栈
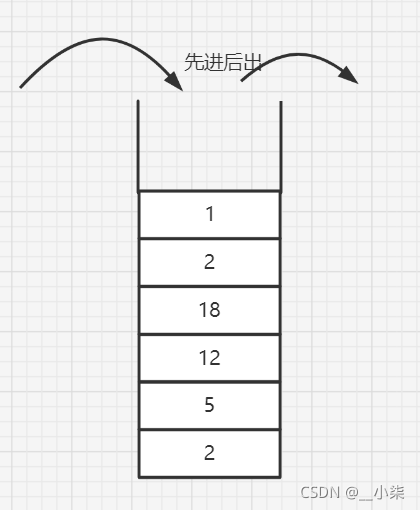
stack头文件
#pragma once
#define INIT_SIZE 10
typedef struct Stack
{
int* base;
int stackSize;
int top;
}Stack, * PStack;
void InitStack(PStack ps);
bool Push(PStack ps, int val);
bool GetTop(PStack ps, int* rtval);
bool Pop(PStack ps, int* rtval);
bool IsEmpty(PStack ps);
int GetLength(PStack ps);
void Clear(PStack ps);
void Destroy(PStack ps);
stack.cpp文件
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
#include "stack.h"
void InitStack(PStack ps)
{
assert(ps != NULL);
if (ps == NULL)
return;
ps->base = (int*)malloc(INIT_SIZE * sizeof(int));
assert(ps->base != NULL);
ps->top = 0;
ps->stackSize = INIT_SIZE;
}
bool IsFull(PStack ps)
{
return ps->top == ps->stackSize;
}
void Inc(PStack ps)
{
ps->stackSize *= 2;
ps->base = (int*)realloc(ps->base, ps->stackSize * sizeof(int));
assert(ps->base != NULL);
}
bool Push(PStack ps, int val)
{
if (IsFull(ps))
{
Inc(ps);
}
ps->base[ps->top++] = val;
return true;
}
bool GetTop(PStack ps, int* rtval)
{
if (IsEmpty(ps))
return false;
*rtval = ps->base[ps->top - 1];
return true;
}
bool Pop(PStack ps, int* rtval)
{
if (IsEmpty(ps))
return false;
*rtval = ps->base[--ps->top];
return true;
}
bool IsEmpty(PStack ps)
{
return ps->top == 0;
}
int GetLength(PStack ps)
{
return ps->top;
}
void Clear(PStack ps)
{
ps->top = 0;
}
void Destroy(PStack ps)
{
free(ps->base);
ps->base = NULL;
ps->top = 0;
ps->stackSize = 0;
}
链式栈
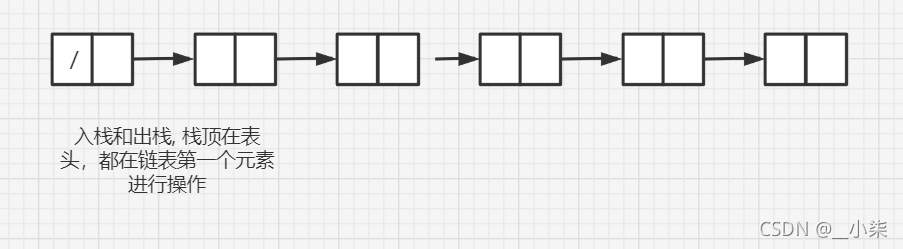
lstack头文件
#pragma once
typedef struct LSNode
{
int data;
struct LSNode* next;
}LSNode, * PLStack;
void InitStack(PLStack ps);
bool Push(PLStack ps, int val);
bool GetTop(PLStack ps, int* rtval);
bool Pop(PLStack ps, int* rtval);
bool IsEmpty(PLStack ps);
int GetLength(PLStack ps);
void Clear(PLStack ps);
void Destroy(PLStack ps);
lstack.cpp文件
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
#include "lstack.h"
void InitStack(PLStack ps)
{
assert(ps != NULL);
if (ps == NULL)
return;
ps->next = NULL;
}
bool Push(PLStack ps, int val)
{
LSNode* p = (LSNode*)malloc(sizeof(LSNode));
assert(p != NULL);
p->data = val;
p->next = ps->next;
ps->next = p;
return true;
}
bool GetTop(PLStack ps, int* rtval)
{
if (IsEmpty(ps))
return false;
*rtval = ps->next->data;
return true;
}
bool Pop(PLStack ps, int* rtval)
{
if (IsEmpty(ps))
return false;
GetTop(ps, rtval);
LSNode* p = ps->next;
ps->next = p->next;
free(p);
p = NULL;
return true;
}
bool IsEmpty(PLStack ps)
{
return ps->next == NULL;
}
int GetLength(PLStack ps)
{
int count = 0;
LSNode* p = ps->next;
for (; p != NULL; p = p->next)
{
count++;
}
return count;
}
void Clear(PLStack ps)
{
Destroy(ps);
}
void Destroy(PLStack ps)
{
LSNode* p;
while (ps->next !=NULL )
{
p = ps->next;
ps->next = p->next;
free(p);
}
}