl;
}
else if (select == 'n') {
cout << "退出清空界面" << endl;
system("pause");
system("cls");
return;
}
else {
cout << "输入错误,请重新输入" << endl;
}
system("pause");
system("cls");
}
int main() {
int select = 0;
AddressBook book;
book.currentNum = 0;
while (true) {
ShowMenu();
cin >> select;
switch (select)
{
case 1:
addPerson(&book);
break;
case 2:
showPerson(&book);
break;
case 3:
deletePerson(&book);
break;
case 4:
searchPerson(&book);
break;
case 5:
modifyPerson(&book);
break;
case 6:
cleanPerson(&book);
break;
case 0:
cout << "欢迎下次使用" << endl;
system("pause");
return 0;
default:
break;
}
}
}