1、得到当前时间
const date = new Date()
console.log(date) //Thu Jun 15 2023 15:50:25 GMT+0800 (中国标准时间)
2、得到指定时间
const date1=new Date('2023-6-17 12:00:00')
3、 日期对象方法
目标:能够使用日期对象中的方法写出常见日期
使用场景:因为日期对象返回的数据我们不能直接使用,所以需要转换为实际开发中常用的格式
const date = new Date()
console.log(date.getFullYear()) //2023
console.log(date.getDate()) //15
console.log(date.toLocaleString()) // 2023/6/15 17:33:50
console.log(date.toLocaleDateString()) // 2023/6/15
console.log(date.toLocaleTimeString()) // 2023/6/15
4、时间戳
使用场景: 如果计算倒计时效果,前面方法无法直接计算,需要借助于时间戳完成
什么是时间戳:
是指1970年01月01日00时00分00秒起至现在的毫秒数,它是一种特殊的计量时间的方式算法:
1)将来的时间戳-现在的时间戳=剩余时间毫秒数
2)剩余时间毫秒数 转换为 剩余时间的 年月日时分秒 就是 倒计时时间
3)比如 将来时间戳 2000ms- 现在时间戳1000ms =1000ms
4)1000ms转换为就是0小时0分1秒
5、倒计时案例
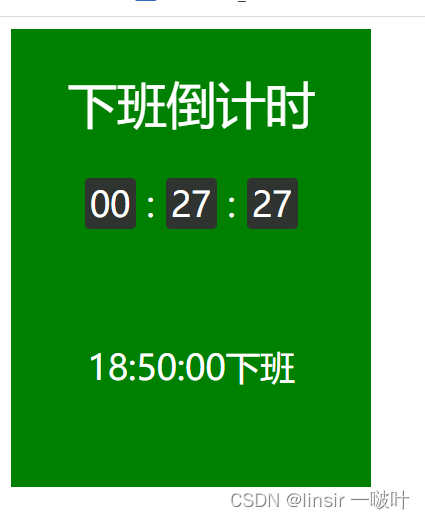
<style>
.countdown {
width: 240px;
height: 305px;
text-align: center;
line-height: 1;
color: #fff;
background-color: green;
/* 解决塌陷问题 */
overflow: hidden;
}
.countdown .title {
font-size: 33px;
}
.countdown .tips {
/* 使父元素外边距塌陷 */
margin-top: 80px;
font-size: 23px;
}
.countdown small {
font-size: 17px;
}
.countdown .clock {
width: 142px;
margin: auto;
/* 清除浮动 */
overflow: hidden;
}
.countdown .clock span,
.countdown .clock i {
display: block;
text-align: center;
line-height: 34px;
font-size: 23px;
float: left;
}
.countdown .clock span {
width: 34px;
height: 34px;
border-radius: 3px;
background-color: #303430;
}
.countdown .clock i {
width: 20px;
font-style: normal;
}
</style>
</head>
<body>
<div class="countdown">
<p class="title">下班倒计时</p>
<p class="clock">
<span id="hour">00</span>
<i>:</i>
<span id="minutes">00</span>
<i>:</i>
<span id="scond">00</span>
</p>
<p class="tips">18:50:00下班</p>
</div>
<script>
function getCountTime() {
// 得到当前的时间戳
const now = +new Date()
// 得到将来的时间戳
const last = +new Date('2023-6-15 18:50:00')
// 得到剩余的时间戳
const count = (last - now) / 1000
// 转换为 时分秒
let h = parseInt(count / 60 / 60 % 24)
h = h < 10 ? '0' + h : h
let m = parseInt(count / 60 % 60)
m = m < 10 ? '0' + m : m
let s = parseInt(count % 60)
s = s < 10 ? '0' + s : s
console.log(h, m, s)
document.querySelector('#hour').innerHTML = h
document.querySelector('#minutes').innerHTML = m
document.querySelector('#scond').innerHTML = s
}
// 先调用一次
getCountTime()
// 再开启定时器
setInterval(getCountTime, 1000)
</script>
</body>