一、图
- 图
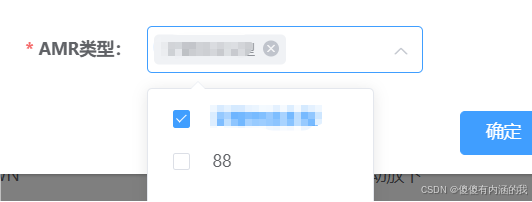
二、HTML
<div>
<el-form
:model="ruleForm"
:rules="rules"
ref="ruleForm"
label-width="100px"
>
<el-form-item
label="AMR类型:"
prop="amrTypeIds"
style="margin-bottom: 10px"
>
<el-cascader
v-model="ruleForm.amrTypeIds"
:options="amrTypeOptions"
:props="{ multiple: true }"
placeholder="请选择"
clearable
></el-cascader>
</el-form-item>
<div style="text-align: right;margin-bottom: 10px;">
<el-button
type="primary"
style="margin: 20px auto 0 auto"
@click="onSubmit()"
>
确定
</el-button>
<el-button style="margin: 10px auto 0 10px" @click="cancel()">
取消
</el-button>
</div>
</el-form>
</div>
下拉框内容
- :options=“amrTypeOptions” :下拉框数据
框内row
- v-model=“ruleForm.amrTypeIds”:框内的内容显示
ids参数
- prop=“amrTypeIds”:有两个参数,一个id一个name,看保存的时候后端要哪个,一般都是id,写id
- :props=“{ multiple: true }”:可多选
三、rules
data() {
var checkAmrType = (rule, value, callback) => {
if (!value || value.length === 0) {
callback(new Error("请选择至少一个AMR类型"));
} else {
callback();
}
};
}
return{
rules: {
amrTypeIds: [
{
required: true,
validator: checkAmrType,
trigger: "blur",
},
],
},
amrTypeOptions: [],
ruleForm: {
amrTypeIds: [],
},
};
}
四、下拉列表数据获取
created() {
this.fetchSelectOptions();
},
methods: {
async fetchSelectOptions() {
try {
const amrTypeResponse = await amrTypeList({});
this.amrTypeOptions = amrTypeResponse.data;
console.log("下拉框AMR:", this.amrTypeOptions);
} catch (error) {}
},
}
五、onload
onLoad(page, params = {}) {
this.loading = true;
getTest(
page.currentPage,
page.pageSize,
Object.assign(params, this.query)
).then((res) => {
this.page.total = res.data.total;
this.tableData = res.data.records.map((record) => {
if (Array.isArray(record.amrTypeNames)) {
record.amrTypeNames = record.amrTypeNames.join(", ");
}
return record;
});
this.loading = false;
});
},
数组变字符串
- [“叉臂伸出车型”, “88”]变成 叉臂伸出车型, 88 显示在列表:意思是=>amrTypeNames 数组,转成字符串
- map:遍历
- Array.isArray:判断是否是数组
- record.amrTypeNames.join(", ");:转换成字符串
六、rowEdit
rowEdit(row) {
this.editRowData = row;
console.log(111, row);
this.ruleForm = { ...this.editRowData };
this.$nextTick(() => {
this.$refs.ruleForm.clearValidate();
});
this.editDrawer = true;
},
清除校验提示
- this.$refs.ruleForm.clearValidate() :清除所有校验提示
- nextTick :nextTick 可以确保在修改数据后,执行一些需要在 DOM 更新完成后的逻辑。
- this.ruleForm = { …this.editRowData }; :避免选了 没保存,打开还显示选了的
七、onsubmit 等
onSubmit() {
const flatSubtaskIds = this.ruleForm.amrTypeIds.flat();
this.$refs.ruleForm.validate((valid) => {
if (valid) {
updateAmrType({
subtaskId: this.ruleForm.id,
amrTypeIds: flatSubtaskIds,
}).then((res) => {
if (res.code !== 1) {
this.$message({
type: "error",
message: res.msg,
});
} else {
this.$message({
type: "success",
message: res.msg,
});
this.onLoad(this.page, this.query);
this.editClose();
}
});
} else {
console.log("校验未通过");
this.$message.error("请填写所有必填项");
return false;
}
});
},
cancel() {
this.dialogFullScreen = false;
this.editDrawer = false;
},
editClose() {
this.dialogFullScreen = false;
this.editDrawer = false;
this.$refs.ruleForm.resetFields();
},
扁平化
- amrTypeIds: flatSubtaskIds,:amrTypeIds传递的值是[[“1810523824710606849”], [“1828708082608340993”]],改成[“1810523824710606849”, “1828708082608340993”]
- 扁平化处理:flat() 方法,这个方法会将嵌套的数组打平,默认只会扁平化一层。
重置表单字段(解决:打开弹框时仍显示之前未保存的修改)
- this.$refs.ruleForm.resetFields(); : 重置表单字段
在关闭弹框后如果没有被重置,会导致再次打开弹框时仍显示之前未保存的修改