回溯算法基础
77. 组合
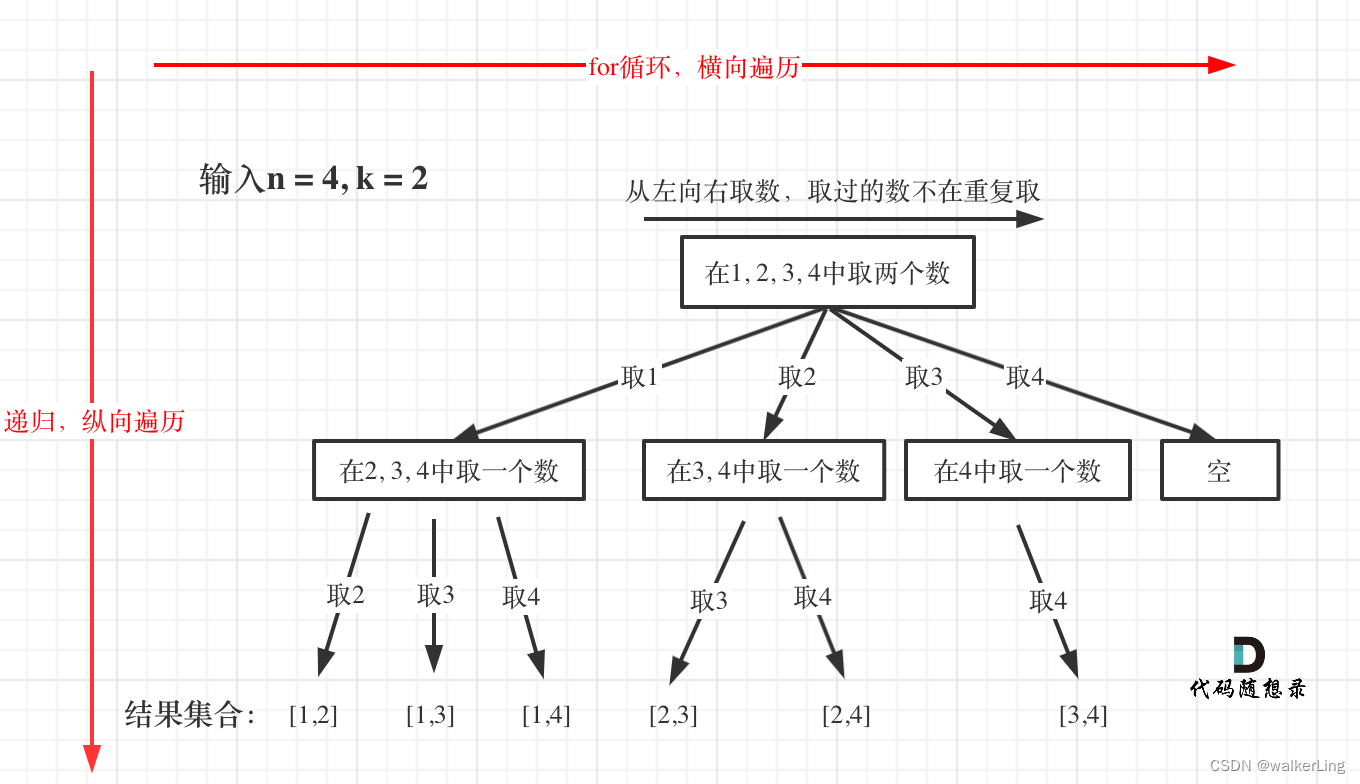
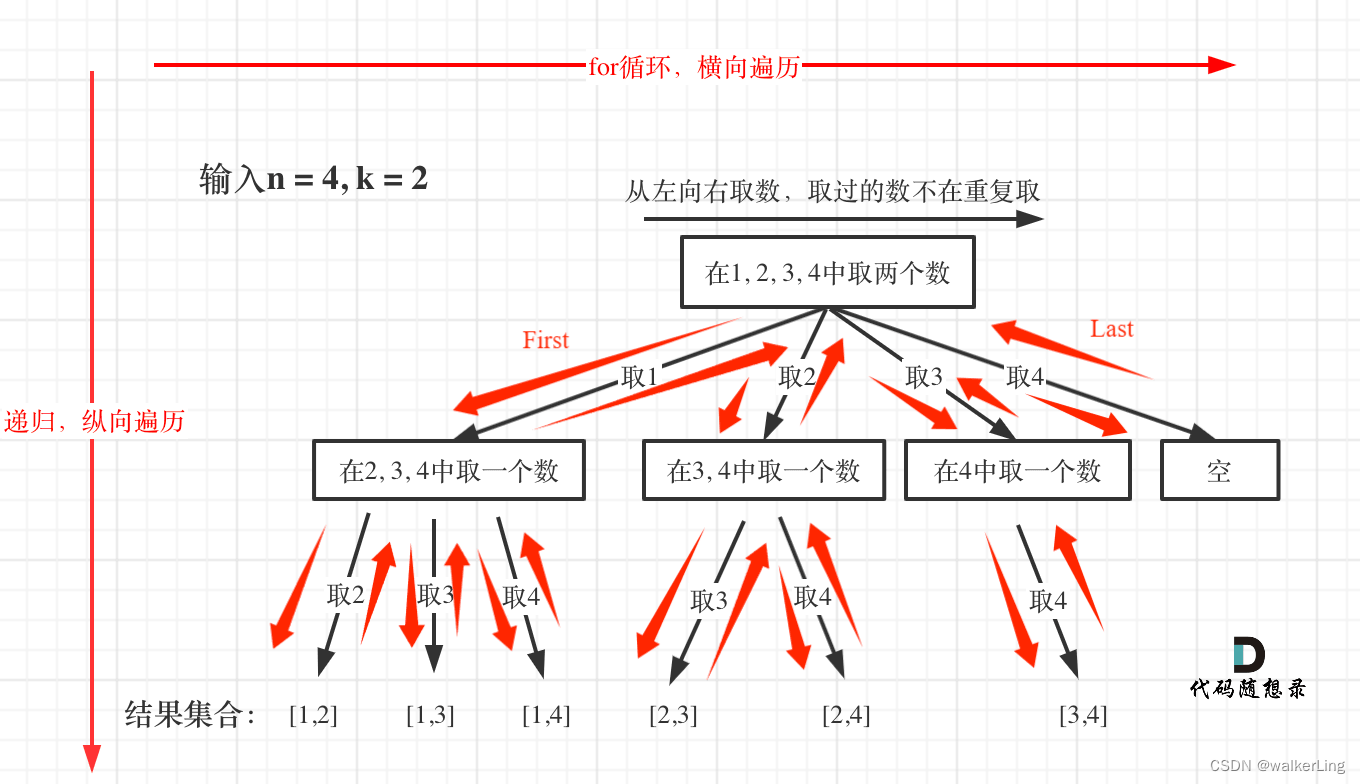
class Solution {
//回溯法
//定义二维数组存储合法组合结果
List<List<Integer>> result = new ArrayList<>();
//存储单次合法结果
LinkedList<Integer> path = new LinkedList<>();
public List<List<Integer>> combine(int n, int k) {
//n为树宽,k为树高,1为初始开始的位置
combine1(n, k, 1);
return result;
}
public void combine1(int n,int k, int startIndex){
//终止条件
if(path.size() == k){
//将当前path加入最终结果
result.add(new LinkedList<>(path));
}
//递归回溯部分
//for循环为横向遍历
for(int i = startIndex; i <= n; i++){
path.add(i);
//纵向递归
combine1(n, k, i + 1);
//回溯弹出
path.removeLast();
}
}
}
class Solution {
//回溯法
//定义二维数组存储合法组合结果
List<List<Integer>> result = new ArrayList<>();
//存储单次合法结果
LinkedList<Integer> path = new LinkedList<>();
public List<List<Integer>> combine(int n, int k) {
if(k <= 0 || n < k){
return result;
}
//n为树宽,k为树高,1为初始开始的位置
combine1(n, k, 1);
return result;
}
public void combine1(int n,int k, int startIndex){
//终止条件
if(path.size() == k){
//将当前path加入最终结果
result.add(new LinkedList<>(path));
//结束,返回
//debug点:记得写递归结束的return!
return;
}
//递归回溯部分
//for循环为横向遍历
for(int i = startIndex; i <= n - (k - path.size()) + 1; i++){
path.add(i);
//纵向递归
combine1(n, k, i + 1);
//回溯弹出
path.removeLast();
}
}
}