JAVA 函数
函数的定义
- 函数是什么?函数是一段具有独立功能的代码 可以减少程序冗余 并且提高效率与利用率。
- 如何定义函数?函数要有函数的名称 要有函数执行程序的空间 要有数据 要有结果等。。。。
函数的语法格式
以 public static void main(String[] args) {
。。。。。。。
return 。。
}为例子
- 其中 public为修饰符 指的是函数的访问权限。 private protected
- static 为函数类型 本地函数 native 静态函数 static 同步函数 synchronized
- void 为返回值类型 (这里表示无返回值)
- main 为函数名
- ()中的为参数列表 外界向函数传入的数据(实际参数),由这些参数变量进行接收(形式参
数)- {}中为函数体
- return 仅仅表示函数结束!如果函数有返回值,则return后跟返回值;如果没有返回值,则
- return可以不写,但是是存在的(隐藏的 在最后一行)
根据形参和返回值来看,函数有以下几种分类
- 有参数有返回值
计算一个数字a的b次幂
public class Sample
{
public static double pow (double a , int b)
{
if (a == 0)
{ return 0;
}
if (b == 0)
{ return 1;
}
double result = 1.0;
for (int i = 0; i < Math.abs(b) ; i++) {
result *= a;
}return b > 0 ? result : 1 / result;
}
public static void main(String[] args)
{ System.out.println(pow(2,2));
System.out.println(pow(2,-3));
}
}
- 有参数没返回值
将三个字符串的反转,并拼接打印
public class Sample {
public static void main(String[] args) {
print("123","456","789");
}
//123 456 789 =? 321654987
public static void print(String s1 , String s2 , String s3) { System.out.println(reverse(s1) + reverse(s2) + reverse(s3));
}public static String reverse(String s) {
String res = "";
for (int i = s.length() - 1; i >= 0; i--) { res += s.charAt(i);
}return res;
}
}
- 没有参数有返回值
获取当前时间的字符串
public class Sample {
public static void main(String[] args) { String currentTime = getTime(); System.out.println(currentTime);
}public static String getTime() {
//1.获取总毫秒数
long millis = System.currentTimeMillis();
//2.计算总秒数
long seconds = millis / 1000;
//3.计算总分钟数
long minutes = seconds / 60;
//4.计算总小时数
long hours = minutes / 60;
//5.计算当前小时数
long currentHours = hours % 24;
//6.计算当前分钟数
long currenrMinutes = minutes % 60;
//7.计算当前秒数
long currentSeconds = seconds % 60;
return currentHours + ":" + currenrMinutes + ":" + currentSeconds;
}
}
- 没参数没返回值
public class Sample {
public static void main(String[] args) { String currentTime = getTime(); System.out.println(currentTime);
}public static String getTime() {
//1.获取总毫秒数
long millis = System.currentTimeMillis();
//2.计算总秒数
long seconds = millis / 1000;
//3.计算总分钟数
long minutes = seconds / 60;
//4.计算总小时数
long hours = minutes / 60;
//5.计算当前小时数
long currentHours = hours % 24;
//6.计算当前分钟数
long currenrMinutes = minutes % 60;
//7.计算当前秒数
long currentSeconds = seconds % 60;
System.out.println(currentHours + ":" + currenrMinutes + ":" +
currentSeconds);
}
}
函数的运行原理
函数的运行是基于栈运行的栈:是一种先进后出的容器,我们这里面所说的栈是指JVM中的栈内存空间每一个函数,叫做栈帧,栈帧中所包含的内容有函数的定义,参数列表,函数的执行内容代码每一个函数要运行,就相当于这个栈帧进入到栈内存中-入栈如果一个函数即将结束,将这个栈帧从栈顶移出-出栈如果栈内存中有多个栈帧,运行的是最上面的栈帧,底下的栈帧暂停运行,直到该栈帧为栈顶元素比如:主函数先进栈,开始逐行运行,如果执行到第n行,调用另外一个函数A,则主函数在第n行暂停运行,将另一个函数A的栈帧入栈,再继续逐行运行,直到函数A的内容执行完毕,函数A出栈,主函数接着从第n行继续向下执行。以此类推
图片演示
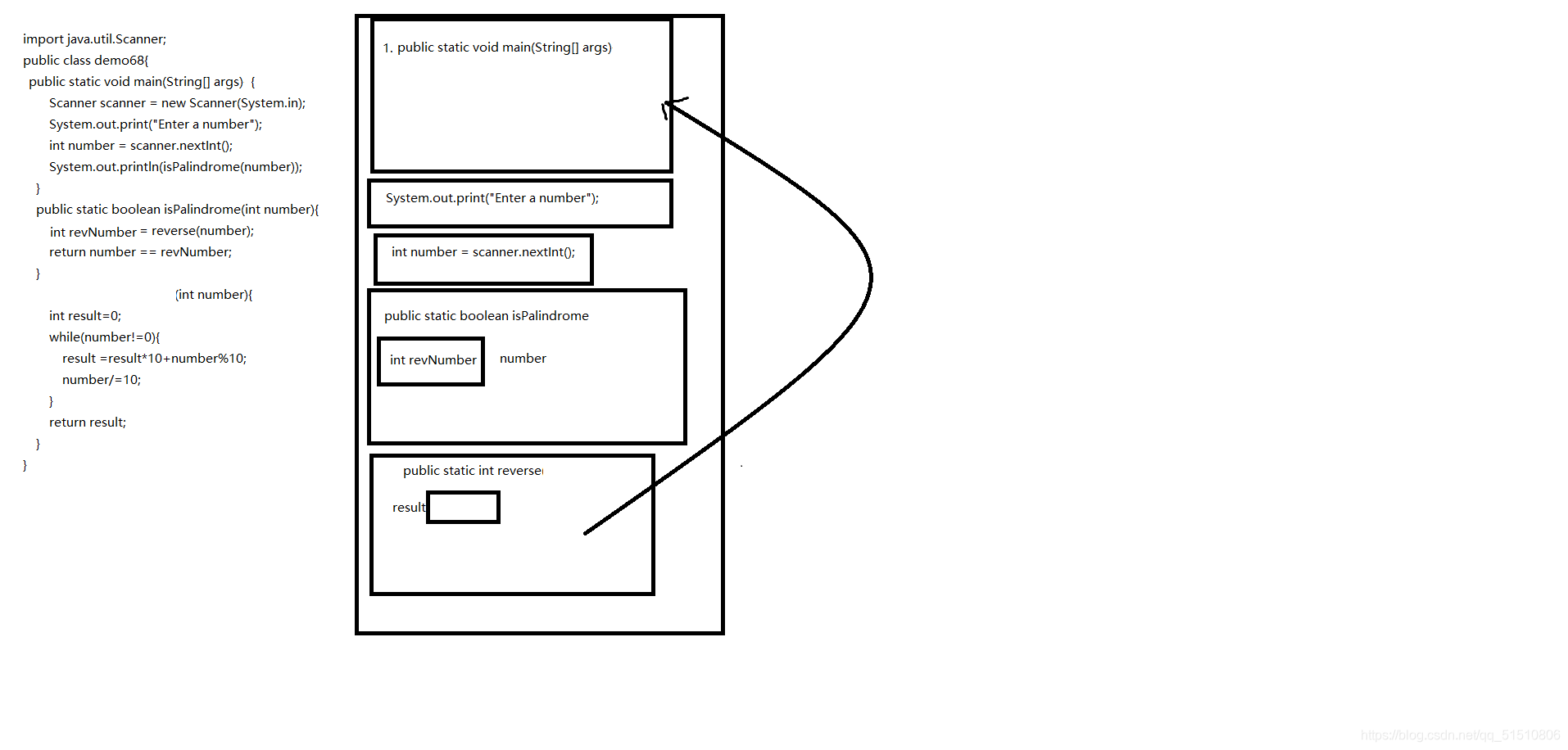
函数的重载
同一个类中可以出现多个同名函数,这个现象就叫做函数的重载( overload )如何来区分同名函数是否是重载关系呢?前提必须是同名,和返回值类型无关(返回值类型只和函数的计算功能相关),和权限也没有关系, 和形式参数的名称也无关!只和形式参数的数据类型有关(数量,排列组合)
public static void show ( int a , float b , char c ){}下列哪些是该函数的重载:
int show(int x, float y, char z) :不算重载 数据类型都是 int float charvoid show(float b,int a,char c) :算重载,顺序不一样 float int charvoid show(int a,int b,int c) :算重载,顺序不一样 int int intdouble show() :算重载,参数不一样
寻找重载函数的流程:
1. 看是否有确切的参数定义匹配,int int 找 int int
2. 看是否有可兼容的参数定义匹配,int int找double double或int double或double int
3. 如果可兼容的参数定义匹配较多,会报引用确定报错 引用不明确
函数的递归
两个递归案例
- 递归实现斐波那契数列
public class Sample {
public static void main(String[] args) {
System.out.println(f(35));
System.out.println(fibo_it(35));
}
public static int fibo_it(int x) {
if (x == 1 || x == 2) {
return 1;
}
int a = 1;
int b = 1;
int c = 0;
for (int i = 3; i <= x; i++)
{ c = a + b;
a = b;
b = c;
}
return c;
}
public static int f(int x)
{ if (x == 1 || x == 2) {
return 1; }
else {
return f(x-1) + f(x-2);
}
}
}
- 递归解决汉诺塔问题
public class Demo81 {
/*前4个 x->z
前3个 x->y
前2个 x->z
前1个 x->y
第2个 x->z
前1个 y->z
第3个 x->y
前2个 z->y
前1个 z->x
第2个 z->y
前1个 x->y
第4个 x->z
前3个 y->z
前2个 y->x
前1个 y->z
第2个 y->
前1个 z->x
第3个 y->z
前2个 x->z
前1个 x->y
第2个 x->z
前1个 y->z */
public static void main(String[] args) {
String x = "x";
String y = "y";
String z = "z";
hano(3,x,y,z);
//前3层从 x->z
//前2层从 x->y
}
public static void hano(int level,String begin,String mid,String end) {
if (level == 1) {
System.out.println(begin+"->"+end);
} else {
//前level-1层
hano(level - 1,begin,end,mid);
System.out.println(begin+"->"+end);
//前leve1-1层
hano(level - 1,mid,begin,end);
}
}
}