一. Map集合
- Interface Map< K , V> : key 代表键,V为键所映射的值,键不能重复而值可以重复(如学号和姓名)。
- 底层基于hash表,保证键的唯一性
- 如果把Javabean类对象作为值,需要在Javabean类中重写hashcode()和equals()方法,保证键的唯一性

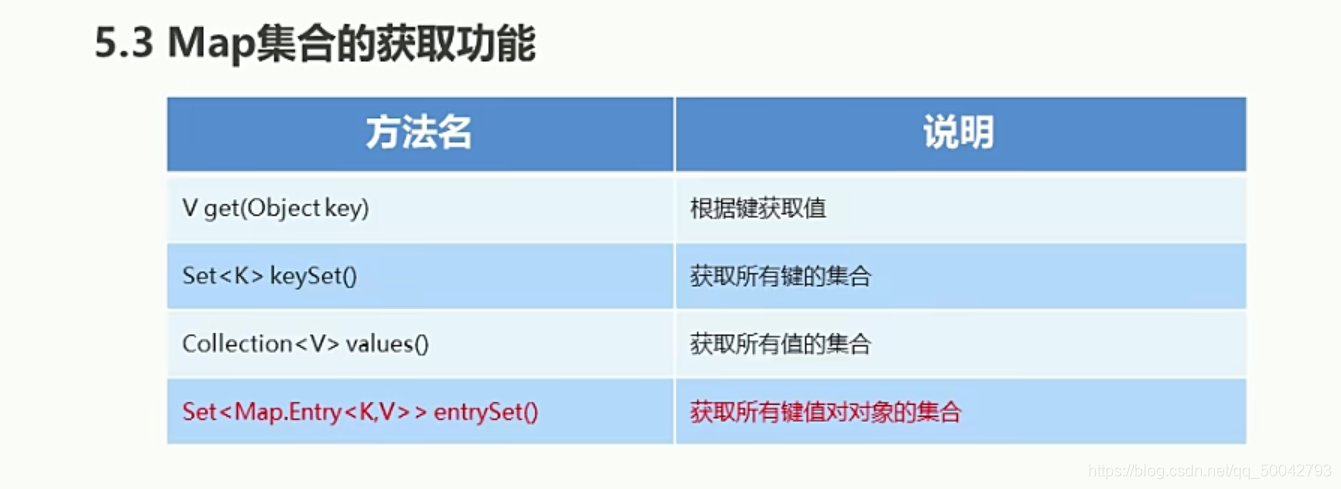
public class HashMap1 {
public static void main(String[] args) {
Map<String, String> map = new HashMap<>();
map.put("前前男友", "叶修");
map.put("前男友", "黄少天");
map.put("现男友", "周泽楷");
System.out.println(map);
String s = map.get("现男友");
System.out.println(s);
Set<String> set = map.keySet();
for (String key1 : set) {
String value1 = map.get(key1);
System.out.println(key1);
System.out.println(value1);
}
map.forEach((key, value) -> {
System.out.println(key);
System.out.println(value);
});
}
}
- ArrayList集合存储hashmap集合对象,并遍历的案例
public class HashMap2 {
public static void main(String[] args) {
ArrayList<HashMap<String, String>> list = new ArrayList();
HashMap<String, String> map1 = new HashMap<>();
map1.put("男神", "五条悟");
map1.put("同学", "伏黑");
map1.put("男朋友", "狗卷");
map1.put("总结", "人生巅峰");
HashMap<String, String> map2 = new HashMap<>();
map1.put("同桌", "越前");
map1.put("哥哥", "不二");
map1.put("竹马", "降谷晓");
map1.put("邻居", "柯南");
HashMap<String, String> map3 = new HashMap<>();
map1.put("叔叔", "杀生丸");
map1.put("朋友", "唐三");
map1.put("兄弟", "叶修");
map1.put("老婆", "淮竹");
Collections.addAll(list, map1,map2,map3);
for (HashMap<String, String> map : list) {
map.forEach((key,value)->{
System.out.println(key+"...."+value);
}) ;
}
}
}
- 键盘录入字符串,统计字符出现的次数
使用TreeMap集合对键进行自然排序
public class HashMap3 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.println("请输入:");
String line = sc.nextLine();
TreeMap<Character,Integer> map=new TreeMap<>();
for (int i = 0; i < line.length(); i++) {
char c = line.charAt(i);
Integer integer = map.get(c);
if (integer==null){
map.put(c,1);
}else{
integer++;
map.put(c, integer);
}
}
StringBuilder sb=new StringBuilder();
map.forEach((key,value)->{
sb.append(key).append("(").append(value).append(")");
});
System.out.println(sb.toString());
}
}
二. Collections的使用
- 概述:针对集合操作的工具类
- 常用方法
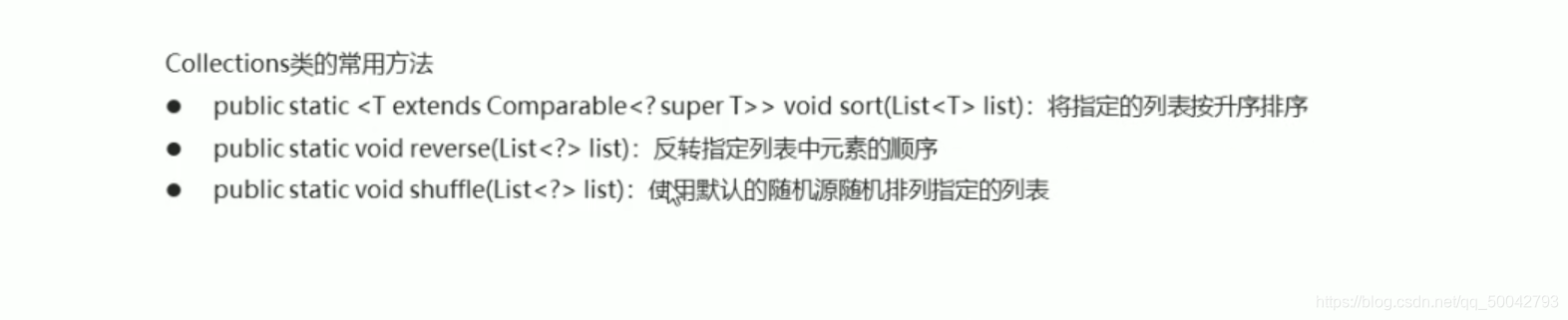
public class HashMap4 {
public static void main(String[] args) {
ArrayList<Integer> list=new ArrayList<>();
Collections.addAll(list, 30,13,32,532);
Collections.sort(list);
System.out.println(list);
Collections.shuffle(list);
System.out.println(list);
Collections.reverse(list);
System.out.println(list);
}
}
- 案例:ArrayList存储学生对象按年龄从小到大排序,年龄相同时,按照姓名的字母顺序排序
public class HashMap5 {
public static void main(String[] args) {
ArrayList<Student> list = new ArrayList<>();
Student s1 = new Student("ahkk", 25);
Student s2 = new Student("dfdsf", 25);
Student s3 = new Student("drv", 18);
Collections.addAll(list, s1, s2, s3);
Collections.sort(list, new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
int num = s1.getAge() - s2.getAge();
int num1 = num == 0 ? s1.getName().compareTo(s2.getName()) : num;
return num1;
}
});
System.out.println(list);
}
}