using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 打飞机
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//项目的整体规划
//1.界面
//2.战机控制
//3.敌机生成器
//4.游戏逻辑控制
//打字游戏 示例
//1.设计背景
//2.字母随机生成
int x = 0;
Timer Createtime = new Timer();
Timer flyTime = new Timer();
private void Form1_Load(object sender, EventArgs e)
{
this.Size = new Size(1920, 1000);
this.BackColor = Color.Green;
this.Left = Screen.PrimaryScreen.WorkingArea.Width / 2 - this.Width / 2;
this.Top = Screen.PrimaryScreen.WorkingArea.Height / 2 - this.Height / 2;
//创建游戏区
BG.Width = 1500;
BG.Height = 900;
BG.BackColor = Color.White;
this.Controls.Add(BG);
BG.Location = new Point(20,30);
//字母生成器CreenTime
Createtime.Interval = 750;
Createtime.Tick += Createtime_Tick;
Createtime.Start();
//控制字母移动flyTime
flyTime.Interval = 10;
flyTime.Tick += FlyTime_Tick;
flyTime.Start();
//飞机先搞出来
player.Size = new Size(80,80);
player.Left = BG.Width / 2 - player.Width / 2;
player.Top = BG.Height - player.Height;
player.Image = Image.FromFile(@"../../imge/feiji.jpg");
player.SizeMode = PictureBoxSizeMode.StretchImage;
player.Tag = "plan";
BG.Controls.Add(player);
this.KeyPress += Form1_KeyPress;
//计分板
fs.Text = 0+"分";
fs.Font = new Font("",20);
fs.Left = BG.Width + 180;
fs.Top += 200;
fs.BackColor = Color.Pink;
fs.Size = new Size(100,40);
fs.AutoSize = true;
this.Controls.Add(fs);
Createtime.Stop();
flyTime.Stop();
//开始按钮
label1.Text = "开始游戏";
label1.Left = BG.Width + 180;
label1.Font = new Font("", 20);
//记录掉落
jl.Text = 0 + "个";
jl.Font = new Font("", 20);
jl.Left = BG.Width + 180;
jl.Top += 300;
jl.BackColor = Color.Pink;
jl.Size = new Size(100, 40);
jl.AutoSize = true;
this.Controls.Add(jl);
}
private void label1_Click(object sender, EventArgs e)
{
//Label cs = (Label)sender; 记录事件发起者 是谁 找到其 属于局部到局部
if (label1.Text == "开始游戏")
{
Createtime.Start();
flyTime.Start();
label1.Text = "暂停";
}
else if (label1.Text == "暂停")
{
Createtime.Stop();
flyTime.Stop();
label1.Text = "开始游戏";
}
}
Label jl = new Label();//记录
PictureBox player = new PictureBox();//飞机
Panel BG = new Panel(); //游戏界面
Random r = new Random();//字母
Label fs = new Label();//分数
int y = 0;//记录掉落的的字母
Label cx = new Label();//重新开始
private void Createtime_Tick(object sender, EventArgs e)
{//lb是字母控件
Label lb = new Label();
lb.Tag = "zimu";
lb.Text = ((char)r.Next(97,123)).ToString();
lb.Font = new Font("",r.Next(20,35));
lb.ForeColor = Color.FromArgb(r.Next(255),r.Next(255),r.Next(255));
lb.Top = 0;
lb.AutoSize = true;//设置大小为字体大小
lb.Left = r.Next(0,BG.Width-lb.Width);
lb.BackColor = Color.Transparent;//透明色
BG.Controls.Add(lb);
}
//按键单击
private void Form1_KeyPress(object sender, KeyPressEventArgs e)
{
//事件2,e参数,e事件触发者对象
//e.KeyChar
foreach (Control item in BG.Controls)
{
//判断按键和那个字母对应
if (item.Text==e.KeyChar.ToString()&&item.Tag.ToString()=="zimu")
{
item.Tag = "biaoji";
//则先控制飞机在字母正下方
player.Left = item.Left + item.Width / 2 - player.Width / 2;
//创建子弹
PictureBox bullet = new PictureBox();
bullet.Size = new Size(6,30);
bullet.Tag = "zd";
bullet.Image = Image.FromFile(@"../../imge/Ammo1.png");
bullet.SizeMode = PictureBoxSizeMode.StretchImage;
bullet.Left = player.Left + player.Width / 2 - bullet.Width / 2;
bullet.Top = player.Top - bullet.Height;
BG.Controls.Add(bullet);
//跳出当前的事件,return以下的事件中的代码全部无效
return;
}
}
}
//控制字母移动
private void FlyTime_Tick(object sender, EventArgs e)
{
foreach (Control item in BG.Controls)
{
if (item.Tag.ToString() == "zimu" || item.Tag.ToString() == "biaoji")
{
item.Top += 2;
if (item.Top >= BG.Height)
{
y++;
jl.Text = y.ToString()+"个";
if (y>=5)
{
Createtime.Stop();
flyTime.Stop();
MessageBox.Show("请重新开始");
}
item.Dispose();
}
}
//item的tag是zd则这个itme是子弹
if (item.Tag.ToString() == "zd")
{
item.Top -= 8;
if (item.Top < item.Height)
{
item.Dispose();
}
foreach (Control zm in BG.Controls)
{
if (zm.Tag.ToString() == "biaoji") //改变其tag
{
if (item.Top <= zm.Top + zm.Height && item.Left + item.Width / 2 == zm.Left + zm.Width / 2)
{
item.Dispose(); //子弹消失
zm.Dispose();//字母消失
x+=5;
fs.Text =x.ToString()+"分";
//爆炸效果
PictureBox boBox = new PictureBox();
boBox.Tag = 0;
boBox.Size = new Size(50,50);
boBox.Location = new Point(zm.Left+zm.Width/2-boBox.Width/2,zm.Top+zm.Height/2-boBox.Height/2);
BG.Controls.Add(boBox);
boBox.Image = imageList1.Images[0];
//pictruebox中用timer来控制他的播放
Timer botimer = new Timer();
botimer.Tag = boBox;
botimer.Interval = 70;
botimer.Tick += Botimer_Tick;
botimer.Start();
}
}
}
}
}
}
private void Botimer_Tick(object sender, EventArgs e)
{
Timer bomtimer=(Timer)sender;
PictureBox picture=(PictureBox)bomtimer.Tag;
picture.Image = imageList1.Images[(int)picture.Tag];
picture.Tag = (int)picture.Tag + 1;
if ((int)picture.Tag>31)
{
bomtimer.Dispose();
picture.Dispose();
}
}
}
}
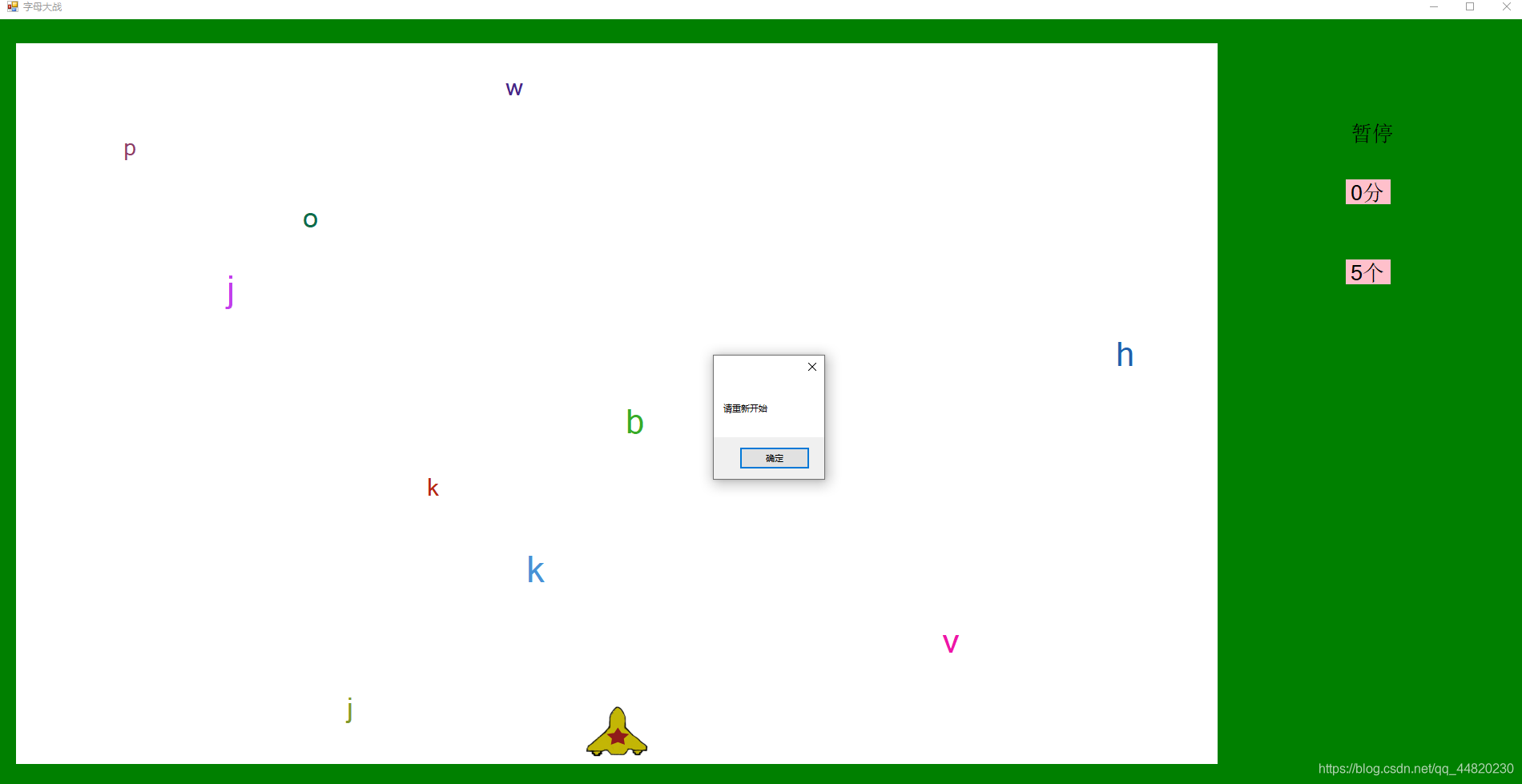