vector模板类代码
#include <iostream>
using namespace std;
template <typename T>
class vector
{
T* start;
T* last;
T* end;
public:
vector():start(NULL),last(NULL),end(NULL) {}
vector(const vector& other)
{
int f = other.last-other.start;
int h = other.end-other.start;
start = new T[h];
last = start+f;
end = start+h;
for(int i=0; i<f;i++)
*(start+i) = *(other.start+i);
}
vector& operator=( const vector& other )
{
if(this != &other)
{
int f = other.last-other.start;
int h = other.end-other.start;
start = new T[h];
last = start+f;
end = start+h;
for(int i=0; i<f;i++)
*(start+i) = *(other.start+i);
}
return *this;
}
T& operator[]( int loc )
{
return start[loc];
}
~vector(){cout<<"析构函数"<<endl;}
vector(int num, const T &val )
{
start = new T[num];
last = start+num;
end = start+num;
for(int i=0;i<num;i++)
*(start+i) = val;
}
vector( T* start, T* end )
{
int len = end-start;
this->start = new T[len];
this->last = this->start+len;
this->end = this->start+len;
for(int i=0;i<len;i++)
this->start[i] = start[i];
}
int size()
{
return last-start;
}
int capacity()
{
return end-start;
}
bool empty()
{
return start==last ?1:0;
}
bool full()
{
return last==end ?1:0;
}
void pop_back()
{
if(!empty())
{
*(--last) = (T)NULL;
}
else
cout<<"vector容量为空"<<endl;
}
void push_back( const T &val )
{
if(!full())
{
*last++=val;
}
else
{
int len = end-start;
if(len == 0)
{
start = new T (val);
last = start+1;
end = start+1;
}
else{
T *temp = new T[len*2];
for(int i=0;i<len;i++)
temp[i] = start[i];
temp[len] = val;
delete []start;
start = temp;
last = start+len+1;
end = start+len*2;
}
}
}
T &at( int loc )
{
if(loc >=last-start)
throw string("数组下标越界");
else
return start[loc];
}
T &back()
{
return *(last-1);
}
T &front()
{
return *start;
}
T* begin()
{
return start;
}
T* _end()
{
return last;
}
void clear()
{
last = start;
}
};
int main()
{
vector<int> v1;
cout<<"v1.size = "<<v1.size()<<endl;
cout<<"v1.capacity = "<<v1.capacity()<<endl;
cout<<"************************************************************\n";
for(int i=1; i<=20; i++)
{
v1.push_back(i);
cout<<"v1.size = "<<v1.size()<<" v1.capacity = "<<v1.capacity()<<endl;
}
for(auto i=0; i<v1.size(); i++)
{
cout<<v1.at(i)<<" ";
}
cout<<endl;
v1.pop_back();
for(int i=0; i<v1.size(); i++)
{
cout<<v1.at(i)<<" ";
}
cout<<endl;
v1.front() = 100;
v1.back() = 200;
for(int i=0; i<v1.size(); i++)
{
cout<<v1.at(i)<<" ";
}
cout<<endl;
for(auto p=v1.begin(); p!=v1._end(); p++)
{
cout<<*p<<" ";
}
cout<<endl;
v1.clear();
cout<<"v1.size = "<<v1.size()<<endl;
cout<<"v1.capacity = "<<v1.capacity()<<endl;
for(int i=0; i<v1.capacity(); i++)
{
cout<<v1[i]<<" ";
try {
cout<<v1.at(i)<<" ";
} catch (string e) {
cout<<e;
}
cout<<" ";
}
cout<<endl;
vector<char> v2(5, 'A');
for(int i=0; i<v2.size(); i++)
{
cout<<v2[i]<<" ";
}
cout<<endl;
cout<<"v2.size = "<<v2.size()<<endl;
cout<<"v2.capacity = "<<v2.capacity()<<endl;
v2.push_back('G');
cout<<"v2.capacity = "<<v2.capacity()<<endl;
int arr[] = {3,8,4,6,2,9,1,0,7};
vector<int> v3(arr, arr+5);
for(int i=0; i<v3.size(); i++)
{
cout<<v3[i]<<" ";
}
cout<<endl;
return 0;
}
测试结果
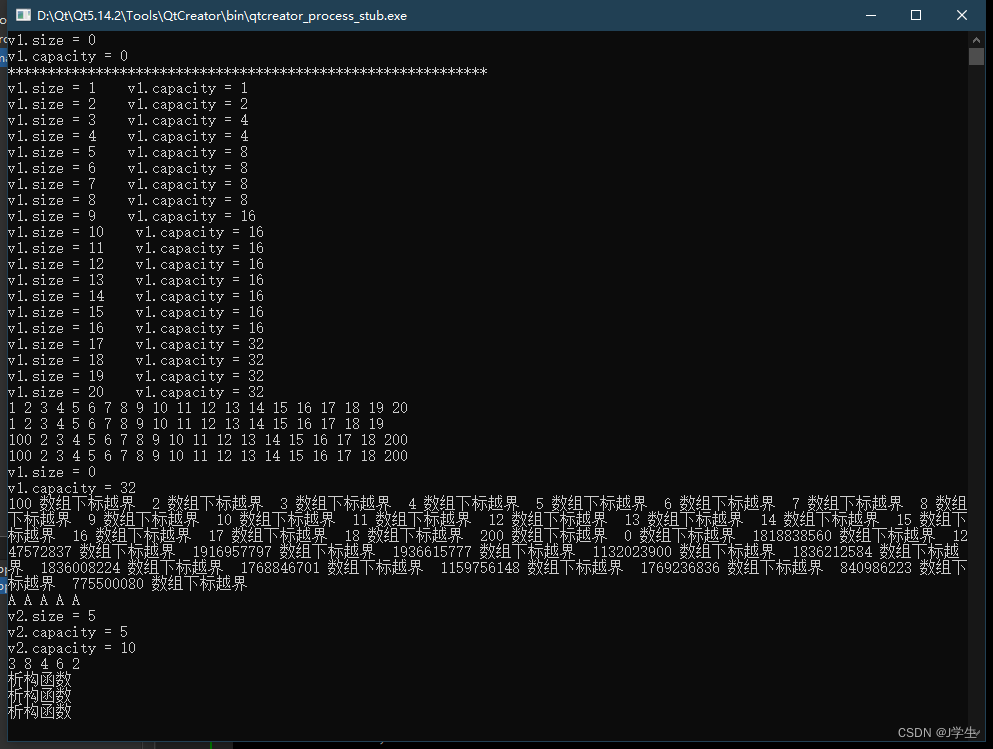