1.判断当前主机的CPU生产商,其信息在/proc/cpuinfo文件中vendor_id一行中
2.根据用户输入成绩,判断优良中差(A,B,C,D, 注意边界问题)
3.判断 sshd 进程是否运行,如果服务启动打印启动,未启动则打印未启动(使用查看进程和端口两种方式)
4.检查主机是否存活,并输出结果(使用for循环实现:主机数>3)
5.编写脚本,判断当前系统剩余内存大小,如果低于100M,邮件报警管理员,使用计划任务,每
10分钟检查一次。
1.判断当前主机的CPU生产商,其信息在/proc/cpuinfo文件中vendor_id一行中
整体操作图:
1.首先通过命令获得CPU厂商
cat /proc/cpuinfo |grep vendor_id|cut -d":" -f2
2.再将获得的结果通过正则表达式进行匹配
#!/bin/bash
vendor=`cat /proc/cpuinfo |grep vendor_id|cut -d":" -f2`
if [[ $vendor =~ [[:space:]]*GenuineIntel$ ]]
then echo "intel"
elif [[ $vendor =~ [[:space:]]*Authentic$ ]]
then echo 'AMD'
else
echo 'other'
fi
3.脚本运行结果
[root@localhost day_3]# bash output_cpu_vendor.sh
intel
2.根据用户输入成绩,判断优良中差(A,B,C,D, 注意边界问题)
ps:会使用以下表达式!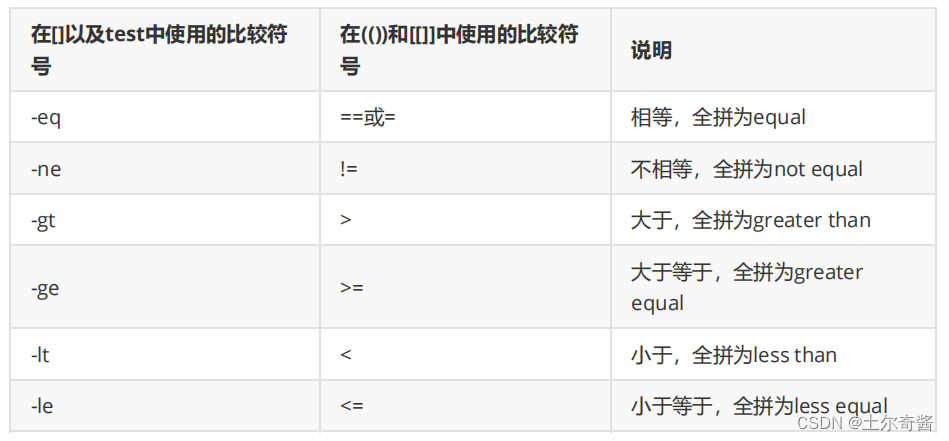
脚本文件:
#!/bin/bash
#对输入成绩进行等级判定
read -p "请输入你正确的分数:" score
if [[ -z "$score" ]]
then
echo "不能为空!"
elif echo "$score"|grep "[a-zA-Z]" >/dev/null
then
echo "要求输入的为数字"
elif [[ $score -gt 100 || $score -lt 0 ]]
then
echo "请输入0~100的数字!"
elif [[ $score -ge 90 ]]
then
echo "成绩为A"
elif test $score -ge 80
then
echo "成绩为B"
elif [ $score -ge 60 ]
then
echo "成绩为C"
else
echo "成绩为D"
fi
运行结果:
[root@localhost day_3]# bash score_definition.sh
请输入你正确的分数:100
成绩为A
[root@localhost day_3]# bash score_definition.sh
请输入你正确的分数:88
成绩为B
[root@localhost day_3]# bash score_definition.sh
请输入你正确的分数:65
成绩为C
[root@localhost day_3]# bash score_definition.sh
请输入你正确的分数:59
成绩为D
[root@localhost day_3]# bash score_definition.sh
请输入你正确的分数:
不能为空!
[root@localhost day_3]# bash score_definition.sh
请输入你正确的分数:alibaba
要求输入的为数字
3.判断 sshd 进程是否运行,如果服务启动打印启动,未启动则打印未启动(使用查看进程和端口两种方式)
编写脚本:
#!/bin/bash
sshd_pro=`ps -ef|grep sshd|grep -v grep|wc -l` #查看进程
sshd_port=`netstat -lntup | grep -w 22 | wc -l` #查看端口
if [[ $sshd_pro > 0 || $sshd_port > 0 ]] #若>0表示sshd正在运行中
then echo "sshd is running"
else
echo 'sshd not runnung'
fi
运行脚本:
[root@localhost day_3]# bash sshd_running.sh
sshd is running
[root@localhost day_3]# bash sshd_running.sh lntup
sshd is running
4.检查主机是否存活,并输出结果(使用for循环实现:主机数>3)
编写脚本
#!/bin/bash
for i in {128..132}
do
ping -i 0.5 -c 2 -w 1 192.168.248.$i >/dev/null
if [ $? -eq 0 ]
then
echo "192.168.248.$1 is online"
else
echo "192.168.248.$I is off"
fi
done
运行脚本
[root@localhost day_3]# bash compter_live.sh
192.168.248. is off
192.168.248. is off
192.168.248. is off
192.168.248. is off
192.168.248. is online
5.编写脚本,判断当前系统剩余内存大小,如果低于100M,邮件报警管理员,使用计划任务,每10分钟检查一次。
[root@localhost test]# vim free_mem.sh
#!/bin/bash
free_mem=$(free -m | grep "Mem:" | tr -s " "| cut -d " " -f4)
if [ "$free_mem" -le 100 ];then
echo "剩余内存:${free_mem},低于100M" | mail -s "内存报警" root@localhost
fi
~
[root@localhost test]# chmod a+rx free_mem.sh
[root@localhost test]# crontab -e */10 * * * * /test/free_mem.sh &>/dev/null