easyChart报表导出
效果图:
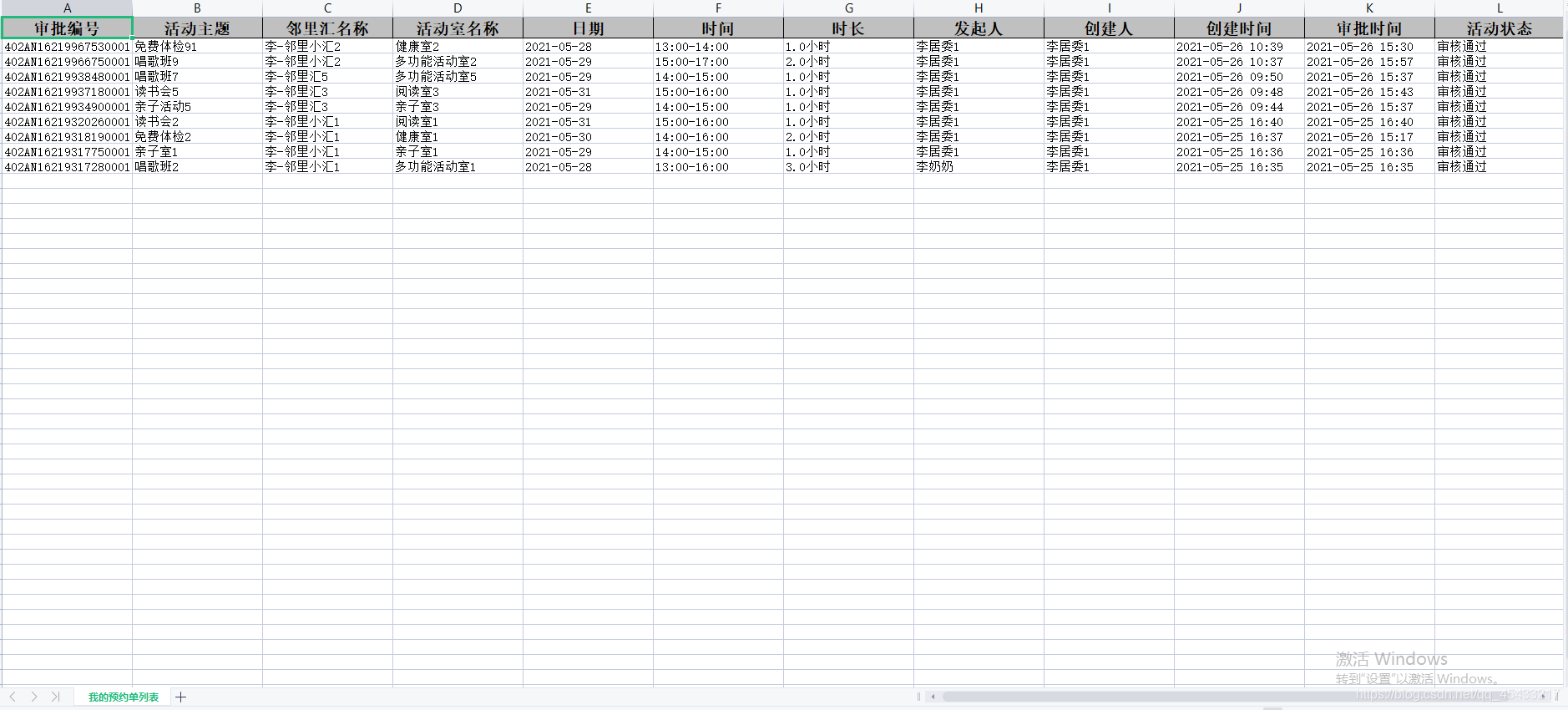
一、后台
1. 依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>2.1.6</version>
</dependency>
2. excel对象
import com.alibaba.excel.annotation.ExcelProperty;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class ActivityExcelBO {
@ExcelProperty(value = "审批编号",index = 0)
private String approveNo;
@ExcelProperty(value = "活动主题",index = 1)
private String topic;
@ExcelProperty(value = "邻里汇名称",index = 2)
private String remitName;
@ExcelProperty(value = "活动室名称",index = 3)
private String playRoomName;
@ExcelProperty(value = "日期",index = 4)
private String holdDateStr;
@ExcelProperty(value = "时间",index = 5)
private String holdTimeStr;
@ExcelProperty(value = "时长",index = 6)
private String timeLong;
@ExcelProperty(value = "发起人",index = 7)
private String initiatorMenName;
@ExcelProperty(value = "创建人",index = 8)
private String addMenName;
@ExcelProperty(value = "创建时间",index = 9)
private String addTimeStr;
@ExcelProperty(value = "审批时间",index = 10)
private String approveTimeStr;
@ExcelProperty(value = "活动状态",index = 11)
private String statusName;
}
3. 自动设置列宽的类
import com.alibaba.excel.metadata.CellData;
import com.alibaba.excel.metadata.Head;
import com.alibaba.excel.write.metadata.holder.WriteSheetHolder;
import com.alibaba.excel.write.style.column.AbstractColumnWidthStyleStrategy;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Sheet;
import java.util.List;
/**
* 自动设置列宽
*/
public class ExcelWidthStyleStrategy extends AbstractColumnWidthStyleStrategy {
// 统计setColumnWidth被调用多少次
private static int count = 0;
@Override
protected void setColumnWidth(WriteSheetHolder writeSheetHolder, List<CellData> cellDataList, Cell cell, Head head,
Integer relativeRowIndex, Boolean isHead) {
// 简单设置
Sheet sheet = writeSheetHolder.getSheet();
sheet.setColumnWidth(cell.getColumnIndex(), 5000);
System.out.println(count++);
}
}
4. controller
/**
* 预约单报表导出
*/
@PostMapping(value = "GetActivityListExcel")
@ApiOperation(value = "预约单报表导出", notes = "预约单报表导出")
public void GetApproveListExcel(HttpServletResponse response, @RequestBody ActivityPageQuery pageQuery) {
// 验证用户信息
var tempVal = TokenUse.verificationTokenInfo(request);
assert tempVal != null;
BoardMessage message;
try {
List<ActivityExcelBO> activityExcelBOList = iActivityService.selectActivityList(pageQuery);
String title = "我的预约单列表";
// 这里注意 用swagger 会导致各种问题,请直接用浏览器或者用postman
response.setContentType("application/vnd.ms-excel");
response.setCharacterEncoding("utf-8");
// 这里URLEncoder.encode可以防止中文乱码 当然和easyexcel没有关系
String fileName = URLEncoder.encode(title, StandardCharsets.UTF_8);
response.setHeader("Content-disposition", "attachment;filename=" + fileName + ".xlsx");
EasyExcel.write(response.getOutputStream(), ActivityExcelBO.class)
.sheet(title)
.registerWriteHandler(new ExcelWidthStyleStrategy()) // 设置宽度
.doWrite(activityExcelBOList);
} catch (Exception e) {
e.printStackTrace();
}
}
二、前台
1. 按钮
<el-button size="mini" icon="el-icon-download" type="info" @click="exportExcel()">下载本报表</el-button>
2. js代码
<script>
import axios from 'axios'
const vueConfig = require('../../../../config/config.js')
import { getToken } from '@/utils/auth'
export default {
name: 'myAllAudit',
data() {
return {
searchform: {
createTime: '',
auditTime: '',
approveEndTime: '',
approveStartTime: '',
createEndTime: '',
createStartTime: '',
keyWords: '',
neighboursRemitId: '',
playRoomId: '',
initiatorMenName: '',
addMenId: '',
status: 1,
},
},
}
methods: {
exportExcel() {
axios({
// 用axios发送post请求
method: 'post',
url:
'http://' +
vueConfig.webIp +
':' +
vueConfig.webPort +
'/approve/GetApproveListExcel', // 请求地址
data: this.searchform, // 参数
responseType: 'blob', // 表明返回服务器返回的数据类型
headers: {
'Content-Type': 'application/json',
tokenid: getToken(),
},
})
.then((res) => {
var nameStr = this.createFileName()
// 处理返回的文件流
const blob = new Blob([res.data]) //new Blob([res])中不加data就会返回下图中[objece objece]内容(少取一层)
const fileName = '预约单列表'+nameStr+'.xlsx' //下载文件名称
const elink = document.createElement('a')
elink.download = fileName
elink.style.display = 'none'
elink.href = URL.createObjectURL(blob)
document.body.appendChild(elink)
elink.click()
URL.revokeObjectURL(elink.href) // 释放URL 对象
document.body.removeChild(elink)
})
.catch(function (error) {
console.log(error)
this.$message({
type: 'error',
message: '导出报表失败!',
})
})
},
createFileName() {
var now = new Date()
var year = now.getFullYear() //得到年份
var month = now.getMonth() //得到月份
var date = now.getDate() //得到日期
var hour = now.getHours() //得到小时
var minu = now.getMinutes() //得到分钟
month = month + 1
if (month < 10) month = '0' + month
if (date < 10) date = '0' + date
var number = now.getSeconds() % 43 //这将产生一个基于目前时间的0到42的整数。
var time = year + month + date + hour + minu
return time + '_' + number
},
}
</script>