<template>
<div class="edit-table-container">
<el-row>
<el-row type="flex" justify="space-between">
<el-row type="flex" align="bottom">
<slot />
</el-row>
<el-row
v-if="type=='update'"
type="flex"
justify="end"
>
<el-button type="primary" size="mini" icon="el-icon-circle-plus-outline" @click="addRow">添加行</el-button>
</el-row>
</el-row>
<el-table :data="tableData" border highlight-current-row>
<el-table-column
v-for="(item, $i) in columnName"
:key="$i"
:label="item.name"
:min-width="item.minWidth?item.minWidth: '100px'"
header-align="center"
:fixed="item.fixed"
>
<template slot-scope="scope">
<div>
<!-- 编辑表单 -->
<template v-if="scope.row.isEdit">
<el-form
:ref="'form' + [scope.row.id]"
:model="scope.row"
:rules="item.rules"
:show-message="false"
>
<!-- input类型 -->
<template v-if="item.type == 'input'">
<el-form-item :prop="item.code">
<el-input
v-model="scope.row[item.code]"
size="mini"
clearable
>
<template v-if="item.unit" slot="append">{{ item.unit }}</template>
</el-input>
</el-form-item>
</template>
<!-- select类型 -->
<template v-if="item.type == 'select'">
<el-form-item :prop="item.code">
<el-select
v-model="scope.row[item.code]"
size="mini"
clearable
placeholder="请选择"
>
<el-option v-for="(v,$index) in item.option" :key="$index" :label="v.label" :value="v.label" />
</el-select>
</el-form-item>
</template>
</el-form>
</template>
<!-- 文本类型 -->
<template v-else>
<!-- 链接 -->
<template v-if="item.type =='link'">
<el-link type="primary" :underline="false">{{ scope.row[item.code] }}</el-link>
</template>
<!-- 打印 -->
<template v-if="item.type =='print'">
<el-button type="warning" plain size="mini">{{ scope.row[item.code] }}</el-button>
</template>
<!-- 文本 -->
<template v-if="!item.type">
<el-col>{{ scope.row[item.code] }}</el-col>
</template>
</template>
</div>
</template>
</el-table-column>
<el-table-column
v-if="type=='update'"
label="操作"
header-align="center"
width="200px"
fixed="right"
>
<template slot-scope="scope">
<el-button
v-if="scope.row.isEdit"
type="primary"
size="mini"
icon="el-icon-success"
plain
@click="confirm(scope.row)"
>确认</el-button>
<el-button
v-if="scope.row.isEdit"
size="mini"
plain
@click="cancel(scope.row, scope.$index)"
>取消</el-button>
<el-button
v-else
type="primary"
size="mini"
icon="el-icon-edit"
plain
@click="edit(scope.row)"
>编辑</el-button>
<el-button
v-if="!scope.row.isEdit"
type="danger"
size="mini"
icon="el-icon-delete"
plain
@click="deleteRow(scope.row)"
>删除</el-button>
</template>
</el-table-column>
</el-table>
</el-row>
</div>
</template>
<script>
import { deepClone } from '@/utils'
export default {
name: 'EditTable',
props: {
columnName: {
type: Array,
required: true,
default: () => []
},
tableDataInit: {
type: Array,
default: () => []
},
type: {
type: String,
default: 'view',
required: true
}
},
data() {
return {
tableDataCopy: deepClone(this.tableDataInit),
defaultAddRow: { isAdd: true, isEdit: true, id: 0 }
}
},
computed: {
tableData() {
if (!this.tableDataInit || this.tableDataInit.length < 0) return []
else {
this.tableDataInit.map(item => {
if (item.isEdit) return
else this.$set(item, 'isEdit', false)
})
return this.tableDataInit
}
}
},
watch: {
tableData(current) {
console.log(current, 'current')
}
},
methods: {
addRow() {
const isAdd = this.tableData.some(v => v.isEdit === true)
if (!isAdd) {
const emptyRow = {}
this.columnName.map(v => {
emptyRow[v.code] = ''
})
this.tableData.push(Object.assign(emptyRow, this.defaultAddRow))
} else this.$message.warning('请先保存正在编辑的行')
},
confirm(row) {
const refName = 'form' + row.id
const flag = this.$refs[refName].every(v => {
const paramsObj = {
flag: false
}
v.validate(valid => {
paramsObj.flag = valid
})
return paramsObj.flag
})
if (flag) {
row.isEdit = !row.isEdit
row.isAdd = false
this.tableDataCopy = deepClone(this.tableData)
}
},
cancel(row, index) {
if (row.isAdd) {
this.tableData.splice(this.tableData.length - 1, 1)
} else {
Object.keys(row).forEach(keys => {
if (keys !== 'isEdit') {
row[keys] = this.tableDataCopy[index][keys]
}
})
row.isEdit = !row.isEdit
}
},
edit(row) {
const isEdit = this.tableData.some(v => {
return v.isEdit === true
})
if (!isEdit) {
row.isEdit = !row.isEdit
} else this.$message.warning('请先保存正在编辑的行')
},
deleteRow(row) {
if (row.isAdd) {
this.cancel(row)
} else {
this.$confirm('确定要删除该行吗?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
this.$message({
type: 'success',
message: '删除成功!'
})
}).catch(() => {
this.$message({
type: 'info',
message: '已取消'
})
})
}
}
}
}
</script>
<style lang="scss" scoped>
.el-form-item {
margin-bottom: 5px;
}
.edit-table-container{
margin-bottom: 20px;
}
</style>
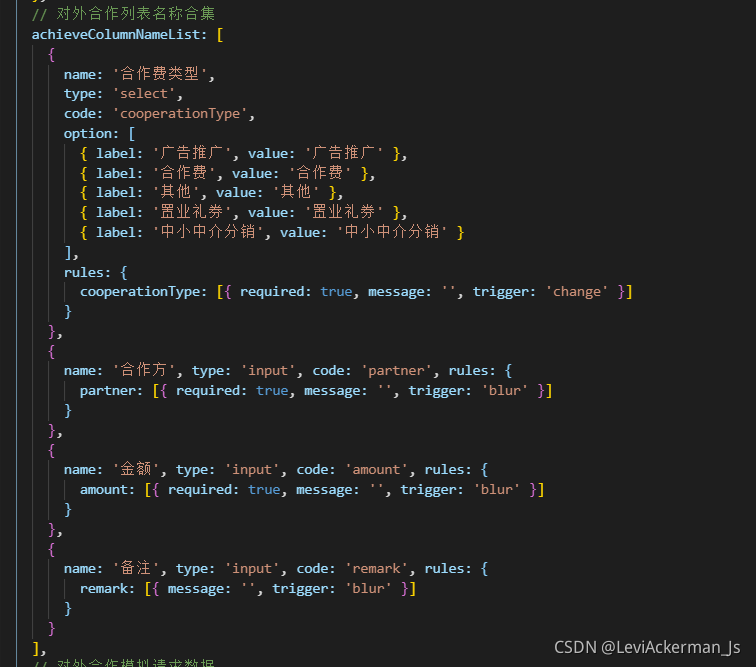