学习单向链表和^o^
List的接口
import java.util.Comparator;
public interface List<E> extends Iterable<E> {
public void add(E element);
public void add(int index, E element);
public void remove(E element);
public E remove(int index);
public E get(int index);
public E set(int index, E element);
public int size();
public int indexOf(E element);
public boolean isEmpty();
public boolean contains(E element);
public void clear();
public void sort(Comparator<E> c);
public List<E> subList(int fromIndex, int toIndex);
}
单向链表的实现
package 动态链表;
import 接口.List;
import java.util.Comparator;
import java.util.Iterator;
//单向链表
public class LinkedSinglyList<E> implements List<E> {
//定义一个节点,内部类
private class Node {
E data;//数据域 用来存储数据
Node next;//指针域 用来存储下一个节点对象的地址
public Node() {
this(null, null);
}
public Node(E data) {
this(data, null);
}
public Node(E data, Node next) {
this.data = data;
this.next = next;
}
@Override
public String toString() {
return data.toString();
}
}
private Node head;//是链表当中的头指针,指向第一个结点对象
private Node tail;//是链表当中的尾指针 指向最后一个结点对象
private int size;//是链表当中的有效元素个数
public LinkedSinglyList() {
head = null;
tail = null;
size = 0;
}
public LinkedSinglyList(E[] arr) {
for (E e : arr) {
add(e);
}
}
/**
* 默认在链表尾部添加元素
*
* @param element
*/
@Override
public void add(E element) {
add(size, element);
}
/**
* 在链表指定角标index添加一个元素
*
* @param index
* @param element
*/
@Override
public void add(int index, E element) {
if (index < 0 || index > size) {
throw new ArrayIndexOutOfBoundsException("add index out of bounds");
}
//创建一个新的结点对象
Node node = new Node(element);
if (isEmpty()) {
//链表为空
head = node;
tail = node;
//size++;
} else if (index == 0) {
//在表头添加
node.next = head;
head = node;
//size++;
} else if (index == size) {
//在表尾添加
tail.next = node;
tail = node;
//size++;
} else {
//在表中间添加
Node p = head;
//假如有3 个元素,小于(有效元素 - 1)
// 即小于2 在链表中间的位置进行添加
for (int i = 0; i < index - 1; i++) {
p = p.next;
}
node.next = p.next;//旧结点的下一个 给了 新结点的下一个
p.next = node;//p.next指向新的结点
// size++;
}
size++;
}
/**
* 删除链表中指定的元素element
*
* @param element
*/
@Override
public void remove(E element) {
int index = indexOf(element);
if (index != -1) {
remove(index);
}
}
/**
* 删除链表指定角标处的元素
*
* @param index
* @return
*/
@Override
public E remove(int index) {
if (index < 0 || index >= size) {
throw new IndexOutOfBoundsException("remove index out of bounds");
}
E ret = null;
if (size == 1) {
//链表只剩一个元素
ret = head.data;//或者ret = tail.data;
head = null;
tail = null;
} else if (index == 0) {
//删除表头
Node del = head;
ret = del.data; //head.data
head = del.next;
del.next = null;
} else if (index == size - 1) {
//删除表尾
Node p = head;
while (p.next != tail) {
p = p.next;
}
ret = tail.data;
p.next = null;
tail = p;
} else {
//删除中间某一个元素
Node p = head;
for (int i = 0; i < index - 1; i++) {
// index - 1 获取它的前驱
p = p.next;
}
Node del = p.next;
ret = del.data;
p.next = del.next;
del.next = null;
}
size--;
return ret;
}
@Override
public E get(int index) {
if (index < 0 || index >= size) {
throw new ArrayIndexOutOfBoundsException("get index out of bounds");
}
if (index == 0) {
//获取头
return head.data;
} else if (index == size - 1) {
//获取尾
return tail.data;
} else {
//获取中间
Node p = head;
for (int i = 0; i < index; i++) {
//获取它的本身
p = p.next;
}
return p.data;
}
}
/**
* 修改链表中角标处index元素尾element
*
* @param index
* @param element
* @return
*/
@Override
public E set(int index, E element) {
if (index < 0 || index >= size) {
throw new ArrayIndexOutOfBoundsException("set index out of bounds");
}
E ret = null;
if (index == 0) {
//修改头
ret = head.data;
head.data = element;
} else if (index == size - 1) {
//修改尾
ret = tail.data;
tail.data = element;
} else {
//修改中间某一个元素
Node p = head;
for (int i = 0; i < index; i++) {
p = p.next;
}
ret = p.data;
p.data = element;
}
return ret;
}
@Override
public int size() {
return size;
}
/**
* 查找元素在链表中第一次出现的角标
*
* @param element
* @return -1 返回没有找到指定元素
*/
@Override
public int indexOf(E element) {
Node p = head;
int index = 0;
while (!p.data.equals(element)) {
p = p.next;
index++;
if (p == null) {
return -1;
}
}
return index;
}
@Override
public boolean isEmpty() {
return size == 0 && head == null && tail == null;
}
/**
* 在链表中是否包含元素element
*
* @param element
* @return
*/
@Override
public boolean contains(E element) {
return indexOf(element) != -1;
}
@Override
public void clear() {
head = null;
tail = null;
size = 0;
}
@Override
public void sort(Comparator<E> c) {
if (c == null) {
throw new IllegalArgumentException("comparator can not be null");
}
int j = 0;
E e = null;
for (int i = 1; i < size; i++) {
e = get(i);
for (j = i; j > 0 && c.compare(get(j - 1), e) > 0; j--) {
set(j, get(j - 1));
}
set(j, e);
}
}
@Override
public List<E> subList(int fromIndex, int toIndex) {
if (fromIndex < 0) {
throw new IndexOutOfBoundsException("fromIndex must >= 0");
}
if (toIndex >= size) {
throw new IndexOutOfBoundsException("toIndex must < size");
}
if (fromIndex > toIndex) {
throw new IndexOutOfBoundsException("formIndex must <= toIndex");
}
LinkedSinglyList<E> subList = new LinkedSinglyList<>();
for (int i = fromIndex; i <= toIndex; i++) {
subList.add(get(i));//默认在表尾添加
}
return subList;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder(String.format("LinkedSinglyList:%d [", size));
if (isEmpty()) {
sb.append(']');
} else {
Node p = head;
while (true) {
sb.append(p.data);
if (p == tail) {
sb.append(']');
break;
}
sb.append(',');
p = p.next;
}
}
return sb.toString();
}
@Override
public Iterator<E> iterator() {
return new LinkedListIterator();
}
class LinkedListIterator implements Iterator<E> {
private Node cur = head;
@Override
public boolean hasNext() {
return cur != null;
}
@Override
public E next() {
E ret = cur.data;
cur = cur.next;
return ret;
}
}
}
进行测试
import 动态链表.LinkedSinglyList;
import java.util.Comparator;
import java.util.Iterator;
public class LinkedSinglyListTest {
public static void main(String[] args) {
LinkedSinglyList<Integer> list = new LinkedSinglyList<>();
System.out.println(list);
for (int i = 1; i <= 10; i++) {
list.add(i);
}
System.out.println(list);
//表头添加元素
list.add(0, 111);
list.add(0, 555);
list.add(0, 200);
System.out.println(list);
//在角标5处添加一个元素
list.add(5, 555);
System.out.println(list);
int len = list.size();
for (int i = 0; i < len; i++) {
list.remove(0);
}
System.out.println(list);
list.add(100);
list.add(90);
list.add(80);
list.add(70);
list.add(79);
System.out.println(list);
//排序
list.sort(new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o1.compareTo(o2);
}
});
System.out.println(list);
System.out.println(list.subList(1, 3));
//使用迭代器遍历
Iterator<Integer> it = list.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
}
}
结果截图:
Comparator() {
@Override
public int compare(Integer o1, Integer o2) {
return o1.compareTo(o2);
}
});
System.out.println(list);
System.out.println(list.subList(1, 3));
//使用迭代器遍历
Iterator it = list.iterator();
while (it.hasNext()) {
System.out.println(it.next());
}
}
}
## 结果
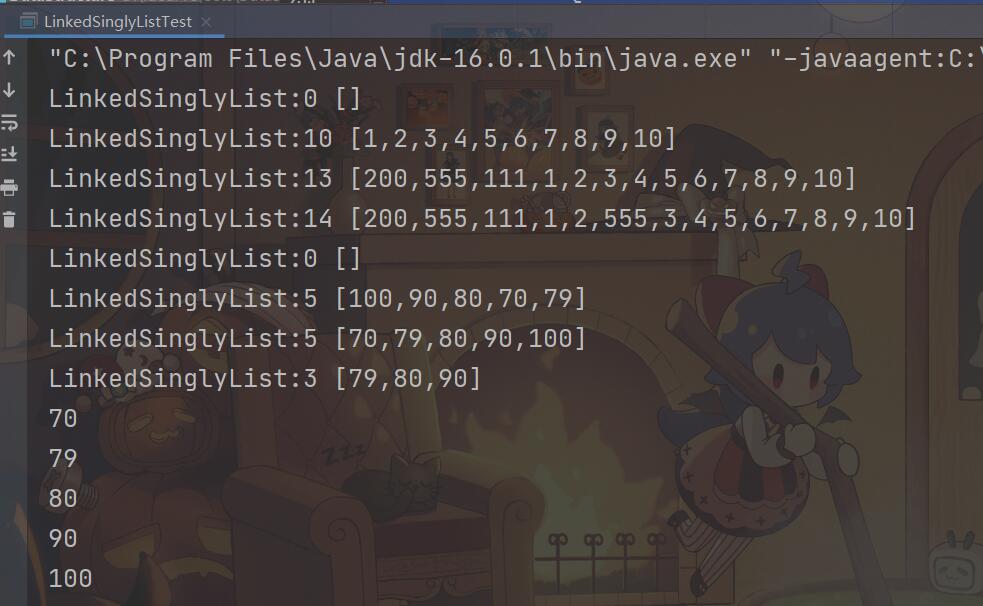