普通插槽
<style>
.app{
background-color: rgba(0,255,255,0.5);
padding-bottom: 10px;
}
.my_Template{
background-color: rgba(0,0,0,0.2);
}
</style>
<!-- 1.创建容器 -->
<div class="app">
<h1>我是父组件</h1>
<hr>
<!-- 7.应用子组件-->
<my-Component>
<h5>我是1号子组件插槽的内容</h5>
</my-Component>
<hr>
<my-Component>
<!-- <h1>我是2号子组件插槽内容</h1> -->
</my-Component>
</div>
<!-- 3.创建模板 -->
<template id="my_Template">
<div class="my_Template">
<h2>我是子组件</h2>
<!-- 8.定义插槽 -->
<slot></slot>
</div>
</template>
<script>
// 2.创建vue实例
const vm = new Vue({
el:'.app',
// 8.给父组件添加数据
data:{
num:5,
},
// 4.创建局部组件
components:{
// 5.创建子组件
'myComponent':{
// 6.关联模板
template:'#my_Template'
}
}
})
</script>
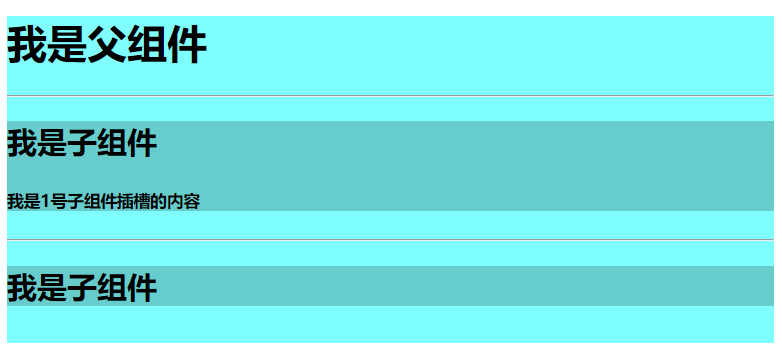
默认值插槽
<style>
.app{
background-color: rgba(0,255,255,0.5);
padding-bottom: 10px;
}
.my_Template{
background-color: rgba(0,0,0,0.2);
}
</style>
<!-- 1.创建容器 -->
<div class="app">
<h1>我是父组件</h1>
<hr>
<!-- 7.应用子组件-->
<my-Component>
<!-- 9.填写子组件插槽内容(可选),如果是有默认插槽,优先使用子组件内设置的插槽内容 -->
<h5>我是1号子组件插槽的内容,我会覆盖插槽的默认内容</h5>
</my-Component>
<hr>
<my-Component>
<!-- <h1>我是子组件2插槽内容</h1> -->
</my-Component>
</div>
<!-- 3.创建模板 -->
<template id="my_Template">
<div class="my_Template">
<h2>我是子组件</h2>
<!-- 8.定义有默认值的插槽 -->
<slot>
<h5>我是插槽默认值</h5>
</slot>
</div>
</template>
<script>
// 2.创建vue实例
const vm = new Vue({
el:'.app',
// 8.给父组件添加数据
data:{
num:5,
},
// 4.创建局部组件
components:{
// 5.创建子组件
'myComponent':{
// 6.关联模板
template:'#my_Template'
}
}
})
</script>
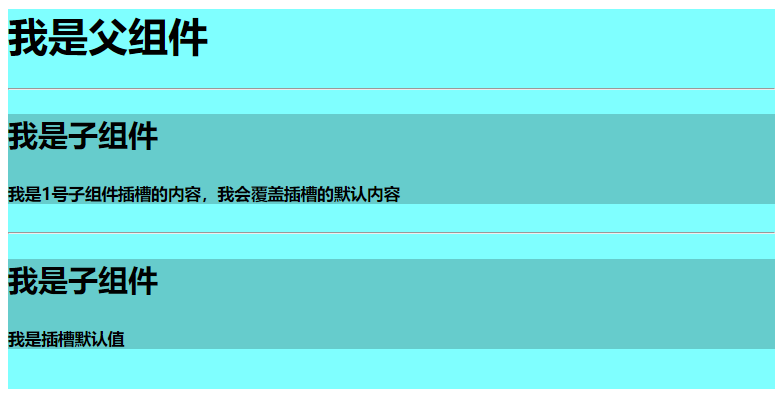
具名插槽
<style>
.app{
background-color: rgba(0,255,255,0.5);
padding-bottom: 10px;
}
.my_Template{
background-color: rgba(0,0,0,0.2);
}
</style>
<!-- 1.创建容器 -->
<div class="app">
<h1>我是父组件</h1>
<hr>
<!-- 7.应用子组件 -->
<my-Component >
<!-- 9.填写子组件插槽内容(可选),如果是有默认插槽,优先使用子组件内设置的插槽内容 -->
<div slot="slot1">
<h4>我是替换名为slot1插槽的内容</h4>
</div>
<div slot="slot2">
<h4>我是替换名为slot2插槽的内容</h4>
</div>
<h1>我是子组件插槽的内容,不会在子组件显示</h1>
</my-Component>
<hr>
</div>
<!-- 3.创建模板 -->
<template id="my_Template">
<div class="my_Template">
<h3>我是子组件</h3>
<!-- 8.定义具名插槽 -->
<slot name="slot1"></slot>
<slot name="slot2"></slot>
</div>
</template>
<script>
// 2.创建vue实例
const vm = new Vue({
el:'.app',
// 8.给父组件添加数据
data:{
num:5,
},
// 4.创建局部组件
components:{
// 5.创建子组件
'myComponent':{
// 6.关联模板
template:'#my_Template'
}
}
})
</script>
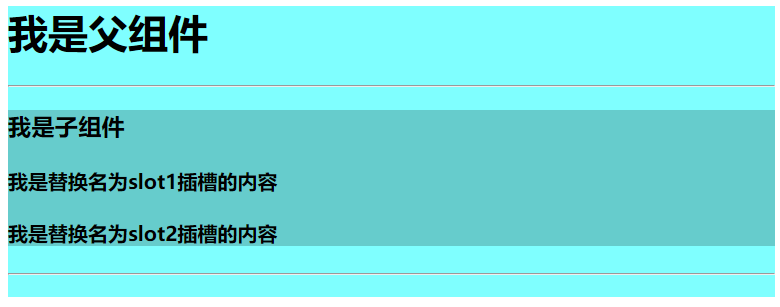