描述
思路
- 根据指定路径生成两个file对象
File start = new File("E:\\pjava");
File end = new File("F:\\");
- 实现删除方法 void move(File start, File end),传入要操作的两个文件对象,获取源文件的文件对象列表,和排序文件列表为空的情况
File[] sFile = start.listFiles();
if(sFile == null) return;
- 遍历文件对象列表,如果是文件的话,就接着使用I/O流,将相关文件复制到目的路径下
if(file.isFile()){
//在end路径下生成一个相同的文件
File file1 = new File(end, file.getName());
//创建i/o流
FileInputStream rStream = null;
FileOutputStream eStream = null;
//获得文件名
try {
rStream = new FileInputStream(file.getPath());
eStream = new FileOutputStream(file1.getPath());
//写入数据
int len;
//每次读1024kb的十倍的长度
byte[] bys = new byte[1024*10];
while((len = rStream.read(bys)) != -1 ){
//往目标文件写入数据
eStream.write(bys);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
- 是文件夹,就在目的路径下生成对应文件夹
/是文件夹就继续搬动,并创建文件夹
String name = file.getName();
String ePath = end.getPath() + "/" + name;
File f = new File(ePath);
//创建文件夹
f.mkdir();
- 递归该文件夹下的文件,复制到目的路径下对应的文件夹
move(file,end);
import java.io.*;
public class file_test2_copy {
public static void main(String[] args) throws IOException {
File start = new File("E:\\pjava");
File end = new File("F:\\");
move(start,end);
}
private static void move(File start, File end) {
File[] sFile = start.listFiles();
if(sFile == null) return;
//创建原文件夹
for (File file : sFile) {
//该文件对象是文件
//往文件输入内容
if(file.isFile()){
//在end路径下生成一个相同的文件
File file1 = new File(end, file.getName());
//创建i/o流
FileInputStream rStream = null;
FileOutputStream eStream = null;
//获得文件名
try {
rStream = new FileInputStream(file.getPath());
eStream = new FileOutputStream(file1.getPath());
//写入数据
int len;
byte[] bys = new byte[1024*10];
while((len = rStream.read(bys)) != -1 ){
//往目标文件写入数据
eStream.write(bys);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}finally {
if(rStream != null){
try {
rStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(eStream != null){
try {
eStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}else{
//是文件夹就继续搬动,并创建文件夹
String name = file.getName();
String ePath = end.getPath() + "/" + name;
File f = new File(ePath);
//创建文件夹
f.mkdir();
//递归循环
move(file,end);
}
}
}
}
- 结果
- 运行前
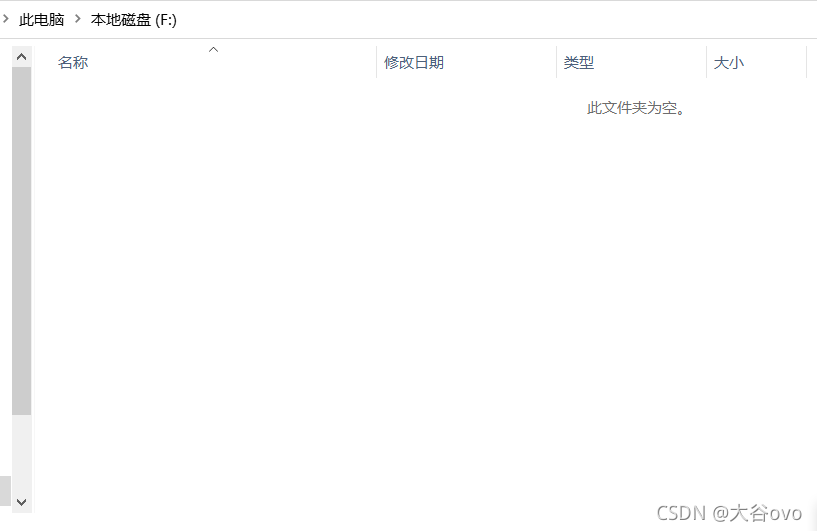
- 运行后
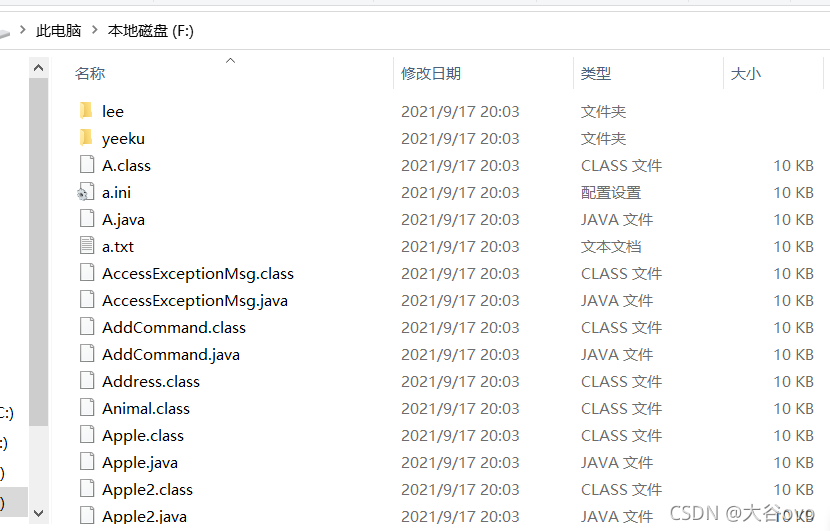
注意
- 输出输入流,要看谁是主体
- 这里我们写的test源文件是主体,从外面读文件进test源文件是输入流
- 输入流的是read()方法,获得的是byte字节数据,是一串数字
- 从test源文件写数据到外面文件是输出流
- 输出流的是write()方法,是传入字节数据,将byte字节数据写入到相关文件中去,在写入时,会转换为它本来代表的含义